Trying to write a file from an Azure WebJob
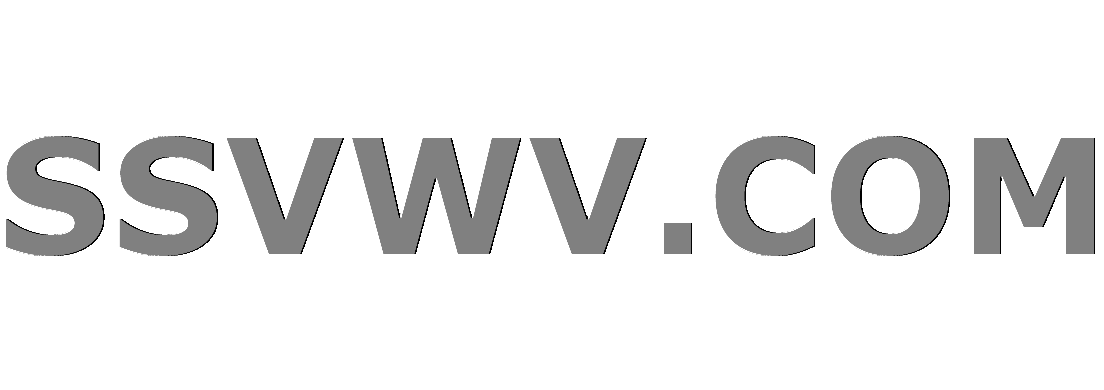
Multi tool use
My plan is to have the WebJob send the newly generated CSV to a blob storage where it's picked up by an Azure Function and emailed out to a group of recipients.
I'm using the CsvWriter library to generate the CSV. I'm told that ideally I should upload straight to the blob storage container, however I'm not sure how I'm going to do that when CsvWriter is only able to write to the filesystem using whatever TextWriter we pass to it through the constructor.
So what I'm trying to do instead is allow CsvWriter to write the file to the filesystem, and then I'll pick that up and upload it to the blob storage. The problem here is that every directory I try to get it to write to denies access to be WebJob. I have tried Environment.GetEnvironmentVariable("WEBJOBS_ROOT_PATH")
as well as %HOME%
and d:sitewwwroot
.
What directory do I need to be writing to?

add a comment |
My plan is to have the WebJob send the newly generated CSV to a blob storage where it's picked up by an Azure Function and emailed out to a group of recipients.
I'm using the CsvWriter library to generate the CSV. I'm told that ideally I should upload straight to the blob storage container, however I'm not sure how I'm going to do that when CsvWriter is only able to write to the filesystem using whatever TextWriter we pass to it through the constructor.
So what I'm trying to do instead is allow CsvWriter to write the file to the filesystem, and then I'll pick that up and upload it to the blob storage. The problem here is that every directory I try to get it to write to denies access to be WebJob. I have tried Environment.GetEnvironmentVariable("WEBJOBS_ROOT_PATH")
as well as %HOME%
and d:sitewwwroot
.
What directory do I need to be writing to?

add a comment |
My plan is to have the WebJob send the newly generated CSV to a blob storage where it's picked up by an Azure Function and emailed out to a group of recipients.
I'm using the CsvWriter library to generate the CSV. I'm told that ideally I should upload straight to the blob storage container, however I'm not sure how I'm going to do that when CsvWriter is only able to write to the filesystem using whatever TextWriter we pass to it through the constructor.
So what I'm trying to do instead is allow CsvWriter to write the file to the filesystem, and then I'll pick that up and upload it to the blob storage. The problem here is that every directory I try to get it to write to denies access to be WebJob. I have tried Environment.GetEnvironmentVariable("WEBJOBS_ROOT_PATH")
as well as %HOME%
and d:sitewwwroot
.
What directory do I need to be writing to?

My plan is to have the WebJob send the newly generated CSV to a blob storage where it's picked up by an Azure Function and emailed out to a group of recipients.
I'm using the CsvWriter library to generate the CSV. I'm told that ideally I should upload straight to the blob storage container, however I'm not sure how I'm going to do that when CsvWriter is only able to write to the filesystem using whatever TextWriter we pass to it through the constructor.
So what I'm trying to do instead is allow CsvWriter to write the file to the filesystem, and then I'll pick that up and upload it to the blob storage. The problem here is that every directory I try to get it to write to denies access to be WebJob. I have tried Environment.GetEnvironmentVariable("WEBJOBS_ROOT_PATH")
as well as %HOME%
and d:sitewwwroot
.
What directory do I need to be writing to?


edited Nov 15 '18 at 18:28
user928112
asked Nov 15 '18 at 18:10
user928112user928112
8819
8819
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can avoid it altogether by using a StringWriter
, you do not have to use the file system:
using (var stringWriter = new StringWriter())
using (var csvWriter = new CsvHelper.CsvWriter(stringWriter))
csvWriter.WriteComment("Test");
csvWriter.Flush();
var blob = container.GetBlockBlobReference("test.csv");
await blob.UploadTextAsync(stringWriter.ToString());
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325528%2ftrying-to-write-a-file-from-an-azure-webjob%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can avoid it altogether by using a StringWriter
, you do not have to use the file system:
using (var stringWriter = new StringWriter())
using (var csvWriter = new CsvHelper.CsvWriter(stringWriter))
csvWriter.WriteComment("Test");
csvWriter.Flush();
var blob = container.GetBlockBlobReference("test.csv");
await blob.UploadTextAsync(stringWriter.ToString());
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
add a comment |
You can avoid it altogether by using a StringWriter
, you do not have to use the file system:
using (var stringWriter = new StringWriter())
using (var csvWriter = new CsvHelper.CsvWriter(stringWriter))
csvWriter.WriteComment("Test");
csvWriter.Flush();
var blob = container.GetBlockBlobReference("test.csv");
await blob.UploadTextAsync(stringWriter.ToString());
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
add a comment |
You can avoid it altogether by using a StringWriter
, you do not have to use the file system:
using (var stringWriter = new StringWriter())
using (var csvWriter = new CsvHelper.CsvWriter(stringWriter))
csvWriter.WriteComment("Test");
csvWriter.Flush();
var blob = container.GetBlockBlobReference("test.csv");
await blob.UploadTextAsync(stringWriter.ToString());
You can avoid it altogether by using a StringWriter
, you do not have to use the file system:
using (var stringWriter = new StringWriter())
using (var csvWriter = new CsvHelper.CsvWriter(stringWriter))
csvWriter.WriteComment("Test");
csvWriter.Flush();
var blob = container.GetBlockBlobReference("test.csv");
await blob.UploadTextAsync(stringWriter.ToString());
answered Nov 15 '18 at 19:36


Peter BonsPeter Bons
10.2k32345
10.2k32345
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
add a comment |
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
This will work, but you’d need to account for network failures with a well thought through retry pattern.
– Josh
Nov 16 '18 at 14:33
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
@Josh that is correct, but this is the case for every scenario that involves network communication and as such, I see it as a seperate issue. Also not that the azure storage sdk has built-in retry policies
– Peter Bons
Nov 16 '18 at 14:59
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325528%2ftrying-to-write-a-file-from-an-azure-webjob%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EWuWn7FlY6jtTaE2i zspy8q5GFQxOth8yQSuw2yRYc2GbWgW50hY,9ieZbyU ZpX56ioWZpPjGjny,FUVyYVcvQzjjARx