How to communicate with parent application while it's in background?
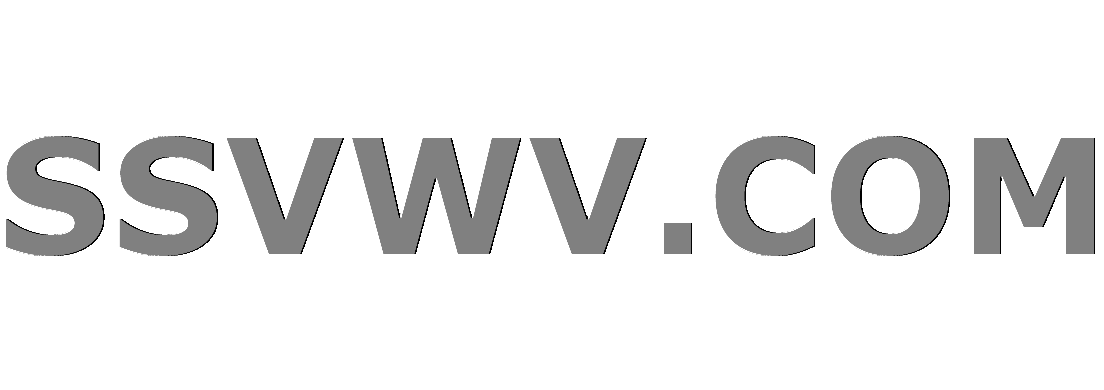
Multi tool use
I'm trying to develop watchOS 3 with Xamarin. My watch is communicating with parent application while it's active. When iOS app is killed or in background state, my watch doesn't receive any updated data. I'm sending request from the watch every 10 seconds in order to get updated data. I'm using WCSession for connection. The question is: is it possible to activate parent application from watch extension?
My functions for connectivity:
public void StartSession()
if (session != null)
session.Delegate = this;
session.ActivateSession();
Console.WriteLine($"Started Watch Connectivity Session on Device");
public override void SessionReachabilityDidChange(WCSession session)
Console.WriteLine($"Watch connectivity Reachable:(session.Reachable ? '✓' : '✗') from Device");
// handle session reachability change
if (session.Reachable)
// great! continue on with Interactive Messaging
else
// 😥 prompt the user to unlock their iOS device
#region Application Context Methods
public void UpdateApplicationContext(Dictionary<string, object> applicationContext)
// Application context doesnt need the watch to be reachable, it will be received when opened
if (validSession != null)
try
var NSValues = applicationContext.Values.Select(x => new NSString(JsonConvert.SerializeObject(x))).ToArray();
var NSKeys = applicationContext.Keys.Select(x => new NSString(x)).ToArray();
var NSApplicationContext = NSDictionary<NSString, NSObject>.FromObjectsAndKeys(NSValues, NSKeys);
NSError error;
var sendSuccessfully = validSession.UpdateApplicationContext(NSApplicationContext, out error);
if (sendSuccessfully)
Console.WriteLine($"Sent App Context from Device nPayLoad: NSApplicationContext.ToString() n");
else
Console.WriteLine($"Error Updating Application Context: error.LocalizedDescription");
catch (Exception ex)
Console.WriteLine($"Exception Updating Application Context: ex.Message");
public override void DidReceiveApplicationContext(WCSession session, NSDictionary<NSString, NSObject> applicationContext)
Console.WriteLine($"Receiving Message on Device");
if (ApplicationContextUpdated != null)
var keys = applicationContext.Keys.Select(k => k.ToString()).ToArray();
var values = applicationContext.Values.Select(v => JsonConvert.DeserializeObject(v.ToString())).ToArray();
var dictionary = keys.Zip(values, (k, v) => new Key = k, Value = v )
.ToDictionary(x => x.Key, x => x.Value);
ApplicationContextUpdated(session, dictionary);
#endregion
xamarin.ios watchkit watchconnectivity
add a comment |
I'm trying to develop watchOS 3 with Xamarin. My watch is communicating with parent application while it's active. When iOS app is killed or in background state, my watch doesn't receive any updated data. I'm sending request from the watch every 10 seconds in order to get updated data. I'm using WCSession for connection. The question is: is it possible to activate parent application from watch extension?
My functions for connectivity:
public void StartSession()
if (session != null)
session.Delegate = this;
session.ActivateSession();
Console.WriteLine($"Started Watch Connectivity Session on Device");
public override void SessionReachabilityDidChange(WCSession session)
Console.WriteLine($"Watch connectivity Reachable:(session.Reachable ? '✓' : '✗') from Device");
// handle session reachability change
if (session.Reachable)
// great! continue on with Interactive Messaging
else
// 😥 prompt the user to unlock their iOS device
#region Application Context Methods
public void UpdateApplicationContext(Dictionary<string, object> applicationContext)
// Application context doesnt need the watch to be reachable, it will be received when opened
if (validSession != null)
try
var NSValues = applicationContext.Values.Select(x => new NSString(JsonConvert.SerializeObject(x))).ToArray();
var NSKeys = applicationContext.Keys.Select(x => new NSString(x)).ToArray();
var NSApplicationContext = NSDictionary<NSString, NSObject>.FromObjectsAndKeys(NSValues, NSKeys);
NSError error;
var sendSuccessfully = validSession.UpdateApplicationContext(NSApplicationContext, out error);
if (sendSuccessfully)
Console.WriteLine($"Sent App Context from Device nPayLoad: NSApplicationContext.ToString() n");
else
Console.WriteLine($"Error Updating Application Context: error.LocalizedDescription");
catch (Exception ex)
Console.WriteLine($"Exception Updating Application Context: ex.Message");
public override void DidReceiveApplicationContext(WCSession session, NSDictionary<NSString, NSObject> applicationContext)
Console.WriteLine($"Receiving Message on Device");
if (ApplicationContextUpdated != null)
var keys = applicationContext.Keys.Select(k => k.ToString()).ToArray();
var values = applicationContext.Values.Select(v => JsonConvert.DeserializeObject(v.ToString())).ToArray();
var dictionary = keys.Zip(values, (k, v) => new Key = k, Value = v )
.ToDictionary(x => x.Key, x => x.Value);
ApplicationContextUpdated(session, dictionary);
#endregion
xamarin.ios watchkit watchconnectivity
OpenParentApplication works only in WatchOS1. WactchOS2,3 must communicate with WCSession and WatchConnectivity.
– Vitali
Nov 14 at 7:09
You need to create a background task in the iPhone app otherwise the OS kills your app off before it can finish downloading the data. Here's some docs to help: developer.apple.com/library/ios/documentation/iPhone/Conceptual/…
– Lucas Zhang - MSFT
Nov 14 at 8:09
add a comment |
I'm trying to develop watchOS 3 with Xamarin. My watch is communicating with parent application while it's active. When iOS app is killed or in background state, my watch doesn't receive any updated data. I'm sending request from the watch every 10 seconds in order to get updated data. I'm using WCSession for connection. The question is: is it possible to activate parent application from watch extension?
My functions for connectivity:
public void StartSession()
if (session != null)
session.Delegate = this;
session.ActivateSession();
Console.WriteLine($"Started Watch Connectivity Session on Device");
public override void SessionReachabilityDidChange(WCSession session)
Console.WriteLine($"Watch connectivity Reachable:(session.Reachable ? '✓' : '✗') from Device");
// handle session reachability change
if (session.Reachable)
// great! continue on with Interactive Messaging
else
// 😥 prompt the user to unlock their iOS device
#region Application Context Methods
public void UpdateApplicationContext(Dictionary<string, object> applicationContext)
// Application context doesnt need the watch to be reachable, it will be received when opened
if (validSession != null)
try
var NSValues = applicationContext.Values.Select(x => new NSString(JsonConvert.SerializeObject(x))).ToArray();
var NSKeys = applicationContext.Keys.Select(x => new NSString(x)).ToArray();
var NSApplicationContext = NSDictionary<NSString, NSObject>.FromObjectsAndKeys(NSValues, NSKeys);
NSError error;
var sendSuccessfully = validSession.UpdateApplicationContext(NSApplicationContext, out error);
if (sendSuccessfully)
Console.WriteLine($"Sent App Context from Device nPayLoad: NSApplicationContext.ToString() n");
else
Console.WriteLine($"Error Updating Application Context: error.LocalizedDescription");
catch (Exception ex)
Console.WriteLine($"Exception Updating Application Context: ex.Message");
public override void DidReceiveApplicationContext(WCSession session, NSDictionary<NSString, NSObject> applicationContext)
Console.WriteLine($"Receiving Message on Device");
if (ApplicationContextUpdated != null)
var keys = applicationContext.Keys.Select(k => k.ToString()).ToArray();
var values = applicationContext.Values.Select(v => JsonConvert.DeserializeObject(v.ToString())).ToArray();
var dictionary = keys.Zip(values, (k, v) => new Key = k, Value = v )
.ToDictionary(x => x.Key, x => x.Value);
ApplicationContextUpdated(session, dictionary);
#endregion
xamarin.ios watchkit watchconnectivity
I'm trying to develop watchOS 3 with Xamarin. My watch is communicating with parent application while it's active. When iOS app is killed or in background state, my watch doesn't receive any updated data. I'm sending request from the watch every 10 seconds in order to get updated data. I'm using WCSession for connection. The question is: is it possible to activate parent application from watch extension?
My functions for connectivity:
public void StartSession()
if (session != null)
session.Delegate = this;
session.ActivateSession();
Console.WriteLine($"Started Watch Connectivity Session on Device");
public override void SessionReachabilityDidChange(WCSession session)
Console.WriteLine($"Watch connectivity Reachable:(session.Reachable ? '✓' : '✗') from Device");
// handle session reachability change
if (session.Reachable)
// great! continue on with Interactive Messaging
else
// 😥 prompt the user to unlock their iOS device
#region Application Context Methods
public void UpdateApplicationContext(Dictionary<string, object> applicationContext)
// Application context doesnt need the watch to be reachable, it will be received when opened
if (validSession != null)
try
var NSValues = applicationContext.Values.Select(x => new NSString(JsonConvert.SerializeObject(x))).ToArray();
var NSKeys = applicationContext.Keys.Select(x => new NSString(x)).ToArray();
var NSApplicationContext = NSDictionary<NSString, NSObject>.FromObjectsAndKeys(NSValues, NSKeys);
NSError error;
var sendSuccessfully = validSession.UpdateApplicationContext(NSApplicationContext, out error);
if (sendSuccessfully)
Console.WriteLine($"Sent App Context from Device nPayLoad: NSApplicationContext.ToString() n");
else
Console.WriteLine($"Error Updating Application Context: error.LocalizedDescription");
catch (Exception ex)
Console.WriteLine($"Exception Updating Application Context: ex.Message");
public override void DidReceiveApplicationContext(WCSession session, NSDictionary<NSString, NSObject> applicationContext)
Console.WriteLine($"Receiving Message on Device");
if (ApplicationContextUpdated != null)
var keys = applicationContext.Keys.Select(k => k.ToString()).ToArray();
var values = applicationContext.Values.Select(v => JsonConvert.DeserializeObject(v.ToString())).ToArray();
var dictionary = keys.Zip(values, (k, v) => new Key = k, Value = v )
.ToDictionary(x => x.Key, x => x.Value);
ApplicationContextUpdated(session, dictionary);
#endregion
xamarin.ios watchkit watchconnectivity
xamarin.ios watchkit watchconnectivity
asked Nov 12 at 12:56


Vitali
119214
119214
OpenParentApplication works only in WatchOS1. WactchOS2,3 must communicate with WCSession and WatchConnectivity.
– Vitali
Nov 14 at 7:09
You need to create a background task in the iPhone app otherwise the OS kills your app off before it can finish downloading the data. Here's some docs to help: developer.apple.com/library/ios/documentation/iPhone/Conceptual/…
– Lucas Zhang - MSFT
Nov 14 at 8:09
add a comment |
OpenParentApplication works only in WatchOS1. WactchOS2,3 must communicate with WCSession and WatchConnectivity.
– Vitali
Nov 14 at 7:09
You need to create a background task in the iPhone app otherwise the OS kills your app off before it can finish downloading the data. Here's some docs to help: developer.apple.com/library/ios/documentation/iPhone/Conceptual/…
– Lucas Zhang - MSFT
Nov 14 at 8:09
OpenParentApplication works only in WatchOS1. WactchOS2,3 must communicate with WCSession and WatchConnectivity.
– Vitali
Nov 14 at 7:09
OpenParentApplication works only in WatchOS1. WactchOS2,3 must communicate with WCSession and WatchConnectivity.
– Vitali
Nov 14 at 7:09
You need to create a background task in the iPhone app otherwise the OS kills your app off before it can finish downloading the data. Here's some docs to help: developer.apple.com/library/ios/documentation/iPhone/Conceptual/…
– Lucas Zhang - MSFT
Nov 14 at 8:09
You need to create a background task in the iPhone app otherwise the OS kills your app off before it can finish downloading the data. Here's some docs to help: developer.apple.com/library/ios/documentation/iPhone/Conceptual/…
– Lucas Zhang - MSFT
Nov 14 at 8:09
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53262656%2fhow-to-communicate-with-parent-application-while-its-in-background%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53262656%2fhow-to-communicate-with-parent-application-while-its-in-background%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VMECOFy9LOcubbK8AtUgvH iy
OpenParentApplication works only in WatchOS1. WactchOS2,3 must communicate with WCSession and WatchConnectivity.
– Vitali
Nov 14 at 7:09
You need to create a background task in the iPhone app otherwise the OS kills your app off before it can finish downloading the data. Here's some docs to help: developer.apple.com/library/ios/documentation/iPhone/Conceptual/…
– Lucas Zhang - MSFT
Nov 14 at 8:09