Convert SOAP XML string to object or just read its contents
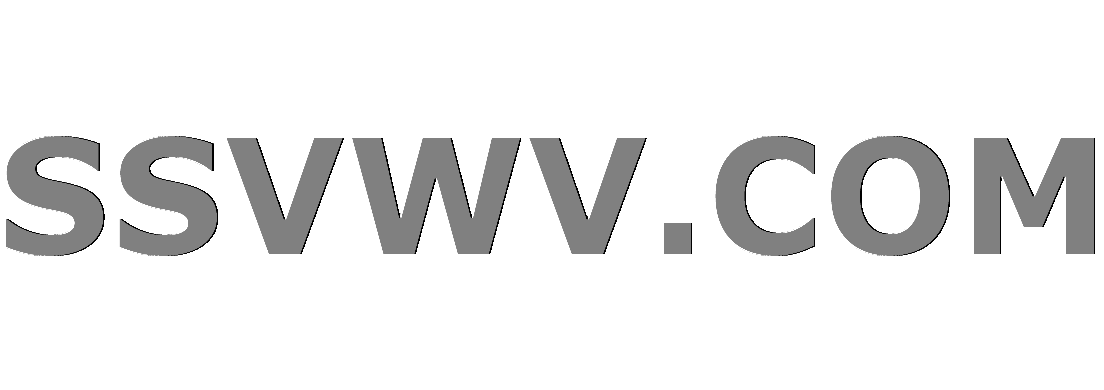
Multi tool use
Am receiving a SOAP XML response from an endpoint and I am trying to read its' contents. I have tried a couple of methods but it keeps giving me null
String xml = "<?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"><soap:Body><CashOutPaymentRequestResponse xmlns="http://tempuri.org/"><CashOutPaymentRequestResult><ResponseCode>1002</ResponseCode><ResponseDesc>FAC Code and Amount does not match, Contact your Admin.</ResponseDesc></CashOutPaymentRequestResult></CashOutPaymentRequestResponse></soap:Body></soap:Envelope>";
JAXBContext jaxbContext = JAXBContext.newInstance(cashOutPaymentRequestEnvelope.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
StringReader reader = new StringReader(xml);
cashOutPaymentRequestEnvelope person = (cashOutPaymentRequestEnvelope) unmarshaller.unmarshal(reader);
My method 2
Element rootdecryptedXml = XmlParseHelper.getStringXml(xml);
String responseUnEscapedXml = StringEscapeUtils.unescapeXml(XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml));
String responseEscapedXml = XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml);
System.out.println("step 1.. n" + responseEscapedXml);
Element root = XmlParseHelper.getStringXml(responseEscapedXml);
System.out.println("step 2aa...n" + XmlParseHelper.getString("ResponseCode", root));
System.out.println("step 2bb...n" + XmlParseHelper.getString("ResponseDesc", root));
None of the methods seem to work, they all give me null
My getString function
public static String getString(String tagName, Element element)
if (element == null)
return " ";
NodeList list = element.getElementsByTagName(tagName);
if (list != null && list.getLength() > 0)
NodeList subList = list.item(0).getChildNodes();
if (subList != null && subList.getLength() > 0)
return subList.item(0).getNodeValue();
return " ";
My get StringXml
public static Element getStringXml(String responseXml) throws SAXException, IOException, ParserConfigurationException
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
Document document;
Element rootdecryptedXml = null;
DocumentBuilder builder;
builder = factory.newDocumentBuilder();
document = builder.parse(new InputSource(new StringReader(responseXml)));
rootdecryptedXml = document.getDocumentElement();
return rootdecryptedXml;
java xml soap
add a comment |
Am receiving a SOAP XML response from an endpoint and I am trying to read its' contents. I have tried a couple of methods but it keeps giving me null
String xml = "<?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"><soap:Body><CashOutPaymentRequestResponse xmlns="http://tempuri.org/"><CashOutPaymentRequestResult><ResponseCode>1002</ResponseCode><ResponseDesc>FAC Code and Amount does not match, Contact your Admin.</ResponseDesc></CashOutPaymentRequestResult></CashOutPaymentRequestResponse></soap:Body></soap:Envelope>";
JAXBContext jaxbContext = JAXBContext.newInstance(cashOutPaymentRequestEnvelope.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
StringReader reader = new StringReader(xml);
cashOutPaymentRequestEnvelope person = (cashOutPaymentRequestEnvelope) unmarshaller.unmarshal(reader);
My method 2
Element rootdecryptedXml = XmlParseHelper.getStringXml(xml);
String responseUnEscapedXml = StringEscapeUtils.unescapeXml(XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml));
String responseEscapedXml = XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml);
System.out.println("step 1.. n" + responseEscapedXml);
Element root = XmlParseHelper.getStringXml(responseEscapedXml);
System.out.println("step 2aa...n" + XmlParseHelper.getString("ResponseCode", root));
System.out.println("step 2bb...n" + XmlParseHelper.getString("ResponseDesc", root));
None of the methods seem to work, they all give me null
My getString function
public static String getString(String tagName, Element element)
if (element == null)
return " ";
NodeList list = element.getElementsByTagName(tagName);
if (list != null && list.getLength() > 0)
NodeList subList = list.item(0).getChildNodes();
if (subList != null && subList.getLength() > 0)
return subList.item(0).getNodeValue();
return " ";
My get StringXml
public static Element getStringXml(String responseXml) throws SAXException, IOException, ParserConfigurationException
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
Document document;
Element rootdecryptedXml = null;
DocumentBuilder builder;
builder = factory.newDocumentBuilder();
document = builder.parse(new InputSource(new StringReader(responseXml)));
rootdecryptedXml = document.getDocumentElement();
return rootdecryptedXml;
java xml soap
1
Your first method using JAXB is sound - provided the JAXB Java class is the correct one for the given XML. You just need to feed the JAXB Unmarshaller just the relevant XML (the <CashOutPaymentRequestResult>...</CashOutPaymentRequestResult> part ) without the SOAP envelope. Use the SAAJ API to retrieve this and then unmarshall it. See docs.oracle.com/cd/E19644-01/817-5452/wsgjaxm.html for SAAJ.
– Michal
Nov 15 '18 at 16:04
Thanks @Michal was able to get it to work. Would post the implementation
– alex davies
Nov 15 '18 at 16:50
add a comment |
Am receiving a SOAP XML response from an endpoint and I am trying to read its' contents. I have tried a couple of methods but it keeps giving me null
String xml = "<?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"><soap:Body><CashOutPaymentRequestResponse xmlns="http://tempuri.org/"><CashOutPaymentRequestResult><ResponseCode>1002</ResponseCode><ResponseDesc>FAC Code and Amount does not match, Contact your Admin.</ResponseDesc></CashOutPaymentRequestResult></CashOutPaymentRequestResponse></soap:Body></soap:Envelope>";
JAXBContext jaxbContext = JAXBContext.newInstance(cashOutPaymentRequestEnvelope.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
StringReader reader = new StringReader(xml);
cashOutPaymentRequestEnvelope person = (cashOutPaymentRequestEnvelope) unmarshaller.unmarshal(reader);
My method 2
Element rootdecryptedXml = XmlParseHelper.getStringXml(xml);
String responseUnEscapedXml = StringEscapeUtils.unescapeXml(XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml));
String responseEscapedXml = XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml);
System.out.println("step 1.. n" + responseEscapedXml);
Element root = XmlParseHelper.getStringXml(responseEscapedXml);
System.out.println("step 2aa...n" + XmlParseHelper.getString("ResponseCode", root));
System.out.println("step 2bb...n" + XmlParseHelper.getString("ResponseDesc", root));
None of the methods seem to work, they all give me null
My getString function
public static String getString(String tagName, Element element)
if (element == null)
return " ";
NodeList list = element.getElementsByTagName(tagName);
if (list != null && list.getLength() > 0)
NodeList subList = list.item(0).getChildNodes();
if (subList != null && subList.getLength() > 0)
return subList.item(0).getNodeValue();
return " ";
My get StringXml
public static Element getStringXml(String responseXml) throws SAXException, IOException, ParserConfigurationException
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
Document document;
Element rootdecryptedXml = null;
DocumentBuilder builder;
builder = factory.newDocumentBuilder();
document = builder.parse(new InputSource(new StringReader(responseXml)));
rootdecryptedXml = document.getDocumentElement();
return rootdecryptedXml;
java xml soap
Am receiving a SOAP XML response from an endpoint and I am trying to read its' contents. I have tried a couple of methods but it keeps giving me null
String xml = "<?xml version="1.0" encoding="utf-8"?><soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"><soap:Body><CashOutPaymentRequestResponse xmlns="http://tempuri.org/"><CashOutPaymentRequestResult><ResponseCode>1002</ResponseCode><ResponseDesc>FAC Code and Amount does not match, Contact your Admin.</ResponseDesc></CashOutPaymentRequestResult></CashOutPaymentRequestResponse></soap:Body></soap:Envelope>";
JAXBContext jaxbContext = JAXBContext.newInstance(cashOutPaymentRequestEnvelope.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
StringReader reader = new StringReader(xml);
cashOutPaymentRequestEnvelope person = (cashOutPaymentRequestEnvelope) unmarshaller.unmarshal(reader);
My method 2
Element rootdecryptedXml = XmlParseHelper.getStringXml(xml);
String responseUnEscapedXml = StringEscapeUtils.unescapeXml(XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml));
String responseEscapedXml = XmlParseHelper.getString("CashOutPaymentRequestResult", rootdecryptedXml);
System.out.println("step 1.. n" + responseEscapedXml);
Element root = XmlParseHelper.getStringXml(responseEscapedXml);
System.out.println("step 2aa...n" + XmlParseHelper.getString("ResponseCode", root));
System.out.println("step 2bb...n" + XmlParseHelper.getString("ResponseDesc", root));
None of the methods seem to work, they all give me null
My getString function
public static String getString(String tagName, Element element)
if (element == null)
return " ";
NodeList list = element.getElementsByTagName(tagName);
if (list != null && list.getLength() > 0)
NodeList subList = list.item(0).getChildNodes();
if (subList != null && subList.getLength() > 0)
return subList.item(0).getNodeValue();
return " ";
My get StringXml
public static Element getStringXml(String responseXml) throws SAXException, IOException, ParserConfigurationException
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
Document document;
Element rootdecryptedXml = null;
DocumentBuilder builder;
builder = factory.newDocumentBuilder();
document = builder.parse(new InputSource(new StringReader(responseXml)));
rootdecryptedXml = document.getDocumentElement();
return rootdecryptedXml;
java xml soap
java xml soap
asked Nov 15 '18 at 10:16


alex daviesalex davies
11210
11210
1
Your first method using JAXB is sound - provided the JAXB Java class is the correct one for the given XML. You just need to feed the JAXB Unmarshaller just the relevant XML (the <CashOutPaymentRequestResult>...</CashOutPaymentRequestResult> part ) without the SOAP envelope. Use the SAAJ API to retrieve this and then unmarshall it. See docs.oracle.com/cd/E19644-01/817-5452/wsgjaxm.html for SAAJ.
– Michal
Nov 15 '18 at 16:04
Thanks @Michal was able to get it to work. Would post the implementation
– alex davies
Nov 15 '18 at 16:50
add a comment |
1
Your first method using JAXB is sound - provided the JAXB Java class is the correct one for the given XML. You just need to feed the JAXB Unmarshaller just the relevant XML (the <CashOutPaymentRequestResult>...</CashOutPaymentRequestResult> part ) without the SOAP envelope. Use the SAAJ API to retrieve this and then unmarshall it. See docs.oracle.com/cd/E19644-01/817-5452/wsgjaxm.html for SAAJ.
– Michal
Nov 15 '18 at 16:04
Thanks @Michal was able to get it to work. Would post the implementation
– alex davies
Nov 15 '18 at 16:50
1
1
Your first method using JAXB is sound - provided the JAXB Java class is the correct one for the given XML. You just need to feed the JAXB Unmarshaller just the relevant XML (the <CashOutPaymentRequestResult>...</CashOutPaymentRequestResult> part ) without the SOAP envelope. Use the SAAJ API to retrieve this and then unmarshall it. See docs.oracle.com/cd/E19644-01/817-5452/wsgjaxm.html for SAAJ.
– Michal
Nov 15 '18 at 16:04
Your first method using JAXB is sound - provided the JAXB Java class is the correct one for the given XML. You just need to feed the JAXB Unmarshaller just the relevant XML (the <CashOutPaymentRequestResult>...</CashOutPaymentRequestResult> part ) without the SOAP envelope. Use the SAAJ API to retrieve this and then unmarshall it. See docs.oracle.com/cd/E19644-01/817-5452/wsgjaxm.html for SAAJ.
– Michal
Nov 15 '18 at 16:04
Thanks @Michal was able to get it to work. Would post the implementation
– alex davies
Nov 15 '18 at 16:50
Thanks @Michal was able to get it to work. Would post the implementation
– alex davies
Nov 15 '18 at 16:50
add a comment |
1 Answer
1
active
oldest
votes
Thanks to Michal's comment I developed a solution to this
InputStream is = new ByteArrayInputStream(xml.getBytes());
MessageFactory factory = MessageFactory.newInstance();
SOAPMessage message = factory.createMessage(null, is);
NodeList listResult = message.getSOAPBody().getElementsByTagName("CashOutPaymentRequestResult");
for (int i = 0; i < listResult.getLength(); i++)
NodeList children = listResult.item(i).getChildNodes();
for (int k = 0; k < children.getLength(); k++)
System.out.println(children.item(k).getNodeName());
System.out.println(children.item(k).getTextContent());
if (children.item(k).getNodeName().equals("ResponseCode"))
if (children.item(k).getNodeName().equals("ResponseDesc"))
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317124%2fconvert-soap-xml-string-to-object-or-just-read-its-contents%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks to Michal's comment I developed a solution to this
InputStream is = new ByteArrayInputStream(xml.getBytes());
MessageFactory factory = MessageFactory.newInstance();
SOAPMessage message = factory.createMessage(null, is);
NodeList listResult = message.getSOAPBody().getElementsByTagName("CashOutPaymentRequestResult");
for (int i = 0; i < listResult.getLength(); i++)
NodeList children = listResult.item(i).getChildNodes();
for (int k = 0; k < children.getLength(); k++)
System.out.println(children.item(k).getNodeName());
System.out.println(children.item(k).getTextContent());
if (children.item(k).getNodeName().equals("ResponseCode"))
if (children.item(k).getNodeName().equals("ResponseDesc"))
add a comment |
Thanks to Michal's comment I developed a solution to this
InputStream is = new ByteArrayInputStream(xml.getBytes());
MessageFactory factory = MessageFactory.newInstance();
SOAPMessage message = factory.createMessage(null, is);
NodeList listResult = message.getSOAPBody().getElementsByTagName("CashOutPaymentRequestResult");
for (int i = 0; i < listResult.getLength(); i++)
NodeList children = listResult.item(i).getChildNodes();
for (int k = 0; k < children.getLength(); k++)
System.out.println(children.item(k).getNodeName());
System.out.println(children.item(k).getTextContent());
if (children.item(k).getNodeName().equals("ResponseCode"))
if (children.item(k).getNodeName().equals("ResponseDesc"))
add a comment |
Thanks to Michal's comment I developed a solution to this
InputStream is = new ByteArrayInputStream(xml.getBytes());
MessageFactory factory = MessageFactory.newInstance();
SOAPMessage message = factory.createMessage(null, is);
NodeList listResult = message.getSOAPBody().getElementsByTagName("CashOutPaymentRequestResult");
for (int i = 0; i < listResult.getLength(); i++)
NodeList children = listResult.item(i).getChildNodes();
for (int k = 0; k < children.getLength(); k++)
System.out.println(children.item(k).getNodeName());
System.out.println(children.item(k).getTextContent());
if (children.item(k).getNodeName().equals("ResponseCode"))
if (children.item(k).getNodeName().equals("ResponseDesc"))
Thanks to Michal's comment I developed a solution to this
InputStream is = new ByteArrayInputStream(xml.getBytes());
MessageFactory factory = MessageFactory.newInstance();
SOAPMessage message = factory.createMessage(null, is);
NodeList listResult = message.getSOAPBody().getElementsByTagName("CashOutPaymentRequestResult");
for (int i = 0; i < listResult.getLength(); i++)
NodeList children = listResult.item(i).getChildNodes();
for (int k = 0; k < children.getLength(); k++)
System.out.println(children.item(k).getNodeName());
System.out.println(children.item(k).getTextContent());
if (children.item(k).getNodeName().equals("ResponseCode"))
if (children.item(k).getNodeName().equals("ResponseDesc"))
answered Nov 15 '18 at 17:09


alex daviesalex davies
11210
11210
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317124%2fconvert-soap-xml-string-to-object-or-just-read-its-contents%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
o2,ujjbVWYrAHT3DCRarBZipXG2xNeApJPjxnNK7 DDcf yN1Ty h6Xns33HN DT5eE0Q9p6YkU5LkHi RXrg RPCMyeHCB Garh1pBnCP
1
Your first method using JAXB is sound - provided the JAXB Java class is the correct one for the given XML. You just need to feed the JAXB Unmarshaller just the relevant XML (the <CashOutPaymentRequestResult>...</CashOutPaymentRequestResult> part ) without the SOAP envelope. Use the SAAJ API to retrieve this and then unmarshall it. See docs.oracle.com/cd/E19644-01/817-5452/wsgjaxm.html for SAAJ.
– Michal
Nov 15 '18 at 16:04
Thanks @Michal was able to get it to work. Would post the implementation
– alex davies
Nov 15 '18 at 16:50