setState is not updating DOM
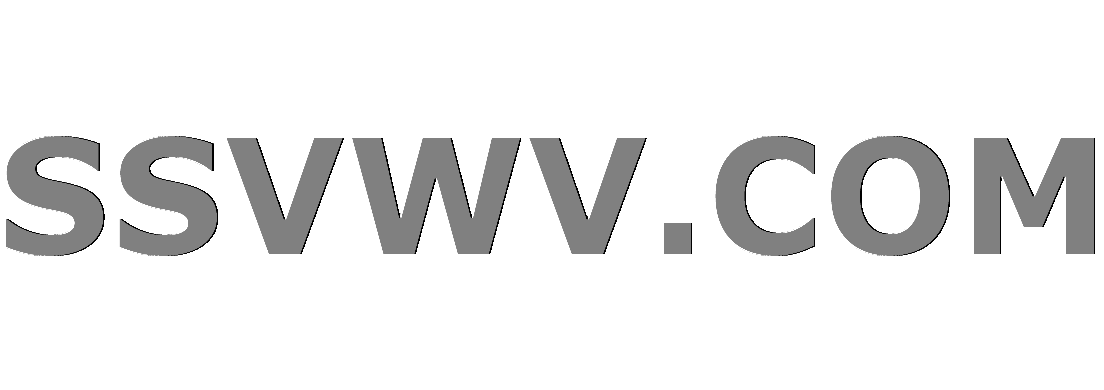
Multi tool use
up vote
1
down vote
favorite
I have this component in my react app. This basically update the state after every second. But The value <p>this.state.time</p>
is not changing. I print the value of state it is changing after every second.
import React from 'react'
import './style.css'
class List extends React.Component
constructor(props)
super(props)
this.state =
time:420
this.handleCountdown = this.handleCountdown.bind(this)
handleCountdown()
console.log('4')
console.log(this.state)
setInterval(()=>
this.setState(time:this.state.time-1)
console.log('here')
console.log(this.state)
,1000)
shouldComponentUpdate(nextProps, nextState)
console.log('1')
if(this.props.start != nextProps.start)
console.log('in')
if(nextProps.start == false)
this.setState(time:420)
console.log('2')
return true
else if(nextProps.start == true)
this.handleCountdown()
console.log('3')
return true
else
return false
render()
console.log(this.state)
return(
<div className="test-clock">
<p>this.state.time</p>
<p className='test-clock-subheading'>this.props.start=='true'?'Min remaining':'Start sudo contest'</p>
</div>
)
export default List
It is child component. I import it to parent and then use it.
Can any body tell why DOM is not updating?
this.forceUpdate() will update the DOM. But why this.setState() is not
updating DOM?
javascript reactjs
add a comment |
up vote
1
down vote
favorite
I have this component in my react app. This basically update the state after every second. But The value <p>this.state.time</p>
is not changing. I print the value of state it is changing after every second.
import React from 'react'
import './style.css'
class List extends React.Component
constructor(props)
super(props)
this.state =
time:420
this.handleCountdown = this.handleCountdown.bind(this)
handleCountdown()
console.log('4')
console.log(this.state)
setInterval(()=>
this.setState(time:this.state.time-1)
console.log('here')
console.log(this.state)
,1000)
shouldComponentUpdate(nextProps, nextState)
console.log('1')
if(this.props.start != nextProps.start)
console.log('in')
if(nextProps.start == false)
this.setState(time:420)
console.log('2')
return true
else if(nextProps.start == true)
this.handleCountdown()
console.log('3')
return true
else
return false
render()
console.log(this.state)
return(
<div className="test-clock">
<p>this.state.time</p>
<p className='test-clock-subheading'>this.props.start=='true'?'Min remaining':'Start sudo contest'</p>
</div>
)
export default List
It is child component. I import it to parent and then use it.
Can any body tell why DOM is not updating?
this.forceUpdate() will update the DOM. But why this.setState() is not
updating DOM?
javascript reactjs
1
This is the reason why you should use the getDerivedStateFromProps lifecycle method.
– Donny Verduijn
21 hours ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have this component in my react app. This basically update the state after every second. But The value <p>this.state.time</p>
is not changing. I print the value of state it is changing after every second.
import React from 'react'
import './style.css'
class List extends React.Component
constructor(props)
super(props)
this.state =
time:420
this.handleCountdown = this.handleCountdown.bind(this)
handleCountdown()
console.log('4')
console.log(this.state)
setInterval(()=>
this.setState(time:this.state.time-1)
console.log('here')
console.log(this.state)
,1000)
shouldComponentUpdate(nextProps, nextState)
console.log('1')
if(this.props.start != nextProps.start)
console.log('in')
if(nextProps.start == false)
this.setState(time:420)
console.log('2')
return true
else if(nextProps.start == true)
this.handleCountdown()
console.log('3')
return true
else
return false
render()
console.log(this.state)
return(
<div className="test-clock">
<p>this.state.time</p>
<p className='test-clock-subheading'>this.props.start=='true'?'Min remaining':'Start sudo contest'</p>
</div>
)
export default List
It is child component. I import it to parent and then use it.
Can any body tell why DOM is not updating?
this.forceUpdate() will update the DOM. But why this.setState() is not
updating DOM?
javascript reactjs
I have this component in my react app. This basically update the state after every second. But The value <p>this.state.time</p>
is not changing. I print the value of state it is changing after every second.
import React from 'react'
import './style.css'
class List extends React.Component
constructor(props)
super(props)
this.state =
time:420
this.handleCountdown = this.handleCountdown.bind(this)
handleCountdown()
console.log('4')
console.log(this.state)
setInterval(()=>
this.setState(time:this.state.time-1)
console.log('here')
console.log(this.state)
,1000)
shouldComponentUpdate(nextProps, nextState)
console.log('1')
if(this.props.start != nextProps.start)
console.log('in')
if(nextProps.start == false)
this.setState(time:420)
console.log('2')
return true
else if(nextProps.start == true)
this.handleCountdown()
console.log('3')
return true
else
return false
render()
console.log(this.state)
return(
<div className="test-clock">
<p>this.state.time</p>
<p className='test-clock-subheading'>this.props.start=='true'?'Min remaining':'Start sudo contest'</p>
</div>
)
export default List
It is child component. I import it to parent and then use it.
Can any body tell why DOM is not updating?
this.forceUpdate() will update the DOM. But why this.setState() is not
updating DOM?
javascript reactjs
javascript reactjs
asked 22 hours ago
Rajan Lagah
554313
554313
1
This is the reason why you should use the getDerivedStateFromProps lifecycle method.
– Donny Verduijn
21 hours ago
add a comment |
1
This is the reason why you should use the getDerivedStateFromProps lifecycle method.
– Donny Verduijn
21 hours ago
1
1
This is the reason why you should use the getDerivedStateFromProps lifecycle method.
– Donny Verduijn
21 hours ago
This is the reason why you should use the getDerivedStateFromProps lifecycle method.
– Donny Verduijn
21 hours ago
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You also need to check if your state has changed. Add the following to your shouldComponentUpdate
method.
}
else if ( this.state.time !== nextState.time
return true;
As mentioned in the documentation:
Use
shouldComponentUpdate()
to let React know if a component’s output
is not affected by the current change in state or props.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You also need to check if your state has changed. Add the following to your shouldComponentUpdate
method.
}
else if ( this.state.time !== nextState.time
return true;
As mentioned in the documentation:
Use
shouldComponentUpdate()
to let React know if a component’s output
is not affected by the current change in state or props.
add a comment |
up vote
2
down vote
accepted
You also need to check if your state has changed. Add the following to your shouldComponentUpdate
method.
}
else if ( this.state.time !== nextState.time
return true;
As mentioned in the documentation:
Use
shouldComponentUpdate()
to let React know if a component’s output
is not affected by the current change in state or props.
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You also need to check if your state has changed. Add the following to your shouldComponentUpdate
method.
}
else if ( this.state.time !== nextState.time
return true;
As mentioned in the documentation:
Use
shouldComponentUpdate()
to let React know if a component’s output
is not affected by the current change in state or props.
You also need to check if your state has changed. Add the following to your shouldComponentUpdate
method.
}
else if ( this.state.time !== nextState.time
return true;
As mentioned in the documentation:
Use
shouldComponentUpdate()
to let React know if a component’s output
is not affected by the current change in state or props.
answered 21 hours ago
lumio
4,31622035
4,31622035
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237191%2fsetstate-is-not-updating-dom%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
7ztBzBP 4IcsTB7jJ1cfXV45wx0ViqUiBQjjZ83enIj HGAk3JUbX eVX4 2 QPmLx,y0
1
This is the reason why you should use the getDerivedStateFromProps lifecycle method.
– Donny Verduijn
21 hours ago