How can I check collision between two controls created from different classes(In multiple class instances)
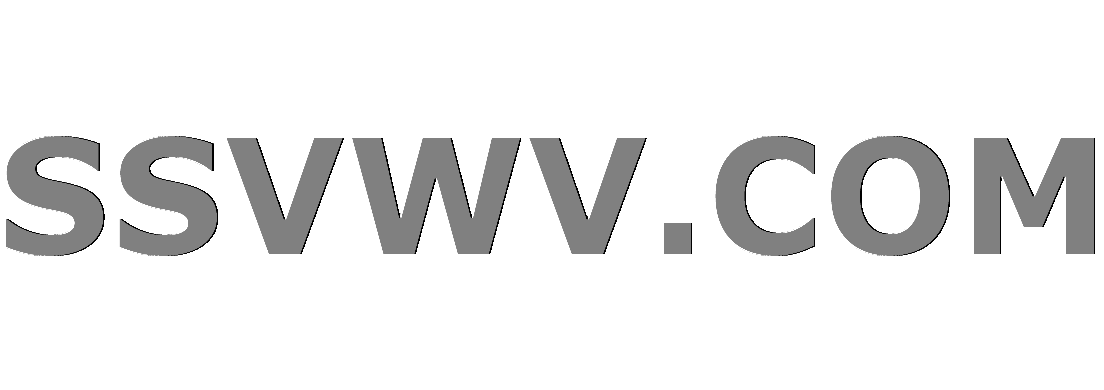
Multi tool use
up vote
0
down vote
favorite
I'm making a simple game in C#
where you have to shoot to kill enemies(both are pictureboxs
(the shot and the enemy)).
I have a class separated just for the shot creation and handling and another for the enemy.
In my enemy class
, I have 2 methods
(one dedicated to create the control itself) and another just to call it(spawn the enemy)
The problem with this code I have, It will only collides with the first class(monster) created, and not with the 3 that I have spawn in my form with a for loop.
public static PictureBox Monstro_P; //Static picturebox variable that is the enemy control
public void spawn_Monstro(Form form, int _X, int _Y) //Method that spawns the monster
Monstro_S(form, _X, _Y);
public void Monstro_S(Form form, int _X, int _Y)//Method that creates the control
Monstro_P = new PictureBox()
BackColor = Color.Chocolate,
Size = new Size(20, 35),
Left = _X,
Top = _Y,
Tag = "Monstro"
;
aa.Interval = 10;
aa.Tick += new EventHandler(aa_Timer);
aa.Start();
form.Controls.Add(Monstro_P);
Monstro_PB = new ProgressBar() //Represents the health bar of the enemy
Width = Monstro_P.Width,
Height = 10,
Location = Monstro_P.Location,
Maximum = vida,
Value = vida,
;
Monstro_PB.Top -= 15;
form.Controls.Add(Monstro_PB);
In my shot class(That is going to check if both of those collided or not)
public PictureBox Tiro_P; //Public variable that is the shot control
public void Spawn_Tiro(Form form, int _X, int _Y)//Method that spawns te shot
Tiro_C(form, _X, _Y);
public void Tiro_C(Form form, int _X, int _Y)//Method that creates the control
Tiro_P = new PictureBox()
Left = _X,
Top = _Y,
Size = new Size(10, 8),
BackColor = Color.Crimson,
Tag = "Tiro"
;
Tiro_P.LocationChanged += new EventHandler(Tiro_Localizao); //PictureBox LocationChanged event to check when it hits
Tiro_T.Interval = 10;
Tiro_T.Start();
Tiro_T.Tick += new EventHandler(Tiro_Timer);
form.Controls.Add(Tiro_P);
void Tiro_Timer(object sender, EventArgs e)//Timer for the class
Tiro_P.Left += 5; //Moves the picturebox(shot in this case)
void Tiro_Localizao(object sender, EventArgs e)//LocationChanged event
if (Monstro.Monstro_P.Bounds.IntersectsWith(Tiro_P.Bounds))
MessageBox.Show("you got hit!");
//if the static variable(monster picturebox) collides with the shot control
Form1 Load Code where I'm spawning the Monster Class:
private void Form1_Load(object sender, EventArgs e)
for (int a = 0; a < 3; a++) //Spawns a monster 3 times in random locations
var x = rnd.Next(50, 550);
var y = rnd.Next(50, 550);
m = new Monstro();
m.spawn_Monstro(this, x, y);
And my code to spawns the shot everytime I click on the form:
private void Form1_Click(object sender, EventArgs e)//Form Click Event
_Tiro = new Tiro(); //Class new instance of class
_Tiro.Spawn_Tiro(this, Cursor.Position.X, Cursor.Position.Y);
EDIT: I have put the exact same code on the timer, cause I thought the PictureBox LocationChanged was not working, but it doens't work either.
c# winforms class instance
add a comment |
up vote
0
down vote
favorite
I'm making a simple game in C#
where you have to shoot to kill enemies(both are pictureboxs
(the shot and the enemy)).
I have a class separated just for the shot creation and handling and another for the enemy.
In my enemy class
, I have 2 methods
(one dedicated to create the control itself) and another just to call it(spawn the enemy)
The problem with this code I have, It will only collides with the first class(monster) created, and not with the 3 that I have spawn in my form with a for loop.
public static PictureBox Monstro_P; //Static picturebox variable that is the enemy control
public void spawn_Monstro(Form form, int _X, int _Y) //Method that spawns the monster
Monstro_S(form, _X, _Y);
public void Monstro_S(Form form, int _X, int _Y)//Method that creates the control
Monstro_P = new PictureBox()
BackColor = Color.Chocolate,
Size = new Size(20, 35),
Left = _X,
Top = _Y,
Tag = "Monstro"
;
aa.Interval = 10;
aa.Tick += new EventHandler(aa_Timer);
aa.Start();
form.Controls.Add(Monstro_P);
Monstro_PB = new ProgressBar() //Represents the health bar of the enemy
Width = Monstro_P.Width,
Height = 10,
Location = Monstro_P.Location,
Maximum = vida,
Value = vida,
;
Monstro_PB.Top -= 15;
form.Controls.Add(Monstro_PB);
In my shot class(That is going to check if both of those collided or not)
public PictureBox Tiro_P; //Public variable that is the shot control
public void Spawn_Tiro(Form form, int _X, int _Y)//Method that spawns te shot
Tiro_C(form, _X, _Y);
public void Tiro_C(Form form, int _X, int _Y)//Method that creates the control
Tiro_P = new PictureBox()
Left = _X,
Top = _Y,
Size = new Size(10, 8),
BackColor = Color.Crimson,
Tag = "Tiro"
;
Tiro_P.LocationChanged += new EventHandler(Tiro_Localizao); //PictureBox LocationChanged event to check when it hits
Tiro_T.Interval = 10;
Tiro_T.Start();
Tiro_T.Tick += new EventHandler(Tiro_Timer);
form.Controls.Add(Tiro_P);
void Tiro_Timer(object sender, EventArgs e)//Timer for the class
Tiro_P.Left += 5; //Moves the picturebox(shot in this case)
void Tiro_Localizao(object sender, EventArgs e)//LocationChanged event
if (Monstro.Monstro_P.Bounds.IntersectsWith(Tiro_P.Bounds))
MessageBox.Show("you got hit!");
//if the static variable(monster picturebox) collides with the shot control
Form1 Load Code where I'm spawning the Monster Class:
private void Form1_Load(object sender, EventArgs e)
for (int a = 0; a < 3; a++) //Spawns a monster 3 times in random locations
var x = rnd.Next(50, 550);
var y = rnd.Next(50, 550);
m = new Monstro();
m.spawn_Monstro(this, x, y);
And my code to spawns the shot everytime I click on the form:
private void Form1_Click(object sender, EventArgs e)//Form Click Event
_Tiro = new Tiro(); //Class new instance of class
_Tiro.Spawn_Tiro(this, Cursor.Position.X, Cursor.Position.Y);
EDIT: I have put the exact same code on the timer, cause I thought the PictureBox LocationChanged was not working, but it doens't work either.
c# winforms class instance
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm making a simple game in C#
where you have to shoot to kill enemies(both are pictureboxs
(the shot and the enemy)).
I have a class separated just for the shot creation and handling and another for the enemy.
In my enemy class
, I have 2 methods
(one dedicated to create the control itself) and another just to call it(spawn the enemy)
The problem with this code I have, It will only collides with the first class(monster) created, and not with the 3 that I have spawn in my form with a for loop.
public static PictureBox Monstro_P; //Static picturebox variable that is the enemy control
public void spawn_Monstro(Form form, int _X, int _Y) //Method that spawns the monster
Monstro_S(form, _X, _Y);
public void Monstro_S(Form form, int _X, int _Y)//Method that creates the control
Monstro_P = new PictureBox()
BackColor = Color.Chocolate,
Size = new Size(20, 35),
Left = _X,
Top = _Y,
Tag = "Monstro"
;
aa.Interval = 10;
aa.Tick += new EventHandler(aa_Timer);
aa.Start();
form.Controls.Add(Monstro_P);
Monstro_PB = new ProgressBar() //Represents the health bar of the enemy
Width = Monstro_P.Width,
Height = 10,
Location = Monstro_P.Location,
Maximum = vida,
Value = vida,
;
Monstro_PB.Top -= 15;
form.Controls.Add(Monstro_PB);
In my shot class(That is going to check if both of those collided or not)
public PictureBox Tiro_P; //Public variable that is the shot control
public void Spawn_Tiro(Form form, int _X, int _Y)//Method that spawns te shot
Tiro_C(form, _X, _Y);
public void Tiro_C(Form form, int _X, int _Y)//Method that creates the control
Tiro_P = new PictureBox()
Left = _X,
Top = _Y,
Size = new Size(10, 8),
BackColor = Color.Crimson,
Tag = "Tiro"
;
Tiro_P.LocationChanged += new EventHandler(Tiro_Localizao); //PictureBox LocationChanged event to check when it hits
Tiro_T.Interval = 10;
Tiro_T.Start();
Tiro_T.Tick += new EventHandler(Tiro_Timer);
form.Controls.Add(Tiro_P);
void Tiro_Timer(object sender, EventArgs e)//Timer for the class
Tiro_P.Left += 5; //Moves the picturebox(shot in this case)
void Tiro_Localizao(object sender, EventArgs e)//LocationChanged event
if (Monstro.Monstro_P.Bounds.IntersectsWith(Tiro_P.Bounds))
MessageBox.Show("you got hit!");
//if the static variable(monster picturebox) collides with the shot control
Form1 Load Code where I'm spawning the Monster Class:
private void Form1_Load(object sender, EventArgs e)
for (int a = 0; a < 3; a++) //Spawns a monster 3 times in random locations
var x = rnd.Next(50, 550);
var y = rnd.Next(50, 550);
m = new Monstro();
m.spawn_Monstro(this, x, y);
And my code to spawns the shot everytime I click on the form:
private void Form1_Click(object sender, EventArgs e)//Form Click Event
_Tiro = new Tiro(); //Class new instance of class
_Tiro.Spawn_Tiro(this, Cursor.Position.X, Cursor.Position.Y);
EDIT: I have put the exact same code on the timer, cause I thought the PictureBox LocationChanged was not working, but it doens't work either.
c# winforms class instance
I'm making a simple game in C#
where you have to shoot to kill enemies(both are pictureboxs
(the shot and the enemy)).
I have a class separated just for the shot creation and handling and another for the enemy.
In my enemy class
, I have 2 methods
(one dedicated to create the control itself) and another just to call it(spawn the enemy)
The problem with this code I have, It will only collides with the first class(monster) created, and not with the 3 that I have spawn in my form with a for loop.
public static PictureBox Monstro_P; //Static picturebox variable that is the enemy control
public void spawn_Monstro(Form form, int _X, int _Y) //Method that spawns the monster
Monstro_S(form, _X, _Y);
public void Monstro_S(Form form, int _X, int _Y)//Method that creates the control
Monstro_P = new PictureBox()
BackColor = Color.Chocolate,
Size = new Size(20, 35),
Left = _X,
Top = _Y,
Tag = "Monstro"
;
aa.Interval = 10;
aa.Tick += new EventHandler(aa_Timer);
aa.Start();
form.Controls.Add(Monstro_P);
Monstro_PB = new ProgressBar() //Represents the health bar of the enemy
Width = Monstro_P.Width,
Height = 10,
Location = Monstro_P.Location,
Maximum = vida,
Value = vida,
;
Monstro_PB.Top -= 15;
form.Controls.Add(Monstro_PB);
In my shot class(That is going to check if both of those collided or not)
public PictureBox Tiro_P; //Public variable that is the shot control
public void Spawn_Tiro(Form form, int _X, int _Y)//Method that spawns te shot
Tiro_C(form, _X, _Y);
public void Tiro_C(Form form, int _X, int _Y)//Method that creates the control
Tiro_P = new PictureBox()
Left = _X,
Top = _Y,
Size = new Size(10, 8),
BackColor = Color.Crimson,
Tag = "Tiro"
;
Tiro_P.LocationChanged += new EventHandler(Tiro_Localizao); //PictureBox LocationChanged event to check when it hits
Tiro_T.Interval = 10;
Tiro_T.Start();
Tiro_T.Tick += new EventHandler(Tiro_Timer);
form.Controls.Add(Tiro_P);
void Tiro_Timer(object sender, EventArgs e)//Timer for the class
Tiro_P.Left += 5; //Moves the picturebox(shot in this case)
void Tiro_Localizao(object sender, EventArgs e)//LocationChanged event
if (Monstro.Monstro_P.Bounds.IntersectsWith(Tiro_P.Bounds))
MessageBox.Show("you got hit!");
//if the static variable(monster picturebox) collides with the shot control
Form1 Load Code where I'm spawning the Monster Class:
private void Form1_Load(object sender, EventArgs e)
for (int a = 0; a < 3; a++) //Spawns a monster 3 times in random locations
var x = rnd.Next(50, 550);
var y = rnd.Next(50, 550);
m = new Monstro();
m.spawn_Monstro(this, x, y);
And my code to spawns the shot everytime I click on the form:
private void Form1_Click(object sender, EventArgs e)//Form Click Event
_Tiro = new Tiro(); //Class new instance of class
_Tiro.Spawn_Tiro(this, Cursor.Position.X, Cursor.Position.Y);
EDIT: I have put the exact same code on the timer, cause I thought the PictureBox LocationChanged was not working, but it doens't work either.
c# winforms class instance
c# winforms class instance
asked Nov 10 at 12:15


Jake Peralta
13
13
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53238850%2fhow-can-i-check-collision-between-two-controls-created-from-different-classesin%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
FRl 2 5 Ryo4AJklWbZ9jetglC4RNGcYT1d1EZRb n9kkhX3 Q5X67YtEAj5xSk0H7uX,1cw,X,1Mcun,f A