Case insensitive hashmap for strings in java
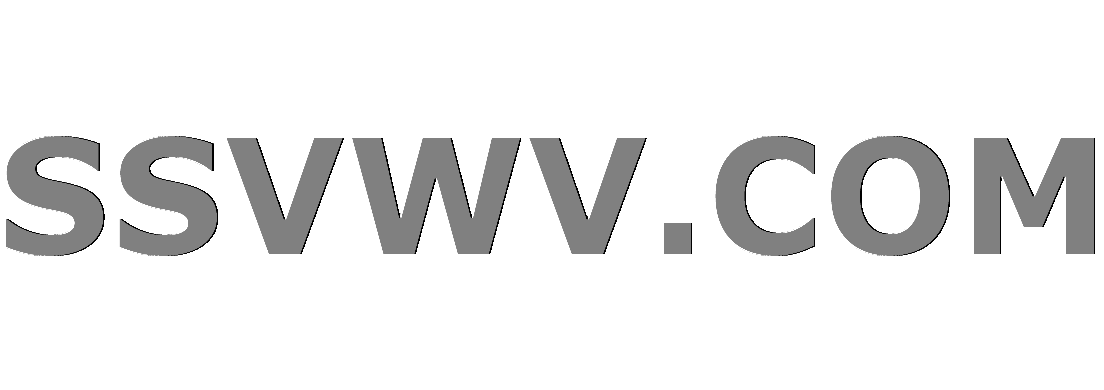
Multi tool use
I came across this post for a case insensitive hashmap and tried to implement it but I'm not getting the expected result. For some reason it's not returning the value when I do get with a different casing and is returning null, and I thought that you didn't really need a non-default constructor in this case but I'm not sure.
public class CaseInsensitiveMap extends HashMap<String, Integer>
@Override
public Integer put(String key, Integer value)
return super.put(key.toLowerCase(), value);
// not @Override because that would require the key parameter to be of type Object
public Integer get(String key)
return super.get(key.toLowerCase());
and used like so;
HashMap<String, Integer> stuff = new CaseInsensitiveMap();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
but result is;
happy=11
null
happy=11
java case superclass
add a comment |
I came across this post for a case insensitive hashmap and tried to implement it but I'm not getting the expected result. For some reason it's not returning the value when I do get with a different casing and is returning null, and I thought that you didn't really need a non-default constructor in this case but I'm not sure.
public class CaseInsensitiveMap extends HashMap<String, Integer>
@Override
public Integer put(String key, Integer value)
return super.put(key.toLowerCase(), value);
// not @Override because that would require the key parameter to be of type Object
public Integer get(String key)
return super.get(key.toLowerCase());
and used like so;
HashMap<String, Integer> stuff = new CaseInsensitiveMap();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
but result is;
happy=11
null
happy=11
java case superclass
same link but different answer stackoverflow.com/a/22336599/2310289 - using case insensitive treemap
– Scary Wombat
Nov 13 '18 at 2:20
add a comment |
I came across this post for a case insensitive hashmap and tried to implement it but I'm not getting the expected result. For some reason it's not returning the value when I do get with a different casing and is returning null, and I thought that you didn't really need a non-default constructor in this case but I'm not sure.
public class CaseInsensitiveMap extends HashMap<String, Integer>
@Override
public Integer put(String key, Integer value)
return super.put(key.toLowerCase(), value);
// not @Override because that would require the key parameter to be of type Object
public Integer get(String key)
return super.get(key.toLowerCase());
and used like so;
HashMap<String, Integer> stuff = new CaseInsensitiveMap();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
but result is;
happy=11
null
happy=11
java case superclass
I came across this post for a case insensitive hashmap and tried to implement it but I'm not getting the expected result. For some reason it's not returning the value when I do get with a different casing and is returning null, and I thought that you didn't really need a non-default constructor in this case but I'm not sure.
public class CaseInsensitiveMap extends HashMap<String, Integer>
@Override
public Integer put(String key, Integer value)
return super.put(key.toLowerCase(), value);
// not @Override because that would require the key parameter to be of type Object
public Integer get(String key)
return super.get(key.toLowerCase());
and used like so;
HashMap<String, Integer> stuff = new CaseInsensitiveMap();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
but result is;
happy=11
null
happy=11
java case superclass
java case superclass
edited Nov 13 '18 at 3:37


buræquete
5,11141748
5,11141748
asked Nov 13 '18 at 2:06


Shinji-sanShinji-san
469614
469614
same link but different answer stackoverflow.com/a/22336599/2310289 - using case insensitive treemap
– Scary Wombat
Nov 13 '18 at 2:20
add a comment |
same link but different answer stackoverflow.com/a/22336599/2310289 - using case insensitive treemap
– Scary Wombat
Nov 13 '18 at 2:20
same link but different answer stackoverflow.com/a/22336599/2310289 - using case insensitive treemap
– Scary Wombat
Nov 13 '18 at 2:20
same link but different answer stackoverflow.com/a/22336599/2310289 - using case insensitive treemap
– Scary Wombat
Nov 13 '18 at 2:20
add a comment |
2 Answers
2
active
oldest
votes
As String is marked as final, may consider extends CharSequence
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class CaseInsensitiveMap<K extends CharSequence, V> implements Map<K, V>
private Map<K, V> map = new HashMap<K, V>();
@Override
public int size()
return map.size();
@Override
public boolean isEmpty()
return map.isEmpty();
@Override
public boolean containsKey(Object key)
return map.containsKey(key.toString().toLowerCase());
@Override
public boolean containsValue(Object value)
return map.containsValue(value);
@Override
public V get(Object key)
return map.get(key.toString().toLowerCase());
@Override
public V put(K key, V value)
return map.put((K) key.toString().toLowerCase(), value);
@Override
public V remove(Object key)
return map.remove(key.toString().toLowerCase());
@Override
public void putAll(Map<? extends K, ? extends V> m)
map.putAll(m);
@Override
public void clear()
map.clear();
@Override
public Set<K> keySet()
return map.keySet();
@Override
public Collection<V> values()
return map.values();
@Override
public Set<java.util.Map.Entry<K, V> > entrySet()
return map.entrySet();
@Override
public String toString()
return map.toString();
The testing class needed to modified as the following:
import java.util.*;
import java.io.File;
import java.io.FileNotFoundException;
public class Tester
public static void main(String args)
Map<String, Integer> stuff = new CaseInsensitiveMap<String, Integer>();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
add a comment |
Simple fix;
CaseInsensitiveMap stuff = new CaseInsensitiveMap();
prints out;
happy=11
11
happy=11
CaseInsensitiveMap
is extending HashMap<String, Integer>
so it is a subclass of it, the fact that you reference stuff
as HashMap
(as the superclass) allows it to use default get
method. You can even see in an IDE that your custom get(String)
in CaseInsensitiveMap
is not even used.
Only overridden methods will be used if you use superclass reference for a subclass, as you've done in your code. That is why only your custom put(String, Integer)
method works since it is overriding the method in super
.
Referencing Subclass objects with Subclass vs Superclass reference for more info on that issue.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272732%2fcase-insensitive-hashmap-for-strings-in-java%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As String is marked as final, may consider extends CharSequence
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class CaseInsensitiveMap<K extends CharSequence, V> implements Map<K, V>
private Map<K, V> map = new HashMap<K, V>();
@Override
public int size()
return map.size();
@Override
public boolean isEmpty()
return map.isEmpty();
@Override
public boolean containsKey(Object key)
return map.containsKey(key.toString().toLowerCase());
@Override
public boolean containsValue(Object value)
return map.containsValue(value);
@Override
public V get(Object key)
return map.get(key.toString().toLowerCase());
@Override
public V put(K key, V value)
return map.put((K) key.toString().toLowerCase(), value);
@Override
public V remove(Object key)
return map.remove(key.toString().toLowerCase());
@Override
public void putAll(Map<? extends K, ? extends V> m)
map.putAll(m);
@Override
public void clear()
map.clear();
@Override
public Set<K> keySet()
return map.keySet();
@Override
public Collection<V> values()
return map.values();
@Override
public Set<java.util.Map.Entry<K, V> > entrySet()
return map.entrySet();
@Override
public String toString()
return map.toString();
The testing class needed to modified as the following:
import java.util.*;
import java.io.File;
import java.io.FileNotFoundException;
public class Tester
public static void main(String args)
Map<String, Integer> stuff = new CaseInsensitiveMap<String, Integer>();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
add a comment |
As String is marked as final, may consider extends CharSequence
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class CaseInsensitiveMap<K extends CharSequence, V> implements Map<K, V>
private Map<K, V> map = new HashMap<K, V>();
@Override
public int size()
return map.size();
@Override
public boolean isEmpty()
return map.isEmpty();
@Override
public boolean containsKey(Object key)
return map.containsKey(key.toString().toLowerCase());
@Override
public boolean containsValue(Object value)
return map.containsValue(value);
@Override
public V get(Object key)
return map.get(key.toString().toLowerCase());
@Override
public V put(K key, V value)
return map.put((K) key.toString().toLowerCase(), value);
@Override
public V remove(Object key)
return map.remove(key.toString().toLowerCase());
@Override
public void putAll(Map<? extends K, ? extends V> m)
map.putAll(m);
@Override
public void clear()
map.clear();
@Override
public Set<K> keySet()
return map.keySet();
@Override
public Collection<V> values()
return map.values();
@Override
public Set<java.util.Map.Entry<K, V> > entrySet()
return map.entrySet();
@Override
public String toString()
return map.toString();
The testing class needed to modified as the following:
import java.util.*;
import java.io.File;
import java.io.FileNotFoundException;
public class Tester
public static void main(String args)
Map<String, Integer> stuff = new CaseInsensitiveMap<String, Integer>();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
add a comment |
As String is marked as final, may consider extends CharSequence
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class CaseInsensitiveMap<K extends CharSequence, V> implements Map<K, V>
private Map<K, V> map = new HashMap<K, V>();
@Override
public int size()
return map.size();
@Override
public boolean isEmpty()
return map.isEmpty();
@Override
public boolean containsKey(Object key)
return map.containsKey(key.toString().toLowerCase());
@Override
public boolean containsValue(Object value)
return map.containsValue(value);
@Override
public V get(Object key)
return map.get(key.toString().toLowerCase());
@Override
public V put(K key, V value)
return map.put((K) key.toString().toLowerCase(), value);
@Override
public V remove(Object key)
return map.remove(key.toString().toLowerCase());
@Override
public void putAll(Map<? extends K, ? extends V> m)
map.putAll(m);
@Override
public void clear()
map.clear();
@Override
public Set<K> keySet()
return map.keySet();
@Override
public Collection<V> values()
return map.values();
@Override
public Set<java.util.Map.Entry<K, V> > entrySet()
return map.entrySet();
@Override
public String toString()
return map.toString();
The testing class needed to modified as the following:
import java.util.*;
import java.io.File;
import java.io.FileNotFoundException;
public class Tester
public static void main(String args)
Map<String, Integer> stuff = new CaseInsensitiveMap<String, Integer>();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
As String is marked as final, may consider extends CharSequence
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class CaseInsensitiveMap<K extends CharSequence, V> implements Map<K, V>
private Map<K, V> map = new HashMap<K, V>();
@Override
public int size()
return map.size();
@Override
public boolean isEmpty()
return map.isEmpty();
@Override
public boolean containsKey(Object key)
return map.containsKey(key.toString().toLowerCase());
@Override
public boolean containsValue(Object value)
return map.containsValue(value);
@Override
public V get(Object key)
return map.get(key.toString().toLowerCase());
@Override
public V put(K key, V value)
return map.put((K) key.toString().toLowerCase(), value);
@Override
public V remove(Object key)
return map.remove(key.toString().toLowerCase());
@Override
public void putAll(Map<? extends K, ? extends V> m)
map.putAll(m);
@Override
public void clear()
map.clear();
@Override
public Set<K> keySet()
return map.keySet();
@Override
public Collection<V> values()
return map.values();
@Override
public Set<java.util.Map.Entry<K, V> > entrySet()
return map.entrySet();
@Override
public String toString()
return map.toString();
The testing class needed to modified as the following:
import java.util.*;
import java.io.File;
import java.io.FileNotFoundException;
public class Tester
public static void main(String args)
Map<String, Integer> stuff = new CaseInsensitiveMap<String, Integer>();
stuff.put("happy", 11);
System.out.println(stuff);
Integer result = stuff.get("HAPPy");
System.out.println(result);
System.out.println(stuff);
edited Nov 13 '18 at 2:54
answered Nov 13 '18 at 2:49


Miller Cy ChanMiller Cy Chan
20929
20929
add a comment |
add a comment |
Simple fix;
CaseInsensitiveMap stuff = new CaseInsensitiveMap();
prints out;
happy=11
11
happy=11
CaseInsensitiveMap
is extending HashMap<String, Integer>
so it is a subclass of it, the fact that you reference stuff
as HashMap
(as the superclass) allows it to use default get
method. You can even see in an IDE that your custom get(String)
in CaseInsensitiveMap
is not even used.
Only overridden methods will be used if you use superclass reference for a subclass, as you've done in your code. That is why only your custom put(String, Integer)
method works since it is overriding the method in super
.
Referencing Subclass objects with Subclass vs Superclass reference for more info on that issue.
add a comment |
Simple fix;
CaseInsensitiveMap stuff = new CaseInsensitiveMap();
prints out;
happy=11
11
happy=11
CaseInsensitiveMap
is extending HashMap<String, Integer>
so it is a subclass of it, the fact that you reference stuff
as HashMap
(as the superclass) allows it to use default get
method. You can even see in an IDE that your custom get(String)
in CaseInsensitiveMap
is not even used.
Only overridden methods will be used if you use superclass reference for a subclass, as you've done in your code. That is why only your custom put(String, Integer)
method works since it is overriding the method in super
.
Referencing Subclass objects with Subclass vs Superclass reference for more info on that issue.
add a comment |
Simple fix;
CaseInsensitiveMap stuff = new CaseInsensitiveMap();
prints out;
happy=11
11
happy=11
CaseInsensitiveMap
is extending HashMap<String, Integer>
so it is a subclass of it, the fact that you reference stuff
as HashMap
(as the superclass) allows it to use default get
method. You can even see in an IDE that your custom get(String)
in CaseInsensitiveMap
is not even used.
Only overridden methods will be used if you use superclass reference for a subclass, as you've done in your code. That is why only your custom put(String, Integer)
method works since it is overriding the method in super
.
Referencing Subclass objects with Subclass vs Superclass reference for more info on that issue.
Simple fix;
CaseInsensitiveMap stuff = new CaseInsensitiveMap();
prints out;
happy=11
11
happy=11
CaseInsensitiveMap
is extending HashMap<String, Integer>
so it is a subclass of it, the fact that you reference stuff
as HashMap
(as the superclass) allows it to use default get
method. You can even see in an IDE that your custom get(String)
in CaseInsensitiveMap
is not even used.
Only overridden methods will be used if you use superclass reference for a subclass, as you've done in your code. That is why only your custom put(String, Integer)
method works since it is overriding the method in super
.
Referencing Subclass objects with Subclass vs Superclass reference for more info on that issue.
edited Nov 13 '18 at 3:37
answered Nov 13 '18 at 2:58


buræqueteburæquete
5,11141748
5,11141748
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272732%2fcase-insensitive-hashmap-for-strings-in-java%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
clomc VCeaXn0n 8X8wuC1Ot bddmXmx5MfrUZ3 Z2g,92Bo,dni
same link but different answer stackoverflow.com/a/22336599/2310289 - using case insensitive treemap
– Scary Wombat
Nov 13 '18 at 2:20