Exceptions while using MiniDumpWriteDump() Win32 API while debuging
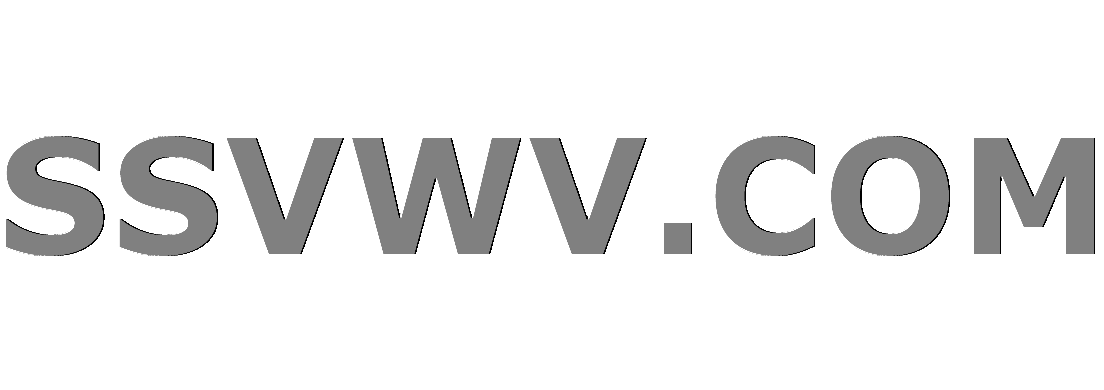
Multi tool use
I spawn a process "2" from a process "1" in C++/CLI. While both are running, process "1" kills process "2" (by design). My goal is to produce a mini dump of "2" just before killing it.
Here is my code:
// mpProcess started with System::Diagnostics::Process... etc.
System::IO::FileStream^ fs = gcnew System::IO::FileStream("MyPath.dmp");
MiniDumpWriteDump( mpProcess->Handle.ToPointer(), mpProcess->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr);
fs->Close();
When I start process "1" from a command line, without attaching it to the debugger, it runs normally, starts process "2" then dumps it then kills it.
When I start process "1" in the debugger, I get 2-3 AccessViolationException when I step over the call to MiniDumpWriteDump
, but if I click onto "Continue" everything goes fine and the dump file is produced.
What is going on? Is there something wrong in my design? Notice that it is the first time I use this API, I didn't even know I could dump such a file 24 hours ago ;-) ! I will be grateful for your kind help to improve my skills with dump files.
EDIT 1 Added Exception information:
Here is the message I got:
Exception thrown at 0x000007FED860FD31 (mscordacwks.dll) in MYProg.exe: 0xC0000005: Access violation reading location 0x0000000000000000.
If there is a handler for this exception, the program may be safely continued.
EDIT 2 Added a snippet and a stack trace
Process 1: The "killer"
// Process1.cpp : main project file.
#include "stdafx.h"
#define WIN32_LEAN_AND_MEAN
#include <windows.h>
#include <dbghelp.h>
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process1! I'll "minidump" Process2 then kill it!");
System::Diagnostics::Process^ p = gcnew System::Diagnostics::Process();
p->StartInfo->FileName = "Process2.exe";
p->Start();
System::Threading::Thread::Sleep( 3000 );
System::IO::FileStream^ fs = gcnew System::IO::FileStream( "minidump.dmp", System::IO::FileMode::Create );
MiniDumpWriteDump( p->Handle.ToPointer(), p->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr );
fs->Close();
p->Kill();
return 0;
Process 2: the "dumped"
// Process2.cpp : main project file.
#include "stdafx.h"
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process2! I'm waiting to be killed by Process1!");
// Do nothing, wait to be killed
while ( true )
System::Threading::Thread::Sleep( 1000 );
return 0;
Stack trace when I break it from within the (AccessViolation)Exception dialog:
mscordacwks.dll!000007fed860fd31() Unknown
mscordacwks.dll!000007fed861028c() Unknown
mscordacwks.dll!000007fed8610fd2() Unknown
mscordacwks.dll!000007fed861165f() Unknown
mscordacwks.dll!000007fed861176e() Unknown
dbghelp.dll!GenGetAuxMemory(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS *) Unknown
dbghelp.dll!GenGetProcessInfo(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS * *) Unknown
dbghelp.dll!MiniDumpProvideDump() Unknown
dbghelp.dll!MiniDumpWriteDump() Unknown
[Managed to Native Transition]
[CURSOR]>>> Process1.exe!main(array<System::String^>^ args=array<System::String^>(0)) Line 24 C++
Process1.exe!mainCRTStartupStrArray(array<System::String^>^ arguments=array<System::String^>(0)) Line 249 C++
[Native to Managed Transition]
mscoreei.dll!000007feee467a6d() Unknown
mscoree.dll!_CorExeMain_Exported() Unknown
kernel32.dll!BaseThreadInitThunk() Unknown
ntdll.dll!RtlUserThreadStart() Unknown
.net c++-cli dump minidump minidumpwritedump
|
show 2 more comments
I spawn a process "2" from a process "1" in C++/CLI. While both are running, process "1" kills process "2" (by design). My goal is to produce a mini dump of "2" just before killing it.
Here is my code:
// mpProcess started with System::Diagnostics::Process... etc.
System::IO::FileStream^ fs = gcnew System::IO::FileStream("MyPath.dmp");
MiniDumpWriteDump( mpProcess->Handle.ToPointer(), mpProcess->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr);
fs->Close();
When I start process "1" from a command line, without attaching it to the debugger, it runs normally, starts process "2" then dumps it then kills it.
When I start process "1" in the debugger, I get 2-3 AccessViolationException when I step over the call to MiniDumpWriteDump
, but if I click onto "Continue" everything goes fine and the dump file is produced.
What is going on? Is there something wrong in my design? Notice that it is the first time I use this API, I didn't even know I could dump such a file 24 hours ago ;-) ! I will be grateful for your kind help to improve my skills with dump files.
EDIT 1 Added Exception information:
Here is the message I got:
Exception thrown at 0x000007FED860FD31 (mscordacwks.dll) in MYProg.exe: 0xC0000005: Access violation reading location 0x0000000000000000.
If there is a handler for this exception, the program may be safely continued.
EDIT 2 Added a snippet and a stack trace
Process 1: The "killer"
// Process1.cpp : main project file.
#include "stdafx.h"
#define WIN32_LEAN_AND_MEAN
#include <windows.h>
#include <dbghelp.h>
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process1! I'll "minidump" Process2 then kill it!");
System::Diagnostics::Process^ p = gcnew System::Diagnostics::Process();
p->StartInfo->FileName = "Process2.exe";
p->Start();
System::Threading::Thread::Sleep( 3000 );
System::IO::FileStream^ fs = gcnew System::IO::FileStream( "minidump.dmp", System::IO::FileMode::Create );
MiniDumpWriteDump( p->Handle.ToPointer(), p->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr );
fs->Close();
p->Kill();
return 0;
Process 2: the "dumped"
// Process2.cpp : main project file.
#include "stdafx.h"
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process2! I'm waiting to be killed by Process1!");
// Do nothing, wait to be killed
while ( true )
System::Threading::Thread::Sleep( 1000 );
return 0;
Stack trace when I break it from within the (AccessViolation)Exception dialog:
mscordacwks.dll!000007fed860fd31() Unknown
mscordacwks.dll!000007fed861028c() Unknown
mscordacwks.dll!000007fed8610fd2() Unknown
mscordacwks.dll!000007fed861165f() Unknown
mscordacwks.dll!000007fed861176e() Unknown
dbghelp.dll!GenGetAuxMemory(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS *) Unknown
dbghelp.dll!GenGetProcessInfo(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS * *) Unknown
dbghelp.dll!MiniDumpProvideDump() Unknown
dbghelp.dll!MiniDumpWriteDump() Unknown
[Managed to Native Transition]
[CURSOR]>>> Process1.exe!main(array<System::String^>^ args=array<System::String^>(0)) Line 24 C++
Process1.exe!mainCRTStartupStrArray(array<System::String^>^ arguments=array<System::String^>(0)) Line 249 C++
[Native to Managed Transition]
mscoreei.dll!000007feee467a6d() Unknown
mscoree.dll!_CorExeMain_Exported() Unknown
kernel32.dll!BaseThreadInitThunk() Unknown
ntdll.dll!RtlUserThreadStart() Unknown
.net c++-cli dump minidump minidumpwritedump
@HansPassant See my Edit 2. You'll find a snippet of both apps and the stack trace when it breaks on exception. Note: VS 2015 Update 3 if it can help...
– dom_beau
Nov 13 '18 at 16:27
@HansPassant ...also note: created from scratch sostdafx.h/.cpp
,AssemblyInfo.cpp
etc are auto created by VS. I did not modify them...
– dom_beau
Nov 13 '18 at 16:29
@HansPassant dbghelp.dll: 6.1.7601.17514; both my apps were created on the same system/solution one after the other. The platform target is 8.1, the .NET is 4.6.1.
– dom_beau
Nov 13 '18 at 16:55
That is ancient Win7. Do you have any memory of using a recent SDK version of dbghelp.dll? Do you have a local copy of it? Double-check with the debugger's Debug > Windows > Modules window, it shows the actual dbghelp.dll that got loaded. I meant platform as in x64 vs Win32/x86.
– Hans Passant
Nov 13 '18 at 17:06
1
I don't think you have a real problem, just the debugger showing you more than you bargained for. DbgHelp knows how to take a lickin' and keep on tickin'. Root cause is surely a very old version of dbghelp.dll probing a very new version of the CLR. I have no good way to verify that.
– Hans Passant
Nov 13 '18 at 17:56
|
show 2 more comments
I spawn a process "2" from a process "1" in C++/CLI. While both are running, process "1" kills process "2" (by design). My goal is to produce a mini dump of "2" just before killing it.
Here is my code:
// mpProcess started with System::Diagnostics::Process... etc.
System::IO::FileStream^ fs = gcnew System::IO::FileStream("MyPath.dmp");
MiniDumpWriteDump( mpProcess->Handle.ToPointer(), mpProcess->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr);
fs->Close();
When I start process "1" from a command line, without attaching it to the debugger, it runs normally, starts process "2" then dumps it then kills it.
When I start process "1" in the debugger, I get 2-3 AccessViolationException when I step over the call to MiniDumpWriteDump
, but if I click onto "Continue" everything goes fine and the dump file is produced.
What is going on? Is there something wrong in my design? Notice that it is the first time I use this API, I didn't even know I could dump such a file 24 hours ago ;-) ! I will be grateful for your kind help to improve my skills with dump files.
EDIT 1 Added Exception information:
Here is the message I got:
Exception thrown at 0x000007FED860FD31 (mscordacwks.dll) in MYProg.exe: 0xC0000005: Access violation reading location 0x0000000000000000.
If there is a handler for this exception, the program may be safely continued.
EDIT 2 Added a snippet and a stack trace
Process 1: The "killer"
// Process1.cpp : main project file.
#include "stdafx.h"
#define WIN32_LEAN_AND_MEAN
#include <windows.h>
#include <dbghelp.h>
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process1! I'll "minidump" Process2 then kill it!");
System::Diagnostics::Process^ p = gcnew System::Diagnostics::Process();
p->StartInfo->FileName = "Process2.exe";
p->Start();
System::Threading::Thread::Sleep( 3000 );
System::IO::FileStream^ fs = gcnew System::IO::FileStream( "minidump.dmp", System::IO::FileMode::Create );
MiniDumpWriteDump( p->Handle.ToPointer(), p->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr );
fs->Close();
p->Kill();
return 0;
Process 2: the "dumped"
// Process2.cpp : main project file.
#include "stdafx.h"
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process2! I'm waiting to be killed by Process1!");
// Do nothing, wait to be killed
while ( true )
System::Threading::Thread::Sleep( 1000 );
return 0;
Stack trace when I break it from within the (AccessViolation)Exception dialog:
mscordacwks.dll!000007fed860fd31() Unknown
mscordacwks.dll!000007fed861028c() Unknown
mscordacwks.dll!000007fed8610fd2() Unknown
mscordacwks.dll!000007fed861165f() Unknown
mscordacwks.dll!000007fed861176e() Unknown
dbghelp.dll!GenGetAuxMemory(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS *) Unknown
dbghelp.dll!GenGetProcessInfo(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS * *) Unknown
dbghelp.dll!MiniDumpProvideDump() Unknown
dbghelp.dll!MiniDumpWriteDump() Unknown
[Managed to Native Transition]
[CURSOR]>>> Process1.exe!main(array<System::String^>^ args=array<System::String^>(0)) Line 24 C++
Process1.exe!mainCRTStartupStrArray(array<System::String^>^ arguments=array<System::String^>(0)) Line 249 C++
[Native to Managed Transition]
mscoreei.dll!000007feee467a6d() Unknown
mscoree.dll!_CorExeMain_Exported() Unknown
kernel32.dll!BaseThreadInitThunk() Unknown
ntdll.dll!RtlUserThreadStart() Unknown
.net c++-cli dump minidump minidumpwritedump
I spawn a process "2" from a process "1" in C++/CLI. While both are running, process "1" kills process "2" (by design). My goal is to produce a mini dump of "2" just before killing it.
Here is my code:
// mpProcess started with System::Diagnostics::Process... etc.
System::IO::FileStream^ fs = gcnew System::IO::FileStream("MyPath.dmp");
MiniDumpWriteDump( mpProcess->Handle.ToPointer(), mpProcess->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr);
fs->Close();
When I start process "1" from a command line, without attaching it to the debugger, it runs normally, starts process "2" then dumps it then kills it.
When I start process "1" in the debugger, I get 2-3 AccessViolationException when I step over the call to MiniDumpWriteDump
, but if I click onto "Continue" everything goes fine and the dump file is produced.
What is going on? Is there something wrong in my design? Notice that it is the first time I use this API, I didn't even know I could dump such a file 24 hours ago ;-) ! I will be grateful for your kind help to improve my skills with dump files.
EDIT 1 Added Exception information:
Here is the message I got:
Exception thrown at 0x000007FED860FD31 (mscordacwks.dll) in MYProg.exe: 0xC0000005: Access violation reading location 0x0000000000000000.
If there is a handler for this exception, the program may be safely continued.
EDIT 2 Added a snippet and a stack trace
Process 1: The "killer"
// Process1.cpp : main project file.
#include "stdafx.h"
#define WIN32_LEAN_AND_MEAN
#include <windows.h>
#include <dbghelp.h>
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process1! I'll "minidump" Process2 then kill it!");
System::Diagnostics::Process^ p = gcnew System::Diagnostics::Process();
p->StartInfo->FileName = "Process2.exe";
p->Start();
System::Threading::Thread::Sleep( 3000 );
System::IO::FileStream^ fs = gcnew System::IO::FileStream( "minidump.dmp", System::IO::FileMode::Create );
MiniDumpWriteDump( p->Handle.ToPointer(), p->Id, fs->SafeFileHandle->DangerousGetHandle().ToPointer(), MINIDUMP_TYPE::MiniDumpNormal, nullptr, nullptr, nullptr );
fs->Close();
p->Kill();
return 0;
Process 2: the "dumped"
// Process2.cpp : main project file.
#include "stdafx.h"
using namespace System;
int main(array<System::String ^> ^args)
Console::WriteLine(L"Hello, I'm Process2! I'm waiting to be killed by Process1!");
// Do nothing, wait to be killed
while ( true )
System::Threading::Thread::Sleep( 1000 );
return 0;
Stack trace when I break it from within the (AccessViolation)Exception dialog:
mscordacwks.dll!000007fed860fd31() Unknown
mscordacwks.dll!000007fed861028c() Unknown
mscordacwks.dll!000007fed8610fd2() Unknown
mscordacwks.dll!000007fed861165f() Unknown
mscordacwks.dll!000007fed861176e() Unknown
dbghelp.dll!GenGetAuxMemory(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS *) Unknown
dbghelp.dll!GenGetProcessInfo(struct _MINIDUMP_STATE *,struct _INTERNAL_PROCESS * *) Unknown
dbghelp.dll!MiniDumpProvideDump() Unknown
dbghelp.dll!MiniDumpWriteDump() Unknown
[Managed to Native Transition]
[CURSOR]>>> Process1.exe!main(array<System::String^>^ args=array<System::String^>(0)) Line 24 C++
Process1.exe!mainCRTStartupStrArray(array<System::String^>^ arguments=array<System::String^>(0)) Line 249 C++
[Native to Managed Transition]
mscoreei.dll!000007feee467a6d() Unknown
mscoree.dll!_CorExeMain_Exported() Unknown
kernel32.dll!BaseThreadInitThunk() Unknown
ntdll.dll!RtlUserThreadStart() Unknown
.net c++-cli dump minidump minidumpwritedump
.net c++-cli dump minidump minidumpwritedump
edited Nov 13 '18 at 16:24
dom_beau
asked Nov 13 '18 at 13:41
dom_beaudom_beau
95821343
95821343
@HansPassant See my Edit 2. You'll find a snippet of both apps and the stack trace when it breaks on exception. Note: VS 2015 Update 3 if it can help...
– dom_beau
Nov 13 '18 at 16:27
@HansPassant ...also note: created from scratch sostdafx.h/.cpp
,AssemblyInfo.cpp
etc are auto created by VS. I did not modify them...
– dom_beau
Nov 13 '18 at 16:29
@HansPassant dbghelp.dll: 6.1.7601.17514; both my apps were created on the same system/solution one after the other. The platform target is 8.1, the .NET is 4.6.1.
– dom_beau
Nov 13 '18 at 16:55
That is ancient Win7. Do you have any memory of using a recent SDK version of dbghelp.dll? Do you have a local copy of it? Double-check with the debugger's Debug > Windows > Modules window, it shows the actual dbghelp.dll that got loaded. I meant platform as in x64 vs Win32/x86.
– Hans Passant
Nov 13 '18 at 17:06
1
I don't think you have a real problem, just the debugger showing you more than you bargained for. DbgHelp knows how to take a lickin' and keep on tickin'. Root cause is surely a very old version of dbghelp.dll probing a very new version of the CLR. I have no good way to verify that.
– Hans Passant
Nov 13 '18 at 17:56
|
show 2 more comments
@HansPassant See my Edit 2. You'll find a snippet of both apps and the stack trace when it breaks on exception. Note: VS 2015 Update 3 if it can help...
– dom_beau
Nov 13 '18 at 16:27
@HansPassant ...also note: created from scratch sostdafx.h/.cpp
,AssemblyInfo.cpp
etc are auto created by VS. I did not modify them...
– dom_beau
Nov 13 '18 at 16:29
@HansPassant dbghelp.dll: 6.1.7601.17514; both my apps were created on the same system/solution one after the other. The platform target is 8.1, the .NET is 4.6.1.
– dom_beau
Nov 13 '18 at 16:55
That is ancient Win7. Do you have any memory of using a recent SDK version of dbghelp.dll? Do you have a local copy of it? Double-check with the debugger's Debug > Windows > Modules window, it shows the actual dbghelp.dll that got loaded. I meant platform as in x64 vs Win32/x86.
– Hans Passant
Nov 13 '18 at 17:06
1
I don't think you have a real problem, just the debugger showing you more than you bargained for. DbgHelp knows how to take a lickin' and keep on tickin'. Root cause is surely a very old version of dbghelp.dll probing a very new version of the CLR. I have no good way to verify that.
– Hans Passant
Nov 13 '18 at 17:56
@HansPassant See my Edit 2. You'll find a snippet of both apps and the stack trace when it breaks on exception. Note: VS 2015 Update 3 if it can help...
– dom_beau
Nov 13 '18 at 16:27
@HansPassant See my Edit 2. You'll find a snippet of both apps and the stack trace when it breaks on exception. Note: VS 2015 Update 3 if it can help...
– dom_beau
Nov 13 '18 at 16:27
@HansPassant ...also note: created from scratch so
stdafx.h/.cpp
, AssemblyInfo.cpp
etc are auto created by VS. I did not modify them...– dom_beau
Nov 13 '18 at 16:29
@HansPassant ...also note: created from scratch so
stdafx.h/.cpp
, AssemblyInfo.cpp
etc are auto created by VS. I did not modify them...– dom_beau
Nov 13 '18 at 16:29
@HansPassant dbghelp.dll: 6.1.7601.17514; both my apps were created on the same system/solution one after the other. The platform target is 8.1, the .NET is 4.6.1.
– dom_beau
Nov 13 '18 at 16:55
@HansPassant dbghelp.dll: 6.1.7601.17514; both my apps were created on the same system/solution one after the other. The platform target is 8.1, the .NET is 4.6.1.
– dom_beau
Nov 13 '18 at 16:55
That is ancient Win7. Do you have any memory of using a recent SDK version of dbghelp.dll? Do you have a local copy of it? Double-check with the debugger's Debug > Windows > Modules window, it shows the actual dbghelp.dll that got loaded. I meant platform as in x64 vs Win32/x86.
– Hans Passant
Nov 13 '18 at 17:06
That is ancient Win7. Do you have any memory of using a recent SDK version of dbghelp.dll? Do you have a local copy of it? Double-check with the debugger's Debug > Windows > Modules window, it shows the actual dbghelp.dll that got loaded. I meant platform as in x64 vs Win32/x86.
– Hans Passant
Nov 13 '18 at 17:06
1
1
I don't think you have a real problem, just the debugger showing you more than you bargained for. DbgHelp knows how to take a lickin' and keep on tickin'. Root cause is surely a very old version of dbghelp.dll probing a very new version of the CLR. I have no good way to verify that.
– Hans Passant
Nov 13 '18 at 17:56
I don't think you have a real problem, just the debugger showing you more than you bargained for. DbgHelp knows how to take a lickin' and keep on tickin'. Root cause is surely a very old version of dbghelp.dll probing a very new version of the CLR. I have no good way to verify that.
– Hans Passant
Nov 13 '18 at 17:56
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282342%2fexceptions-while-using-minidumpwritedump-win32-api-while-debuging%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282342%2fexceptions-while-using-minidumpwritedump-win32-api-while-debuging%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cQ7cpz,ZjvM1tS2Aq7
@HansPassant See my Edit 2. You'll find a snippet of both apps and the stack trace when it breaks on exception. Note: VS 2015 Update 3 if it can help...
– dom_beau
Nov 13 '18 at 16:27
@HansPassant ...also note: created from scratch so
stdafx.h/.cpp
,AssemblyInfo.cpp
etc are auto created by VS. I did not modify them...– dom_beau
Nov 13 '18 at 16:29
@HansPassant dbghelp.dll: 6.1.7601.17514; both my apps were created on the same system/solution one after the other. The platform target is 8.1, the .NET is 4.6.1.
– dom_beau
Nov 13 '18 at 16:55
That is ancient Win7. Do you have any memory of using a recent SDK version of dbghelp.dll? Do you have a local copy of it? Double-check with the debugger's Debug > Windows > Modules window, it shows the actual dbghelp.dll that got loaded. I meant platform as in x64 vs Win32/x86.
– Hans Passant
Nov 13 '18 at 17:06
1
I don't think you have a real problem, just the debugger showing you more than you bargained for. DbgHelp knows how to take a lickin' and keep on tickin'. Root cause is surely a very old version of dbghelp.dll probing a very new version of the CLR. I have no good way to verify that.
– Hans Passant
Nov 13 '18 at 17:56