String extraction and http request creation
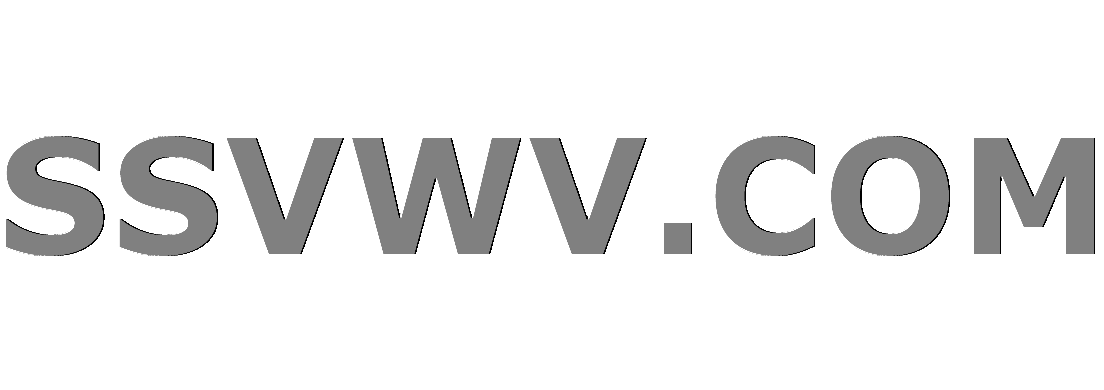
Multi tool use
I'm trying to extract a specific string (URL address) from each JSON result (in the tweets.txt file) and then create HTTP GET request with the extracted URL address, the HTTP response should be saved as a new HTML file in the directory.
The string I'm trying to extract is the value of specific JSON value.
for example:
"display_url": test.com/y8HTdfdfKMFz
my code:
# # # # TWITTER STREAM LISTENER # # # #
class StdOutListener(StreamListener):
"""
This is a basic listener that just prints received tweets to stdout.
"""
def __init__(self, fetched_tweets_filename):
self.fetched_tweets_filename = fetched_tweets_filename
def on_data(self, data):
try:
print(data)
with open(self.fetched_tweets_filename, 'a') as tf:
tf.write(data)
return True
except BaseException as e:
print("Error on_data %s" % str(e))
return True
def on_error(self, status):
print(status)
if __name__ == '__main__':
# Authenticate using config.py and connect to Twitter Streaming API.
hash_tag_list = ["donal trump"]
fetched_tweets_filename = "tweets.txt"
twitter_streamer = TwitterStreamer()
twitter_streamer.stream_tweets(fetched_tweets_filename, hash_tag_list)
The JSON result:
"created_at":"Wed Nov 14 11:12:59 +0000 2018","id":1062664687601496064,"id_str":"1062664687601496064","text":"This is test https://t.co/V3tNm99tdn fdfdnn#osectraining","source":"u003ca href="http://twitter.com" rel="nofollow"u003eTwitter Web Clientu003c/au003e","truncated":false,"in_reply_to_status_id":null,"in_reply_to_status_id_str":null,"in_reply_to_user_id":null,"in_reply_to_user_id_str":null,"in_reply_to_screen_name":null,"user":"id":961508561217052675,"id_str":"961508561217052675","name":"line Sec","screen_name":"oseining","location":"US","url":"https://www.ocurity.com","description":"field","translator_type":"none","protected":false,"verified":false,"followers_count":2,"friends_count":51,"listed_count":0,"favourites_count":0,"statuses_count":3,"created_at":"Thu Feb 08 07:54:39 +0000 2018","utc_offset":null,"time_zone":null,"geo_enabled":false,"lang":"en","contributors_enabled":false,"is_translator":false,"profile_background_color":"000000","profile_background_image_url":"http://abs.twimg.com/images/themes/theme1/bg.png","profile_background_image_url_https":"https://abs.twimg.com/images/themes/theme1/bg.png","profile_background_tile":false,"profile_link_color":"1B95E0","profile_sidebar_border_color":"000000","profile_sidebar_fill_color":"000000","profile_text_color":"000000","profile_use_background_image":false,"profile_image_url":"http://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_image_url_https":"https://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_banner_url":"https://pbs.twimg.com/profile_banners/961508561217052675/1518076913","default_profile":false,"default_profile_image":false,"following":null,"follow_request_sent":null,"notifications":null,"geo":null,"coordinates":null,"place":null,"contributors":null,"is_quote_status":false,"quote_count":0,"reply_count":0,"retweet_count":0,"favorite_count":0,"entities":"hashtags":["text":"osectraining","indices":[44,57]],"urls":["url":"https://t.co/V3tNm99tdn","expanded_url":"https://pastebin.com/y8HTKMFz","display_url":"pastebin.com/y8HTKMFz","indices":[13,36]],"user_mentions":,"symbols":,"favorited":false,"retweeted":false,"possibly_sensitive":false,"filter_level":"low","lang":"tr","timestamp_ms":"1542193979536"enter code here
python
add a comment |
I'm trying to extract a specific string (URL address) from each JSON result (in the tweets.txt file) and then create HTTP GET request with the extracted URL address, the HTTP response should be saved as a new HTML file in the directory.
The string I'm trying to extract is the value of specific JSON value.
for example:
"display_url": test.com/y8HTdfdfKMFz
my code:
# # # # TWITTER STREAM LISTENER # # # #
class StdOutListener(StreamListener):
"""
This is a basic listener that just prints received tweets to stdout.
"""
def __init__(self, fetched_tweets_filename):
self.fetched_tweets_filename = fetched_tweets_filename
def on_data(self, data):
try:
print(data)
with open(self.fetched_tweets_filename, 'a') as tf:
tf.write(data)
return True
except BaseException as e:
print("Error on_data %s" % str(e))
return True
def on_error(self, status):
print(status)
if __name__ == '__main__':
# Authenticate using config.py and connect to Twitter Streaming API.
hash_tag_list = ["donal trump"]
fetched_tweets_filename = "tweets.txt"
twitter_streamer = TwitterStreamer()
twitter_streamer.stream_tweets(fetched_tweets_filename, hash_tag_list)
The JSON result:
"created_at":"Wed Nov 14 11:12:59 +0000 2018","id":1062664687601496064,"id_str":"1062664687601496064","text":"This is test https://t.co/V3tNm99tdn fdfdnn#osectraining","source":"u003ca href="http://twitter.com" rel="nofollow"u003eTwitter Web Clientu003c/au003e","truncated":false,"in_reply_to_status_id":null,"in_reply_to_status_id_str":null,"in_reply_to_user_id":null,"in_reply_to_user_id_str":null,"in_reply_to_screen_name":null,"user":"id":961508561217052675,"id_str":"961508561217052675","name":"line Sec","screen_name":"oseining","location":"US","url":"https://www.ocurity.com","description":"field","translator_type":"none","protected":false,"verified":false,"followers_count":2,"friends_count":51,"listed_count":0,"favourites_count":0,"statuses_count":3,"created_at":"Thu Feb 08 07:54:39 +0000 2018","utc_offset":null,"time_zone":null,"geo_enabled":false,"lang":"en","contributors_enabled":false,"is_translator":false,"profile_background_color":"000000","profile_background_image_url":"http://abs.twimg.com/images/themes/theme1/bg.png","profile_background_image_url_https":"https://abs.twimg.com/images/themes/theme1/bg.png","profile_background_tile":false,"profile_link_color":"1B95E0","profile_sidebar_border_color":"000000","profile_sidebar_fill_color":"000000","profile_text_color":"000000","profile_use_background_image":false,"profile_image_url":"http://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_image_url_https":"https://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_banner_url":"https://pbs.twimg.com/profile_banners/961508561217052675/1518076913","default_profile":false,"default_profile_image":false,"following":null,"follow_request_sent":null,"notifications":null,"geo":null,"coordinates":null,"place":null,"contributors":null,"is_quote_status":false,"quote_count":0,"reply_count":0,"retweet_count":0,"favorite_count":0,"entities":"hashtags":["text":"osectraining","indices":[44,57]],"urls":["url":"https://t.co/V3tNm99tdn","expanded_url":"https://pastebin.com/y8HTKMFz","display_url":"pastebin.com/y8HTKMFz","indices":[13,36]],"user_mentions":,"symbols":,"favorited":false,"retweeted":false,"possibly_sensitive":false,"filter_level":"low","lang":"tr","timestamp_ms":"1542193979536"enter code here
python
add a comment |
I'm trying to extract a specific string (URL address) from each JSON result (in the tweets.txt file) and then create HTTP GET request with the extracted URL address, the HTTP response should be saved as a new HTML file in the directory.
The string I'm trying to extract is the value of specific JSON value.
for example:
"display_url": test.com/y8HTdfdfKMFz
my code:
# # # # TWITTER STREAM LISTENER # # # #
class StdOutListener(StreamListener):
"""
This is a basic listener that just prints received tweets to stdout.
"""
def __init__(self, fetched_tweets_filename):
self.fetched_tweets_filename = fetched_tweets_filename
def on_data(self, data):
try:
print(data)
with open(self.fetched_tweets_filename, 'a') as tf:
tf.write(data)
return True
except BaseException as e:
print("Error on_data %s" % str(e))
return True
def on_error(self, status):
print(status)
if __name__ == '__main__':
# Authenticate using config.py and connect to Twitter Streaming API.
hash_tag_list = ["donal trump"]
fetched_tweets_filename = "tweets.txt"
twitter_streamer = TwitterStreamer()
twitter_streamer.stream_tweets(fetched_tweets_filename, hash_tag_list)
The JSON result:
"created_at":"Wed Nov 14 11:12:59 +0000 2018","id":1062664687601496064,"id_str":"1062664687601496064","text":"This is test https://t.co/V3tNm99tdn fdfdnn#osectraining","source":"u003ca href="http://twitter.com" rel="nofollow"u003eTwitter Web Clientu003c/au003e","truncated":false,"in_reply_to_status_id":null,"in_reply_to_status_id_str":null,"in_reply_to_user_id":null,"in_reply_to_user_id_str":null,"in_reply_to_screen_name":null,"user":"id":961508561217052675,"id_str":"961508561217052675","name":"line Sec","screen_name":"oseining","location":"US","url":"https://www.ocurity.com","description":"field","translator_type":"none","protected":false,"verified":false,"followers_count":2,"friends_count":51,"listed_count":0,"favourites_count":0,"statuses_count":3,"created_at":"Thu Feb 08 07:54:39 +0000 2018","utc_offset":null,"time_zone":null,"geo_enabled":false,"lang":"en","contributors_enabled":false,"is_translator":false,"profile_background_color":"000000","profile_background_image_url":"http://abs.twimg.com/images/themes/theme1/bg.png","profile_background_image_url_https":"https://abs.twimg.com/images/themes/theme1/bg.png","profile_background_tile":false,"profile_link_color":"1B95E0","profile_sidebar_border_color":"000000","profile_sidebar_fill_color":"000000","profile_text_color":"000000","profile_use_background_image":false,"profile_image_url":"http://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_image_url_https":"https://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_banner_url":"https://pbs.twimg.com/profile_banners/961508561217052675/1518076913","default_profile":false,"default_profile_image":false,"following":null,"follow_request_sent":null,"notifications":null,"geo":null,"coordinates":null,"place":null,"contributors":null,"is_quote_status":false,"quote_count":0,"reply_count":0,"retweet_count":0,"favorite_count":0,"entities":"hashtags":["text":"osectraining","indices":[44,57]],"urls":["url":"https://t.co/V3tNm99tdn","expanded_url":"https://pastebin.com/y8HTKMFz","display_url":"pastebin.com/y8HTKMFz","indices":[13,36]],"user_mentions":,"symbols":,"favorited":false,"retweeted":false,"possibly_sensitive":false,"filter_level":"low","lang":"tr","timestamp_ms":"1542193979536"enter code here
python
I'm trying to extract a specific string (URL address) from each JSON result (in the tweets.txt file) and then create HTTP GET request with the extracted URL address, the HTTP response should be saved as a new HTML file in the directory.
The string I'm trying to extract is the value of specific JSON value.
for example:
"display_url": test.com/y8HTdfdfKMFz
my code:
# # # # TWITTER STREAM LISTENER # # # #
class StdOutListener(StreamListener):
"""
This is a basic listener that just prints received tweets to stdout.
"""
def __init__(self, fetched_tweets_filename):
self.fetched_tweets_filename = fetched_tweets_filename
def on_data(self, data):
try:
print(data)
with open(self.fetched_tweets_filename, 'a') as tf:
tf.write(data)
return True
except BaseException as e:
print("Error on_data %s" % str(e))
return True
def on_error(self, status):
print(status)
if __name__ == '__main__':
# Authenticate using config.py and connect to Twitter Streaming API.
hash_tag_list = ["donal trump"]
fetched_tweets_filename = "tweets.txt"
twitter_streamer = TwitterStreamer()
twitter_streamer.stream_tweets(fetched_tweets_filename, hash_tag_list)
The JSON result:
"created_at":"Wed Nov 14 11:12:59 +0000 2018","id":1062664687601496064,"id_str":"1062664687601496064","text":"This is test https://t.co/V3tNm99tdn fdfdnn#osectraining","source":"u003ca href="http://twitter.com" rel="nofollow"u003eTwitter Web Clientu003c/au003e","truncated":false,"in_reply_to_status_id":null,"in_reply_to_status_id_str":null,"in_reply_to_user_id":null,"in_reply_to_user_id_str":null,"in_reply_to_screen_name":null,"user":"id":961508561217052675,"id_str":"961508561217052675","name":"line Sec","screen_name":"oseining","location":"US","url":"https://www.ocurity.com","description":"field","translator_type":"none","protected":false,"verified":false,"followers_count":2,"friends_count":51,"listed_count":0,"favourites_count":0,"statuses_count":3,"created_at":"Thu Feb 08 07:54:39 +0000 2018","utc_offset":null,"time_zone":null,"geo_enabled":false,"lang":"en","contributors_enabled":false,"is_translator":false,"profile_background_color":"000000","profile_background_image_url":"http://abs.twimg.com/images/themes/theme1/bg.png","profile_background_image_url_https":"https://abs.twimg.com/images/themes/theme1/bg.png","profile_background_tile":false,"profile_link_color":"1B95E0","profile_sidebar_border_color":"000000","profile_sidebar_fill_color":"000000","profile_text_color":"000000","profile_use_background_image":false,"profile_image_url":"http://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_image_url_https":"https://pbs.twimg.com/profile_images/961510231346958336/d_KhBeTD_normal.jpg","profile_banner_url":"https://pbs.twimg.com/profile_banners/961508561217052675/1518076913","default_profile":false,"default_profile_image":false,"following":null,"follow_request_sent":null,"notifications":null,"geo":null,"coordinates":null,"place":null,"contributors":null,"is_quote_status":false,"quote_count":0,"reply_count":0,"retweet_count":0,"favorite_count":0,"entities":"hashtags":["text":"osectraining","indices":[44,57]],"urls":["url":"https://t.co/V3tNm99tdn","expanded_url":"https://pastebin.com/y8HTKMFz","display_url":"pastebin.com/y8HTKMFz","indices":[13,36]],"user_mentions":,"symbols":,"favorited":false,"retweeted":false,"possibly_sensitive":false,"filter_level":"low","lang":"tr","timestamp_ms":"1542193979536"enter code here
python
python
edited Nov 15 '18 at 11:30
bugnet17
asked Nov 15 '18 at 10:20
bugnet17bugnet17
687
687
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
I'm not sure I understood you 100% but, if you save the tweets to a file, as a json dump you can easily convert the json result to a python dict as follows:
import json
with open('tweets.txt') as handle:
dictdump = json.loads(handle.read())
and then you have just a python dict value:
my_url = dictdump['display_url']
and then use requests
module to send a get/post whatever you want to the url
import requests
r = requests.get(my_url)
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
add a comment |
This is building on top of @Avishay Cohen's answer, since you are opening the file in append mode, there should be more than one json string in your tweets.txt
So what you could do is something like this:
import json
import requests
with open('tweets.txt') as input_file:
for line in input_file:
tweet_json = json.loads(line)
response = requests.get(tweet_json.get('display_url')) if 'display_url' in tweet_json else
if response and response.status_code()==200:
print(response.text)
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317207%2fstring-extraction-and-http-request-creation%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm not sure I understood you 100% but, if you save the tweets to a file, as a json dump you can easily convert the json result to a python dict as follows:
import json
with open('tweets.txt') as handle:
dictdump = json.loads(handle.read())
and then you have just a python dict value:
my_url = dictdump['display_url']
and then use requests
module to send a get/post whatever you want to the url
import requests
r = requests.get(my_url)
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
add a comment |
I'm not sure I understood you 100% but, if you save the tweets to a file, as a json dump you can easily convert the json result to a python dict as follows:
import json
with open('tweets.txt') as handle:
dictdump = json.loads(handle.read())
and then you have just a python dict value:
my_url = dictdump['display_url']
and then use requests
module to send a get/post whatever you want to the url
import requests
r = requests.get(my_url)
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
add a comment |
I'm not sure I understood you 100% but, if you save the tweets to a file, as a json dump you can easily convert the json result to a python dict as follows:
import json
with open('tweets.txt') as handle:
dictdump = json.loads(handle.read())
and then you have just a python dict value:
my_url = dictdump['display_url']
and then use requests
module to send a get/post whatever you want to the url
import requests
r = requests.get(my_url)
I'm not sure I understood you 100% but, if you save the tweets to a file, as a json dump you can easily convert the json result to a python dict as follows:
import json
with open('tweets.txt') as handle:
dictdump = json.loads(handle.read())
and then you have just a python dict value:
my_url = dictdump['display_url']
and then use requests
module to send a get/post whatever you want to the url
import requests
r = requests.get(my_url)
answered Nov 15 '18 at 10:52
Avishay CohenAvishay Cohen
750825
750825
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
add a comment |
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
error : Traceback (most recent call last): File "request2.py", line 3, in <module> dictdump = json.loads(handle.read()) File "/usr/lib/python2.7/json/__init__.py", line 339, in loads return _default_decoder.decode(s) File "/usr/lib/python2.7/json/decoder.py", line 367, in decode raise ValueError(errmsg("Extra data", s, end, len(s))) ValueError: Extra data: line 2 column 1 - line 81 column 1 (char 5379 - 470853)
– bugnet17
Nov 15 '18 at 11:52
add a comment |
This is building on top of @Avishay Cohen's answer, since you are opening the file in append mode, there should be more than one json string in your tweets.txt
So what you could do is something like this:
import json
import requests
with open('tweets.txt') as input_file:
for line in input_file:
tweet_json = json.loads(line)
response = requests.get(tweet_json.get('display_url')) if 'display_url' in tweet_json else
if response and response.status_code()==200:
print(response.text)
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
add a comment |
This is building on top of @Avishay Cohen's answer, since you are opening the file in append mode, there should be more than one json string in your tweets.txt
So what you could do is something like this:
import json
import requests
with open('tweets.txt') as input_file:
for line in input_file:
tweet_json = json.loads(line)
response = requests.get(tweet_json.get('display_url')) if 'display_url' in tweet_json else
if response and response.status_code()==200:
print(response.text)
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
add a comment |
This is building on top of @Avishay Cohen's answer, since you are opening the file in append mode, there should be more than one json string in your tweets.txt
So what you could do is something like this:
import json
import requests
with open('tweets.txt') as input_file:
for line in input_file:
tweet_json = json.loads(line)
response = requests.get(tweet_json.get('display_url')) if 'display_url' in tweet_json else
if response and response.status_code()==200:
print(response.text)
This is building on top of @Avishay Cohen's answer, since you are opening the file in append mode, there should be more than one json string in your tweets.txt
So what you could do is something like this:
import json
import requests
with open('tweets.txt') as input_file:
for line in input_file:
tweet_json = json.loads(line)
response = requests.get(tweet_json.get('display_url')) if 'display_url' in tweet_json else
if response and response.status_code()==200:
print(response.text)
edited Nov 15 '18 at 11:23
answered Nov 15 '18 at 11:06
kumarDkumarD
162317
162317
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
add a comment |
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
There is some problem. response.txt doesn't create. When I add "print response" for debugging I got this output:
– bugnet17
Nov 15 '18 at 11:28
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
The json which you have attached doesn't contain any key called 'display_url', can you add the name of the attribute which you are referring as url?
– kumarD
Nov 15 '18 at 11:31
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
"display_url":"test.com"
– bugnet17
Nov 15 '18 at 11:36
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
well this is not present in the outside part of the dictionary itself, its embedded within the entities list, are you sure that there will only be one item in the 'entities' list ?
– kumarD
Nov 15 '18 at 12:04
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
Take a look in the JSON result in the question. there is a value: "display_url":"pastebin.com/y8HTKMFz". I need to extract the URL part, "pastebin.com/y8HTKMFz" in this case.
– bugnet17
Nov 15 '18 at 16:35
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317207%2fstring-extraction-and-http-request-creation%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WWNa4RGkk hbpQWuMZDbzCox Jo Ppr47ez1 PY7 yDhJKLnHQDjcekYMAK6NOEWUV 6irMFlvJ4B T,8hF2dQGdvi6DBb qa4bFiFtbri