Django DRF: Schema for bulk creation api
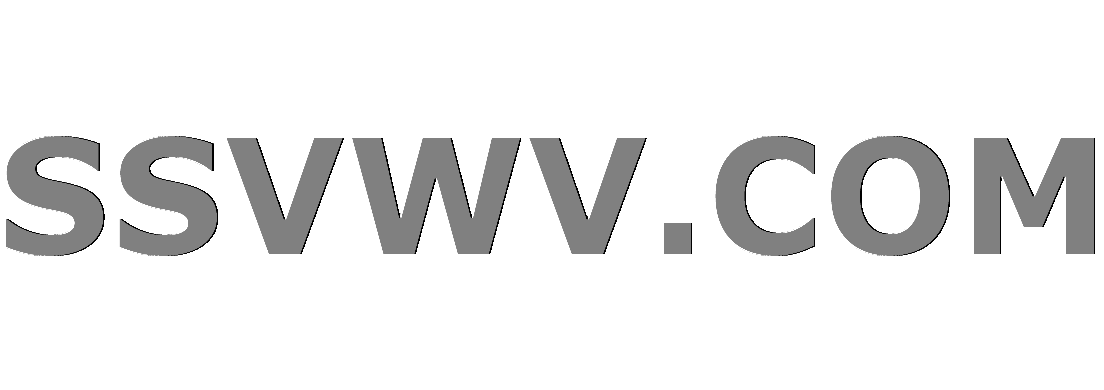
Multi tool use
I'm using django-rest-framework to build my API in which supports bulk create/update.
In these cases, the api will accept a list of object like
[
"foo":"bar",
"foo":"bar"
]
The code I'm using to allow bulk apis is just a small modification to add option many=True for serializer if the data is a list. It's like:
class FooViewSet(views.ModelViewSet):
def create(self, request, *args, **kwargs):
many = isinstance(request.data, list)
if many:
serializer = self.get_serializer(data=request.data, many=True)
serializer.is_valid(raise_exception=True)
self.perform_bulk_create(serializer)
else:
................
I'm using drf_yasg for api doc generation.
But the problem is the schema generated keep detecting my request body just the single model only. Is there any config to make DRF schema generator knows that it will accept a list type?
Here is the schema which DRF generated
"post":
"operationId": "foos_create",
"description": "",
"parameters": [
"name": "data",
"in": "body",
"required": true,
"schema":
"$ref": "#/definitions/Foo"
],
"responses":
"201":
"description": "",
"schema":
"$ref": "#/definitions/Foo"
,
"tags": [
"foos"
]
My expectation is the schema would be the array type of Foo definition
Any help will be appreciated. Thanks for your time.
django django-rest-framework
|
show 1 more comment
I'm using django-rest-framework to build my API in which supports bulk create/update.
In these cases, the api will accept a list of object like
[
"foo":"bar",
"foo":"bar"
]
The code I'm using to allow bulk apis is just a small modification to add option many=True for serializer if the data is a list. It's like:
class FooViewSet(views.ModelViewSet):
def create(self, request, *args, **kwargs):
many = isinstance(request.data, list)
if many:
serializer = self.get_serializer(data=request.data, many=True)
serializer.is_valid(raise_exception=True)
self.perform_bulk_create(serializer)
else:
................
I'm using drf_yasg for api doc generation.
But the problem is the schema generated keep detecting my request body just the single model only. Is there any config to make DRF schema generator knows that it will accept a list type?
Here is the schema which DRF generated
"post":
"operationId": "foos_create",
"description": "",
"parameters": [
"name": "data",
"in": "body",
"required": true,
"schema":
"$ref": "#/definitions/Foo"
],
"responses":
"201":
"description": "",
"schema":
"$ref": "#/definitions/Foo"
,
"tags": [
"foos"
]
My expectation is the schema would be the array type of Foo definition
Any help will be appreciated. Thanks for your time.
django django-rest-framework
What do you mean generate schema and schema generator?
– Red Cricket
Nov 13 '18 at 3:50
@RedCricket Sorry for making you confused. What I mean is the schemas which DRF generated for the API docs (I'm using Swagger)
– Tuan nguyen
Nov 13 '18 at 3:52
Ah. Can you post a screen shot of the problem?
– Red Cricket
Nov 13 '18 at 3:54
Hi @RedCricket, I updated my question with the swagger json schema has problem. Hope it would be useful.
– Tuan nguyen
Nov 13 '18 at 4:03
sorry for all the questions I am still new to Django and DRF. Are you using: github.com/miki725/django-rest-framework-bulk ?
– Red Cricket
Nov 13 '18 at 4:24
|
show 1 more comment
I'm using django-rest-framework to build my API in which supports bulk create/update.
In these cases, the api will accept a list of object like
[
"foo":"bar",
"foo":"bar"
]
The code I'm using to allow bulk apis is just a small modification to add option many=True for serializer if the data is a list. It's like:
class FooViewSet(views.ModelViewSet):
def create(self, request, *args, **kwargs):
many = isinstance(request.data, list)
if many:
serializer = self.get_serializer(data=request.data, many=True)
serializer.is_valid(raise_exception=True)
self.perform_bulk_create(serializer)
else:
................
I'm using drf_yasg for api doc generation.
But the problem is the schema generated keep detecting my request body just the single model only. Is there any config to make DRF schema generator knows that it will accept a list type?
Here is the schema which DRF generated
"post":
"operationId": "foos_create",
"description": "",
"parameters": [
"name": "data",
"in": "body",
"required": true,
"schema":
"$ref": "#/definitions/Foo"
],
"responses":
"201":
"description": "",
"schema":
"$ref": "#/definitions/Foo"
,
"tags": [
"foos"
]
My expectation is the schema would be the array type of Foo definition
Any help will be appreciated. Thanks for your time.
django django-rest-framework
I'm using django-rest-framework to build my API in which supports bulk create/update.
In these cases, the api will accept a list of object like
[
"foo":"bar",
"foo":"bar"
]
The code I'm using to allow bulk apis is just a small modification to add option many=True for serializer if the data is a list. It's like:
class FooViewSet(views.ModelViewSet):
def create(self, request, *args, **kwargs):
many = isinstance(request.data, list)
if many:
serializer = self.get_serializer(data=request.data, many=True)
serializer.is_valid(raise_exception=True)
self.perform_bulk_create(serializer)
else:
................
I'm using drf_yasg for api doc generation.
But the problem is the schema generated keep detecting my request body just the single model only. Is there any config to make DRF schema generator knows that it will accept a list type?
Here is the schema which DRF generated
"post":
"operationId": "foos_create",
"description": "",
"parameters": [
"name": "data",
"in": "body",
"required": true,
"schema":
"$ref": "#/definitions/Foo"
],
"responses":
"201":
"description": "",
"schema":
"$ref": "#/definitions/Foo"
,
"tags": [
"foos"
]
My expectation is the schema would be the array type of Foo definition
Any help will be appreciated. Thanks for your time.
django django-rest-framework
django django-rest-framework
edited Nov 13 '18 at 4:41
Tuan nguyen
asked Nov 13 '18 at 3:44


Tuan nguyenTuan nguyen
490616
490616
What do you mean generate schema and schema generator?
– Red Cricket
Nov 13 '18 at 3:50
@RedCricket Sorry for making you confused. What I mean is the schemas which DRF generated for the API docs (I'm using Swagger)
– Tuan nguyen
Nov 13 '18 at 3:52
Ah. Can you post a screen shot of the problem?
– Red Cricket
Nov 13 '18 at 3:54
Hi @RedCricket, I updated my question with the swagger json schema has problem. Hope it would be useful.
– Tuan nguyen
Nov 13 '18 at 4:03
sorry for all the questions I am still new to Django and DRF. Are you using: github.com/miki725/django-rest-framework-bulk ?
– Red Cricket
Nov 13 '18 at 4:24
|
show 1 more comment
What do you mean generate schema and schema generator?
– Red Cricket
Nov 13 '18 at 3:50
@RedCricket Sorry for making you confused. What I mean is the schemas which DRF generated for the API docs (I'm using Swagger)
– Tuan nguyen
Nov 13 '18 at 3:52
Ah. Can you post a screen shot of the problem?
– Red Cricket
Nov 13 '18 at 3:54
Hi @RedCricket, I updated my question with the swagger json schema has problem. Hope it would be useful.
– Tuan nguyen
Nov 13 '18 at 4:03
sorry for all the questions I am still new to Django and DRF. Are you using: github.com/miki725/django-rest-framework-bulk ?
– Red Cricket
Nov 13 '18 at 4:24
What do you mean generate schema and schema generator?
– Red Cricket
Nov 13 '18 at 3:50
What do you mean generate schema and schema generator?
– Red Cricket
Nov 13 '18 at 3:50
@RedCricket Sorry for making you confused. What I mean is the schemas which DRF generated for the API docs (I'm using Swagger)
– Tuan nguyen
Nov 13 '18 at 3:52
@RedCricket Sorry for making you confused. What I mean is the schemas which DRF generated for the API docs (I'm using Swagger)
– Tuan nguyen
Nov 13 '18 at 3:52
Ah. Can you post a screen shot of the problem?
– Red Cricket
Nov 13 '18 at 3:54
Ah. Can you post a screen shot of the problem?
– Red Cricket
Nov 13 '18 at 3:54
Hi @RedCricket, I updated my question with the swagger json schema has problem. Hope it would be useful.
– Tuan nguyen
Nov 13 '18 at 4:03
Hi @RedCricket, I updated my question with the swagger json schema has problem. Hope it would be useful.
– Tuan nguyen
Nov 13 '18 at 4:03
sorry for all the questions I am still new to Django and DRF. Are you using: github.com/miki725/django-rest-framework-bulk ?
– Red Cricket
Nov 13 '18 at 4:24
sorry for all the questions I am still new to Django and DRF. Are you using: github.com/miki725/django-rest-framework-bulk ?
– Red Cricket
Nov 13 '18 at 4:24
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273478%2fdjango-drf-schema-for-bulk-creation-api%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273478%2fdjango-drf-schema-for-bulk-creation-api%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ClvSb,gNA,PnzbvQihLD VMNQ13HWXWcXC6 CJs0mKCw 478tbc3FGFhO4dKnbTeOxSqaOkG,cGJOzSlySpcWRjviyON8ml
What do you mean generate schema and schema generator?
– Red Cricket
Nov 13 '18 at 3:50
@RedCricket Sorry for making you confused. What I mean is the schemas which DRF generated for the API docs (I'm using Swagger)
– Tuan nguyen
Nov 13 '18 at 3:52
Ah. Can you post a screen shot of the problem?
– Red Cricket
Nov 13 '18 at 3:54
Hi @RedCricket, I updated my question with the swagger json schema has problem. Hope it would be useful.
– Tuan nguyen
Nov 13 '18 at 4:03
sorry for all the questions I am still new to Django and DRF. Are you using: github.com/miki725/django-rest-framework-bulk ?
– Red Cricket
Nov 13 '18 at 4:24