PHP - preg_replace on an array not working as expected
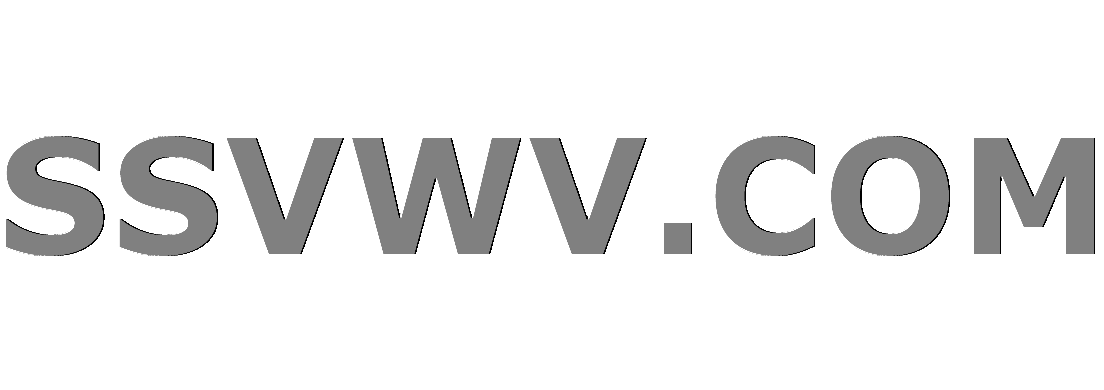
Multi tool use
up vote
-1
down vote
favorite
I have a PHP array that looks like this..
Array
(
[0] => post: 746
[1] => post: 2
[2] => post: 84
)
I am trying to remove the post:
from each item in the array and return one that looks like this...
Array
(
[0] => 746
[1] => 2
[2] => 84
)
I have attempted to use preg_replace like this...
$array = preg_replace('/^post: *([0-9]+)/', $array );
print_r($array);
But this is not working for me, how should I be doing this?
php
add a comment |
up vote
-1
down vote
favorite
I have a PHP array that looks like this..
Array
(
[0] => post: 746
[1] => post: 2
[2] => post: 84
)
I am trying to remove the post:
from each item in the array and return one that looks like this...
Array
(
[0] => 746
[1] => 2
[2] => 84
)
I have attempted to use preg_replace like this...
$array = preg_replace('/^post: *([0-9]+)/', $array );
print_r($array);
But this is not working for me, how should I be doing this?
php
1
preg_replace()
requires three parameters. You are using only 2. This will give syntax errors
– Madhur Bhaiya
Nov 11 at 17:19
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I have a PHP array that looks like this..
Array
(
[0] => post: 746
[1] => post: 2
[2] => post: 84
)
I am trying to remove the post:
from each item in the array and return one that looks like this...
Array
(
[0] => 746
[1] => 2
[2] => 84
)
I have attempted to use preg_replace like this...
$array = preg_replace('/^post: *([0-9]+)/', $array );
print_r($array);
But this is not working for me, how should I be doing this?
php
I have a PHP array that looks like this..
Array
(
[0] => post: 746
[1] => post: 2
[2] => post: 84
)
I am trying to remove the post:
from each item in the array and return one that looks like this...
Array
(
[0] => 746
[1] => 2
[2] => 84
)
I have attempted to use preg_replace like this...
$array = preg_replace('/^post: *([0-9]+)/', $array );
print_r($array);
But this is not working for me, how should I be doing this?
php
php
edited Nov 11 at 17:15
gview
10.9k22639
10.9k22639
asked Nov 11 at 17:14
fightstarr20
2,7141148104
2,7141148104
1
preg_replace()
requires three parameters. You are using only 2. This will give syntax errors
– Madhur Bhaiya
Nov 11 at 17:19
add a comment |
1
preg_replace()
requires three parameters. You are using only 2. This will give syntax errors
– Madhur Bhaiya
Nov 11 at 17:19
1
1
preg_replace()
requires three parameters. You are using only 2. This will give syntax errors– Madhur Bhaiya
Nov 11 at 17:19
preg_replace()
requires three parameters. You are using only 2. This will give syntax errors– Madhur Bhaiya
Nov 11 at 17:19
add a comment |
5 Answers
5
active
oldest
votes
up vote
1
down vote
You've missed the second argument of preg_replace
function, which is with what should replace the match, also your regex has small problem, here is the fixed version:
preg_replace('/^post:s*([0-9]+)$/', '$1', $array );
Demo: https://3v4l.org/64fO6
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
1
@fightstarr20 when catching white spaces you should uses
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks
– shaddy
Nov 11 at 17:30
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
add a comment |
up vote
0
down vote
You don't have a pattern for the replacement, or a empty string place holder.
mixed preg_replace ( mixed $pattern , mixed $replacement , mixed $subject)
Is what you are trying to do (there are other args, but they are optional).
$array = preg_replace('/post: /', '', $array );
Should do it.
<?php
$array=array("post: 746",
"post: 2",
"post: 84");
$array = preg_replace('/^post: /', '', $array );
print_r($array);
?>
Array
(
[0] => 746
[1] => 2
[2] => 84
)
add a comment |
up vote
0
down vote
You could do this without using a regex using array_map and substr to check the prefix and return the string without the prefix:
$items = [
"post: 674",
"post: 2",
"post: 84",
];
$result = array_map(function($x)
$prefix = "post: ";
if (substr($x, 0, strlen($prefix)) == $prefix)
return substr($x, strlen($prefix));
return $x;
, $items);
print_r($result);
Result:
Array
(
[0] => 674
[1] => 2
[2] => 84
)
add a comment |
up vote
0
down vote
There are many ways to do this that don't involve regular expressions, which are really not needed for breaking up a simple string like this.
For example:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
$o = explode(': ', $n);
return (int)$o[1];
, $input);
var_dump($output);
And here's another one that is probably even faster:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
return (int)substr($n, strpos($n, ':')+1);
, $input);
var_dump($output);
If you don't need integers in the output just remove the cast to int.
Or just use str_replace, which in many cases like this is a drop in replacement for preg_replace.
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = str_replace('post: ', '', $input);
var_dump($output);
add a comment |
up vote
0
down vote
You can use array_map()
to iterate the array then strip out any non-digital characters via filter_var()
with FILTER_SANITIZE_NUMBER_INT
or trim()
with a "character mask" containing the six undesired characters.
You can also let preg_replace()
do the iterating for you. Using preg_replace()
offers the most brief syntax, but regular expressions are often slower than non-preg_ techniques and it may be overkill for your seemingly simple task.
Codes: (Demo)
$array = ["post: 746", "post: 2", "post: 84"];
// remove all non-integer characters
var_export(array_map(function($v)return filter_var($v, FILTER_SANITIZE_NUMBER_INT);, $array));
// only necessary if you have elements with non-"post: " AND non-integer substrings
var_export(preg_replace('~^post: ~', '', $array));
// I shuffled the character mask to prove order doesn't matter
var_export(array_map(function($v)return trim($v, ': opst');, $array));
Output: (from each technique is the same)
array (
0 => '746',
1 => '2',
2 => '84',
)
p.s. If anyone is going to entertain the idea of using explode()
to create an array of each element then store the second element of the array as the new desired string (and I wouldn't go to such trouble) be sure to:
- split on
or
:
(colon, space) or evenpost:
(post, colon, space) because splitting on:
(colon only) forces you to tidy up the second element's leading space and - use
explode()
's 3rd parameter (limit
) and set it to2
because logically, you don't need more than two elements
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You've missed the second argument of preg_replace
function, which is with what should replace the match, also your regex has small problem, here is the fixed version:
preg_replace('/^post:s*([0-9]+)$/', '$1', $array );
Demo: https://3v4l.org/64fO6
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
1
@fightstarr20 when catching white spaces you should uses
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks
– shaddy
Nov 11 at 17:30
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
add a comment |
up vote
1
down vote
You've missed the second argument of preg_replace
function, which is with what should replace the match, also your regex has small problem, here is the fixed version:
preg_replace('/^post:s*([0-9]+)$/', '$1', $array );
Demo: https://3v4l.org/64fO6
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
1
@fightstarr20 when catching white spaces you should uses
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks
– shaddy
Nov 11 at 17:30
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
add a comment |
up vote
1
down vote
up vote
1
down vote
You've missed the second argument of preg_replace
function, which is with what should replace the match, also your regex has small problem, here is the fixed version:
preg_replace('/^post:s*([0-9]+)$/', '$1', $array );
Demo: https://3v4l.org/64fO6
You've missed the second argument of preg_replace
function, which is with what should replace the match, also your regex has small problem, here is the fixed version:
preg_replace('/^post:s*([0-9]+)$/', '$1', $array );
Demo: https://3v4l.org/64fO6
edited Nov 11 at 17:24


Madhur Bhaiya
19.1k62236
19.1k62236
answered Nov 11 at 17:19


shaddy
6,09331631
6,09331631
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
1
@fightstarr20 when catching white spaces you should uses
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks
– shaddy
Nov 11 at 17:30
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
add a comment |
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
1
@fightstarr20 when catching white spaces you should uses
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks
– shaddy
Nov 11 at 17:30
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
Thank you, that work now. What was the issue with the regex?
– fightstarr20
Nov 11 at 17:22
1
1
@fightstarr20 when catching white spaces you should use
s
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks– shaddy
Nov 11 at 17:30
@fightstarr20 when catching white spaces you should use
s
instead of space character. If you find my answer helpful, please mark it as accepted. Thanks– shaddy
Nov 11 at 17:30
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
I'm not a huge proponent of micro optimization, but it's worth pointing out that this is going to be slower than a lot of the other answers pointing you away from preg_replace for this question. Use Regex when you need it, but you absolutely don't need it for this problem.
– gview
Nov 11 at 18:02
add a comment |
up vote
0
down vote
You don't have a pattern for the replacement, or a empty string place holder.
mixed preg_replace ( mixed $pattern , mixed $replacement , mixed $subject)
Is what you are trying to do (there are other args, but they are optional).
$array = preg_replace('/post: /', '', $array );
Should do it.
<?php
$array=array("post: 746",
"post: 2",
"post: 84");
$array = preg_replace('/^post: /', '', $array );
print_r($array);
?>
Array
(
[0] => 746
[1] => 2
[2] => 84
)
add a comment |
up vote
0
down vote
You don't have a pattern for the replacement, or a empty string place holder.
mixed preg_replace ( mixed $pattern , mixed $replacement , mixed $subject)
Is what you are trying to do (there are other args, but they are optional).
$array = preg_replace('/post: /', '', $array );
Should do it.
<?php
$array=array("post: 746",
"post: 2",
"post: 84");
$array = preg_replace('/^post: /', '', $array );
print_r($array);
?>
Array
(
[0] => 746
[1] => 2
[2] => 84
)
add a comment |
up vote
0
down vote
up vote
0
down vote
You don't have a pattern for the replacement, or a empty string place holder.
mixed preg_replace ( mixed $pattern , mixed $replacement , mixed $subject)
Is what you are trying to do (there are other args, but they are optional).
$array = preg_replace('/post: /', '', $array );
Should do it.
<?php
$array=array("post: 746",
"post: 2",
"post: 84");
$array = preg_replace('/^post: /', '', $array );
print_r($array);
?>
Array
(
[0] => 746
[1] => 2
[2] => 84
)
You don't have a pattern for the replacement, or a empty string place holder.
mixed preg_replace ( mixed $pattern , mixed $replacement , mixed $subject)
Is what you are trying to do (there are other args, but they are optional).
$array = preg_replace('/post: /', '', $array );
Should do it.
<?php
$array=array("post: 746",
"post: 2",
"post: 84");
$array = preg_replace('/^post: /', '', $array );
print_r($array);
?>
Array
(
[0] => 746
[1] => 2
[2] => 84
)
answered Nov 11 at 17:24
ivanivan
1,594258
1,594258
add a comment |
add a comment |
up vote
0
down vote
You could do this without using a regex using array_map and substr to check the prefix and return the string without the prefix:
$items = [
"post: 674",
"post: 2",
"post: 84",
];
$result = array_map(function($x)
$prefix = "post: ";
if (substr($x, 0, strlen($prefix)) == $prefix)
return substr($x, strlen($prefix));
return $x;
, $items);
print_r($result);
Result:
Array
(
[0] => 674
[1] => 2
[2] => 84
)
add a comment |
up vote
0
down vote
You could do this without using a regex using array_map and substr to check the prefix and return the string without the prefix:
$items = [
"post: 674",
"post: 2",
"post: 84",
];
$result = array_map(function($x)
$prefix = "post: ";
if (substr($x, 0, strlen($prefix)) == $prefix)
return substr($x, strlen($prefix));
return $x;
, $items);
print_r($result);
Result:
Array
(
[0] => 674
[1] => 2
[2] => 84
)
add a comment |
up vote
0
down vote
up vote
0
down vote
You could do this without using a regex using array_map and substr to check the prefix and return the string without the prefix:
$items = [
"post: 674",
"post: 2",
"post: 84",
];
$result = array_map(function($x)
$prefix = "post: ";
if (substr($x, 0, strlen($prefix)) == $prefix)
return substr($x, strlen($prefix));
return $x;
, $items);
print_r($result);
Result:
Array
(
[0] => 674
[1] => 2
[2] => 84
)
You could do this without using a regex using array_map and substr to check the prefix and return the string without the prefix:
$items = [
"post: 674",
"post: 2",
"post: 84",
];
$result = array_map(function($x)
$prefix = "post: ";
if (substr($x, 0, strlen($prefix)) == $prefix)
return substr($x, strlen($prefix));
return $x;
, $items);
print_r($result);
Result:
Array
(
[0] => 674
[1] => 2
[2] => 84
)
answered Nov 11 at 17:30
The fourth bird
19.4k71323
19.4k71323
add a comment |
add a comment |
up vote
0
down vote
There are many ways to do this that don't involve regular expressions, which are really not needed for breaking up a simple string like this.
For example:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
$o = explode(': ', $n);
return (int)$o[1];
, $input);
var_dump($output);
And here's another one that is probably even faster:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
return (int)substr($n, strpos($n, ':')+1);
, $input);
var_dump($output);
If you don't need integers in the output just remove the cast to int.
Or just use str_replace, which in many cases like this is a drop in replacement for preg_replace.
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = str_replace('post: ', '', $input);
var_dump($output);
add a comment |
up vote
0
down vote
There are many ways to do this that don't involve regular expressions, which are really not needed for breaking up a simple string like this.
For example:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
$o = explode(': ', $n);
return (int)$o[1];
, $input);
var_dump($output);
And here's another one that is probably even faster:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
return (int)substr($n, strpos($n, ':')+1);
, $input);
var_dump($output);
If you don't need integers in the output just remove the cast to int.
Or just use str_replace, which in many cases like this is a drop in replacement for preg_replace.
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = str_replace('post: ', '', $input);
var_dump($output);
add a comment |
up vote
0
down vote
up vote
0
down vote
There are many ways to do this that don't involve regular expressions, which are really not needed for breaking up a simple string like this.
For example:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
$o = explode(': ', $n);
return (int)$o[1];
, $input);
var_dump($output);
And here's another one that is probably even faster:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
return (int)substr($n, strpos($n, ':')+1);
, $input);
var_dump($output);
If you don't need integers in the output just remove the cast to int.
Or just use str_replace, which in many cases like this is a drop in replacement for preg_replace.
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = str_replace('post: ', '', $input);
var_dump($output);
There are many ways to do this that don't involve regular expressions, which are really not needed for breaking up a simple string like this.
For example:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
$o = explode(': ', $n);
return (int)$o[1];
, $input);
var_dump($output);
And here's another one that is probably even faster:
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = array_map(function ($n)
return (int)substr($n, strpos($n, ':')+1);
, $input);
var_dump($output);
If you don't need integers in the output just remove the cast to int.
Or just use str_replace, which in many cases like this is a drop in replacement for preg_replace.
<?php
$input = Array( 'post: 746', 'post: 2', 'post: 84');
$output = str_replace('post: ', '', $input);
var_dump($output);
edited Nov 11 at 18:00
answered Nov 11 at 17:25
gview
10.9k22639
10.9k22639
add a comment |
add a comment |
up vote
0
down vote
You can use array_map()
to iterate the array then strip out any non-digital characters via filter_var()
with FILTER_SANITIZE_NUMBER_INT
or trim()
with a "character mask" containing the six undesired characters.
You can also let preg_replace()
do the iterating for you. Using preg_replace()
offers the most brief syntax, but regular expressions are often slower than non-preg_ techniques and it may be overkill for your seemingly simple task.
Codes: (Demo)
$array = ["post: 746", "post: 2", "post: 84"];
// remove all non-integer characters
var_export(array_map(function($v)return filter_var($v, FILTER_SANITIZE_NUMBER_INT);, $array));
// only necessary if you have elements with non-"post: " AND non-integer substrings
var_export(preg_replace('~^post: ~', '', $array));
// I shuffled the character mask to prove order doesn't matter
var_export(array_map(function($v)return trim($v, ': opst');, $array));
Output: (from each technique is the same)
array (
0 => '746',
1 => '2',
2 => '84',
)
p.s. If anyone is going to entertain the idea of using explode()
to create an array of each element then store the second element of the array as the new desired string (and I wouldn't go to such trouble) be sure to:
- split on
or
:
(colon, space) or evenpost:
(post, colon, space) because splitting on:
(colon only) forces you to tidy up the second element's leading space and - use
explode()
's 3rd parameter (limit
) and set it to2
because logically, you don't need more than two elements
add a comment |
up vote
0
down vote
You can use array_map()
to iterate the array then strip out any non-digital characters via filter_var()
with FILTER_SANITIZE_NUMBER_INT
or trim()
with a "character mask" containing the six undesired characters.
You can also let preg_replace()
do the iterating for you. Using preg_replace()
offers the most brief syntax, but regular expressions are often slower than non-preg_ techniques and it may be overkill for your seemingly simple task.
Codes: (Demo)
$array = ["post: 746", "post: 2", "post: 84"];
// remove all non-integer characters
var_export(array_map(function($v)return filter_var($v, FILTER_SANITIZE_NUMBER_INT);, $array));
// only necessary if you have elements with non-"post: " AND non-integer substrings
var_export(preg_replace('~^post: ~', '', $array));
// I shuffled the character mask to prove order doesn't matter
var_export(array_map(function($v)return trim($v, ': opst');, $array));
Output: (from each technique is the same)
array (
0 => '746',
1 => '2',
2 => '84',
)
p.s. If anyone is going to entertain the idea of using explode()
to create an array of each element then store the second element of the array as the new desired string (and I wouldn't go to such trouble) be sure to:
- split on
or
:
(colon, space) or evenpost:
(post, colon, space) because splitting on:
(colon only) forces you to tidy up the second element's leading space and - use
explode()
's 3rd parameter (limit
) and set it to2
because logically, you don't need more than two elements
add a comment |
up vote
0
down vote
up vote
0
down vote
You can use array_map()
to iterate the array then strip out any non-digital characters via filter_var()
with FILTER_SANITIZE_NUMBER_INT
or trim()
with a "character mask" containing the six undesired characters.
You can also let preg_replace()
do the iterating for you. Using preg_replace()
offers the most brief syntax, but regular expressions are often slower than non-preg_ techniques and it may be overkill for your seemingly simple task.
Codes: (Demo)
$array = ["post: 746", "post: 2", "post: 84"];
// remove all non-integer characters
var_export(array_map(function($v)return filter_var($v, FILTER_SANITIZE_NUMBER_INT);, $array));
// only necessary if you have elements with non-"post: " AND non-integer substrings
var_export(preg_replace('~^post: ~', '', $array));
// I shuffled the character mask to prove order doesn't matter
var_export(array_map(function($v)return trim($v, ': opst');, $array));
Output: (from each technique is the same)
array (
0 => '746',
1 => '2',
2 => '84',
)
p.s. If anyone is going to entertain the idea of using explode()
to create an array of each element then store the second element of the array as the new desired string (and I wouldn't go to such trouble) be sure to:
- split on
or
:
(colon, space) or evenpost:
(post, colon, space) because splitting on:
(colon only) forces you to tidy up the second element's leading space and - use
explode()
's 3rd parameter (limit
) and set it to2
because logically, you don't need more than two elements
You can use array_map()
to iterate the array then strip out any non-digital characters via filter_var()
with FILTER_SANITIZE_NUMBER_INT
or trim()
with a "character mask" containing the six undesired characters.
You can also let preg_replace()
do the iterating for you. Using preg_replace()
offers the most brief syntax, but regular expressions are often slower than non-preg_ techniques and it may be overkill for your seemingly simple task.
Codes: (Demo)
$array = ["post: 746", "post: 2", "post: 84"];
// remove all non-integer characters
var_export(array_map(function($v)return filter_var($v, FILTER_SANITIZE_NUMBER_INT);, $array));
// only necessary if you have elements with non-"post: " AND non-integer substrings
var_export(preg_replace('~^post: ~', '', $array));
// I shuffled the character mask to prove order doesn't matter
var_export(array_map(function($v)return trim($v, ': opst');, $array));
Output: (from each technique is the same)
array (
0 => '746',
1 => '2',
2 => '84',
)
p.s. If anyone is going to entertain the idea of using explode()
to create an array of each element then store the second element of the array as the new desired string (and I wouldn't go to such trouble) be sure to:
- split on
or
:
(colon, space) or evenpost:
(post, colon, space) because splitting on:
(colon only) forces you to tidy up the second element's leading space and - use
explode()
's 3rd parameter (limit
) and set it to2
because logically, you don't need more than two elements
edited Nov 16 at 21:46
answered Nov 16 at 21:41


mickmackusa
21.4k83256
21.4k83256
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251188%2fphp-preg-replace-on-an-array-not-working-as-expected%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
raStT8vXB9,K3WPuCRVRFwyz,exg
1
preg_replace()
requires three parameters. You are using only 2. This will give syntax errors– Madhur Bhaiya
Nov 11 at 17:19