Remove element from a pointer and pointer to pointer in C
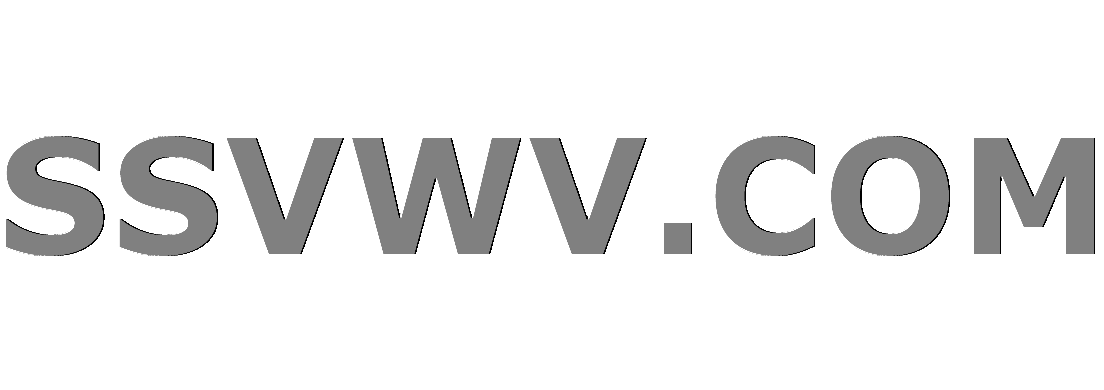
Multi tool use
Still learning, this is a segment of code I'm working on and I'm trying to remove an element(s) from a pointer/pointer-pointer. The problem is near the end of the code.
int total, tempX = 0;
printf("Input total people:n");fflush(stdout);
scanf("%d",&total);
printf("You entered: %in", total);
char **nAmer = (char**) malloc(total * sizeof(char*)); //pointer pointer for username
for (tempX=0; tempX<total; tempX++)
nAmer[tempX] = malloc(21);
double *nUmer = (double*) malloc(total* sizeof(double)); //pointer for usernumber
printf("input their name and number:n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
scanf("%20s %lf", nAmer[tempX], &nUmer[tempX]);
printf("Let me read that back:n");
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
printf("Enter name to remove user(s):n");fflush(stdout);
scanf("%20s",searcher);
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]); //I can assume this wont work well
free(nUmer[tempX]); //I know this is a problem
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
I know free (nAmer[tempX]);
works but doesn't allow for the read back after its removal. What would fix this?
c pointers pointer-to-pointer
add a comment |
Still learning, this is a segment of code I'm working on and I'm trying to remove an element(s) from a pointer/pointer-pointer. The problem is near the end of the code.
int total, tempX = 0;
printf("Input total people:n");fflush(stdout);
scanf("%d",&total);
printf("You entered: %in", total);
char **nAmer = (char**) malloc(total * sizeof(char*)); //pointer pointer for username
for (tempX=0; tempX<total; tempX++)
nAmer[tempX] = malloc(21);
double *nUmer = (double*) malloc(total* sizeof(double)); //pointer for usernumber
printf("input their name and number:n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
scanf("%20s %lf", nAmer[tempX], &nUmer[tempX]);
printf("Let me read that back:n");
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
printf("Enter name to remove user(s):n");fflush(stdout);
scanf("%20s",searcher);
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]); //I can assume this wont work well
free(nUmer[tempX]); //I know this is a problem
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
I know free (nAmer[tempX]);
works but doesn't allow for the read back after its removal. What would fix this?
c pointers pointer-to-pointer
1
Welcome to Stack Overflow! don't cast malloc
– Barmar
Nov 12 '18 at 17:19
add a comment |
Still learning, this is a segment of code I'm working on and I'm trying to remove an element(s) from a pointer/pointer-pointer. The problem is near the end of the code.
int total, tempX = 0;
printf("Input total people:n");fflush(stdout);
scanf("%d",&total);
printf("You entered: %in", total);
char **nAmer = (char**) malloc(total * sizeof(char*)); //pointer pointer for username
for (tempX=0; tempX<total; tempX++)
nAmer[tempX] = malloc(21);
double *nUmer = (double*) malloc(total* sizeof(double)); //pointer for usernumber
printf("input their name and number:n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
scanf("%20s %lf", nAmer[tempX], &nUmer[tempX]);
printf("Let me read that back:n");
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
printf("Enter name to remove user(s):n");fflush(stdout);
scanf("%20s",searcher);
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]); //I can assume this wont work well
free(nUmer[tempX]); //I know this is a problem
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
I know free (nAmer[tempX]);
works but doesn't allow for the read back after its removal. What would fix this?
c pointers pointer-to-pointer
Still learning, this is a segment of code I'm working on and I'm trying to remove an element(s) from a pointer/pointer-pointer. The problem is near the end of the code.
int total, tempX = 0;
printf("Input total people:n");fflush(stdout);
scanf("%d",&total);
printf("You entered: %in", total);
char **nAmer = (char**) malloc(total * sizeof(char*)); //pointer pointer for username
for (tempX=0; tempX<total; tempX++)
nAmer[tempX] = malloc(21);
double *nUmer = (double*) malloc(total* sizeof(double)); //pointer for usernumber
printf("input their name and number:n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
scanf("%20s %lf", nAmer[tempX], &nUmer[tempX]);
printf("Let me read that back:n");
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
printf("Enter name to remove user(s):n");fflush(stdout);
scanf("%20s",searcher);
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]); //I can assume this wont work well
free(nUmer[tempX]); //I know this is a problem
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
I know free (nAmer[tempX]);
works but doesn't allow for the read back after its removal. What would fix this?
c pointers pointer-to-pointer
c pointers pointer-to-pointer
asked Nov 12 '18 at 17:17


Bryan Rosen
205
205
1
Welcome to Stack Overflow! don't cast malloc
– Barmar
Nov 12 '18 at 17:19
add a comment |
1
Welcome to Stack Overflow! don't cast malloc
– Barmar
Nov 12 '18 at 17:19
1
1
Welcome to Stack Overflow! don't cast malloc
– Barmar
Nov 12 '18 at 17:19
Welcome to Stack Overflow! don't cast malloc
– Barmar
Nov 12 '18 at 17:19
add a comment |
2 Answers
2
active
oldest
votes
You shouldn't free(nUmer[tempX]);
because this isn't a pointer.
When you free one of the name pointers, you can set it to NULL
. Then the loop that prints the array that can skip it.
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]);
nAmer[tempX] = NULL;
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
if (nAmer[tempX])
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
You have another mistake:
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
This should just be * sizeof(char)
(or you can just leave this out, since sizeof(char)
is defined to be 1
).
Luckily this allocates more memory than needed, not less.
you can't remove thenUmer
element. You don't need to remove it, you just ignore it because the correspondingnAmer
element isNULL
.
– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because theif
skips it.
– Barmar
Nov 12 '18 at 17:38
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
add a comment |
You have two options.
- Change the pointer in
nAmer
toNULL
after you freed it, don't changenUmer
. That way when processing an entry innAmer[i]
ornUmer[i]
you can check ifnAmer[i]
is valid (!=NULL
) or not (==NULL
) and ignore the entry if it is invalid. - You can move all entries after the removed entry one up in the array and remember that now there are not
total
number of entries but onlytotal-1
number of entries.
Please do not free(nUmer[i])
. The entries in nUmer
are doubles and not pointers. You cannot free
them.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267071%2fremove-element-from-a-pointer-and-pointer-to-pointer-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You shouldn't free(nUmer[tempX]);
because this isn't a pointer.
When you free one of the name pointers, you can set it to NULL
. Then the loop that prints the array that can skip it.
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]);
nAmer[tempX] = NULL;
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
if (nAmer[tempX])
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
You have another mistake:
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
This should just be * sizeof(char)
(or you can just leave this out, since sizeof(char)
is defined to be 1
).
Luckily this allocates more memory than needed, not less.
you can't remove thenUmer
element. You don't need to remove it, you just ignore it because the correspondingnAmer
element isNULL
.
– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because theif
skips it.
– Barmar
Nov 12 '18 at 17:38
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
add a comment |
You shouldn't free(nUmer[tempX]);
because this isn't a pointer.
When you free one of the name pointers, you can set it to NULL
. Then the loop that prints the array that can skip it.
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]);
nAmer[tempX] = NULL;
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
if (nAmer[tempX])
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
You have another mistake:
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
This should just be * sizeof(char)
(or you can just leave this out, since sizeof(char)
is defined to be 1
).
Luckily this allocates more memory than needed, not less.
you can't remove thenUmer
element. You don't need to remove it, you just ignore it because the correspondingnAmer
element isNULL
.
– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because theif
skips it.
– Barmar
Nov 12 '18 at 17:38
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
add a comment |
You shouldn't free(nUmer[tempX]);
because this isn't a pointer.
When you free one of the name pointers, you can set it to NULL
. Then the loop that prints the array that can skip it.
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]);
nAmer[tempX] = NULL;
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
if (nAmer[tempX])
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
You have another mistake:
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
This should just be * sizeof(char)
(or you can just leave this out, since sizeof(char)
is defined to be 1
).
Luckily this allocates more memory than needed, not less.
You shouldn't free(nUmer[tempX]);
because this isn't a pointer.
When you free one of the name pointers, you can set it to NULL
. Then the loop that prints the array that can skip it.
for (tempX = 0; tempX < total; tempX++)
if (strcmp(searcher,nAmer[tempX])==0) //what is better to replace this section?
free(nAmer[tempX]);
nAmer[tempX] = NULL;
printf("Let me read that back with removed user(s):n");fflush(stdout);
for (tempX = 0; tempX<total; tempX++)
if (nAmer[tempX])
printf("Name: %s Number: %lfn", nAmer[tempX], nUmer[tempX]);
You have another mistake:
char *searcher = (char*) malloc(21 * sizeof(char*)); //temporary string made by the user to compare names
This should just be * sizeof(char)
(or you can just leave this out, since sizeof(char)
is defined to be 1
).
Luckily this allocates more memory than needed, not less.
answered Nov 12 '18 at 17:25
Barmar
419k34244344
419k34244344
you can't remove thenUmer
element. You don't need to remove it, you just ignore it because the correspondingnAmer
element isNULL
.
– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because theif
skips it.
– Barmar
Nov 12 '18 at 17:38
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
add a comment |
you can't remove thenUmer
element. You don't need to remove it, you just ignore it because the correspondingnAmer
element isNULL
.
– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because theif
skips it.
– Barmar
Nov 12 '18 at 17:38
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
you can't remove the
nUmer
element. You don't need to remove it, you just ignore it because the corresponding nAmer
element is NULL
.– Barmar
Nov 12 '18 at 17:38
you can't remove the
nUmer
element. You don't need to remove it, you just ignore it because the corresponding nAmer
element is NULL
.– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because the
if
skips it.– Barmar
Nov 12 '18 at 17:38
The null shouldn't be printed, because the
if
skips it.– Barmar
Nov 12 '18 at 17:38
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
BTW, a better way to do this would be with an array of pointers to structures, rather than 2 arrays.
– Barmar
Nov 12 '18 at 17:39
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
yeah didn't notice that if statement, I know about structures and linked lists being better for this, but I wanted to learn more about pointers
– Bryan Rosen
Nov 12 '18 at 17:43
add a comment |
You have two options.
- Change the pointer in
nAmer
toNULL
after you freed it, don't changenUmer
. That way when processing an entry innAmer[i]
ornUmer[i]
you can check ifnAmer[i]
is valid (!=NULL
) or not (==NULL
) and ignore the entry if it is invalid. - You can move all entries after the removed entry one up in the array and remember that now there are not
total
number of entries but onlytotal-1
number of entries.
Please do not free(nUmer[i])
. The entries in nUmer
are doubles and not pointers. You cannot free
them.
add a comment |
You have two options.
- Change the pointer in
nAmer
toNULL
after you freed it, don't changenUmer
. That way when processing an entry innAmer[i]
ornUmer[i]
you can check ifnAmer[i]
is valid (!=NULL
) or not (==NULL
) and ignore the entry if it is invalid. - You can move all entries after the removed entry one up in the array and remember that now there are not
total
number of entries but onlytotal-1
number of entries.
Please do not free(nUmer[i])
. The entries in nUmer
are doubles and not pointers. You cannot free
them.
add a comment |
You have two options.
- Change the pointer in
nAmer
toNULL
after you freed it, don't changenUmer
. That way when processing an entry innAmer[i]
ornUmer[i]
you can check ifnAmer[i]
is valid (!=NULL
) or not (==NULL
) and ignore the entry if it is invalid. - You can move all entries after the removed entry one up in the array and remember that now there are not
total
number of entries but onlytotal-1
number of entries.
Please do not free(nUmer[i])
. The entries in nUmer
are doubles and not pointers. You cannot free
them.
You have two options.
- Change the pointer in
nAmer
toNULL
after you freed it, don't changenUmer
. That way when processing an entry innAmer[i]
ornUmer[i]
you can check ifnAmer[i]
is valid (!=NULL
) or not (==NULL
) and ignore the entry if it is invalid. - You can move all entries after the removed entry one up in the array and remember that now there are not
total
number of entries but onlytotal-1
number of entries.
Please do not free(nUmer[i])
. The entries in nUmer
are doubles and not pointers. You cannot free
them.
answered Nov 12 '18 at 17:26
Werner Henze
10.4k72651
10.4k72651
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53267071%2fremove-element-from-a-pointer-and-pointer-to-pointer-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uyiAevLlbMdd9IX e80V7TY7OqFzWK6tn1xmCoPK o,TiFc,F tPDfm2NeR AZK5bR74,n5r2MWeEZbbjhITOMSFlU4o,UHz OE7JA,cOGBd
1
Welcome to Stack Overflow! don't cast malloc
– Barmar
Nov 12 '18 at 17:19