Ruby how to return index of a pair
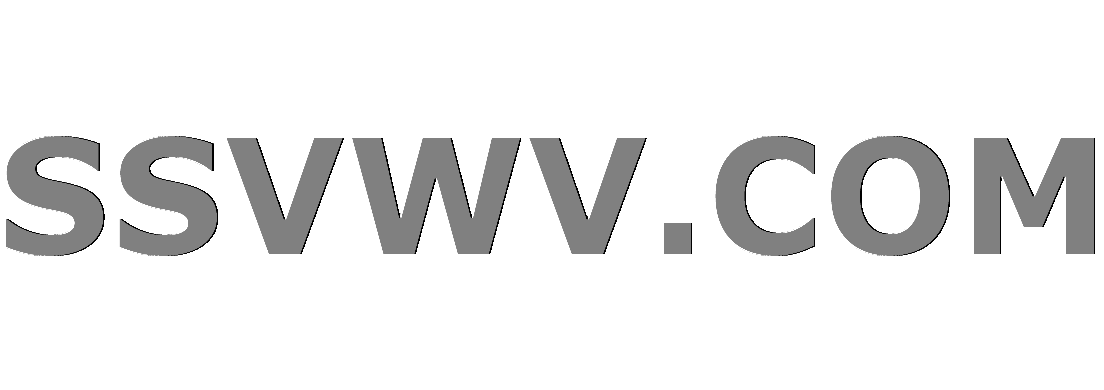
Multi tool use
up vote
0
down vote
favorite
Today I got a task with given array and 'target' which is a sum of 2 integers within that list. After some time I came out with draft solution but it does not seem to be passing all of the tests. Algorithm seems to be considering integer at [0] twice.
def two_sum(numbers, target)
numbers.combination 2 do |a, b|
if a + b == target
return numbers.index(a), numbers.index(b)
end
end
end
print two_sum([1, 2, 3], 4) # Expected [0, 2] *OK
print two_sum([1234, 5678, 9012], 14690) # Expected [1, 2] *OK
print two_sum([2, 2, 3], 4) # Expected [0, 1]) but I get [0, 0]
I have tried to use .map first instead of .combination(2) method but with the exact same result :-/
ruby algorithm math indexing
add a comment |
up vote
0
down vote
favorite
Today I got a task with given array and 'target' which is a sum of 2 integers within that list. After some time I came out with draft solution but it does not seem to be passing all of the tests. Algorithm seems to be considering integer at [0] twice.
def two_sum(numbers, target)
numbers.combination 2 do |a, b|
if a + b == target
return numbers.index(a), numbers.index(b)
end
end
end
print two_sum([1, 2, 3], 4) # Expected [0, 2] *OK
print two_sum([1234, 5678, 9012], 14690) # Expected [1, 2] *OK
print two_sum([2, 2, 3], 4) # Expected [0, 1]) but I get [0, 0]
I have tried to use .map first instead of .combination(2) method but with the exact same result :-/
ruby algorithm math indexing
4
Hint: What is[2,2,3].index(2)
? And you need to learn how to use a debugger to step through your code and watch what it is doing.
– mu is too short
Nov 11 at 22:23
Use the index for the combination
– Lenin Raj Rajasekaran
Nov 11 at 22:29
Thanks guys. 'you need to learn how to use a debugger to step through your code and watch what it is doing' - noted
– Krzynek
Nov 11 at 22:55
1
@Krzynek : Since the question is already answered and accepted and tagged with the algorithm, you would have already moved on to the next problem, and that's fine. But looking at your 3 tests,numbers
seems a sorted list. Which means you can reduce the time-complexity of your code if you look just close enough with a different approach. Maybe do that exercise when you get some time. :)
– Surya
Nov 12 at 10:08
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Today I got a task with given array and 'target' which is a sum of 2 integers within that list. After some time I came out with draft solution but it does not seem to be passing all of the tests. Algorithm seems to be considering integer at [0] twice.
def two_sum(numbers, target)
numbers.combination 2 do |a, b|
if a + b == target
return numbers.index(a), numbers.index(b)
end
end
end
print two_sum([1, 2, 3], 4) # Expected [0, 2] *OK
print two_sum([1234, 5678, 9012], 14690) # Expected [1, 2] *OK
print two_sum([2, 2, 3], 4) # Expected [0, 1]) but I get [0, 0]
I have tried to use .map first instead of .combination(2) method but with the exact same result :-/
ruby algorithm math indexing
Today I got a task with given array and 'target' which is a sum of 2 integers within that list. After some time I came out with draft solution but it does not seem to be passing all of the tests. Algorithm seems to be considering integer at [0] twice.
def two_sum(numbers, target)
numbers.combination 2 do |a, b|
if a + b == target
return numbers.index(a), numbers.index(b)
end
end
end
print two_sum([1, 2, 3], 4) # Expected [0, 2] *OK
print two_sum([1234, 5678, 9012], 14690) # Expected [1, 2] *OK
print two_sum([2, 2, 3], 4) # Expected [0, 1]) but I get [0, 0]
I have tried to use .map first instead of .combination(2) method but with the exact same result :-/
ruby algorithm math indexing
ruby algorithm math indexing
edited Nov 11 at 22:13


Sebastian Palma
15.3k41933
15.3k41933
asked Nov 11 at 22:12
Krzynek
465
465
4
Hint: What is[2,2,3].index(2)
? And you need to learn how to use a debugger to step through your code and watch what it is doing.
– mu is too short
Nov 11 at 22:23
Use the index for the combination
– Lenin Raj Rajasekaran
Nov 11 at 22:29
Thanks guys. 'you need to learn how to use a debugger to step through your code and watch what it is doing' - noted
– Krzynek
Nov 11 at 22:55
1
@Krzynek : Since the question is already answered and accepted and tagged with the algorithm, you would have already moved on to the next problem, and that's fine. But looking at your 3 tests,numbers
seems a sorted list. Which means you can reduce the time-complexity of your code if you look just close enough with a different approach. Maybe do that exercise when you get some time. :)
– Surya
Nov 12 at 10:08
add a comment |
4
Hint: What is[2,2,3].index(2)
? And you need to learn how to use a debugger to step through your code and watch what it is doing.
– mu is too short
Nov 11 at 22:23
Use the index for the combination
– Lenin Raj Rajasekaran
Nov 11 at 22:29
Thanks guys. 'you need to learn how to use a debugger to step through your code and watch what it is doing' - noted
– Krzynek
Nov 11 at 22:55
1
@Krzynek : Since the question is already answered and accepted and tagged with the algorithm, you would have already moved on to the next problem, and that's fine. But looking at your 3 tests,numbers
seems a sorted list. Which means you can reduce the time-complexity of your code if you look just close enough with a different approach. Maybe do that exercise when you get some time. :)
– Surya
Nov 12 at 10:08
4
4
Hint: What is
[2,2,3].index(2)
? And you need to learn how to use a debugger to step through your code and watch what it is doing.– mu is too short
Nov 11 at 22:23
Hint: What is
[2,2,3].index(2)
? And you need to learn how to use a debugger to step through your code and watch what it is doing.– mu is too short
Nov 11 at 22:23
Use the index for the combination
– Lenin Raj Rajasekaran
Nov 11 at 22:29
Use the index for the combination
– Lenin Raj Rajasekaran
Nov 11 at 22:29
Thanks guys. 'you need to learn how to use a debugger to step through your code and watch what it is doing' - noted
– Krzynek
Nov 11 at 22:55
Thanks guys. 'you need to learn how to use a debugger to step through your code and watch what it is doing' - noted
– Krzynek
Nov 11 at 22:55
1
1
@Krzynek : Since the question is already answered and accepted and tagged with the algorithm, you would have already moved on to the next problem, and that's fine. But looking at your 3 tests,
numbers
seems a sorted list. Which means you can reduce the time-complexity of your code if you look just close enough with a different approach. Maybe do that exercise when you get some time. :)– Surya
Nov 12 at 10:08
@Krzynek : Since the question is already answered and accepted and tagged with the algorithm, you would have already moved on to the next problem, and that's fine. But looking at your 3 tests,
numbers
seems a sorted list. Which means you can reduce the time-complexity of your code if you look just close enough with a different approach. Maybe do that exercise when you get some time. :)– Surya
Nov 12 at 10:08
add a comment |
1 Answer
1
active
oldest
votes
up vote
5
down vote
accepted
def two_sum(numbers, target)
[*0..numbers.size-1].combination(2).find
end
two_sum([1234, 5678, 9012], 14690)
#=> [1, 2]
two_sum([1234, 5678, 9012], 14691)
#=> nil
Here is a more efficient method that may prove useful when the arrays are large.
require 'set'
def two_sum(arr, target)
if target.even?
half = target/2
first = arr.index(half)
if first
last = arr.rindex(half)
return [first, last] unless last.nil? || first == last
end
end
a1, a2 = arr.uniq.partition n
s = a2.to_set
n = a1.find
n.nil? ? nil : [arr.index(n), arr.index(target-n)]
end
If target
is even I first check to see if one-half of it appears at least twice in arr
. If so, we are finished (except for determining and returning the associated indices). Even if the method does not terminate after this step it is required this step does not result in an early termination it is required before the next steps are performed.
If target
is odd or is even but one-half of it appears less than twice in arr
I construct a temporary array that contains unique values in arr
and then partition that into two arrays, a1
, containing values no greater than target/2
and a2
, containing values greater than target/2
. It follows that if two numbers sum to target
one must be in a1
and the other must be in a2
.
To speed calculations I then convert a2
to a set s
, and then loop through a1
looking for a value n
such that s
contains target-n
. Let's try it.
arr = 100_000.times.map rand(1_000_000)
puts "target i1 arr[i1] i2 arr[i2] calc time (secs)"
puts "---------------------------------------------------------"
1000.times do
t = Time.now
target = rand(1_000_000)
i1, i2 = two_sum(arr, target)
print "#target -> "
print i1.nil? ? "nil " :
"#i1 #arr[i1] #i2 #arr[i2]"
puts " #(Time.now-t).round(4) secs"
end
prints
target i1 arr[i1] i2 arr[i2] calc time (secs)
---------------------------------------------------------
215113 -> 41 90943 11198 124170 0.027
344479 -> 0 78758 63570 265721 0.0237
188352 -> 190 79209 39912 109143 0.0275
457 -> nil 0.0255
923135 -> 78 84600 43928 838535 0.0207
59391 -> 2 5779 5454 53612 0.0289
259142 -> 73 58864 29278 200278 0.0284
364486 -> 8049 182243 89704 182243 0.001
895164 -> 13 205843 7705 689321 0.0228
880575 -> 20 440073 6195 440502 0.021
We see that arr
does not contain two numbers that sum to 457
. Also, notice the very short time in the antepenultimate row. That's because one-helf of target
(364486/2 #=> 182243
) appears at least twice in arr
.
1
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
1
[*0..numbers.size-1]
can alternatively be written(0..numbers.size-1).to_a
.
– Cary Swoveland
Nov 11 at 23:32
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53253757%2fruby-how-to-return-index-of-a-pair%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
accepted
def two_sum(numbers, target)
[*0..numbers.size-1].combination(2).find
end
two_sum([1234, 5678, 9012], 14690)
#=> [1, 2]
two_sum([1234, 5678, 9012], 14691)
#=> nil
Here is a more efficient method that may prove useful when the arrays are large.
require 'set'
def two_sum(arr, target)
if target.even?
half = target/2
first = arr.index(half)
if first
last = arr.rindex(half)
return [first, last] unless last.nil? || first == last
end
end
a1, a2 = arr.uniq.partition n
s = a2.to_set
n = a1.find
n.nil? ? nil : [arr.index(n), arr.index(target-n)]
end
If target
is even I first check to see if one-half of it appears at least twice in arr
. If so, we are finished (except for determining and returning the associated indices). Even if the method does not terminate after this step it is required this step does not result in an early termination it is required before the next steps are performed.
If target
is odd or is even but one-half of it appears less than twice in arr
I construct a temporary array that contains unique values in arr
and then partition that into two arrays, a1
, containing values no greater than target/2
and a2
, containing values greater than target/2
. It follows that if two numbers sum to target
one must be in a1
and the other must be in a2
.
To speed calculations I then convert a2
to a set s
, and then loop through a1
looking for a value n
such that s
contains target-n
. Let's try it.
arr = 100_000.times.map rand(1_000_000)
puts "target i1 arr[i1] i2 arr[i2] calc time (secs)"
puts "---------------------------------------------------------"
1000.times do
t = Time.now
target = rand(1_000_000)
i1, i2 = two_sum(arr, target)
print "#target -> "
print i1.nil? ? "nil " :
"#i1 #arr[i1] #i2 #arr[i2]"
puts " #(Time.now-t).round(4) secs"
end
prints
target i1 arr[i1] i2 arr[i2] calc time (secs)
---------------------------------------------------------
215113 -> 41 90943 11198 124170 0.027
344479 -> 0 78758 63570 265721 0.0237
188352 -> 190 79209 39912 109143 0.0275
457 -> nil 0.0255
923135 -> 78 84600 43928 838535 0.0207
59391 -> 2 5779 5454 53612 0.0289
259142 -> 73 58864 29278 200278 0.0284
364486 -> 8049 182243 89704 182243 0.001
895164 -> 13 205843 7705 689321 0.0228
880575 -> 20 440073 6195 440502 0.021
We see that arr
does not contain two numbers that sum to 457
. Also, notice the very short time in the antepenultimate row. That's because one-helf of target
(364486/2 #=> 182243
) appears at least twice in arr
.
1
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
1
[*0..numbers.size-1]
can alternatively be written(0..numbers.size-1).to_a
.
– Cary Swoveland
Nov 11 at 23:32
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
add a comment |
up vote
5
down vote
accepted
def two_sum(numbers, target)
[*0..numbers.size-1].combination(2).find
end
two_sum([1234, 5678, 9012], 14690)
#=> [1, 2]
two_sum([1234, 5678, 9012], 14691)
#=> nil
Here is a more efficient method that may prove useful when the arrays are large.
require 'set'
def two_sum(arr, target)
if target.even?
half = target/2
first = arr.index(half)
if first
last = arr.rindex(half)
return [first, last] unless last.nil? || first == last
end
end
a1, a2 = arr.uniq.partition n
s = a2.to_set
n = a1.find
n.nil? ? nil : [arr.index(n), arr.index(target-n)]
end
If target
is even I first check to see if one-half of it appears at least twice in arr
. If so, we are finished (except for determining and returning the associated indices). Even if the method does not terminate after this step it is required this step does not result in an early termination it is required before the next steps are performed.
If target
is odd or is even but one-half of it appears less than twice in arr
I construct a temporary array that contains unique values in arr
and then partition that into two arrays, a1
, containing values no greater than target/2
and a2
, containing values greater than target/2
. It follows that if two numbers sum to target
one must be in a1
and the other must be in a2
.
To speed calculations I then convert a2
to a set s
, and then loop through a1
looking for a value n
such that s
contains target-n
. Let's try it.
arr = 100_000.times.map rand(1_000_000)
puts "target i1 arr[i1] i2 arr[i2] calc time (secs)"
puts "---------------------------------------------------------"
1000.times do
t = Time.now
target = rand(1_000_000)
i1, i2 = two_sum(arr, target)
print "#target -> "
print i1.nil? ? "nil " :
"#i1 #arr[i1] #i2 #arr[i2]"
puts " #(Time.now-t).round(4) secs"
end
prints
target i1 arr[i1] i2 arr[i2] calc time (secs)
---------------------------------------------------------
215113 -> 41 90943 11198 124170 0.027
344479 -> 0 78758 63570 265721 0.0237
188352 -> 190 79209 39912 109143 0.0275
457 -> nil 0.0255
923135 -> 78 84600 43928 838535 0.0207
59391 -> 2 5779 5454 53612 0.0289
259142 -> 73 58864 29278 200278 0.0284
364486 -> 8049 182243 89704 182243 0.001
895164 -> 13 205843 7705 689321 0.0228
880575 -> 20 440073 6195 440502 0.021
We see that arr
does not contain two numbers that sum to 457
. Also, notice the very short time in the antepenultimate row. That's because one-helf of target
(364486/2 #=> 182243
) appears at least twice in arr
.
1
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
1
[*0..numbers.size-1]
can alternatively be written(0..numbers.size-1).to_a
.
– Cary Swoveland
Nov 11 at 23:32
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
add a comment |
up vote
5
down vote
accepted
up vote
5
down vote
accepted
def two_sum(numbers, target)
[*0..numbers.size-1].combination(2).find
end
two_sum([1234, 5678, 9012], 14690)
#=> [1, 2]
two_sum([1234, 5678, 9012], 14691)
#=> nil
Here is a more efficient method that may prove useful when the arrays are large.
require 'set'
def two_sum(arr, target)
if target.even?
half = target/2
first = arr.index(half)
if first
last = arr.rindex(half)
return [first, last] unless last.nil? || first == last
end
end
a1, a2 = arr.uniq.partition n
s = a2.to_set
n = a1.find
n.nil? ? nil : [arr.index(n), arr.index(target-n)]
end
If target
is even I first check to see if one-half of it appears at least twice in arr
. If so, we are finished (except for determining and returning the associated indices). Even if the method does not terminate after this step it is required this step does not result in an early termination it is required before the next steps are performed.
If target
is odd or is even but one-half of it appears less than twice in arr
I construct a temporary array that contains unique values in arr
and then partition that into two arrays, a1
, containing values no greater than target/2
and a2
, containing values greater than target/2
. It follows that if two numbers sum to target
one must be in a1
and the other must be in a2
.
To speed calculations I then convert a2
to a set s
, and then loop through a1
looking for a value n
such that s
contains target-n
. Let's try it.
arr = 100_000.times.map rand(1_000_000)
puts "target i1 arr[i1] i2 arr[i2] calc time (secs)"
puts "---------------------------------------------------------"
1000.times do
t = Time.now
target = rand(1_000_000)
i1, i2 = two_sum(arr, target)
print "#target -> "
print i1.nil? ? "nil " :
"#i1 #arr[i1] #i2 #arr[i2]"
puts " #(Time.now-t).round(4) secs"
end
prints
target i1 arr[i1] i2 arr[i2] calc time (secs)
---------------------------------------------------------
215113 -> 41 90943 11198 124170 0.027
344479 -> 0 78758 63570 265721 0.0237
188352 -> 190 79209 39912 109143 0.0275
457 -> nil 0.0255
923135 -> 78 84600 43928 838535 0.0207
59391 -> 2 5779 5454 53612 0.0289
259142 -> 73 58864 29278 200278 0.0284
364486 -> 8049 182243 89704 182243 0.001
895164 -> 13 205843 7705 689321 0.0228
880575 -> 20 440073 6195 440502 0.021
We see that arr
does not contain two numbers that sum to 457
. Also, notice the very short time in the antepenultimate row. That's because one-helf of target
(364486/2 #=> 182243
) appears at least twice in arr
.
def two_sum(numbers, target)
[*0..numbers.size-1].combination(2).find
end
two_sum([1234, 5678, 9012], 14690)
#=> [1, 2]
two_sum([1234, 5678, 9012], 14691)
#=> nil
Here is a more efficient method that may prove useful when the arrays are large.
require 'set'
def two_sum(arr, target)
if target.even?
half = target/2
first = arr.index(half)
if first
last = arr.rindex(half)
return [first, last] unless last.nil? || first == last
end
end
a1, a2 = arr.uniq.partition n
s = a2.to_set
n = a1.find
n.nil? ? nil : [arr.index(n), arr.index(target-n)]
end
If target
is even I first check to see if one-half of it appears at least twice in arr
. If so, we are finished (except for determining and returning the associated indices). Even if the method does not terminate after this step it is required this step does not result in an early termination it is required before the next steps are performed.
If target
is odd or is even but one-half of it appears less than twice in arr
I construct a temporary array that contains unique values in arr
and then partition that into two arrays, a1
, containing values no greater than target/2
and a2
, containing values greater than target/2
. It follows that if two numbers sum to target
one must be in a1
and the other must be in a2
.
To speed calculations I then convert a2
to a set s
, and then loop through a1
looking for a value n
such that s
contains target-n
. Let's try it.
arr = 100_000.times.map rand(1_000_000)
puts "target i1 arr[i1] i2 arr[i2] calc time (secs)"
puts "---------------------------------------------------------"
1000.times do
t = Time.now
target = rand(1_000_000)
i1, i2 = two_sum(arr, target)
print "#target -> "
print i1.nil? ? "nil " :
"#i1 #arr[i1] #i2 #arr[i2]"
puts " #(Time.now-t).round(4) secs"
end
prints
target i1 arr[i1] i2 arr[i2] calc time (secs)
---------------------------------------------------------
215113 -> 41 90943 11198 124170 0.027
344479 -> 0 78758 63570 265721 0.0237
188352 -> 190 79209 39912 109143 0.0275
457 -> nil 0.0255
923135 -> 78 84600 43928 838535 0.0207
59391 -> 2 5779 5454 53612 0.0289
259142 -> 73 58864 29278 200278 0.0284
364486 -> 8049 182243 89704 182243 0.001
895164 -> 13 205843 7705 689321 0.0228
880575 -> 20 440073 6195 440502 0.021
We see that arr
does not contain two numbers that sum to 457
. Also, notice the very short time in the antepenultimate row. That's because one-helf of target
(364486/2 #=> 182243
) appears at least twice in arr
.
edited Nov 13 at 17:00
answered Nov 11 at 22:28
Cary Swoveland
67.1k53865
67.1k53865
1
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
1
[*0..numbers.size-1]
can alternatively be written(0..numbers.size-1).to_a
.
– Cary Swoveland
Nov 11 at 23:32
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
add a comment |
1
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
1
[*0..numbers.size-1]
can alternatively be written(0..numbers.size-1).to_a
.
– Cary Swoveland
Nov 11 at 23:32
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
1
1
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
What a nice place not to use an exclusive range :)
– Marcin Kołodziej
Nov 11 at 22:34
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
Magic! Now is time to go back to ruby-docs to make sure I completely understand this bit " [*0..numbers.size-1] "
– Krzynek
Nov 11 at 22:51
1
1
[*0..numbers.size-1]
can alternatively be written (0..numbers.size-1).to_a
.– Cary Swoveland
Nov 11 at 23:32
[*0..numbers.size-1]
can alternatively be written (0..numbers.size-1).to_a
.– Cary Swoveland
Nov 11 at 23:32
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
Krzynek, I modified my answer.
– Cary Swoveland
Nov 13 at 1:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53253757%2fruby-how-to-return-index-of-a-pair%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HBSIAnFZ4 cZb7Xts3SV rBK djXeWjTmr0 v kd4 Tpi
4
Hint: What is
[2,2,3].index(2)
? And you need to learn how to use a debugger to step through your code and watch what it is doing.– mu is too short
Nov 11 at 22:23
Use the index for the combination
– Lenin Raj Rajasekaran
Nov 11 at 22:29
Thanks guys. 'you need to learn how to use a debugger to step through your code and watch what it is doing' - noted
– Krzynek
Nov 11 at 22:55
1
@Krzynek : Since the question is already answered and accepted and tagged with the algorithm, you would have already moved on to the next problem, and that's fine. But looking at your 3 tests,
numbers
seems a sorted list. Which means you can reduce the time-complexity of your code if you look just close enough with a different approach. Maybe do that exercise when you get some time. :)– Surya
Nov 12 at 10:08