Shelve giving NoneType error even though it's there (Python)
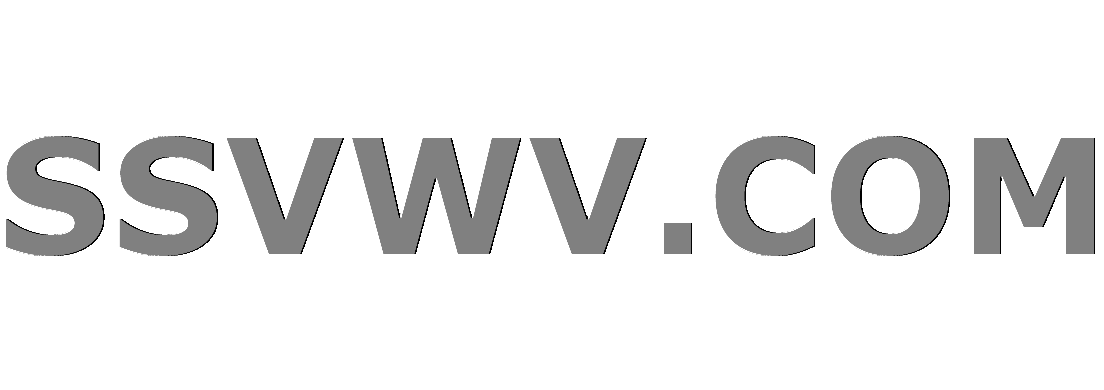
Multi tool use
up vote
0
down vote
favorite
I'm trying to append to a list to then persist data in my shelve db
I have a file "base_de_datos" where I have my lists
Then I have a file "gestionador" where I load or save data
# ___base_de_datos___
clientes =
asesores =
solicitudes =
My code works for clientes
and asesores
but keeps dying at solicitudes it gives me a KeyError
# _____gestionador_____
import shelve
import base_de_datos as bd
def cargar_datos():
'''carga los datos en listas para su uso'''
bd.clientes = cargar_lista("clientes", "Clientes")
bd.asesores = cargar_lista("asesores", "Asesores")
bd.solicitudes = cargar_lista("solicitudes", "Solicitudes")
def guardar_datos():
'''guarda los datos en las listas en archivos para la persistencia'''
guardar_lista(bd.clientes,"Clientes", "clientes")
guardar_lista(bd.asesores,"Asesores", "asesores")
guardar_lista(bd.solicitudes,"Solicitudes", "solicitudes")
def cargar_lista(clave, nombreArchivo):
'''carga los datos al sistema cuando se este ejecutando'''
try:
basededatos = shelve.open(nombreArchivo)
lista = basededatos[clave]
if lista is None:
lista =
return lista
except KeyError:
return print("ERROR Ocurrio un al encontrar los datos")
def guardar_lista(objeto,nombreArchivo, clave):
''' funcion que sirve para guardar los datos en basededatos'''
try:
basededatos = shelve.open(nombreArchivo,'n')
basededatos[clave] = objeto
except FileNotFoundError:
print("ERROR Archivo no existe")
else:
basededatos.close()
basededatos = None
This is how I call Solicitudes
def add_solicitud(self):
datos = Solicitud.promp_init()
solicitud = Solicitud(**datos)
base_de_datos.solicitudes.append(solicitud)
gestionador.guardar_datos()
My class Solicitudes
, where I have the method promp_init()
class Boleta(metaclass=ABCMeta):
@abstractmethod
def __init__(self):
pass
class Solicitud(Boleta):
def __init__(self, date='' , cliente='', asesor=''):
super().__init__()
self.date= date
self.cliente = cliente
self.asesor = asesor
def promp_init():
date= datetime.now()
print("The date is the actual date")
cliente=input_entero()
asesor=input_entero()
return dict(
"date": date,
"cliente": cliente,
"asesor": asesor
)
I get the error once I finish typing Solicitud
's data
'NoneType' object has no attribute 'append'
When I debug on pycharm I already get a KeyError
when trying to open the file for Solicitudes
at the the method cargar_lista
I added
if lista is None:
lista =
because I was told I needed to initialize it first which it worked but after a while the same thing happened again
Edit: Somehow started working, I think It's an error that happens whenever I add a new list to "base_de_datos" and it needs to be initialize.
python-3.x persistence shelve
add a comment |
up vote
0
down vote
favorite
I'm trying to append to a list to then persist data in my shelve db
I have a file "base_de_datos" where I have my lists
Then I have a file "gestionador" where I load or save data
# ___base_de_datos___
clientes =
asesores =
solicitudes =
My code works for clientes
and asesores
but keeps dying at solicitudes it gives me a KeyError
# _____gestionador_____
import shelve
import base_de_datos as bd
def cargar_datos():
'''carga los datos en listas para su uso'''
bd.clientes = cargar_lista("clientes", "Clientes")
bd.asesores = cargar_lista("asesores", "Asesores")
bd.solicitudes = cargar_lista("solicitudes", "Solicitudes")
def guardar_datos():
'''guarda los datos en las listas en archivos para la persistencia'''
guardar_lista(bd.clientes,"Clientes", "clientes")
guardar_lista(bd.asesores,"Asesores", "asesores")
guardar_lista(bd.solicitudes,"Solicitudes", "solicitudes")
def cargar_lista(clave, nombreArchivo):
'''carga los datos al sistema cuando se este ejecutando'''
try:
basededatos = shelve.open(nombreArchivo)
lista = basededatos[clave]
if lista is None:
lista =
return lista
except KeyError:
return print("ERROR Ocurrio un al encontrar los datos")
def guardar_lista(objeto,nombreArchivo, clave):
''' funcion que sirve para guardar los datos en basededatos'''
try:
basededatos = shelve.open(nombreArchivo,'n')
basededatos[clave] = objeto
except FileNotFoundError:
print("ERROR Archivo no existe")
else:
basededatos.close()
basededatos = None
This is how I call Solicitudes
def add_solicitud(self):
datos = Solicitud.promp_init()
solicitud = Solicitud(**datos)
base_de_datos.solicitudes.append(solicitud)
gestionador.guardar_datos()
My class Solicitudes
, where I have the method promp_init()
class Boleta(metaclass=ABCMeta):
@abstractmethod
def __init__(self):
pass
class Solicitud(Boleta):
def __init__(self, date='' , cliente='', asesor=''):
super().__init__()
self.date= date
self.cliente = cliente
self.asesor = asesor
def promp_init():
date= datetime.now()
print("The date is the actual date")
cliente=input_entero()
asesor=input_entero()
return dict(
"date": date,
"cliente": cliente,
"asesor": asesor
)
I get the error once I finish typing Solicitud
's data
'NoneType' object has no attribute 'append'
When I debug on pycharm I already get a KeyError
when trying to open the file for Solicitudes
at the the method cargar_lista
I added
if lista is None:
lista =
because I was told I needed to initialize it first which it worked but after a while the same thing happened again
Edit: Somehow started working, I think It's an error that happens whenever I add a new list to "base_de_datos" and it needs to be initialize.
python-3.x persistence shelve
could you post the full stack trace of error?
– Paritosh Singh
Nov 11 at 17:19
Please include the full traceback, so we don't have to guess exactly what the context is.
– Martijn Pieters♦
Nov 11 at 17:21
Thank you for the response, how do I get the full traceback? is it the errors I get on the console?
– Javier Heisecke
Nov 11 at 17:38
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to append to a list to then persist data in my shelve db
I have a file "base_de_datos" where I have my lists
Then I have a file "gestionador" where I load or save data
# ___base_de_datos___
clientes =
asesores =
solicitudes =
My code works for clientes
and asesores
but keeps dying at solicitudes it gives me a KeyError
# _____gestionador_____
import shelve
import base_de_datos as bd
def cargar_datos():
'''carga los datos en listas para su uso'''
bd.clientes = cargar_lista("clientes", "Clientes")
bd.asesores = cargar_lista("asesores", "Asesores")
bd.solicitudes = cargar_lista("solicitudes", "Solicitudes")
def guardar_datos():
'''guarda los datos en las listas en archivos para la persistencia'''
guardar_lista(bd.clientes,"Clientes", "clientes")
guardar_lista(bd.asesores,"Asesores", "asesores")
guardar_lista(bd.solicitudes,"Solicitudes", "solicitudes")
def cargar_lista(clave, nombreArchivo):
'''carga los datos al sistema cuando se este ejecutando'''
try:
basededatos = shelve.open(nombreArchivo)
lista = basededatos[clave]
if lista is None:
lista =
return lista
except KeyError:
return print("ERROR Ocurrio un al encontrar los datos")
def guardar_lista(objeto,nombreArchivo, clave):
''' funcion que sirve para guardar los datos en basededatos'''
try:
basededatos = shelve.open(nombreArchivo,'n')
basededatos[clave] = objeto
except FileNotFoundError:
print("ERROR Archivo no existe")
else:
basededatos.close()
basededatos = None
This is how I call Solicitudes
def add_solicitud(self):
datos = Solicitud.promp_init()
solicitud = Solicitud(**datos)
base_de_datos.solicitudes.append(solicitud)
gestionador.guardar_datos()
My class Solicitudes
, where I have the method promp_init()
class Boleta(metaclass=ABCMeta):
@abstractmethod
def __init__(self):
pass
class Solicitud(Boleta):
def __init__(self, date='' , cliente='', asesor=''):
super().__init__()
self.date= date
self.cliente = cliente
self.asesor = asesor
def promp_init():
date= datetime.now()
print("The date is the actual date")
cliente=input_entero()
asesor=input_entero()
return dict(
"date": date,
"cliente": cliente,
"asesor": asesor
)
I get the error once I finish typing Solicitud
's data
'NoneType' object has no attribute 'append'
When I debug on pycharm I already get a KeyError
when trying to open the file for Solicitudes
at the the method cargar_lista
I added
if lista is None:
lista =
because I was told I needed to initialize it first which it worked but after a while the same thing happened again
Edit: Somehow started working, I think It's an error that happens whenever I add a new list to "base_de_datos" and it needs to be initialize.
python-3.x persistence shelve
I'm trying to append to a list to then persist data in my shelve db
I have a file "base_de_datos" where I have my lists
Then I have a file "gestionador" where I load or save data
# ___base_de_datos___
clientes =
asesores =
solicitudes =
My code works for clientes
and asesores
but keeps dying at solicitudes it gives me a KeyError
# _____gestionador_____
import shelve
import base_de_datos as bd
def cargar_datos():
'''carga los datos en listas para su uso'''
bd.clientes = cargar_lista("clientes", "Clientes")
bd.asesores = cargar_lista("asesores", "Asesores")
bd.solicitudes = cargar_lista("solicitudes", "Solicitudes")
def guardar_datos():
'''guarda los datos en las listas en archivos para la persistencia'''
guardar_lista(bd.clientes,"Clientes", "clientes")
guardar_lista(bd.asesores,"Asesores", "asesores")
guardar_lista(bd.solicitudes,"Solicitudes", "solicitudes")
def cargar_lista(clave, nombreArchivo):
'''carga los datos al sistema cuando se este ejecutando'''
try:
basededatos = shelve.open(nombreArchivo)
lista = basededatos[clave]
if lista is None:
lista =
return lista
except KeyError:
return print("ERROR Ocurrio un al encontrar los datos")
def guardar_lista(objeto,nombreArchivo, clave):
''' funcion que sirve para guardar los datos en basededatos'''
try:
basededatos = shelve.open(nombreArchivo,'n')
basededatos[clave] = objeto
except FileNotFoundError:
print("ERROR Archivo no existe")
else:
basededatos.close()
basededatos = None
This is how I call Solicitudes
def add_solicitud(self):
datos = Solicitud.promp_init()
solicitud = Solicitud(**datos)
base_de_datos.solicitudes.append(solicitud)
gestionador.guardar_datos()
My class Solicitudes
, where I have the method promp_init()
class Boleta(metaclass=ABCMeta):
@abstractmethod
def __init__(self):
pass
class Solicitud(Boleta):
def __init__(self, date='' , cliente='', asesor=''):
super().__init__()
self.date= date
self.cliente = cliente
self.asesor = asesor
def promp_init():
date= datetime.now()
print("The date is the actual date")
cliente=input_entero()
asesor=input_entero()
return dict(
"date": date,
"cliente": cliente,
"asesor": asesor
)
I get the error once I finish typing Solicitud
's data
'NoneType' object has no attribute 'append'
When I debug on pycharm I already get a KeyError
when trying to open the file for Solicitudes
at the the method cargar_lista
I added
if lista is None:
lista =
because I was told I needed to initialize it first which it worked but after a while the same thing happened again
Edit: Somehow started working, I think It's an error that happens whenever I add a new list to "base_de_datos" and it needs to be initialize.
python-3.x persistence shelve
python-3.x persistence shelve
edited Nov 12 at 15:11
asked Nov 11 at 17:15


Javier Heisecke
267
267
could you post the full stack trace of error?
– Paritosh Singh
Nov 11 at 17:19
Please include the full traceback, so we don't have to guess exactly what the context is.
– Martijn Pieters♦
Nov 11 at 17:21
Thank you for the response, how do I get the full traceback? is it the errors I get on the console?
– Javier Heisecke
Nov 11 at 17:38
add a comment |
could you post the full stack trace of error?
– Paritosh Singh
Nov 11 at 17:19
Please include the full traceback, so we don't have to guess exactly what the context is.
– Martijn Pieters♦
Nov 11 at 17:21
Thank you for the response, how do I get the full traceback? is it the errors I get on the console?
– Javier Heisecke
Nov 11 at 17:38
could you post the full stack trace of error?
– Paritosh Singh
Nov 11 at 17:19
could you post the full stack trace of error?
– Paritosh Singh
Nov 11 at 17:19
Please include the full traceback, so we don't have to guess exactly what the context is.
– Martijn Pieters♦
Nov 11 at 17:21
Please include the full traceback, so we don't have to guess exactly what the context is.
– Martijn Pieters♦
Nov 11 at 17:21
Thank you for the response, how do I get the full traceback? is it the errors I get on the console?
– Javier Heisecke
Nov 11 at 17:38
Thank you for the response, how do I get the full traceback? is it the errors I get on the console?
– Javier Heisecke
Nov 11 at 17:38
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251194%2fshelve-giving-nonetype-error-even-though-its-there-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bPdhgGLPr,WQ
could you post the full stack trace of error?
– Paritosh Singh
Nov 11 at 17:19
Please include the full traceback, so we don't have to guess exactly what the context is.
– Martijn Pieters♦
Nov 11 at 17:21
Thank you for the response, how do I get the full traceback? is it the errors I get on the console?
– Javier Heisecke
Nov 11 at 17:38