How to get the target of a JavaScript Proxy?
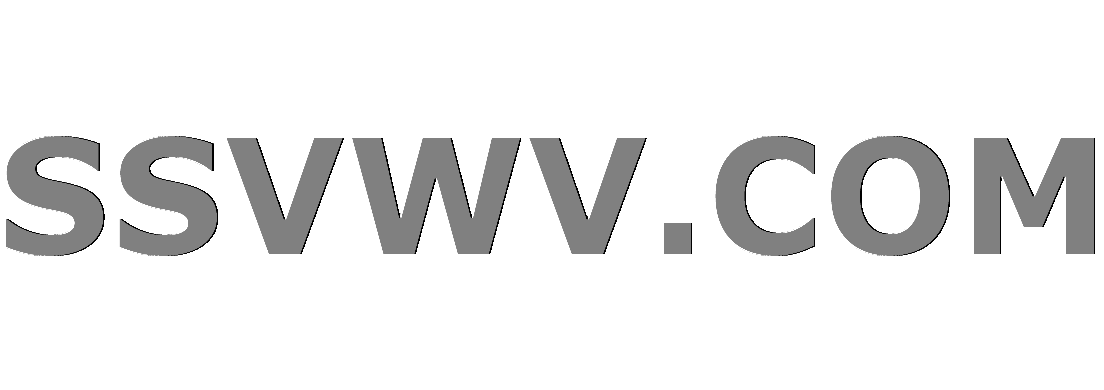
Multi tool use
up vote
0
down vote
favorite
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, );
const myProxy = createProxy();
How to access the target
(which is myArray
) of myProxy
here?
I've tried many ways. Was googled many blog posts, but found no way to get the target :(
javascript ecmascript-6 proxy
add a comment |
up vote
0
down vote
favorite
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, );
const myProxy = createProxy();
How to access the target
(which is myArray
) of myProxy
here?
I've tried many ways. Was googled many blog posts, but found no way to get the target :(
javascript ecmascript-6 proxy
1
You can't. That's why it's a proxy. What are you trying to do, why do you need this?
– Bergi
Jun 29 at 7:54
@Bergi Because if I have an object with circular references, and these circular references are in proxies, there is no way to safe stringify my object. I'll get stack size exceeded error. :(
– Adam
Jun 29 at 7:59
1
Can you make an example of that, please? Circular references (I think you mean those, not dependencies) should work just fine with proxies, asmyProxy === myProxy
still holds. Nothing needs to get its hands on the target.
– Bergi
Jun 29 at 8:02
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, );
const myProxy = createProxy();
How to access the target
(which is myArray
) of myProxy
here?
I've tried many ways. Was googled many blog posts, but found no way to get the target :(
javascript ecmascript-6 proxy
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, );
const myProxy = createProxy();
How to access the target
(which is myArray
) of myProxy
here?
I've tried many ways. Was googled many blog posts, but found no way to get the target :(
javascript ecmascript-6 proxy
javascript ecmascript-6 proxy
asked Jun 29 at 7:33


Adam
2,188827
2,188827
1
You can't. That's why it's a proxy. What are you trying to do, why do you need this?
– Bergi
Jun 29 at 7:54
@Bergi Because if I have an object with circular references, and these circular references are in proxies, there is no way to safe stringify my object. I'll get stack size exceeded error. :(
– Adam
Jun 29 at 7:59
1
Can you make an example of that, please? Circular references (I think you mean those, not dependencies) should work just fine with proxies, asmyProxy === myProxy
still holds. Nothing needs to get its hands on the target.
– Bergi
Jun 29 at 8:02
add a comment |
1
You can't. That's why it's a proxy. What are you trying to do, why do you need this?
– Bergi
Jun 29 at 7:54
@Bergi Because if I have an object with circular references, and these circular references are in proxies, there is no way to safe stringify my object. I'll get stack size exceeded error. :(
– Adam
Jun 29 at 7:59
1
Can you make an example of that, please? Circular references (I think you mean those, not dependencies) should work just fine with proxies, asmyProxy === myProxy
still holds. Nothing needs to get its hands on the target.
– Bergi
Jun 29 at 8:02
1
1
You can't. That's why it's a proxy. What are you trying to do, why do you need this?
– Bergi
Jun 29 at 7:54
You can't. That's why it's a proxy. What are you trying to do, why do you need this?
– Bergi
Jun 29 at 7:54
@Bergi Because if I have an object with circular references, and these circular references are in proxies, there is no way to safe stringify my object. I'll get stack size exceeded error. :(
– Adam
Jun 29 at 7:59
@Bergi Because if I have an object with circular references, and these circular references are in proxies, there is no way to safe stringify my object. I'll get stack size exceeded error. :(
– Adam
Jun 29 at 7:59
1
1
Can you make an example of that, please? Circular references (I think you mean those, not dependencies) should work just fine with proxies, as
myProxy === myProxy
still holds. Nothing needs to get its hands on the target.– Bergi
Jun 29 at 8:02
Can you make an example of that, please? Circular references (I think you mean those, not dependencies) should work just fine with proxies, as
myProxy === myProxy
still holds. Nothing needs to get its hands on the target.– Bergi
Jun 29 at 8:02
add a comment |
5 Answers
5
active
oldest
votes
up vote
1
down vote
There is a clever way to do this - You can add a get trap to the proxy and have it return the target conditionally. Like so..
let resolveMode = false; // Switch that controls if getter returns target or prop.
function resolve(obj)
resolveMode = true; // Turn on our switch
let target = obj.anything; // This gets the target not the prop!
resolveMode = false; // Turn off the switch for the getter to behave normally
return target; // Return what we got!
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray,
get: function(target, prop)
if (resolveMode) return target; // This is where the magic happens!
else return target[prop]; // This is normal behavior..
);
const myProxy = createProxy();
let target = resolve(myProxy);
Remember that the more lines of code you add to traps, the slower the object's performance gets. Hope this helps.
add a comment |
up vote
0
down vote
You can make a copy of the data returned by the proxy using Object.assign()
:
const target_copy = Object.assign(, my_proxy);
This will work for all enumerable own properties existing on the proxy/target.
add a comment |
up vote
0
down vote
As the proxy contain an object you can also do
Object.keys( my_proxy )
And then it become easy to retrieve thing such as Object.keys( my_proxy ).length
add a comment |
up vote
0
down vote
You can if the target is an object.
You will have to create a function in target to retrieve it, that's all.
Example:
class AnyClass
constructor()
this.target = this;
return new Proxy(this, this);
get(obj, prop)
if (prop in obj)
return this[prop];
// your stuff here
getTarget()
return this.target;
And then when you call:
let sample = new AnyClass;
console.log(sample.getTarget());
Will return you the target as you expect :)
add a comment |
up vote
0
down vote
How about adding the following get trap:
const handler =
get: (target, property, receiver) =>
if (property === 'myTarget')
return target
return target[property]
const myArray = [Math.random(), Math.random()];
function createProxy()
// const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, handler);
const myProxy = createProxy();
And you can get the target of the proxy by myProxy.myTarget
:
console.log(myProxy.myTarget) // [0.22089416118932403, 0.08429264462405173]
console.log(myArray === myProxy.myTarget) // true
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
There is a clever way to do this - You can add a get trap to the proxy and have it return the target conditionally. Like so..
let resolveMode = false; // Switch that controls if getter returns target or prop.
function resolve(obj)
resolveMode = true; // Turn on our switch
let target = obj.anything; // This gets the target not the prop!
resolveMode = false; // Turn off the switch for the getter to behave normally
return target; // Return what we got!
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray,
get: function(target, prop)
if (resolveMode) return target; // This is where the magic happens!
else return target[prop]; // This is normal behavior..
);
const myProxy = createProxy();
let target = resolve(myProxy);
Remember that the more lines of code you add to traps, the slower the object's performance gets. Hope this helps.
add a comment |
up vote
1
down vote
There is a clever way to do this - You can add a get trap to the proxy and have it return the target conditionally. Like so..
let resolveMode = false; // Switch that controls if getter returns target or prop.
function resolve(obj)
resolveMode = true; // Turn on our switch
let target = obj.anything; // This gets the target not the prop!
resolveMode = false; // Turn off the switch for the getter to behave normally
return target; // Return what we got!
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray,
get: function(target, prop)
if (resolveMode) return target; // This is where the magic happens!
else return target[prop]; // This is normal behavior..
);
const myProxy = createProxy();
let target = resolve(myProxy);
Remember that the more lines of code you add to traps, the slower the object's performance gets. Hope this helps.
add a comment |
up vote
1
down vote
up vote
1
down vote
There is a clever way to do this - You can add a get trap to the proxy and have it return the target conditionally. Like so..
let resolveMode = false; // Switch that controls if getter returns target or prop.
function resolve(obj)
resolveMode = true; // Turn on our switch
let target = obj.anything; // This gets the target not the prop!
resolveMode = false; // Turn off the switch for the getter to behave normally
return target; // Return what we got!
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray,
get: function(target, prop)
if (resolveMode) return target; // This is where the magic happens!
else return target[prop]; // This is normal behavior..
);
const myProxy = createProxy();
let target = resolve(myProxy);
Remember that the more lines of code you add to traps, the slower the object's performance gets. Hope this helps.
There is a clever way to do this - You can add a get trap to the proxy and have it return the target conditionally. Like so..
let resolveMode = false; // Switch that controls if getter returns target or prop.
function resolve(obj)
resolveMode = true; // Turn on our switch
let target = obj.anything; // This gets the target not the prop!
resolveMode = false; // Turn off the switch for the getter to behave normally
return target; // Return what we got!
function createProxy()
const myArray = [Math.random(), Math.random()];
return new Proxy(myArray,
get: function(target, prop)
if (resolveMode) return target; // This is where the magic happens!
else return target[prop]; // This is normal behavior..
);
const myProxy = createProxy();
let target = resolve(myProxy);
Remember that the more lines of code you add to traps, the slower the object's performance gets. Hope this helps.
answered Nov 11 at 17:06
Soumik Chatterjee
111
111
add a comment |
add a comment |
up vote
0
down vote
You can make a copy of the data returned by the proxy using Object.assign()
:
const target_copy = Object.assign(, my_proxy);
This will work for all enumerable own properties existing on the proxy/target.
add a comment |
up vote
0
down vote
You can make a copy of the data returned by the proxy using Object.assign()
:
const target_copy = Object.assign(, my_proxy);
This will work for all enumerable own properties existing on the proxy/target.
add a comment |
up vote
0
down vote
up vote
0
down vote
You can make a copy of the data returned by the proxy using Object.assign()
:
const target_copy = Object.assign(, my_proxy);
This will work for all enumerable own properties existing on the proxy/target.
You can make a copy of the data returned by the proxy using Object.assign()
:
const target_copy = Object.assign(, my_proxy);
This will work for all enumerable own properties existing on the proxy/target.
edited Sep 25 at 6:05
answered Sep 25 at 5:15
Rashad Saleh
944713
944713
add a comment |
add a comment |
up vote
0
down vote
As the proxy contain an object you can also do
Object.keys( my_proxy )
And then it become easy to retrieve thing such as Object.keys( my_proxy ).length
add a comment |
up vote
0
down vote
As the proxy contain an object you can also do
Object.keys( my_proxy )
And then it become easy to retrieve thing such as Object.keys( my_proxy ).length
add a comment |
up vote
0
down vote
up vote
0
down vote
As the proxy contain an object you can also do
Object.keys( my_proxy )
And then it become easy to retrieve thing such as Object.keys( my_proxy ).length
As the proxy contain an object you can also do
Object.keys( my_proxy )
And then it become easy to retrieve thing such as Object.keys( my_proxy ).length
answered Oct 4 at 10:56


Raccoon
6511125
6511125
add a comment |
add a comment |
up vote
0
down vote
You can if the target is an object.
You will have to create a function in target to retrieve it, that's all.
Example:
class AnyClass
constructor()
this.target = this;
return new Proxy(this, this);
get(obj, prop)
if (prop in obj)
return this[prop];
// your stuff here
getTarget()
return this.target;
And then when you call:
let sample = new AnyClass;
console.log(sample.getTarget());
Will return you the target as you expect :)
add a comment |
up vote
0
down vote
You can if the target is an object.
You will have to create a function in target to retrieve it, that's all.
Example:
class AnyClass
constructor()
this.target = this;
return new Proxy(this, this);
get(obj, prop)
if (prop in obj)
return this[prop];
// your stuff here
getTarget()
return this.target;
And then when you call:
let sample = new AnyClass;
console.log(sample.getTarget());
Will return you the target as you expect :)
add a comment |
up vote
0
down vote
up vote
0
down vote
You can if the target is an object.
You will have to create a function in target to retrieve it, that's all.
Example:
class AnyClass
constructor()
this.target = this;
return new Proxy(this, this);
get(obj, prop)
if (prop in obj)
return this[prop];
// your stuff here
getTarget()
return this.target;
And then when you call:
let sample = new AnyClass;
console.log(sample.getTarget());
Will return you the target as you expect :)
You can if the target is an object.
You will have to create a function in target to retrieve it, that's all.
Example:
class AnyClass
constructor()
this.target = this;
return new Proxy(this, this);
get(obj, prop)
if (prop in obj)
return this[prop];
// your stuff here
getTarget()
return this.target;
And then when you call:
let sample = new AnyClass;
console.log(sample.getTarget());
Will return you the target as you expect :)
answered Nov 7 at 18:20


ragnar
958915
958915
add a comment |
add a comment |
up vote
0
down vote
How about adding the following get trap:
const handler =
get: (target, property, receiver) =>
if (property === 'myTarget')
return target
return target[property]
const myArray = [Math.random(), Math.random()];
function createProxy()
// const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, handler);
const myProxy = createProxy();
And you can get the target of the proxy by myProxy.myTarget
:
console.log(myProxy.myTarget) // [0.22089416118932403, 0.08429264462405173]
console.log(myArray === myProxy.myTarget) // true
add a comment |
up vote
0
down vote
How about adding the following get trap:
const handler =
get: (target, property, receiver) =>
if (property === 'myTarget')
return target
return target[property]
const myArray = [Math.random(), Math.random()];
function createProxy()
// const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, handler);
const myProxy = createProxy();
And you can get the target of the proxy by myProxy.myTarget
:
console.log(myProxy.myTarget) // [0.22089416118932403, 0.08429264462405173]
console.log(myArray === myProxy.myTarget) // true
add a comment |
up vote
0
down vote
up vote
0
down vote
How about adding the following get trap:
const handler =
get: (target, property, receiver) =>
if (property === 'myTarget')
return target
return target[property]
const myArray = [Math.random(), Math.random()];
function createProxy()
// const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, handler);
const myProxy = createProxy();
And you can get the target of the proxy by myProxy.myTarget
:
console.log(myProxy.myTarget) // [0.22089416118932403, 0.08429264462405173]
console.log(myArray === myProxy.myTarget) // true
How about adding the following get trap:
const handler =
get: (target, property, receiver) =>
if (property === 'myTarget')
return target
return target[property]
const myArray = [Math.random(), Math.random()];
function createProxy()
// const myArray = [Math.random(), Math.random()];
return new Proxy(myArray, handler);
const myProxy = createProxy();
And you can get the target of the proxy by myProxy.myTarget
:
console.log(myProxy.myTarget) // [0.22089416118932403, 0.08429264462405173]
console.log(myArray === myProxy.myTarget) // true
edited Nov 22 at 13:25
answered Nov 22 at 13:20
Yuci
3,7012734
3,7012734
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51096547%2fhow-to-get-the-target-of-a-javascript-proxy%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R,NuaN U,ATh0QvL1t33aAMb E,8 sIwgB QlsBdxEc9VK0q,r
1
You can't. That's why it's a proxy. What are you trying to do, why do you need this?
– Bergi
Jun 29 at 7:54
@Bergi Because if I have an object with circular references, and these circular references are in proxies, there is no way to safe stringify my object. I'll get stack size exceeded error. :(
– Adam
Jun 29 at 7:59
1
Can you make an example of that, please? Circular references (I think you mean those, not dependencies) should work just fine with proxies, as
myProxy === myProxy
still holds. Nothing needs to get its hands on the target.– Bergi
Jun 29 at 8:02