How to convert DocumentClient to IDocumentClient in gremlin?
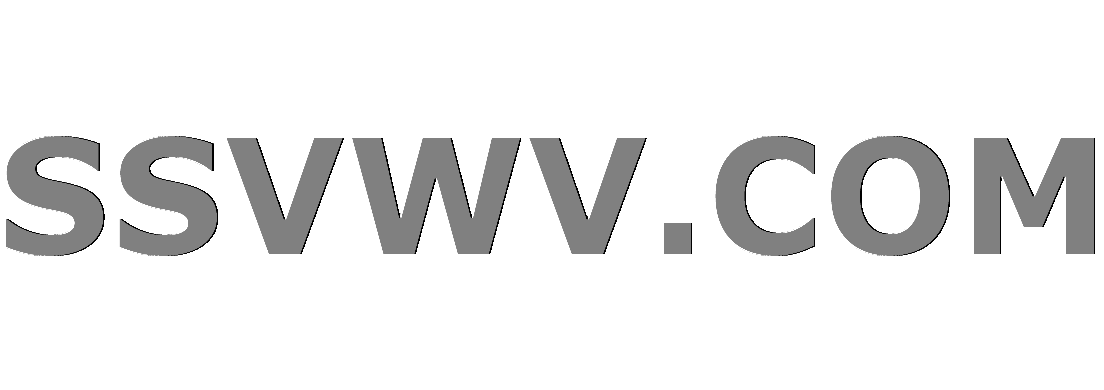
Multi tool use
I am using cosmos db to store and fetch data. Previously I was using DocumentClient like:
public class ProductRepository : IProductRepository
private DocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(DocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
}
But it is not unit testable so I want to convert it to IDocumentClient but IDocumentClient doesn't contain definition for CreateGremlinQuery. So what is the best possible way to convert my methods so that they will be using IDocumentClient? Do I need to use CreateDocumentQuery? if yes, how can I convert CreateGremlimQuery to CreateDocumentQuery?
asp.net-core

add a comment |
I am using cosmos db to store and fetch data. Previously I was using DocumentClient like:
public class ProductRepository : IProductRepository
private DocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(DocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
}
But it is not unit testable so I want to convert it to IDocumentClient but IDocumentClient doesn't contain definition for CreateGremlinQuery. So what is the best possible way to convert my methods so that they will be using IDocumentClient? Do I need to use CreateDocumentQuery? if yes, how can I convert CreateGremlimQuery to CreateDocumentQuery?
asp.net-core

add a comment |
I am using cosmos db to store and fetch data. Previously I was using DocumentClient like:
public class ProductRepository : IProductRepository
private DocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(DocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
}
But it is not unit testable so I want to convert it to IDocumentClient but IDocumentClient doesn't contain definition for CreateGremlinQuery. So what is the best possible way to convert my methods so that they will be using IDocumentClient? Do I need to use CreateDocumentQuery? if yes, how can I convert CreateGremlimQuery to CreateDocumentQuery?
asp.net-core

I am using cosmos db to store and fetch data. Previously I was using DocumentClient like:
public class ProductRepository : IProductRepository
private DocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(DocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = _documentClient.CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
}
But it is not unit testable so I want to convert it to IDocumentClient but IDocumentClient doesn't contain definition for CreateGremlinQuery. So what is the best possible way to convert my methods so that they will be using IDocumentClient? Do I need to use CreateDocumentQuery? if yes, how can I convert CreateGremlimQuery to CreateDocumentQuery?
asp.net-core

asp.net-core

edited Nov 15 '18 at 20:05


Stanislav Kralin
7,91941943
7,91941943
asked Nov 15 '18 at 13:25
AskAsk
294215
294215
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
There are several ways to get around that. The simplest one would be to simply hard cast your IDocumentClient to DocumentClient.
If you go with that approach your code becomes:
public class ProductRepository : IProductRepository
private IDocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(IDocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
You could also create your own extensions for IDocumentClient
.
public static class MoreGraphExtensions
public static IDocumentQuery<T> CreateGremlinQuery<T>(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<T>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
public static IDocumentQuery<object> CreateGremlinQuery(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<object>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
It is a pre-release however, so I do think that Microsoft will get around moving the extension methods at the interface level.
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320521%2fhow-to-convert-documentclient-to-idocumentclient-in-gremlin%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There are several ways to get around that. The simplest one would be to simply hard cast your IDocumentClient to DocumentClient.
If you go with that approach your code becomes:
public class ProductRepository : IProductRepository
private IDocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(IDocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
You could also create your own extensions for IDocumentClient
.
public static class MoreGraphExtensions
public static IDocumentQuery<T> CreateGremlinQuery<T>(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<T>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
public static IDocumentQuery<object> CreateGremlinQuery(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<object>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
It is a pre-release however, so I do think that Microsoft will get around moving the extension methods at the interface level.
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
|
show 1 more comment
There are several ways to get around that. The simplest one would be to simply hard cast your IDocumentClient to DocumentClient.
If you go with that approach your code becomes:
public class ProductRepository : IProductRepository
private IDocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(IDocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
You could also create your own extensions for IDocumentClient
.
public static class MoreGraphExtensions
public static IDocumentQuery<T> CreateGremlinQuery<T>(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<T>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
public static IDocumentQuery<object> CreateGremlinQuery(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<object>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
It is a pre-release however, so I do think that Microsoft will get around moving the extension methods at the interface level.
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
|
show 1 more comment
There are several ways to get around that. The simplest one would be to simply hard cast your IDocumentClient to DocumentClient.
If you go with that approach your code becomes:
public class ProductRepository : IProductRepository
private IDocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(IDocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
You could also create your own extensions for IDocumentClient
.
public static class MoreGraphExtensions
public static IDocumentQuery<T> CreateGremlinQuery<T>(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<T>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
public static IDocumentQuery<object> CreateGremlinQuery(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<object>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
It is a pre-release however, so I do think that Microsoft will get around moving the extension methods at the interface level.
There are several ways to get around that. The simplest one would be to simply hard cast your IDocumentClient to DocumentClient.
If you go with that approach your code becomes:
public class ProductRepository : IProductRepository
private IDocumentClient _documentClient;
private DocumentCollection _graphCollection;
public ProductRepository(IDocumentClient documentClient, DocumentCollection graphCollection)
_documentClient = documentClient;
_graphCollection = graphCollection;
public async Task Create(Product product)
var createQuery = CreateQuery(product);
IDocumentQuery<dynamic> query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, createQuery);
if(query.HasMoreResults)
await query.ExecuteNextAsync();
public async Task<Product> Get(string id)
Product product = null;
var getQuery = @"g.V('" + id + "')";
var query = ((DocumentClient)_documentClient).CreateGremlinQuery<dynamic>(_graphCollection, getQuery);
if (query.HasMoreResults)
var result = await query.ExecuteNextAsync();
if (result.Count == 0)
return product;
var productData = (JObject)result.FirstOrDefault();
product = new Product
name = productData["name"].ToString()
;
return product;
You could also create your own extensions for IDocumentClient
.
public static class MoreGraphExtensions
public static IDocumentQuery<T> CreateGremlinQuery<T>(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<T>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
public static IDocumentQuery<object> CreateGremlinQuery(this IDocumentClient documentClient, DocumentCollection collection, string gremlinExpression, FeedOptions feedOptions = null, GraphSONMode graphSONMode = GraphSONMode.Compact)
return GraphExtensions.CreateGremlinQuery<object>((DocumentClient)documentClient, collection, gremlinExpression, feedOptions, graphSONMode);
It is a pre-release however, so I do think that Microsoft will get around moving the extension methods at the interface level.
answered Nov 15 '18 at 15:12


Nick ChapsasNick Chapsas
3,1511516
3,1511516
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
|
show 1 more comment
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
Thanks Nick. But I think if I go with extension method approach then it would be really hard to create mock for unit test. What do you.think?
– Ask
Nov 15 '18 at 17:01
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
@Ask It would, but it is hard enough to do it with concrete class implementation anyway, and the IDocumentQuery is not easy to unit test to begin with. The current version of the SDK is really hard to unit test so I would go with this solution and focus my effort in integration testing.
– Nick Chapsas
Nov 15 '18 at 17:06
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
Thanks, do you have any good link where I can find unit test for IDocumentClient?
– Ask
Nov 15 '18 at 17:08
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
@Ask You can take a look one how I am unit testing my CosmosDB library, Cosmonaut. It covers most of the unit testable methods of IDocumentClient. You can find it here: github.com/Elfocrash/Cosmonaut/tree/develop/tests/… I also wrote a blog about it. You can find that here: chapsas.com/…
– Nick Chapsas
Nov 15 '18 at 17:10
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
can you tell me one more thing? I have changed my repo as you said but CreateGremlinQuery is an extension method. So is there way to create mock for CreateGremlinQuery?
– Ask
Nov 16 '18 at 5:53
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53320521%2fhow-to-convert-documentclient-to-idocumentclient-in-gremlin%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FcLBy9NIKFk uaFkN 73S7YRgbhZOnRai25dHd dHle1ctBJd