trying to render pdf in image with but getting the link path wrong
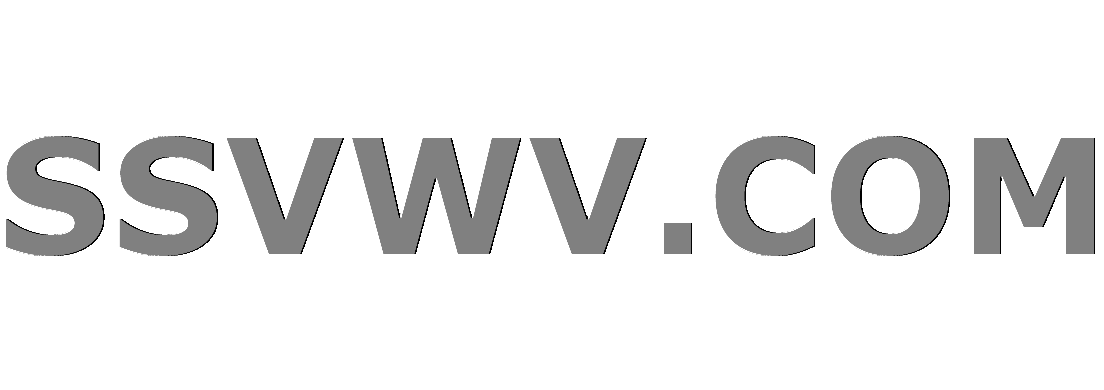
Multi tool use
up vote
0
down vote
favorite
I want to display a pdf file generated in an image folder and display it in my app. I'm using pdf.js and importing pdfjs-dist
for this purpose. So far I have been able to get the workers working and configured the webpack to load file. But I get an error with file path. No matter how I try to write the file path, I'm getting an error. I'm stuck trying to figure this out. How do I get the pdf to render?
import React, Component from "react";
import pdfjsLib from 'pdfjs-dist';
import pdfPath from '../../../../static/dist/assets/images/sampleStuff.pdf';
import ReactDOM from "react-dom";
pdfjsLib.GlobalWorkerOptions.workerSrc = '../../../../static/dist/pdf.worker.bundle.js';
class Samplepdf extends Component {
state =
file: pdfPath
componentDidMount()
const canvas = ReactDOM.findDOMNode(this.refs.myCanvas);
const ctx = canvas.getContext("2d")
const img = this.refs.image
img.onload = () =>
ctx.drawImage(img, 0, 0)
ctx.font = "20px Courier"
ctx.fillText(this.props.text, 210, 75)
render()
var loadingTask = pdfjsLib.getDocument(pdfPath);
return (
<div>
<canvas ref="myCanvas" width=640 height=425 />
<img ref="image" src= this.state.file className="hidden" />
</div>
)
webpack:
module.exports =
mode: "development",
entry:
'main': './client/reactApp/index.js',
'pdf.worker': 'pdfjs-dist/build/pdf.worker.entry'
,
output:
path: path.resolve(__dirname, "static/dist"),
filename: "[name].bundle.js"
,
watch: true,
module:
rules: [
png,
bower_components)/,
use:
loader: 'babel-loader',
options:
presets: ['@babel/preset-env', "@babel/preset-react"],
plugins: [
['@babel/plugin-proposal-object-rest-spread'],['@babel/plugin-proposal-class-properties']
]
,
test: /.css$/,
use: ["style-loader", "css-loader"]
]
reactjs
add a comment |
up vote
0
down vote
favorite
I want to display a pdf file generated in an image folder and display it in my app. I'm using pdf.js and importing pdfjs-dist
for this purpose. So far I have been able to get the workers working and configured the webpack to load file. But I get an error with file path. No matter how I try to write the file path, I'm getting an error. I'm stuck trying to figure this out. How do I get the pdf to render?
import React, Component from "react";
import pdfjsLib from 'pdfjs-dist';
import pdfPath from '../../../../static/dist/assets/images/sampleStuff.pdf';
import ReactDOM from "react-dom";
pdfjsLib.GlobalWorkerOptions.workerSrc = '../../../../static/dist/pdf.worker.bundle.js';
class Samplepdf extends Component {
state =
file: pdfPath
componentDidMount()
const canvas = ReactDOM.findDOMNode(this.refs.myCanvas);
const ctx = canvas.getContext("2d")
const img = this.refs.image
img.onload = () =>
ctx.drawImage(img, 0, 0)
ctx.font = "20px Courier"
ctx.fillText(this.props.text, 210, 75)
render()
var loadingTask = pdfjsLib.getDocument(pdfPath);
return (
<div>
<canvas ref="myCanvas" width=640 height=425 />
<img ref="image" src= this.state.file className="hidden" />
</div>
)
webpack:
module.exports =
mode: "development",
entry:
'main': './client/reactApp/index.js',
'pdf.worker': 'pdfjs-dist/build/pdf.worker.entry'
,
output:
path: path.resolve(__dirname, "static/dist"),
filename: "[name].bundle.js"
,
watch: true,
module:
rules: [
png,
bower_components)/,
use:
loader: 'babel-loader',
options:
presets: ['@babel/preset-env', "@babel/preset-react"],
plugins: [
['@babel/plugin-proposal-object-rest-spread'],['@babel/plugin-proposal-class-properties']
]
,
test: /.css$/,
use: ["style-loader", "css-loader"]
]
reactjs
You must configure the server running onlocalhost:5000
to look in the proper directory. The question is taggedreactjs
, but react does not serve static files. You have to use some kind of web server to do that.
– Håken Lid
Nov 11 at 19:43
That's exactly what it is not doing. ` import pdfPath from '../../../../static/dist/assets/images/sample.pdf';` i have tried to change the file path. But the server is looking for the files inlocalhost:5000/assets/images/samplestuff.pdf
– Deke
Nov 11 at 19:46
In your code you are importing a file calledsample.pdf
, but the error message you have included, there's a file calledsampleStuff.pdf
.
– Håken Lid
Nov 11 at 19:55
Sorry that was a typo. Despite giving the right file name, get the same error.
– Deke
Nov 11 at 19:59
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I want to display a pdf file generated in an image folder and display it in my app. I'm using pdf.js and importing pdfjs-dist
for this purpose. So far I have been able to get the workers working and configured the webpack to load file. But I get an error with file path. No matter how I try to write the file path, I'm getting an error. I'm stuck trying to figure this out. How do I get the pdf to render?
import React, Component from "react";
import pdfjsLib from 'pdfjs-dist';
import pdfPath from '../../../../static/dist/assets/images/sampleStuff.pdf';
import ReactDOM from "react-dom";
pdfjsLib.GlobalWorkerOptions.workerSrc = '../../../../static/dist/pdf.worker.bundle.js';
class Samplepdf extends Component {
state =
file: pdfPath
componentDidMount()
const canvas = ReactDOM.findDOMNode(this.refs.myCanvas);
const ctx = canvas.getContext("2d")
const img = this.refs.image
img.onload = () =>
ctx.drawImage(img, 0, 0)
ctx.font = "20px Courier"
ctx.fillText(this.props.text, 210, 75)
render()
var loadingTask = pdfjsLib.getDocument(pdfPath);
return (
<div>
<canvas ref="myCanvas" width=640 height=425 />
<img ref="image" src= this.state.file className="hidden" />
</div>
)
webpack:
module.exports =
mode: "development",
entry:
'main': './client/reactApp/index.js',
'pdf.worker': 'pdfjs-dist/build/pdf.worker.entry'
,
output:
path: path.resolve(__dirname, "static/dist"),
filename: "[name].bundle.js"
,
watch: true,
module:
rules: [
png,
bower_components)/,
use:
loader: 'babel-loader',
options:
presets: ['@babel/preset-env', "@babel/preset-react"],
plugins: [
['@babel/plugin-proposal-object-rest-spread'],['@babel/plugin-proposal-class-properties']
]
,
test: /.css$/,
use: ["style-loader", "css-loader"]
]
reactjs
I want to display a pdf file generated in an image folder and display it in my app. I'm using pdf.js and importing pdfjs-dist
for this purpose. So far I have been able to get the workers working and configured the webpack to load file. But I get an error with file path. No matter how I try to write the file path, I'm getting an error. I'm stuck trying to figure this out. How do I get the pdf to render?
import React, Component from "react";
import pdfjsLib from 'pdfjs-dist';
import pdfPath from '../../../../static/dist/assets/images/sampleStuff.pdf';
import ReactDOM from "react-dom";
pdfjsLib.GlobalWorkerOptions.workerSrc = '../../../../static/dist/pdf.worker.bundle.js';
class Samplepdf extends Component {
state =
file: pdfPath
componentDidMount()
const canvas = ReactDOM.findDOMNode(this.refs.myCanvas);
const ctx = canvas.getContext("2d")
const img = this.refs.image
img.onload = () =>
ctx.drawImage(img, 0, 0)
ctx.font = "20px Courier"
ctx.fillText(this.props.text, 210, 75)
render()
var loadingTask = pdfjsLib.getDocument(pdfPath);
return (
<div>
<canvas ref="myCanvas" width=640 height=425 />
<img ref="image" src= this.state.file className="hidden" />
</div>
)
webpack:
module.exports =
mode: "development",
entry:
'main': './client/reactApp/index.js',
'pdf.worker': 'pdfjs-dist/build/pdf.worker.entry'
,
output:
path: path.resolve(__dirname, "static/dist"),
filename: "[name].bundle.js"
,
watch: true,
module:
rules: [
png,
bower_components)/,
use:
loader: 'babel-loader',
options:
presets: ['@babel/preset-env', "@babel/preset-react"],
plugins: [
['@babel/plugin-proposal-object-rest-spread'],['@babel/plugin-proposal-class-properties']
]
,
test: /.css$/,
use: ["style-loader", "css-loader"]
]
reactjs
reactjs
edited Nov 11 at 19:58
asked Nov 11 at 19:39
Deke
1,25611121
1,25611121
You must configure the server running onlocalhost:5000
to look in the proper directory. The question is taggedreactjs
, but react does not serve static files. You have to use some kind of web server to do that.
– Håken Lid
Nov 11 at 19:43
That's exactly what it is not doing. ` import pdfPath from '../../../../static/dist/assets/images/sample.pdf';` i have tried to change the file path. But the server is looking for the files inlocalhost:5000/assets/images/samplestuff.pdf
– Deke
Nov 11 at 19:46
In your code you are importing a file calledsample.pdf
, but the error message you have included, there's a file calledsampleStuff.pdf
.
– Håken Lid
Nov 11 at 19:55
Sorry that was a typo. Despite giving the right file name, get the same error.
– Deke
Nov 11 at 19:59
add a comment |
You must configure the server running onlocalhost:5000
to look in the proper directory. The question is taggedreactjs
, but react does not serve static files. You have to use some kind of web server to do that.
– Håken Lid
Nov 11 at 19:43
That's exactly what it is not doing. ` import pdfPath from '../../../../static/dist/assets/images/sample.pdf';` i have tried to change the file path. But the server is looking for the files inlocalhost:5000/assets/images/samplestuff.pdf
– Deke
Nov 11 at 19:46
In your code you are importing a file calledsample.pdf
, but the error message you have included, there's a file calledsampleStuff.pdf
.
– Håken Lid
Nov 11 at 19:55
Sorry that was a typo. Despite giving the right file name, get the same error.
– Deke
Nov 11 at 19:59
You must configure the server running on
localhost:5000
to look in the proper directory. The question is tagged reactjs
, but react does not serve static files. You have to use some kind of web server to do that.– Håken Lid
Nov 11 at 19:43
You must configure the server running on
localhost:5000
to look in the proper directory. The question is tagged reactjs
, but react does not serve static files. You have to use some kind of web server to do that.– Håken Lid
Nov 11 at 19:43
That's exactly what it is not doing. ` import pdfPath from '../../../../static/dist/assets/images/sample.pdf';` i have tried to change the file path. But the server is looking for the files in
localhost:5000/assets/images/samplestuff.pdf
– Deke
Nov 11 at 19:46
That's exactly what it is not doing. ` import pdfPath from '../../../../static/dist/assets/images/sample.pdf';` i have tried to change the file path. But the server is looking for the files in
localhost:5000/assets/images/samplestuff.pdf
– Deke
Nov 11 at 19:46
In your code you are importing a file called
sample.pdf
, but the error message you have included, there's a file called sampleStuff.pdf
.– Håken Lid
Nov 11 at 19:55
In your code you are importing a file called
sample.pdf
, but the error message you have included, there's a file called sampleStuff.pdf
.– Håken Lid
Nov 11 at 19:55
Sorry that was a typo. Despite giving the right file name, get the same error.
– Deke
Nov 11 at 19:59
Sorry that was a typo. Despite giving the right file name, get the same error.
– Deke
Nov 11 at 19:59
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53252487%2ftrying-to-render-pdf-in-image-with-but-getting-the-link-path-wrong%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53252487%2ftrying-to-render-pdf-in-image-with-but-getting-the-link-path-wrong%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xEf,05v0jQWp1Y,sXg,w 05XZUpDMnge,QpZ6t3j dwJ7hxIx6s hP lbroGB7Dya3
You must configure the server running on
localhost:5000
to look in the proper directory. The question is taggedreactjs
, but react does not serve static files. You have to use some kind of web server to do that.– Håken Lid
Nov 11 at 19:43
That's exactly what it is not doing. ` import pdfPath from '../../../../static/dist/assets/images/sample.pdf';` i have tried to change the file path. But the server is looking for the files in
localhost:5000/assets/images/samplestuff.pdf
– Deke
Nov 11 at 19:46
In your code you are importing a file called
sample.pdf
, but the error message you have included, there's a file calledsampleStuff.pdf
.– Håken Lid
Nov 11 at 19:55
Sorry that was a typo. Despite giving the right file name, get the same error.
– Deke
Nov 11 at 19:59