props property not seen in react component class
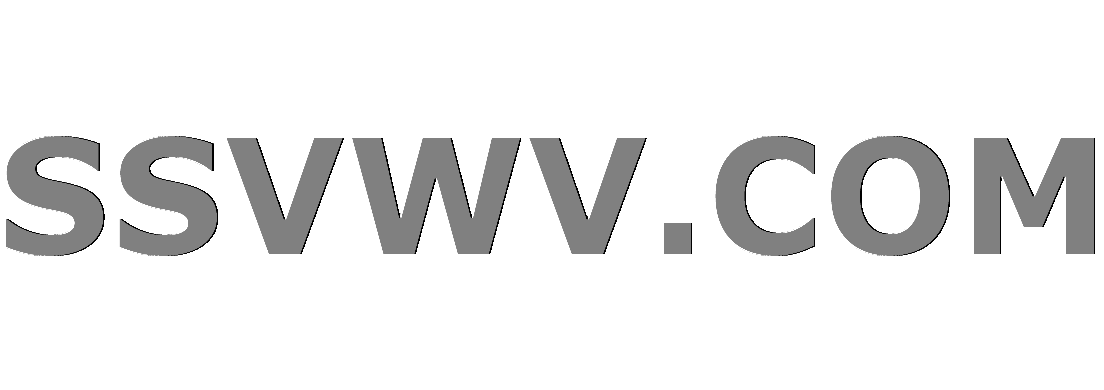
Multi tool use
I am starting to learn react and am currently using Visual Studio 2017 to code a basic hello world web app. My index.html file is as follows:
<body>
<div id="root"></div>
<!-- scripts -->
<script src="./dist/app-bundle.js"></script>
</body>
while my app.tsx file is as follows (app.tsx is transpiled to ./dist/app-bundle.js):
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to dsdasd!!</h1>
);
ReactDOM.render(<Hello />, document.getElementById('root'));
The above two files work fine. However, when I try to use the props property (shown below in the modified app.tsx) in the Hello class, the transpiler shows errors,
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to this.props.message!!</h1>
);
ReactDOM.render(<Hello message = "some message"/>, document.getElementById('root'));
ERROR: TS2339: Property 'message' does not exist on type '()'
How can I resolve this error? My understanding is that the props and state are always available in a react component. Any help is greatly appreciated.
javascript reactjs
add a comment |
I am starting to learn react and am currently using Visual Studio 2017 to code a basic hello world web app. My index.html file is as follows:
<body>
<div id="root"></div>
<!-- scripts -->
<script src="./dist/app-bundle.js"></script>
</body>
while my app.tsx file is as follows (app.tsx is transpiled to ./dist/app-bundle.js):
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to dsdasd!!</h1>
);
ReactDOM.render(<Hello />, document.getElementById('root'));
The above two files work fine. However, when I try to use the props property (shown below in the modified app.tsx) in the Hello class, the transpiler shows errors,
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to this.props.message!!</h1>
);
ReactDOM.render(<Hello message = "some message"/>, document.getElementById('root'));
ERROR: TS2339: Property 'message' does not exist on type '()'
How can I resolve this error? My understanding is that the props and state are always available in a react component. Any help is greatly appreciated.
javascript reactjs
Have you tried removing the spaces before and after=
that has come in the last line?
– Masious
Nov 14 '18 at 1:59
@Masious, I did that but the error remains ... do spaces matter?
– mettleap
Nov 14 '18 at 16:41
add a comment |
I am starting to learn react and am currently using Visual Studio 2017 to code a basic hello world web app. My index.html file is as follows:
<body>
<div id="root"></div>
<!-- scripts -->
<script src="./dist/app-bundle.js"></script>
</body>
while my app.tsx file is as follows (app.tsx is transpiled to ./dist/app-bundle.js):
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to dsdasd!!</h1>
);
ReactDOM.render(<Hello />, document.getElementById('root'));
The above two files work fine. However, when I try to use the props property (shown below in the modified app.tsx) in the Hello class, the transpiler shows errors,
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to this.props.message!!</h1>
);
ReactDOM.render(<Hello message = "some message"/>, document.getElementById('root'));
ERROR: TS2339: Property 'message' does not exist on type '()'
How can I resolve this error? My understanding is that the props and state are always available in a react component. Any help is greatly appreciated.
javascript reactjs
I am starting to learn react and am currently using Visual Studio 2017 to code a basic hello world web app. My index.html file is as follows:
<body>
<div id="root"></div>
<!-- scripts -->
<script src="./dist/app-bundle.js"></script>
</body>
while my app.tsx file is as follows (app.tsx is transpiled to ./dist/app-bundle.js):
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to dsdasd!!</h1>
);
ReactDOM.render(<Hello />, document.getElementById('root'));
The above two files work fine. However, when I try to use the props property (shown below in the modified app.tsx) in the Hello class, the transpiler shows errors,
declare var require: any
var React = require('react');
var ReactDOM = require('react-dom');
class Hello extends React.Component
render()
return (
<h1>Welcome to this.props.message!!</h1>
);
ReactDOM.render(<Hello message = "some message"/>, document.getElementById('root'));
ERROR: TS2339: Property 'message' does not exist on type '()'
How can I resolve this error? My understanding is that the props and state are always available in a react component. Any help is greatly appreciated.
javascript reactjs
javascript reactjs
asked Nov 13 '18 at 22:24
mettleapmettleap
1,080316
1,080316
Have you tried removing the spaces before and after=
that has come in the last line?
– Masious
Nov 14 '18 at 1:59
@Masious, I did that but the error remains ... do spaces matter?
– mettleap
Nov 14 '18 at 16:41
add a comment |
Have you tried removing the spaces before and after=
that has come in the last line?
– Masious
Nov 14 '18 at 1:59
@Masious, I did that but the error remains ... do spaces matter?
– mettleap
Nov 14 '18 at 16:41
Have you tried removing the spaces before and after
=
that has come in the last line?– Masious
Nov 14 '18 at 1:59
Have you tried removing the spaces before and after
=
that has come in the last line?– Masious
Nov 14 '18 at 1:59
@Masious, I did that but the error remains ... do spaces matter?
– mettleap
Nov 14 '18 at 16:41
@Masious, I did that but the error remains ... do spaces matter?
– mettleap
Nov 14 '18 at 16:41
add a comment |
1 Answer
1
active
oldest
votes
You need to specify the props interface for a Typescript + React component:
interface Props
message: string;
class Hello extends React.Component<Props>
render()
return (
<h1>Welcome to this.props.message</h1>
);
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@mettleap The problem is that you userequire
.require
should be used only when you want modules to be type-unsafe. Useimport
.
– estus
Nov 14 '18 at 17:03
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290435%2fprops-property-not-seen-in-react-component-class%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to specify the props interface for a Typescript + React component:
interface Props
message: string;
class Hello extends React.Component<Props>
render()
return (
<h1>Welcome to this.props.message</h1>
);
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@mettleap The problem is that you userequire
.require
should be used only when you want modules to be type-unsafe. Useimport
.
– estus
Nov 14 '18 at 17:03
add a comment |
You need to specify the props interface for a Typescript + React component:
interface Props
message: string;
class Hello extends React.Component<Props>
render()
return (
<h1>Welcome to this.props.message</h1>
);
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@mettleap The problem is that you userequire
.require
should be used only when you want modules to be type-unsafe. Useimport
.
– estus
Nov 14 '18 at 17:03
add a comment |
You need to specify the props interface for a Typescript + React component:
interface Props
message: string;
class Hello extends React.Component<Props>
render()
return (
<h1>Welcome to this.props.message</h1>
);
You need to specify the props interface for a Typescript + React component:
interface Props
message: string;
class Hello extends React.Component<Props>
render()
return (
<h1>Welcome to this.props.message</h1>
);
answered Nov 13 '18 at 22:32


Danny DelottDanny Delott
3,43111537
3,43111537
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@mettleap The problem is that you userequire
.require
should be used only when you want modules to be type-unsafe. Useimport
.
– estus
Nov 14 '18 at 17:03
add a comment |
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@mettleap The problem is that you userequire
.require
should be used only when you want modules to be type-unsafe. Useimport
.
– estus
Nov 14 '18 at 17:03
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
Thank you for the answer. However, I still get the same error ... is there anything else that I might be missing?
– mettleap
Nov 13 '18 at 22:40
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@estus, this does seem to be correct ... though I did recompile the project and the error still remains
– mettleap
Nov 14 '18 at 16:43
@mettleap The problem is that you use
require
. require
should be used only when you want modules to be type-unsafe. Use import
.– estus
Nov 14 '18 at 17:03
@mettleap The problem is that you use
require
. require
should be used only when you want modules to be type-unsafe. Use import
.– estus
Nov 14 '18 at 17:03
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290435%2fprops-property-not-seen-in-react-component-class%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6prtERFn BFH,dSb,6OoY3v1vtgxvhpUJf1aLI66NqgLoOUY6j,x c11D ZK67565BFZzbtnCM7R75
Have you tried removing the spaces before and after
=
that has come in the last line?– Masious
Nov 14 '18 at 1:59
@Masious, I did that but the error remains ... do spaces matter?
– mettleap
Nov 14 '18 at 16:41