How can I initialize a list string property without throwing a null exception?
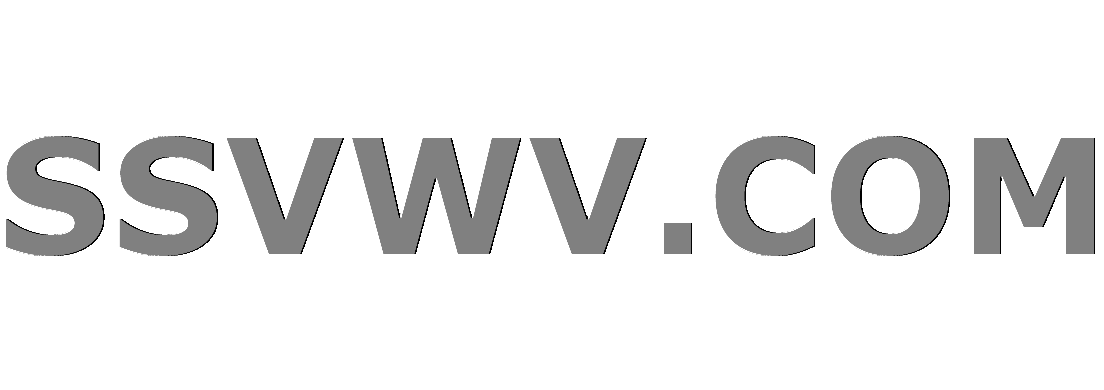
Multi tool use
I have an MVC controller with a jsonResult that transfers a session cart to Squares CreateOrderRequestLineItem List, but when I Initialize the list the code throws a null exception for quantity because it is a required property. I've mapped the quantity and even straight up set it to a string value I set the property quantity but the null exception is thrown before the code even reaches the mapping. Do I need to set the quantity to not null or 0 if null somewhere? Here is my controller code.
The session cart builds a cart based off of a product class and product model with set values of productid, name, quantity, price, and tax.
How do I map to a new list if it throws a null exception before my code can reach the mapping?
Note: I added another List ItemDetail to transfer the session data after the null exception error was already thrown and this didn't work either.
Note two: when I don't use Square object references and just use my generic lists and return json my quantity shows up with no nulls for every item in my cart.
public JsonResult CheckoutRequest(string id)
List<Item> cart = (List<Item>)Session["cart"];
List<ItemDetails> itemDetails = cart.Select(s => new ItemDetails
ProductID = Convert.ToInt32(s.Product.ProductID),
Name = s.Product.Name,
Price = s.Product.Price,
Quantity = Convert.ToString(s.Quantity)
).ToList();
var lineItems = itemDetails.Select(s => new CreateOrderRequestLineItem()
Quantity = "1",
Name = s.Name,
BasePriceMoney = new Money
Amount = s.Price,
Currency = Money.CurrencyEnum.USD,
,
CatalogObjectId = Convert.ToString(s.ProductID),
).ToList();
CreateOrderRequest order = new CreateOrderRequest
ReferenceId = Convert.ToString(Guid.NewGuid()),
LineItems = lineItems
;
// Configuration.Default.AccessToken = "YOUR_ACCESS_TOKEN";
var body = new CreateCheckoutRequest
IdempotencyKey = Convert.ToString(Guid.NewGuid()),
Order = order,
AskForShippingAddress = true,
MerchantSupportEmail = "americanapparelusa@aol.com"
;
return Json(new
locationId,
body
, JsonRequestBehavior.AllowGet);
javascript c# asp.net-mvc visual-studio square-connect
add a comment |
I have an MVC controller with a jsonResult that transfers a session cart to Squares CreateOrderRequestLineItem List, but when I Initialize the list the code throws a null exception for quantity because it is a required property. I've mapped the quantity and even straight up set it to a string value I set the property quantity but the null exception is thrown before the code even reaches the mapping. Do I need to set the quantity to not null or 0 if null somewhere? Here is my controller code.
The session cart builds a cart based off of a product class and product model with set values of productid, name, quantity, price, and tax.
How do I map to a new list if it throws a null exception before my code can reach the mapping?
Note: I added another List ItemDetail to transfer the session data after the null exception error was already thrown and this didn't work either.
Note two: when I don't use Square object references and just use my generic lists and return json my quantity shows up with no nulls for every item in my cart.
public JsonResult CheckoutRequest(string id)
List<Item> cart = (List<Item>)Session["cart"];
List<ItemDetails> itemDetails = cart.Select(s => new ItemDetails
ProductID = Convert.ToInt32(s.Product.ProductID),
Name = s.Product.Name,
Price = s.Product.Price,
Quantity = Convert.ToString(s.Quantity)
).ToList();
var lineItems = itemDetails.Select(s => new CreateOrderRequestLineItem()
Quantity = "1",
Name = s.Name,
BasePriceMoney = new Money
Amount = s.Price,
Currency = Money.CurrencyEnum.USD,
,
CatalogObjectId = Convert.ToString(s.ProductID),
).ToList();
CreateOrderRequest order = new CreateOrderRequest
ReferenceId = Convert.ToString(Guid.NewGuid()),
LineItems = lineItems
;
// Configuration.Default.AccessToken = "YOUR_ACCESS_TOKEN";
var body = new CreateCheckoutRequest
IdempotencyKey = Convert.ToString(Guid.NewGuid()),
Order = order,
AskForShippingAddress = true,
MerchantSupportEmail = "americanapparelusa@aol.com"
;
return Json(new
locationId,
body
, JsonRequestBehavior.AllowGet);
javascript c# asp.net-mvc visual-studio square-connect
Is this line throwing null reference/NRE:List<Item> cart = (List<Item>)Session["cart"]
? Try putting null check against the session variable before casting toList<Item>
.
– Tetsuya Yamamoto
Nov 13 '18 at 4:02
Is theQuantity
property of your ItemDetails class astring
type ? Why ? Try to use the correct types. Use a numeric type to store numeric data.
– Shyju
Nov 13 '18 at 4:05
add a comment |
I have an MVC controller with a jsonResult that transfers a session cart to Squares CreateOrderRequestLineItem List, but when I Initialize the list the code throws a null exception for quantity because it is a required property. I've mapped the quantity and even straight up set it to a string value I set the property quantity but the null exception is thrown before the code even reaches the mapping. Do I need to set the quantity to not null or 0 if null somewhere? Here is my controller code.
The session cart builds a cart based off of a product class and product model with set values of productid, name, quantity, price, and tax.
How do I map to a new list if it throws a null exception before my code can reach the mapping?
Note: I added another List ItemDetail to transfer the session data after the null exception error was already thrown and this didn't work either.
Note two: when I don't use Square object references and just use my generic lists and return json my quantity shows up with no nulls for every item in my cart.
public JsonResult CheckoutRequest(string id)
List<Item> cart = (List<Item>)Session["cart"];
List<ItemDetails> itemDetails = cart.Select(s => new ItemDetails
ProductID = Convert.ToInt32(s.Product.ProductID),
Name = s.Product.Name,
Price = s.Product.Price,
Quantity = Convert.ToString(s.Quantity)
).ToList();
var lineItems = itemDetails.Select(s => new CreateOrderRequestLineItem()
Quantity = "1",
Name = s.Name,
BasePriceMoney = new Money
Amount = s.Price,
Currency = Money.CurrencyEnum.USD,
,
CatalogObjectId = Convert.ToString(s.ProductID),
).ToList();
CreateOrderRequest order = new CreateOrderRequest
ReferenceId = Convert.ToString(Guid.NewGuid()),
LineItems = lineItems
;
// Configuration.Default.AccessToken = "YOUR_ACCESS_TOKEN";
var body = new CreateCheckoutRequest
IdempotencyKey = Convert.ToString(Guid.NewGuid()),
Order = order,
AskForShippingAddress = true,
MerchantSupportEmail = "americanapparelusa@aol.com"
;
return Json(new
locationId,
body
, JsonRequestBehavior.AllowGet);
javascript c# asp.net-mvc visual-studio square-connect
I have an MVC controller with a jsonResult that transfers a session cart to Squares CreateOrderRequestLineItem List, but when I Initialize the list the code throws a null exception for quantity because it is a required property. I've mapped the quantity and even straight up set it to a string value I set the property quantity but the null exception is thrown before the code even reaches the mapping. Do I need to set the quantity to not null or 0 if null somewhere? Here is my controller code.
The session cart builds a cart based off of a product class and product model with set values of productid, name, quantity, price, and tax.
How do I map to a new list if it throws a null exception before my code can reach the mapping?
Note: I added another List ItemDetail to transfer the session data after the null exception error was already thrown and this didn't work either.
Note two: when I don't use Square object references and just use my generic lists and return json my quantity shows up with no nulls for every item in my cart.
public JsonResult CheckoutRequest(string id)
List<Item> cart = (List<Item>)Session["cart"];
List<ItemDetails> itemDetails = cart.Select(s => new ItemDetails
ProductID = Convert.ToInt32(s.Product.ProductID),
Name = s.Product.Name,
Price = s.Product.Price,
Quantity = Convert.ToString(s.Quantity)
).ToList();
var lineItems = itemDetails.Select(s => new CreateOrderRequestLineItem()
Quantity = "1",
Name = s.Name,
BasePriceMoney = new Money
Amount = s.Price,
Currency = Money.CurrencyEnum.USD,
,
CatalogObjectId = Convert.ToString(s.ProductID),
).ToList();
CreateOrderRequest order = new CreateOrderRequest
ReferenceId = Convert.ToString(Guid.NewGuid()),
LineItems = lineItems
;
// Configuration.Default.AccessToken = "YOUR_ACCESS_TOKEN";
var body = new CreateCheckoutRequest
IdempotencyKey = Convert.ToString(Guid.NewGuid()),
Order = order,
AskForShippingAddress = true,
MerchantSupportEmail = "americanapparelusa@aol.com"
;
return Json(new
locationId,
body
, JsonRequestBehavior.AllowGet);
javascript c# asp.net-mvc visual-studio square-connect
javascript c# asp.net-mvc visual-studio square-connect
edited Nov 13 '18 at 5:30


Gihan Saranga Siriwardhana
606424
606424
asked Nov 13 '18 at 4:00
MilesMiles
1
1
Is this line throwing null reference/NRE:List<Item> cart = (List<Item>)Session["cart"]
? Try putting null check against the session variable before casting toList<Item>
.
– Tetsuya Yamamoto
Nov 13 '18 at 4:02
Is theQuantity
property of your ItemDetails class astring
type ? Why ? Try to use the correct types. Use a numeric type to store numeric data.
– Shyju
Nov 13 '18 at 4:05
add a comment |
Is this line throwing null reference/NRE:List<Item> cart = (List<Item>)Session["cart"]
? Try putting null check against the session variable before casting toList<Item>
.
– Tetsuya Yamamoto
Nov 13 '18 at 4:02
Is theQuantity
property of your ItemDetails class astring
type ? Why ? Try to use the correct types. Use a numeric type to store numeric data.
– Shyju
Nov 13 '18 at 4:05
Is this line throwing null reference/NRE:
List<Item> cart = (List<Item>)Session["cart"]
? Try putting null check against the session variable before casting to List<Item>
.– Tetsuya Yamamoto
Nov 13 '18 at 4:02
Is this line throwing null reference/NRE:
List<Item> cart = (List<Item>)Session["cart"]
? Try putting null check against the session variable before casting to List<Item>
.– Tetsuya Yamamoto
Nov 13 '18 at 4:02
Is the
Quantity
property of your ItemDetails class a string
type ? Why ? Try to use the correct types. Use a numeric type to store numeric data.– Shyju
Nov 13 '18 at 4:05
Is the
Quantity
property of your ItemDetails class a string
type ? Why ? Try to use the correct types. Use a numeric type to store numeric data.– Shyju
Nov 13 '18 at 4:05
add a comment |
2 Answers
2
active
oldest
votes
Please change the following
List<Item> cart = (List<Item>)Session["cart"];
into
List<Item> cart = new List<Item>();
if (Session["cart"] != null && Session["cart"].ToString() != null && Session["cart"].ToString() != "")
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
add a comment |
If the Session
variable contains List<Item>
which potentially has null value, you should use as
operator and then check against null value:
var cart = Session["cart"] as List<Item>;
if (cart == null)
// do something
else
// use mapping here
However if the Session
variable contains JSON data, you can try casting as string
type and deserialize it when available:
List<Item> cart = new List<Item>();
if (!string.IsNullOrEmpty(Session["cart"] as string))
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
// use mapping here
else
// do something else
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273580%2fhow-can-i-initialize-a-list-string-property-without-throwing-a-null-exception%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Please change the following
List<Item> cart = (List<Item>)Session["cart"];
into
List<Item> cart = new List<Item>();
if (Session["cart"] != null && Session["cart"].ToString() != null && Session["cart"].ToString() != "")
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
add a comment |
Please change the following
List<Item> cart = (List<Item>)Session["cart"];
into
List<Item> cart = new List<Item>();
if (Session["cart"] != null && Session["cart"].ToString() != null && Session["cart"].ToString() != "")
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
add a comment |
Please change the following
List<Item> cart = (List<Item>)Session["cart"];
into
List<Item> cart = new List<Item>();
if (Session["cart"] != null && Session["cart"].ToString() != null && Session["cart"].ToString() != "")
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
Please change the following
List<Item> cart = (List<Item>)Session["cart"];
into
List<Item> cart = new List<Item>();
if (Session["cart"] != null && Session["cart"].ToString() != null && Session["cart"].ToString() != "")
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
answered Nov 13 '18 at 4:15


Maths RkBalaMaths RkBala
1,9121116
1,9121116
add a comment |
add a comment |
If the Session
variable contains List<Item>
which potentially has null value, you should use as
operator and then check against null value:
var cart = Session["cart"] as List<Item>;
if (cart == null)
// do something
else
// use mapping here
However if the Session
variable contains JSON data, you can try casting as string
type and deserialize it when available:
List<Item> cart = new List<Item>();
if (!string.IsNullOrEmpty(Session["cart"] as string))
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
// use mapping here
else
// do something else
add a comment |
If the Session
variable contains List<Item>
which potentially has null value, you should use as
operator and then check against null value:
var cart = Session["cart"] as List<Item>;
if (cart == null)
// do something
else
// use mapping here
However if the Session
variable contains JSON data, you can try casting as string
type and deserialize it when available:
List<Item> cart = new List<Item>();
if (!string.IsNullOrEmpty(Session["cart"] as string))
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
// use mapping here
else
// do something else
add a comment |
If the Session
variable contains List<Item>
which potentially has null value, you should use as
operator and then check against null value:
var cart = Session["cart"] as List<Item>;
if (cart == null)
// do something
else
// use mapping here
However if the Session
variable contains JSON data, you can try casting as string
type and deserialize it when available:
List<Item> cart = new List<Item>();
if (!string.IsNullOrEmpty(Session["cart"] as string))
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
// use mapping here
else
// do something else
If the Session
variable contains List<Item>
which potentially has null value, you should use as
operator and then check against null value:
var cart = Session["cart"] as List<Item>;
if (cart == null)
// do something
else
// use mapping here
However if the Session
variable contains JSON data, you can try casting as string
type and deserialize it when available:
List<Item> cart = new List<Item>();
if (!string.IsNullOrEmpty(Session["cart"] as string))
cart = JsonConvert.DeserializeObject<List<Item>>(Session["cart"].ToString());
// use mapping here
else
// do something else
answered Nov 13 '18 at 4:34
Tetsuya YamamotoTetsuya Yamamoto
14.8k42040
14.8k42040
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273580%2fhow-can-i-initialize-a-list-string-property-without-throwing-a-null-exception%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P,IYVKdayuzix3UMrDb485 DYJbKkwexGZxKGplWF,201 o71P8NNq,r8J6pD
Is this line throwing null reference/NRE:
List<Item> cart = (List<Item>)Session["cart"]
? Try putting null check against the session variable before casting toList<Item>
.– Tetsuya Yamamoto
Nov 13 '18 at 4:02
Is the
Quantity
property of your ItemDetails class astring
type ? Why ? Try to use the correct types. Use a numeric type to store numeric data.– Shyju
Nov 13 '18 at 4:05