Jackson convertValue does not use JavaTimeModule
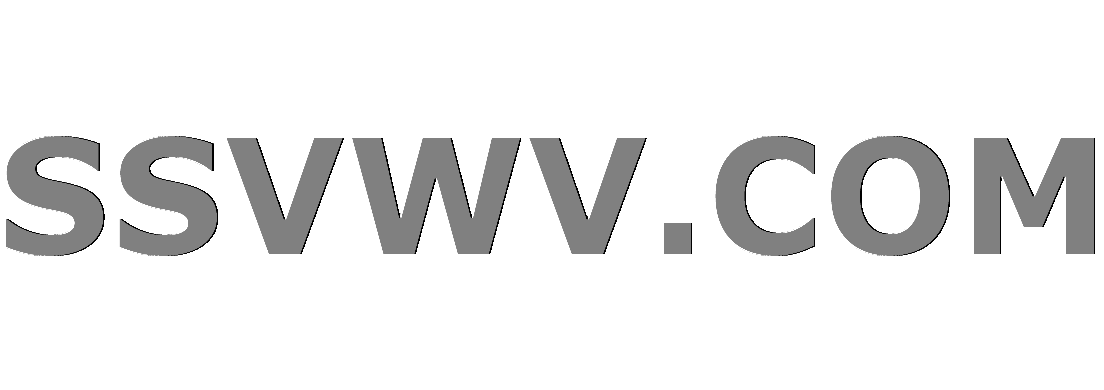
Multi tool use
If I make a POJO class and convert the whole objects to Json using the mapper.writeValueAsString()
method, or let Spring handle the conversion, the JavaTimeModule
is used appropriately and If my POJO had an OffsetDateTime
in it, it will get converted to a string like 2018-10-16T13:49:34.564748+02:00
.
For some scenarios, I need to use the mapper's T convertValue(Object fromValue, Class<T> toValueType)
method and build an ObjectNode
by hand. The method does not convert the OffsetDateTime
objects the same way as the writeValueAsString
.
I construct my Jackson mapper like this:
ObjectMapper defaultMapperObj = new ObjectMapper();
defaultMapperObj.registerModule(new GuavaModule());
defaultMapperObj.registerModule(new Jdk8Module());
defaultMapperObj.registerModule(new JavaTimeModule());
defaultMapperObj.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
Using the mapper, I can easily convert even Lists to their Json strings:
mapper.convertValue(
Arrays.asList(1,2,23), JsonNode.class
)
Results in:
ArrayNode: [1,2,23]
But If I call this:
mappper.convertValue(OffsetDateTime.now(), JsonNode.class)
I get a DecimalNode
: 1542287917.2141993
java json jackson
add a comment |
If I make a POJO class and convert the whole objects to Json using the mapper.writeValueAsString()
method, or let Spring handle the conversion, the JavaTimeModule
is used appropriately and If my POJO had an OffsetDateTime
in it, it will get converted to a string like 2018-10-16T13:49:34.564748+02:00
.
For some scenarios, I need to use the mapper's T convertValue(Object fromValue, Class<T> toValueType)
method and build an ObjectNode
by hand. The method does not convert the OffsetDateTime
objects the same way as the writeValueAsString
.
I construct my Jackson mapper like this:
ObjectMapper defaultMapperObj = new ObjectMapper();
defaultMapperObj.registerModule(new GuavaModule());
defaultMapperObj.registerModule(new Jdk8Module());
defaultMapperObj.registerModule(new JavaTimeModule());
defaultMapperObj.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
Using the mapper, I can easily convert even Lists to their Json strings:
mapper.convertValue(
Arrays.asList(1,2,23), JsonNode.class
)
Results in:
ArrayNode: [1,2,23]
But If I call this:
mappper.convertValue(OffsetDateTime.now(), JsonNode.class)
I get a DecimalNode
: 1542287917.2141993
java json jackson
add a comment |
If I make a POJO class and convert the whole objects to Json using the mapper.writeValueAsString()
method, or let Spring handle the conversion, the JavaTimeModule
is used appropriately and If my POJO had an OffsetDateTime
in it, it will get converted to a string like 2018-10-16T13:49:34.564748+02:00
.
For some scenarios, I need to use the mapper's T convertValue(Object fromValue, Class<T> toValueType)
method and build an ObjectNode
by hand. The method does not convert the OffsetDateTime
objects the same way as the writeValueAsString
.
I construct my Jackson mapper like this:
ObjectMapper defaultMapperObj = new ObjectMapper();
defaultMapperObj.registerModule(new GuavaModule());
defaultMapperObj.registerModule(new Jdk8Module());
defaultMapperObj.registerModule(new JavaTimeModule());
defaultMapperObj.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
Using the mapper, I can easily convert even Lists to their Json strings:
mapper.convertValue(
Arrays.asList(1,2,23), JsonNode.class
)
Results in:
ArrayNode: [1,2,23]
But If I call this:
mappper.convertValue(OffsetDateTime.now(), JsonNode.class)
I get a DecimalNode
: 1542287917.2141993
java json jackson
If I make a POJO class and convert the whole objects to Json using the mapper.writeValueAsString()
method, or let Spring handle the conversion, the JavaTimeModule
is used appropriately and If my POJO had an OffsetDateTime
in it, it will get converted to a string like 2018-10-16T13:49:34.564748+02:00
.
For some scenarios, I need to use the mapper's T convertValue(Object fromValue, Class<T> toValueType)
method and build an ObjectNode
by hand. The method does not convert the OffsetDateTime
objects the same way as the writeValueAsString
.
I construct my Jackson mapper like this:
ObjectMapper defaultMapperObj = new ObjectMapper();
defaultMapperObj.registerModule(new GuavaModule());
defaultMapperObj.registerModule(new Jdk8Module());
defaultMapperObj.registerModule(new JavaTimeModule());
defaultMapperObj.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
Using the mapper, I can easily convert even Lists to their Json strings:
mapper.convertValue(
Arrays.asList(1,2,23), JsonNode.class
)
Results in:
ArrayNode: [1,2,23]
But If I call this:
mappper.convertValue(OffsetDateTime.now(), JsonNode.class)
I get a DecimalNode
: 1542287917.2141993
java json jackson
java json jackson
edited Nov 15 '18 at 14:21
appl3r
asked Nov 15 '18 at 13:19


appl3rappl3r
83611338
83611338
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
From the JavaTimeModule
documentation:
Most
java.time
types are serialized as numbers (integers or decimals as appropriate) if theSerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature is enabled, and otherwise are serialized in standard ISO-8601 string representation. [...]
So, to achieve the desired result, disable the SerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature in your ObjectMapper
instance:
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320401%2fjackson-convertvalue-does-not-use-javatimemodule%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
From the JavaTimeModule
documentation:
Most
java.time
types are serialized as numbers (integers or decimals as appropriate) if theSerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature is enabled, and otherwise are serialized in standard ISO-8601 string representation. [...]
So, to achieve the desired result, disable the SerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature in your ObjectMapper
instance:
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
add a comment |
From the JavaTimeModule
documentation:
Most
java.time
types are serialized as numbers (integers or decimals as appropriate) if theSerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature is enabled, and otherwise are serialized in standard ISO-8601 string representation. [...]
So, to achieve the desired result, disable the SerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature in your ObjectMapper
instance:
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
add a comment |
From the JavaTimeModule
documentation:
Most
java.time
types are serialized as numbers (integers or decimals as appropriate) if theSerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature is enabled, and otherwise are serialized in standard ISO-8601 string representation. [...]
So, to achieve the desired result, disable the SerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature in your ObjectMapper
instance:
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
From the JavaTimeModule
documentation:
Most
java.time
types are serialized as numbers (integers or decimals as appropriate) if theSerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature is enabled, and otherwise are serialized in standard ISO-8601 string representation. [...]
So, to achieve the desired result, disable the SerializationFeature.WRITE_DATES_AS_TIMESTAMPS
feature in your ObjectMapper
instance:
mapper.disable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS);
edited Nov 15 '18 at 13:30
answered Nov 15 '18 at 13:22


cassiomolincassiomolin
60.1k18115190
60.1k18115190
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320401%2fjackson-convertvalue-does-not-use-javatimemodule%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dU0hUKveeYQfi,y5j5ag,cUwrrAswfISzz30DlGGQRvoXaTRZagJWM uThJ,eO,3vSn EqDIr,8GDW0