Conway's soldiers - C
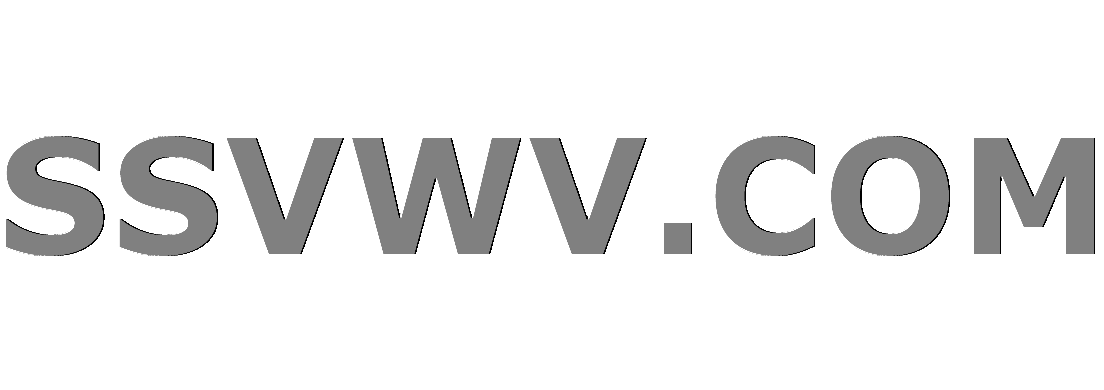
Multi tool use
I am doing an assignment which involves building a C code for Conway's Soldiers. Like this but smaller board https://www.cleverlearning.co.uk/blogs/blogConway.php
The bottom half of the board is filled with pegs or 'X' and the upper part empty or '.'. Pegs can move up, left or right. Would it be correct to check if the pegs can move by calling the below functions:
int can_move_up(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i][j-2] == PEG)
return true;
int can_move_left(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i-2][j] == PEG)
return true;
int can_move_right(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i+2][j] == PEG)
return true;
Any help is much appreciated!
c function
|
show 1 more comment
I am doing an assignment which involves building a C code for Conway's Soldiers. Like this but smaller board https://www.cleverlearning.co.uk/blogs/blogConway.php
The bottom half of the board is filled with pegs or 'X' and the upper part empty or '.'. Pegs can move up, left or right. Would it be correct to check if the pegs can move by calling the below functions:
int can_move_up(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i][j-2] == PEG)
return true;
int can_move_left(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i-2][j] == PEG)
return true;
int can_move_right(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i+2][j] == PEG)
return true;
Any help is much appreciated!
c function
1
Welcome to stackoverflow.com. Please take some time to read the help pages, especially the sections named "What topics can I ask about here?" and "What types of questions should I avoid asking?". Also please take the tour and read about how to ask good questions. Lastly please read this question checklist.
– Some programmer dude
Nov 15 '18 at 10:15
1
You should use pointers to your array instead of copying them to the function.
– A.R.C.
Nov 15 '18 at 10:17
1
What are your functionscan_move_left
etc doing?? They go through the whole board. Shouldn't they be called with a peg position and check if the peg can move?
– Paul Ogilvie
Nov 15 '18 at 10:22
1
@A.R.C. (and whoever gave this an upvote) You should use pointers to your array instead of copying them to the function. Huh? Arrays are never copied to functions. Regardless, whether writingint board[ROWS][COLUMNS]
orint (*board)[COLUMS]
, the C compiler will always do the same - decay arrays in function parameters to pointers.
– Scheff
Nov 15 '18 at 10:35
@A.R.C. If in doubt then compare the outcome ofint square(int board[N_ROWS][N_COLS])
andint square(int (*board)[N_COLS])
- identical upto the least bit. ;-)
– Scheff
Nov 15 '18 at 10:44
|
show 1 more comment
I am doing an assignment which involves building a C code for Conway's Soldiers. Like this but smaller board https://www.cleverlearning.co.uk/blogs/blogConway.php
The bottom half of the board is filled with pegs or 'X' and the upper part empty or '.'. Pegs can move up, left or right. Would it be correct to check if the pegs can move by calling the below functions:
int can_move_up(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i][j-2] == PEG)
return true;
int can_move_left(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i-2][j] == PEG)
return true;
int can_move_right(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i+2][j] == PEG)
return true;
Any help is much appreciated!
c function
I am doing an assignment which involves building a C code for Conway's Soldiers. Like this but smaller board https://www.cleverlearning.co.uk/blogs/blogConway.php
The bottom half of the board is filled with pegs or 'X' and the upper part empty or '.'. Pegs can move up, left or right. Would it be correct to check if the pegs can move by calling the below functions:
int can_move_up(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i][j-2] == PEG)
return true;
int can_move_left(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i-2][j] == PEG)
return true;
int can_move_right(int board[ROWS][COLUMNS])
int i, j;
for(i=0; i < ROWS; ++i)
for (j=0; j < COLUMNS; ++j)
if (board[i][j] != PEG)
return false;
else if (board[i][j] == PEG && board[i+2][j] == PEG)
return true;
Any help is much appreciated!
c function
c function
edited Nov 15 '18 at 10:19
barbsan
2,45051324
2,45051324
asked Nov 15 '18 at 10:14
C Dummy C Dummy
195
195
1
Welcome to stackoverflow.com. Please take some time to read the help pages, especially the sections named "What topics can I ask about here?" and "What types of questions should I avoid asking?". Also please take the tour and read about how to ask good questions. Lastly please read this question checklist.
– Some programmer dude
Nov 15 '18 at 10:15
1
You should use pointers to your array instead of copying them to the function.
– A.R.C.
Nov 15 '18 at 10:17
1
What are your functionscan_move_left
etc doing?? They go through the whole board. Shouldn't they be called with a peg position and check if the peg can move?
– Paul Ogilvie
Nov 15 '18 at 10:22
1
@A.R.C. (and whoever gave this an upvote) You should use pointers to your array instead of copying them to the function. Huh? Arrays are never copied to functions. Regardless, whether writingint board[ROWS][COLUMNS]
orint (*board)[COLUMS]
, the C compiler will always do the same - decay arrays in function parameters to pointers.
– Scheff
Nov 15 '18 at 10:35
@A.R.C. If in doubt then compare the outcome ofint square(int board[N_ROWS][N_COLS])
andint square(int (*board)[N_COLS])
- identical upto the least bit. ;-)
– Scheff
Nov 15 '18 at 10:44
|
show 1 more comment
1
Welcome to stackoverflow.com. Please take some time to read the help pages, especially the sections named "What topics can I ask about here?" and "What types of questions should I avoid asking?". Also please take the tour and read about how to ask good questions. Lastly please read this question checklist.
– Some programmer dude
Nov 15 '18 at 10:15
1
You should use pointers to your array instead of copying them to the function.
– A.R.C.
Nov 15 '18 at 10:17
1
What are your functionscan_move_left
etc doing?? They go through the whole board. Shouldn't they be called with a peg position and check if the peg can move?
– Paul Ogilvie
Nov 15 '18 at 10:22
1
@A.R.C. (and whoever gave this an upvote) You should use pointers to your array instead of copying them to the function. Huh? Arrays are never copied to functions. Regardless, whether writingint board[ROWS][COLUMNS]
orint (*board)[COLUMS]
, the C compiler will always do the same - decay arrays in function parameters to pointers.
– Scheff
Nov 15 '18 at 10:35
@A.R.C. If in doubt then compare the outcome ofint square(int board[N_ROWS][N_COLS])
andint square(int (*board)[N_COLS])
- identical upto the least bit. ;-)
– Scheff
Nov 15 '18 at 10:44
1
1
Welcome to stackoverflow.com. Please take some time to read the help pages, especially the sections named "What topics can I ask about here?" and "What types of questions should I avoid asking?". Also please take the tour and read about how to ask good questions. Lastly please read this question checklist.
– Some programmer dude
Nov 15 '18 at 10:15
Welcome to stackoverflow.com. Please take some time to read the help pages, especially the sections named "What topics can I ask about here?" and "What types of questions should I avoid asking?". Also please take the tour and read about how to ask good questions. Lastly please read this question checklist.
– Some programmer dude
Nov 15 '18 at 10:15
1
1
You should use pointers to your array instead of copying them to the function.
– A.R.C.
Nov 15 '18 at 10:17
You should use pointers to your array instead of copying them to the function.
– A.R.C.
Nov 15 '18 at 10:17
1
1
What are your functions
can_move_left
etc doing?? They go through the whole board. Shouldn't they be called with a peg position and check if the peg can move?– Paul Ogilvie
Nov 15 '18 at 10:22
What are your functions
can_move_left
etc doing?? They go through the whole board. Shouldn't they be called with a peg position and check if the peg can move?– Paul Ogilvie
Nov 15 '18 at 10:22
1
1
@A.R.C. (and whoever gave this an upvote) You should use pointers to your array instead of copying them to the function. Huh? Arrays are never copied to functions. Regardless, whether writing
int board[ROWS][COLUMNS]
or int (*board)[COLUMS]
, the C compiler will always do the same - decay arrays in function parameters to pointers.– Scheff
Nov 15 '18 at 10:35
@A.R.C. (and whoever gave this an upvote) You should use pointers to your array instead of copying them to the function. Huh? Arrays are never copied to functions. Regardless, whether writing
int board[ROWS][COLUMNS]
or int (*board)[COLUMS]
, the C compiler will always do the same - decay arrays in function parameters to pointers.– Scheff
Nov 15 '18 at 10:35
@A.R.C. If in doubt then compare the outcome of
int square(int board[N_ROWS][N_COLS])
and int square(int (*board)[N_COLS])
- identical upto the least bit. ;-)– Scheff
Nov 15 '18 at 10:44
@A.R.C. If in doubt then compare the outcome of
int square(int board[N_ROWS][N_COLS])
and int square(int (*board)[N_COLS])
- identical upto the least bit. ;-)– Scheff
Nov 15 '18 at 10:44
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317078%2fconways-soldiers-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317078%2fconways-soldiers-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9IfYtdOzJ AOTDtUjVakaOEnXomq zsyhcp up 8
1
Welcome to stackoverflow.com. Please take some time to read the help pages, especially the sections named "What topics can I ask about here?" and "What types of questions should I avoid asking?". Also please take the tour and read about how to ask good questions. Lastly please read this question checklist.
– Some programmer dude
Nov 15 '18 at 10:15
1
You should use pointers to your array instead of copying them to the function.
– A.R.C.
Nov 15 '18 at 10:17
1
What are your functions
can_move_left
etc doing?? They go through the whole board. Shouldn't they be called with a peg position and check if the peg can move?– Paul Ogilvie
Nov 15 '18 at 10:22
1
@A.R.C. (and whoever gave this an upvote) You should use pointers to your array instead of copying them to the function. Huh? Arrays are never copied to functions. Regardless, whether writing
int board[ROWS][COLUMNS]
orint (*board)[COLUMS]
, the C compiler will always do the same - decay arrays in function parameters to pointers.– Scheff
Nov 15 '18 at 10:35
@A.R.C. If in doubt then compare the outcome of
int square(int board[N_ROWS][N_COLS])
andint square(int (*board)[N_COLS])
- identical upto the least bit. ;-)– Scheff
Nov 15 '18 at 10:44