How can i place text widget at the horizontal end of a container with a max width, without expanding it
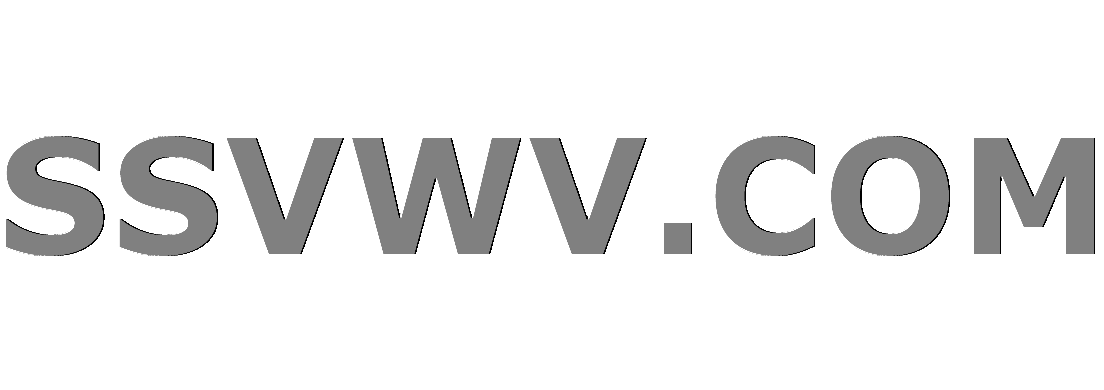
Multi tool use
I have a card widget which wraps a container widget with a max width given to it, this is what it looks like :
this is the code below:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
],
),
),
],
),
))
problem arises when i try to create another text widget under it, that i specifically want to move to the extreme right below the first text, this is what i get:
The 2nd text widget i added expands, goes to the extreme end as i wanted , but also expands the card to the max-width, i set. This is the code for it:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
Text("3:18am")
],
)
],
),
),
],
),
))
But this is what i want
where the date is is horizontally at the end of the container space the initial text created without it being expanded.
How do i solve this issue ?

add a comment |
I have a card widget which wraps a container widget with a max width given to it, this is what it looks like :
this is the code below:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
],
),
),
],
),
))
problem arises when i try to create another text widget under it, that i specifically want to move to the extreme right below the first text, this is what i get:
The 2nd text widget i added expands, goes to the extreme end as i wanted , but also expands the card to the max-width, i set. This is the code for it:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
Text("3:18am")
],
)
],
),
),
],
),
))
But this is what i want
where the date is is horizontally at the end of the container space the initial text created without it being expanded.
How do i solve this issue ?

add a comment |
I have a card widget which wraps a container widget with a max width given to it, this is what it looks like :
this is the code below:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
],
),
),
],
),
))
problem arises when i try to create another text widget under it, that i specifically want to move to the extreme right below the first text, this is what i get:
The 2nd text widget i added expands, goes to the extreme end as i wanted , but also expands the card to the max-width, i set. This is the code for it:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
Text("3:18am")
],
)
],
),
),
],
),
))
But this is what i want
where the date is is horizontally at the end of the container space the initial text created without it being expanded.
How do i solve this issue ?

I have a card widget which wraps a container widget with a max width given to it, this is what it looks like :
this is the code below:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
],
),
),
],
),
))
problem arises when i try to create another text widget under it, that i specifically want to move to the extreme right below the first text, this is what i get:
The 2nd text widget i added expands, goes to the extreme end as i wanted , but also expands the card to the max-width, i set. This is the code for it:
Card(
child: Container(
constraints: new BoxConstraints(
maxWidth: 250.0,
),
margin: EdgeInsets.symmetric(horizontal: 10.0, vertical: 10.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
mainAxisSize: MainAxisSize.min,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Flexible(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(messageSnapshot.inbox),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
children: <Widget>[
Text("3:18am")
],
)
],
),
),
],
),
))
But this is what i want
where the date is is horizontally at the end of the container space the initial text created without it being expanded.
How do i solve this issue ?


asked Nov 13 '18 at 1:18


Oto-obong EshiettOto-obong Eshiett
107111
107111
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Wrap the elements inside the Flexible
widget inside a IntrinsicWidth
widget. This will make all children in the Column
have the same width. Then, inside the Row
for the second element add a MainAxisSize.max
property, so it will mimic the first element width and obey the MainAxisAlign.end
property the way you want to.
new Flexible(
child: new IntrinsicWidth(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(
messageSnapshot.inbox,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"3:18am",
),
],
)
],
),
),
),
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272398%2fhow-can-i-place-text-widget-at-the-horizontal-end-of-a-container-with-a-max-widt%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Wrap the elements inside the Flexible
widget inside a IntrinsicWidth
widget. This will make all children in the Column
have the same width. Then, inside the Row
for the second element add a MainAxisSize.max
property, so it will mimic the first element width and obey the MainAxisAlign.end
property the way you want to.
new Flexible(
child: new IntrinsicWidth(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(
messageSnapshot.inbox,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"3:18am",
),
],
)
],
),
),
),
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
add a comment |
Wrap the elements inside the Flexible
widget inside a IntrinsicWidth
widget. This will make all children in the Column
have the same width. Then, inside the Row
for the second element add a MainAxisSize.max
property, so it will mimic the first element width and obey the MainAxisAlign.end
property the way you want to.
new Flexible(
child: new IntrinsicWidth(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(
messageSnapshot.inbox,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"3:18am",
),
],
)
],
),
),
),
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
add a comment |
Wrap the elements inside the Flexible
widget inside a IntrinsicWidth
widget. This will make all children in the Column
have the same width. Then, inside the Row
for the second element add a MainAxisSize.max
property, so it will mimic the first element width and obey the MainAxisAlign.end
property the way you want to.
new Flexible(
child: new IntrinsicWidth(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(
messageSnapshot.inbox,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"3:18am",
),
],
)
],
),
),
),
Wrap the elements inside the Flexible
widget inside a IntrinsicWidth
widget. This will make all children in the Column
have the same width. Then, inside the Row
for the second element add a MainAxisSize.max
property, so it will mimic the first element width and obey the MainAxisAlign.end
property the way you want to.
new Flexible(
child: new IntrinsicWidth(
child: new Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
new Container(
margin: const EdgeInsets.only(top: 5.0),
child: new Text(
messageSnapshot.inbox,
),
),
Row(
mainAxisAlignment: MainAxisAlignment.end,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Text(
"3:18am",
),
],
)
],
),
),
),
edited Nov 13 '18 at 5:48


CopsOnRoad
2,98411018
2,98411018
answered Nov 13 '18 at 4:50


CardoCardo
514
514
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
add a comment |
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
Perfecto!!!!!!! It worked
– Oto-obong Eshiett
Nov 14 '18 at 12:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272398%2fhow-can-i-place-text-widget-at-the-horizontal-end-of-a-container-with-a-max-widt%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YUbN1B7,mk14Z26qcqa4YM9h1J8nYDF3V3Ov uejF ausvvZ2RPvx