Why does pyplot display opencl calculation result as black screen?
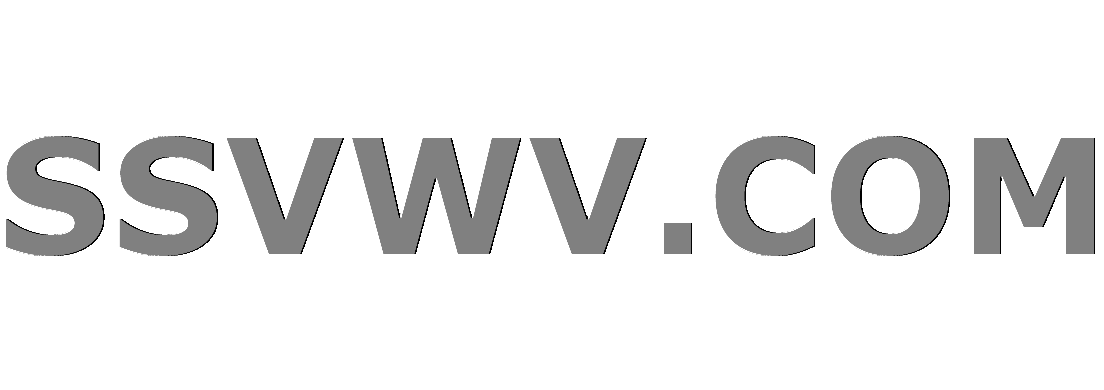
Multi tool use
up vote
0
down vote
favorite
I'm writing a mandelbrot set calculation program with OpenCL to learn something about it. But results of calculations is kinda weird. I have a function that calculates set:
def cl_mandelbrot(ray, maxiter, ctx):
program = cl.Program(ctx, """
#pragma OPENCL EXTENSION cl_khr_byte_addressable_store : enable
__kernel void m_brot(__global float2 *arr,
__global ushort *output,
ushort const maxiters)
int gid = get_global_id(0);
float nreal, real = 0;
float imag = 0;
output[gid] = 0;
for(int curiter = 0; curiter < maxiters; curiter++)
nreal = real*real - imag*imag + arr[gid].x;
imag = 2* real*imag + arr[gid].y;
real = nreal;
if (real*real + imag*imag > 4.0f)
output[gid] = curiter;
break;
""").build()
output = np.empty(arr.shape, dtype=np.uint8)
queue = cl.CommandQueue(ctx)
mem_flags = cl.mem_flags
arr_buf = cl.Buffer(ctx, mem_flags.COPY_HOST_PTR | mem_flags.READ_ONLY, hostbuf=ray)
out_buf = cl.Buffer(ctx, mem_flags.WRITE_ONLY, output.nbytes)
program.m_brot(queue, output.shape, None, arr_buf, out_buf, np.uint16(maxiter))
cl.enqueue_copy(queue, output, out_buf).wait()
return output
And function that passes arguments to cl_mandelbrot() function:
def fillarr_gpu(xmax, xmin, ymax, ymin, iter, width, height, ctx):
lx = np.linspace(xmin, xmax, width, dtype=np.float64)
ly = np.linspace(ymin, ymax, height, dtype=np.float64)
c = lx + ly[:, None]*1j
c = np.ravel(c)
print(c[1])
res = cl_mandelbrot(c, iter, ctx)
print(res.size)
res = res.reshape((width, height))
return res
The problem is with these functions.
Here's the result drawen with pyplot
python matplotlib opencl pyopencl mandelbrot
add a comment |
up vote
0
down vote
favorite
I'm writing a mandelbrot set calculation program with OpenCL to learn something about it. But results of calculations is kinda weird. I have a function that calculates set:
def cl_mandelbrot(ray, maxiter, ctx):
program = cl.Program(ctx, """
#pragma OPENCL EXTENSION cl_khr_byte_addressable_store : enable
__kernel void m_brot(__global float2 *arr,
__global ushort *output,
ushort const maxiters)
int gid = get_global_id(0);
float nreal, real = 0;
float imag = 0;
output[gid] = 0;
for(int curiter = 0; curiter < maxiters; curiter++)
nreal = real*real - imag*imag + arr[gid].x;
imag = 2* real*imag + arr[gid].y;
real = nreal;
if (real*real + imag*imag > 4.0f)
output[gid] = curiter;
break;
""").build()
output = np.empty(arr.shape, dtype=np.uint8)
queue = cl.CommandQueue(ctx)
mem_flags = cl.mem_flags
arr_buf = cl.Buffer(ctx, mem_flags.COPY_HOST_PTR | mem_flags.READ_ONLY, hostbuf=ray)
out_buf = cl.Buffer(ctx, mem_flags.WRITE_ONLY, output.nbytes)
program.m_brot(queue, output.shape, None, arr_buf, out_buf, np.uint16(maxiter))
cl.enqueue_copy(queue, output, out_buf).wait()
return output
And function that passes arguments to cl_mandelbrot() function:
def fillarr_gpu(xmax, xmin, ymax, ymin, iter, width, height, ctx):
lx = np.linspace(xmin, xmax, width, dtype=np.float64)
ly = np.linspace(ymin, ymax, height, dtype=np.float64)
c = lx + ly[:, None]*1j
c = np.ravel(c)
print(c[1])
res = cl_mandelbrot(c, iter, ctx)
print(res.size)
res = res.reshape((width, height))
return res
The problem is with these functions.
Here's the result drawen with pyplot
python matplotlib opencl pyopencl mandelbrot
Show the exact code that generates the plot. Also, assumingres
is the array that is plotted, showres.dtype
,res.min()
andres.max()
.
– Warren Weckesser
Nov 11 at 17:19
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm writing a mandelbrot set calculation program with OpenCL to learn something about it. But results of calculations is kinda weird. I have a function that calculates set:
def cl_mandelbrot(ray, maxiter, ctx):
program = cl.Program(ctx, """
#pragma OPENCL EXTENSION cl_khr_byte_addressable_store : enable
__kernel void m_brot(__global float2 *arr,
__global ushort *output,
ushort const maxiters)
int gid = get_global_id(0);
float nreal, real = 0;
float imag = 0;
output[gid] = 0;
for(int curiter = 0; curiter < maxiters; curiter++)
nreal = real*real - imag*imag + arr[gid].x;
imag = 2* real*imag + arr[gid].y;
real = nreal;
if (real*real + imag*imag > 4.0f)
output[gid] = curiter;
break;
""").build()
output = np.empty(arr.shape, dtype=np.uint8)
queue = cl.CommandQueue(ctx)
mem_flags = cl.mem_flags
arr_buf = cl.Buffer(ctx, mem_flags.COPY_HOST_PTR | mem_flags.READ_ONLY, hostbuf=ray)
out_buf = cl.Buffer(ctx, mem_flags.WRITE_ONLY, output.nbytes)
program.m_brot(queue, output.shape, None, arr_buf, out_buf, np.uint16(maxiter))
cl.enqueue_copy(queue, output, out_buf).wait()
return output
And function that passes arguments to cl_mandelbrot() function:
def fillarr_gpu(xmax, xmin, ymax, ymin, iter, width, height, ctx):
lx = np.linspace(xmin, xmax, width, dtype=np.float64)
ly = np.linspace(ymin, ymax, height, dtype=np.float64)
c = lx + ly[:, None]*1j
c = np.ravel(c)
print(c[1])
res = cl_mandelbrot(c, iter, ctx)
print(res.size)
res = res.reshape((width, height))
return res
The problem is with these functions.
Here's the result drawen with pyplot
python matplotlib opencl pyopencl mandelbrot
I'm writing a mandelbrot set calculation program with OpenCL to learn something about it. But results of calculations is kinda weird. I have a function that calculates set:
def cl_mandelbrot(ray, maxiter, ctx):
program = cl.Program(ctx, """
#pragma OPENCL EXTENSION cl_khr_byte_addressable_store : enable
__kernel void m_brot(__global float2 *arr,
__global ushort *output,
ushort const maxiters)
int gid = get_global_id(0);
float nreal, real = 0;
float imag = 0;
output[gid] = 0;
for(int curiter = 0; curiter < maxiters; curiter++)
nreal = real*real - imag*imag + arr[gid].x;
imag = 2* real*imag + arr[gid].y;
real = nreal;
if (real*real + imag*imag > 4.0f)
output[gid] = curiter;
break;
""").build()
output = np.empty(arr.shape, dtype=np.uint8)
queue = cl.CommandQueue(ctx)
mem_flags = cl.mem_flags
arr_buf = cl.Buffer(ctx, mem_flags.COPY_HOST_PTR | mem_flags.READ_ONLY, hostbuf=ray)
out_buf = cl.Buffer(ctx, mem_flags.WRITE_ONLY, output.nbytes)
program.m_brot(queue, output.shape, None, arr_buf, out_buf, np.uint16(maxiter))
cl.enqueue_copy(queue, output, out_buf).wait()
return output
And function that passes arguments to cl_mandelbrot() function:
def fillarr_gpu(xmax, xmin, ymax, ymin, iter, width, height, ctx):
lx = np.linspace(xmin, xmax, width, dtype=np.float64)
ly = np.linspace(ymin, ymax, height, dtype=np.float64)
c = lx + ly[:, None]*1j
c = np.ravel(c)
print(c[1])
res = cl_mandelbrot(c, iter, ctx)
print(res.size)
res = res.reshape((width, height))
return res
The problem is with these functions.
Here's the result drawen with pyplot
python matplotlib opencl pyopencl mandelbrot
python matplotlib opencl pyopencl mandelbrot
asked Nov 11 at 17:15


DucalisGD
1
1
Show the exact code that generates the plot. Also, assumingres
is the array that is plotted, showres.dtype
,res.min()
andres.max()
.
– Warren Weckesser
Nov 11 at 17:19
add a comment |
Show the exact code that generates the plot. Also, assumingres
is the array that is plotted, showres.dtype
,res.min()
andres.max()
.
– Warren Weckesser
Nov 11 at 17:19
Show the exact code that generates the plot. Also, assuming
res
is the array that is plotted, show res.dtype
, res.min()
and res.max()
.– Warren Weckesser
Nov 11 at 17:19
Show the exact code that generates the plot. Also, assuming
res
is the array that is plotted, show res.dtype
, res.min()
and res.max()
.– Warren Weckesser
Nov 11 at 17:19
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251197%2fwhy-does-pyplot-display-opencl-calculation-result-as-black-screen%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4,cdqKuM,Jbw7N2425,dC1d Le2ydu
Show the exact code that generates the plot. Also, assuming
res
is the array that is plotted, showres.dtype
,res.min()
andres.max()
.– Warren Weckesser
Nov 11 at 17:19