How to map multiple source members so that propertyMap.SourceMembers yields these multiple source members?
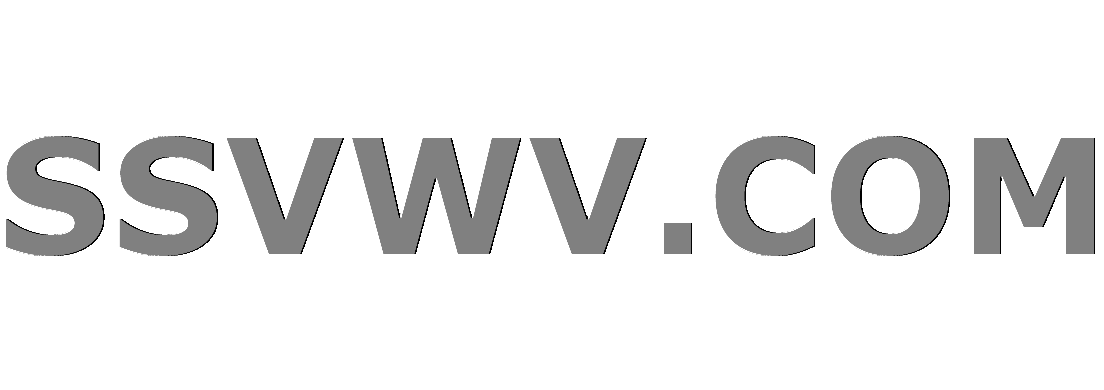
Multi tool use
up vote
1
down vote
favorite
I want to reuse the intialized mappings of the c# Automapper (using version 6.2.2) by looping through all mapped properties.
Let's suppose I have the following:
public class Person
public int Id get; set;
public string FirstName get; set;
public string LastName get; set;
public class PersonDto
public int Id get; set;
public string FullName get; set;
AutoMapper.Mapper.Initialize(cfg =>
cfg.CreateMap<Person, PersonDto>()
.ForMember(dest => dest.FullName,
opt => opt.MapFrom(src => src.FirstName + " " + src.LastName));
;
The loop for Id
behaves as expected:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "Id"
var sourceMember = propertyMap.SourceMember.Name; // = "Id"
var sourceMembers = propertyMap.SourceMembers; // Count = 1
But when I loop through the FullName
property mapping, I want to achieve that the propertyMap.SourceMembers results in the two SourceMembers FirstName
and LastName
:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers; // Count = 0 (want to achieve 2 for FirstName and LastName)
My goal is to create an automated similar mapping for an orderBy
functionality based on the initialized automapper mappings. So I need to know (a) the order and (b) the sourceMembers. In the above case I want get the mapping for FullName
from its source members FirstName
and LastName
(in this order).
Is it somehow possible to correctly register multiple source members so that propertyMap.SourceMembers
yields all mapped source members? If yes, what should the map initialization look like?
PS: I don't want to write the orderBy mappings by hand, since I already have mappings thanks to automapper.
c# automapper
add a comment |
up vote
1
down vote
favorite
I want to reuse the intialized mappings of the c# Automapper (using version 6.2.2) by looping through all mapped properties.
Let's suppose I have the following:
public class Person
public int Id get; set;
public string FirstName get; set;
public string LastName get; set;
public class PersonDto
public int Id get; set;
public string FullName get; set;
AutoMapper.Mapper.Initialize(cfg =>
cfg.CreateMap<Person, PersonDto>()
.ForMember(dest => dest.FullName,
opt => opt.MapFrom(src => src.FirstName + " " + src.LastName));
;
The loop for Id
behaves as expected:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "Id"
var sourceMember = propertyMap.SourceMember.Name; // = "Id"
var sourceMembers = propertyMap.SourceMembers; // Count = 1
But when I loop through the FullName
property mapping, I want to achieve that the propertyMap.SourceMembers results in the two SourceMembers FirstName
and LastName
:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers; // Count = 0 (want to achieve 2 for FirstName and LastName)
My goal is to create an automated similar mapping for an orderBy
functionality based on the initialized automapper mappings. So I need to know (a) the order and (b) the sourceMembers. In the above case I want get the mapping for FullName
from its source members FirstName
and LastName
(in this order).
Is it somehow possible to correctly register multiple source members so that propertyMap.SourceMembers
yields all mapped source members? If yes, what should the map initialization look like?
PS: I don't want to write the orderBy mappings by hand, since I already have mappings thanks to automapper.
c# automapper
1
github.com/AutoMapper/AutoMapper/blob/…
– Lucian Bargaoanu
Nov 10 at 11:48
I didn't find smth that does exactly what you need, but that's the idea. Have a visitor that accumulates MemberExpression-s.
– Lucian Bargaoanu
Nov 10 at 16:18
Thank you for the link Lucian Bargaoanu, I think I know where to go now :-) I'll post my answer as soon as I have a robust solution
– philipp-fx
Nov 10 at 16:28
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I want to reuse the intialized mappings of the c# Automapper (using version 6.2.2) by looping through all mapped properties.
Let's suppose I have the following:
public class Person
public int Id get; set;
public string FirstName get; set;
public string LastName get; set;
public class PersonDto
public int Id get; set;
public string FullName get; set;
AutoMapper.Mapper.Initialize(cfg =>
cfg.CreateMap<Person, PersonDto>()
.ForMember(dest => dest.FullName,
opt => opt.MapFrom(src => src.FirstName + " " + src.LastName));
;
The loop for Id
behaves as expected:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "Id"
var sourceMember = propertyMap.SourceMember.Name; // = "Id"
var sourceMembers = propertyMap.SourceMembers; // Count = 1
But when I loop through the FullName
property mapping, I want to achieve that the propertyMap.SourceMembers results in the two SourceMembers FirstName
and LastName
:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers; // Count = 0 (want to achieve 2 for FirstName and LastName)
My goal is to create an automated similar mapping for an orderBy
functionality based on the initialized automapper mappings. So I need to know (a) the order and (b) the sourceMembers. In the above case I want get the mapping for FullName
from its source members FirstName
and LastName
(in this order).
Is it somehow possible to correctly register multiple source members so that propertyMap.SourceMembers
yields all mapped source members? If yes, what should the map initialization look like?
PS: I don't want to write the orderBy mappings by hand, since I already have mappings thanks to automapper.
c# automapper
I want to reuse the intialized mappings of the c# Automapper (using version 6.2.2) by looping through all mapped properties.
Let's suppose I have the following:
public class Person
public int Id get; set;
public string FirstName get; set;
public string LastName get; set;
public class PersonDto
public int Id get; set;
public string FullName get; set;
AutoMapper.Mapper.Initialize(cfg =>
cfg.CreateMap<Person, PersonDto>()
.ForMember(dest => dest.FullName,
opt => opt.MapFrom(src => src.FirstName + " " + src.LastName));
;
The loop for Id
behaves as expected:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "Id"
var sourceMember = propertyMap.SourceMember.Name; // = "Id"
var sourceMembers = propertyMap.SourceMembers; // Count = 1
But when I loop through the FullName
property mapping, I want to achieve that the propertyMap.SourceMembers results in the two SourceMembers FirstName
and LastName
:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.GetPropertyMaps())
var destProp = propertyMap.DestinationProperty.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers; // Count = 0 (want to achieve 2 for FirstName and LastName)
My goal is to create an automated similar mapping for an orderBy
functionality based on the initialized automapper mappings. So I need to know (a) the order and (b) the sourceMembers. In the above case I want get the mapping for FullName
from its source members FirstName
and LastName
(in this order).
Is it somehow possible to correctly register multiple source members so that propertyMap.SourceMembers
yields all mapped source members? If yes, what should the map initialization look like?
PS: I don't want to write the orderBy mappings by hand, since I already have mappings thanks to automapper.
c# automapper
c# automapper
asked Nov 10 at 11:37
philipp-fx
71111
71111
1
github.com/AutoMapper/AutoMapper/blob/…
– Lucian Bargaoanu
Nov 10 at 11:48
I didn't find smth that does exactly what you need, but that's the idea. Have a visitor that accumulates MemberExpression-s.
– Lucian Bargaoanu
Nov 10 at 16:18
Thank you for the link Lucian Bargaoanu, I think I know where to go now :-) I'll post my answer as soon as I have a robust solution
– philipp-fx
Nov 10 at 16:28
add a comment |
1
github.com/AutoMapper/AutoMapper/blob/…
– Lucian Bargaoanu
Nov 10 at 11:48
I didn't find smth that does exactly what you need, but that's the idea. Have a visitor that accumulates MemberExpression-s.
– Lucian Bargaoanu
Nov 10 at 16:18
Thank you for the link Lucian Bargaoanu, I think I know where to go now :-) I'll post my answer as soon as I have a robust solution
– philipp-fx
Nov 10 at 16:28
1
1
github.com/AutoMapper/AutoMapper/blob/…
– Lucian Bargaoanu
Nov 10 at 11:48
github.com/AutoMapper/AutoMapper/blob/…
– Lucian Bargaoanu
Nov 10 at 11:48
I didn't find smth that does exactly what you need, but that's the idea. Have a visitor that accumulates MemberExpression-s.
– Lucian Bargaoanu
Nov 10 at 16:18
I didn't find smth that does exactly what you need, but that's the idea. Have a visitor that accumulates MemberExpression-s.
– Lucian Bargaoanu
Nov 10 at 16:18
Thank you for the link Lucian Bargaoanu, I think I know where to go now :-) I'll post my answer as soon as I have a robust solution
– philipp-fx
Nov 10 at 16:28
Thank you for the link Lucian Bargaoanu, I think I know where to go now :-) I'll post my answer as soon as I have a robust solution
– philipp-fx
Nov 10 at 16:28
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Thanks to Lucian Bargaoanu, I developed the following dirty workaround.
Please note that this is the first time that I get in touch with interpreting expressions, so bear with me the quality of my solution:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.PropertyMaps)
var destProp = propertyMap.DestinationMember.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember?.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers.ToList();
if (sourceMembers.Count() == 0)
ResolveSourceMembersInOrder(sourceMembers,
propertyMap.CustomMapExpression.Body as BinaryExpression);
// sourceMembers now yield the two desired sourceMembers "FirstName" and "LastName"
public static void ResolveSourceMembersInOrder(List<MemberInfo> memberInfos, BinaryExpression expression)
if (memberInfos == null)
memberInfos = new List<MemberInfo>();
if (expression == null)
return;
var left = expression.Left;
if (left.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(left).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, left as BinaryExpression);
var right = expression.Right;
if (right.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(right).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, right as BinaryExpression);
1
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Thanks to Lucian Bargaoanu, I developed the following dirty workaround.
Please note that this is the first time that I get in touch with interpreting expressions, so bear with me the quality of my solution:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.PropertyMaps)
var destProp = propertyMap.DestinationMember.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember?.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers.ToList();
if (sourceMembers.Count() == 0)
ResolveSourceMembersInOrder(sourceMembers,
propertyMap.CustomMapExpression.Body as BinaryExpression);
// sourceMembers now yield the two desired sourceMembers "FirstName" and "LastName"
public static void ResolveSourceMembersInOrder(List<MemberInfo> memberInfos, BinaryExpression expression)
if (memberInfos == null)
memberInfos = new List<MemberInfo>();
if (expression == null)
return;
var left = expression.Left;
if (left.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(left).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, left as BinaryExpression);
var right = expression.Right;
if (right.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(right).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, right as BinaryExpression);
1
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
add a comment |
up vote
0
down vote
Thanks to Lucian Bargaoanu, I developed the following dirty workaround.
Please note that this is the first time that I get in touch with interpreting expressions, so bear with me the quality of my solution:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.PropertyMaps)
var destProp = propertyMap.DestinationMember.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember?.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers.ToList();
if (sourceMembers.Count() == 0)
ResolveSourceMembersInOrder(sourceMembers,
propertyMap.CustomMapExpression.Body as BinaryExpression);
// sourceMembers now yield the two desired sourceMembers "FirstName" and "LastName"
public static void ResolveSourceMembersInOrder(List<MemberInfo> memberInfos, BinaryExpression expression)
if (memberInfos == null)
memberInfos = new List<MemberInfo>();
if (expression == null)
return;
var left = expression.Left;
if (left.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(left).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, left as BinaryExpression);
var right = expression.Right;
if (right.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(right).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, right as BinaryExpression);
1
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
add a comment |
up vote
0
down vote
up vote
0
down vote
Thanks to Lucian Bargaoanu, I developed the following dirty workaround.
Please note that this is the first time that I get in touch with interpreting expressions, so bear with me the quality of my solution:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.PropertyMaps)
var destProp = propertyMap.DestinationMember.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember?.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers.ToList();
if (sourceMembers.Count() == 0)
ResolveSourceMembersInOrder(sourceMembers,
propertyMap.CustomMapExpression.Body as BinaryExpression);
// sourceMembers now yield the two desired sourceMembers "FirstName" and "LastName"
public static void ResolveSourceMembersInOrder(List<MemberInfo> memberInfos, BinaryExpression expression)
if (memberInfos == null)
memberInfos = new List<MemberInfo>();
if (expression == null)
return;
var left = expression.Left;
if (left.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(left).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, left as BinaryExpression);
var right = expression.Right;
if (right.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(right).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, right as BinaryExpression);
Thanks to Lucian Bargaoanu, I developed the following dirty workaround.
Please note that this is the first time that I get in touch with interpreting expressions, so bear with me the quality of my solution:
var map = AutoMapper.Mapper.Configuration.FindTypeMapFor<Person, PersonDto>();
foreach (var propertyMap in map.PropertyMaps)
var destProp = propertyMap.DestinationMember.Name; // = "FullName"
var sourceMember = propertyMap.SourceMember?.Name; // = "LastName" (I don't care)
var sourceMembers = propertyMap.SourceMembers.ToList();
if (sourceMembers.Count() == 0)
ResolveSourceMembersInOrder(sourceMembers,
propertyMap.CustomMapExpression.Body as BinaryExpression);
// sourceMembers now yield the two desired sourceMembers "FirstName" and "LastName"
public static void ResolveSourceMembersInOrder(List<MemberInfo> memberInfos, BinaryExpression expression)
if (memberInfos == null)
memberInfos = new List<MemberInfo>();
if (expression == null)
return;
var left = expression.Left;
if (left.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(left).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, left as BinaryExpression);
var right = expression.Right;
if (right.NodeType == ExpressionType.MemberAccess)
memberInfos.Add(MemberVisitor.GetMemberPath(right).FirstOrDefault());
else
ResolveSourceMembersInOrder(memberInfos, right as BinaryExpression);
answered Nov 10 at 17:26
philipp-fx
71111
71111
1
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
add a comment |
1
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
1
1
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
See this. It returns things in the right order, but a few tests wouldn't hurt :)
– Lucian Bargaoanu
Nov 11 at 9:50
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238544%2fhow-to-map-multiple-source-members-so-that-propertymap-sourcemembers-yields-thes%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
dF9UXHcK20BYC4W1,SAMoY1 D,HOUUSg sFQP VRgaC4v1 a,RwL
1
github.com/AutoMapper/AutoMapper/blob/…
– Lucian Bargaoanu
Nov 10 at 11:48
I didn't find smth that does exactly what you need, but that's the idea. Have a visitor that accumulates MemberExpression-s.
– Lucian Bargaoanu
Nov 10 at 16:18
Thank you for the link Lucian Bargaoanu, I think I know where to go now :-) I'll post my answer as soon as I have a robust solution
– philipp-fx
Nov 10 at 16:28