How to pass vairable to get() in dicitonary in python?
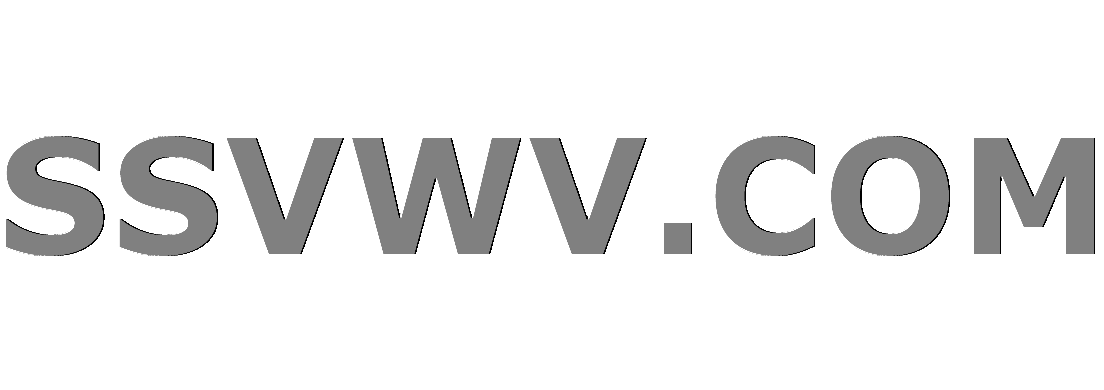
Multi tool use
I am simply trying to solve the following question
You are given a date. Your task is to find what the day is on that date.
Sample imput : 08 10 2018
I came up with following solution:
days='0':'monday','1':'tuesday','2':'wed','3':'thurs','4':'friday','5':'sat','6':'sun'
user_input=datetime.datetime(2018, 11 ,6)
result=user_input.weekday()
print (days.get('result'))
Now it prints 'None' ,I was expecting this to return keys value.
Even print(result)
returns value of 1 and type(result)
returns int.
I want to know why I am not getting the expected output.
There must be a more pythonic way to do this but my major concern is the way get()
is behaving.
python dictionary scripting
add a comment |
I am simply trying to solve the following question
You are given a date. Your task is to find what the day is on that date.
Sample imput : 08 10 2018
I came up with following solution:
days='0':'monday','1':'tuesday','2':'wed','3':'thurs','4':'friday','5':'sat','6':'sun'
user_input=datetime.datetime(2018, 11 ,6)
result=user_input.weekday()
print (days.get('result'))
Now it prints 'None' ,I was expecting this to return keys value.
Even print(result)
returns value of 1 and type(result)
returns int.
I want to know why I am not getting the expected output.
There must be a more pythonic way to do this but my major concern is the way get()
is behaving.
python dictionary scripting
4
I think you meandays.get(result)
notdays.get('result')
. The string'result'
is not in your dictionary. Though maybe the keys in your dictionary should be ints, not strings.
– khelwood
Nov 13 '18 at 14:18
tryprint (days.get(result))
without the quotes.
– Ev. Kounis
Nov 13 '18 at 14:18
days.get(result)
.
– Austin
Nov 13 '18 at 14:19
Eitherprint(days[str(result)])
or change the dictionary keys to integers and thenprint(days[result])
– myrmica
Nov 13 '18 at 14:23
add a comment |
I am simply trying to solve the following question
You are given a date. Your task is to find what the day is on that date.
Sample imput : 08 10 2018
I came up with following solution:
days='0':'monday','1':'tuesday','2':'wed','3':'thurs','4':'friday','5':'sat','6':'sun'
user_input=datetime.datetime(2018, 11 ,6)
result=user_input.weekday()
print (days.get('result'))
Now it prints 'None' ,I was expecting this to return keys value.
Even print(result)
returns value of 1 and type(result)
returns int.
I want to know why I am not getting the expected output.
There must be a more pythonic way to do this but my major concern is the way get()
is behaving.
python dictionary scripting
I am simply trying to solve the following question
You are given a date. Your task is to find what the day is on that date.
Sample imput : 08 10 2018
I came up with following solution:
days='0':'monday','1':'tuesday','2':'wed','3':'thurs','4':'friday','5':'sat','6':'sun'
user_input=datetime.datetime(2018, 11 ,6)
result=user_input.weekday()
print (days.get('result'))
Now it prints 'None' ,I was expecting this to return keys value.
Even print(result)
returns value of 1 and type(result)
returns int.
I want to know why I am not getting the expected output.
There must be a more pythonic way to do this but my major concern is the way get()
is behaving.
python dictionary scripting
python dictionary scripting
asked Nov 13 '18 at 14:16


RustyRusty
1056
1056
4
I think you meandays.get(result)
notdays.get('result')
. The string'result'
is not in your dictionary. Though maybe the keys in your dictionary should be ints, not strings.
– khelwood
Nov 13 '18 at 14:18
tryprint (days.get(result))
without the quotes.
– Ev. Kounis
Nov 13 '18 at 14:18
days.get(result)
.
– Austin
Nov 13 '18 at 14:19
Eitherprint(days[str(result)])
or change the dictionary keys to integers and thenprint(days[result])
– myrmica
Nov 13 '18 at 14:23
add a comment |
4
I think you meandays.get(result)
notdays.get('result')
. The string'result'
is not in your dictionary. Though maybe the keys in your dictionary should be ints, not strings.
– khelwood
Nov 13 '18 at 14:18
tryprint (days.get(result))
without the quotes.
– Ev. Kounis
Nov 13 '18 at 14:18
days.get(result)
.
– Austin
Nov 13 '18 at 14:19
Eitherprint(days[str(result)])
or change the dictionary keys to integers and thenprint(days[result])
– myrmica
Nov 13 '18 at 14:23
4
4
I think you mean
days.get(result)
not days.get('result')
. The string 'result'
is not in your dictionary. Though maybe the keys in your dictionary should be ints, not strings.– khelwood
Nov 13 '18 at 14:18
I think you mean
days.get(result)
not days.get('result')
. The string 'result'
is not in your dictionary. Though maybe the keys in your dictionary should be ints, not strings.– khelwood
Nov 13 '18 at 14:18
try
print (days.get(result))
without the quotes.– Ev. Kounis
Nov 13 '18 at 14:18
try
print (days.get(result))
without the quotes.– Ev. Kounis
Nov 13 '18 at 14:18
days.get(result)
.– Austin
Nov 13 '18 at 14:19
days.get(result)
.– Austin
Nov 13 '18 at 14:19
Either
print(days[str(result)])
or change the dictionary keys to integers and then print(days[result])
– myrmica
Nov 13 '18 at 14:23
Either
print(days[str(result)])
or change the dictionary keys to integers and then print(days[result])
– myrmica
Nov 13 '18 at 14:23
add a comment |
1 Answer
1
active
oldest
votes
Firstly, days.get('result')
is trying to look up the string 'result'
in your dictionary, not the variable result
.
Secondly user_input.weekday()
returns an int, but the keys in your dictionary are strings, not ints.
This would have worked:
days = 0:'monday', 1:'tuesday', 2:'wed', 3:'thurs', 4:'friday', 5:'sat', 6:'sun'
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days.get(result)) # or simply print(days[result])
However, since the possible range of days is the numbers 0 to 6, a list would work just as well.
days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days[result])
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53283017%2fhow-to-pass-vairable-to-get-in-dicitonary-in-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Firstly, days.get('result')
is trying to look up the string 'result'
in your dictionary, not the variable result
.
Secondly user_input.weekday()
returns an int, but the keys in your dictionary are strings, not ints.
This would have worked:
days = 0:'monday', 1:'tuesday', 2:'wed', 3:'thurs', 4:'friday', 5:'sat', 6:'sun'
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days.get(result)) # or simply print(days[result])
However, since the possible range of days is the numbers 0 to 6, a list would work just as well.
days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days[result])
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
add a comment |
Firstly, days.get('result')
is trying to look up the string 'result'
in your dictionary, not the variable result
.
Secondly user_input.weekday()
returns an int, but the keys in your dictionary are strings, not ints.
This would have worked:
days = 0:'monday', 1:'tuesday', 2:'wed', 3:'thurs', 4:'friday', 5:'sat', 6:'sun'
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days.get(result)) # or simply print(days[result])
However, since the possible range of days is the numbers 0 to 6, a list would work just as well.
days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days[result])
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
add a comment |
Firstly, days.get('result')
is trying to look up the string 'result'
in your dictionary, not the variable result
.
Secondly user_input.weekday()
returns an int, but the keys in your dictionary are strings, not ints.
This would have worked:
days = 0:'monday', 1:'tuesday', 2:'wed', 3:'thurs', 4:'friday', 5:'sat', 6:'sun'
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days.get(result)) # or simply print(days[result])
However, since the possible range of days is the numbers 0 to 6, a list would work just as well.
days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days[result])
Firstly, days.get('result')
is trying to look up the string 'result'
in your dictionary, not the variable result
.
Secondly user_input.weekday()
returns an int, but the keys in your dictionary are strings, not ints.
This would have worked:
days = 0:'monday', 1:'tuesday', 2:'wed', 3:'thurs', 4:'friday', 5:'sat', 6:'sun'
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days.get(result)) # or simply print(days[result])
However, since the possible range of days is the numbers 0 to 6, a list would work just as well.
days = ['Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday']
user_input = datetime.datetime(2018, 11, 6)
result = user_input.weekday()
print(days[result])
answered Nov 13 '18 at 14:25


khelwoodkhelwood
30.8k74263
30.8k74263
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
add a comment |
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
I was not aware that I am looking for result as key itself. I was given this question in sys admin Interview scripting part and Idk why dictionary came in mind ,I should have used list. Thanks for the help mate.
– Rusty
Nov 13 '18 at 14:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53283017%2fhow-to-pass-vairable-to-get-in-dicitonary-in-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ri6YooEJM3FrI uaOnN0h5dELpsyGi6w
4
I think you mean
days.get(result)
notdays.get('result')
. The string'result'
is not in your dictionary. Though maybe the keys in your dictionary should be ints, not strings.– khelwood
Nov 13 '18 at 14:18
try
print (days.get(result))
without the quotes.– Ev. Kounis
Nov 13 '18 at 14:18
days.get(result)
.– Austin
Nov 13 '18 at 14:19
Either
print(days[str(result)])
or change the dictionary keys to integers and thenprint(days[result])
– myrmica
Nov 13 '18 at 14:23