How to perform concurrent requests with GuzzleHttp
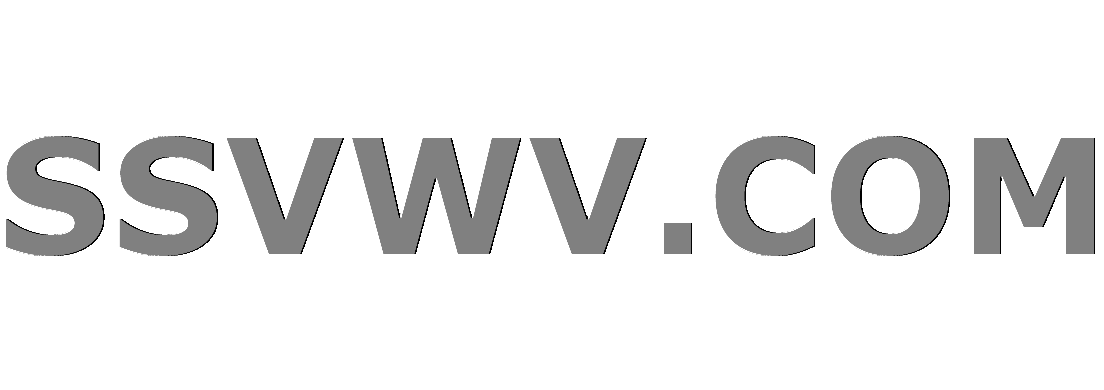
Multi tool use
How do I use Guzzle 6 to create 5 async requests with the following conditions:
- All requests start at the same time
- I want a 500ms timeout value for all requests. If a request times out I DONT want it to interrupt other requests
- If a request returns non-200 I DONT want it to interrupt other requests.
- All requests are on different domains... (so I'm not sure how that fits in with the
base_uri
setting...
If all 5 requests return 200OK < 500ms then I want to be able to loop through their responses...
BUT, if say 2 of them have non-200 and 1 of them times out (over 500ms), I want to still be able to access the responses for the 2 successful ones.
EDIT So far everything works except timeouts are still raising an exception
Here is what I had so far:
<?php
require __DIR__.'/../vendor/autoload.php';
use GuzzleHttpClient;
use GuzzleHttpPromise;
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
// initiate each request but do not block
$promises = [
'success' => $client->getAsync('https://httpbin.org/get'),
'success' => $client->getAsync('https://httpbin.org/delay/1'),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2'),
'fail500' => $client->getAsync('https://httpbin.org/status/500'),
];
// wait on all of the requests to complete. Throws a ConnectException if any
// of the requests fail
$results = Promiseunwrap($promises);
// wait for the requests to complete, even if some of them fail
$results = Promisesettle($promises)->wait();
php guzzle guzzle6
add a comment |
How do I use Guzzle 6 to create 5 async requests with the following conditions:
- All requests start at the same time
- I want a 500ms timeout value for all requests. If a request times out I DONT want it to interrupt other requests
- If a request returns non-200 I DONT want it to interrupt other requests.
- All requests are on different domains... (so I'm not sure how that fits in with the
base_uri
setting...
If all 5 requests return 200OK < 500ms then I want to be able to loop through their responses...
BUT, if say 2 of them have non-200 and 1 of them times out (over 500ms), I want to still be able to access the responses for the 2 successful ones.
EDIT So far everything works except timeouts are still raising an exception
Here is what I had so far:
<?php
require __DIR__.'/../vendor/autoload.php';
use GuzzleHttpClient;
use GuzzleHttpPromise;
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
// initiate each request but do not block
$promises = [
'success' => $client->getAsync('https://httpbin.org/get'),
'success' => $client->getAsync('https://httpbin.org/delay/1'),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2'),
'fail500' => $client->getAsync('https://httpbin.org/status/500'),
];
// wait on all of the requests to complete. Throws a ConnectException if any
// of the requests fail
$results = Promiseunwrap($promises);
// wait for the requests to complete, even if some of them fail
$results = Promisesettle($promises)->wait();
php guzzle guzzle6
Have you looked at stackoverflow answer or Guzzle doc? If the requests succeed, an array ofGuzzleHttpMessageResponse
objects are returned. "A single request failure will not cause the entire pool of requests to fail. Any exceptions thrown while transferring a pool of requests will be aggregated into aGuzzleCommonExceptionMultiTransferException
exception. "These is the comment from their doc.
– user119247
Nov 13 '18 at 0:24
Yes, I'm just trying to make it so no exception is thrown and the other requests can continue as normal. im going to update my code with the latest version i have
– Tallboy
Nov 13 '18 at 0:30
Thanks ill look at that answer, I dont use PHP often though so this is a bit of a headache.
– Tallboy
Nov 13 '18 at 0:32
So currently, if any of the 5 requests fails with 500 the entire response fails. how do i get each request to fail silently so I can just return 200 OK with the successful responses?
– Tallboy
Nov 13 '18 at 0:37
So i have everything working with'http_errors' => false
... the onyl thing left is that timeouts still raise an exception
– Tallboy
Nov 13 '18 at 0:51
add a comment |
How do I use Guzzle 6 to create 5 async requests with the following conditions:
- All requests start at the same time
- I want a 500ms timeout value for all requests. If a request times out I DONT want it to interrupt other requests
- If a request returns non-200 I DONT want it to interrupt other requests.
- All requests are on different domains... (so I'm not sure how that fits in with the
base_uri
setting...
If all 5 requests return 200OK < 500ms then I want to be able to loop through their responses...
BUT, if say 2 of them have non-200 and 1 of them times out (over 500ms), I want to still be able to access the responses for the 2 successful ones.
EDIT So far everything works except timeouts are still raising an exception
Here is what I had so far:
<?php
require __DIR__.'/../vendor/autoload.php';
use GuzzleHttpClient;
use GuzzleHttpPromise;
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
// initiate each request but do not block
$promises = [
'success' => $client->getAsync('https://httpbin.org/get'),
'success' => $client->getAsync('https://httpbin.org/delay/1'),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2'),
'fail500' => $client->getAsync('https://httpbin.org/status/500'),
];
// wait on all of the requests to complete. Throws a ConnectException if any
// of the requests fail
$results = Promiseunwrap($promises);
// wait for the requests to complete, even if some of them fail
$results = Promisesettle($promises)->wait();
php guzzle guzzle6
How do I use Guzzle 6 to create 5 async requests with the following conditions:
- All requests start at the same time
- I want a 500ms timeout value for all requests. If a request times out I DONT want it to interrupt other requests
- If a request returns non-200 I DONT want it to interrupt other requests.
- All requests are on different domains... (so I'm not sure how that fits in with the
base_uri
setting...
If all 5 requests return 200OK < 500ms then I want to be able to loop through their responses...
BUT, if say 2 of them have non-200 and 1 of them times out (over 500ms), I want to still be able to access the responses for the 2 successful ones.
EDIT So far everything works except timeouts are still raising an exception
Here is what I had so far:
<?php
require __DIR__.'/../vendor/autoload.php';
use GuzzleHttpClient;
use GuzzleHttpPromise;
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
// initiate each request but do not block
$promises = [
'success' => $client->getAsync('https://httpbin.org/get'),
'success' => $client->getAsync('https://httpbin.org/delay/1'),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2'),
'fail500' => $client->getAsync('https://httpbin.org/status/500'),
];
// wait on all of the requests to complete. Throws a ConnectException if any
// of the requests fail
$results = Promiseunwrap($promises);
// wait for the requests to complete, even if some of them fail
$results = Promisesettle($promises)->wait();
php guzzle guzzle6
php guzzle guzzle6
edited Nov 13 '18 at 5:07
user969068
73512147
73512147
asked Nov 12 '18 at 23:49
TallboyTallboy
6,3521047122
6,3521047122
Have you looked at stackoverflow answer or Guzzle doc? If the requests succeed, an array ofGuzzleHttpMessageResponse
objects are returned. "A single request failure will not cause the entire pool of requests to fail. Any exceptions thrown while transferring a pool of requests will be aggregated into aGuzzleCommonExceptionMultiTransferException
exception. "These is the comment from their doc.
– user119247
Nov 13 '18 at 0:24
Yes, I'm just trying to make it so no exception is thrown and the other requests can continue as normal. im going to update my code with the latest version i have
– Tallboy
Nov 13 '18 at 0:30
Thanks ill look at that answer, I dont use PHP often though so this is a bit of a headache.
– Tallboy
Nov 13 '18 at 0:32
So currently, if any of the 5 requests fails with 500 the entire response fails. how do i get each request to fail silently so I can just return 200 OK with the successful responses?
– Tallboy
Nov 13 '18 at 0:37
So i have everything working with'http_errors' => false
... the onyl thing left is that timeouts still raise an exception
– Tallboy
Nov 13 '18 at 0:51
add a comment |
Have you looked at stackoverflow answer or Guzzle doc? If the requests succeed, an array ofGuzzleHttpMessageResponse
objects are returned. "A single request failure will not cause the entire pool of requests to fail. Any exceptions thrown while transferring a pool of requests will be aggregated into aGuzzleCommonExceptionMultiTransferException
exception. "These is the comment from their doc.
– user119247
Nov 13 '18 at 0:24
Yes, I'm just trying to make it so no exception is thrown and the other requests can continue as normal. im going to update my code with the latest version i have
– Tallboy
Nov 13 '18 at 0:30
Thanks ill look at that answer, I dont use PHP often though so this is a bit of a headache.
– Tallboy
Nov 13 '18 at 0:32
So currently, if any of the 5 requests fails with 500 the entire response fails. how do i get each request to fail silently so I can just return 200 OK with the successful responses?
– Tallboy
Nov 13 '18 at 0:37
So i have everything working with'http_errors' => false
... the onyl thing left is that timeouts still raise an exception
– Tallboy
Nov 13 '18 at 0:51
Have you looked at stackoverflow answer or Guzzle doc? If the requests succeed, an array of
GuzzleHttpMessageResponse
objects are returned. "A single request failure will not cause the entire pool of requests to fail. Any exceptions thrown while transferring a pool of requests will be aggregated into a GuzzleCommonExceptionMultiTransferException
exception. "These is the comment from their doc.– user119247
Nov 13 '18 at 0:24
Have you looked at stackoverflow answer or Guzzle doc? If the requests succeed, an array of
GuzzleHttpMessageResponse
objects are returned. "A single request failure will not cause the entire pool of requests to fail. Any exceptions thrown while transferring a pool of requests will be aggregated into a GuzzleCommonExceptionMultiTransferException
exception. "These is the comment from their doc.– user119247
Nov 13 '18 at 0:24
Yes, I'm just trying to make it so no exception is thrown and the other requests can continue as normal. im going to update my code with the latest version i have
– Tallboy
Nov 13 '18 at 0:30
Yes, I'm just trying to make it so no exception is thrown and the other requests can continue as normal. im going to update my code with the latest version i have
– Tallboy
Nov 13 '18 at 0:30
Thanks ill look at that answer, I dont use PHP often though so this is a bit of a headache.
– Tallboy
Nov 13 '18 at 0:32
Thanks ill look at that answer, I dont use PHP often though so this is a bit of a headache.
– Tallboy
Nov 13 '18 at 0:32
So currently, if any of the 5 requests fails with 500 the entire response fails. how do i get each request to fail silently so I can just return 200 OK with the successful responses?
– Tallboy
Nov 13 '18 at 0:37
So currently, if any of the 5 requests fails with 500 the entire response fails. how do i get each request to fail silently so I can just return 200 OK with the successful responses?
– Tallboy
Nov 13 '18 at 0:37
So i have everything working with
'http_errors' => false
... the onyl thing left is that timeouts still raise an exception– Tallboy
Nov 13 '18 at 0:51
So i have everything working with
'http_errors' => false
... the onyl thing left is that timeouts still raise an exception– Tallboy
Nov 13 '18 at 0:51
add a comment |
1 Answer
1
active
oldest
votes
Guzzle provides fulfilled
and rejected
callabcks in the pool. here I performed a test by your values, read more at Guzzle docs:
$client = new Client([
'http_errors' => false,
'connect_timeout' => 0.50, //////////////// 0.50
'timeout' => 1.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$requests = function ($total)
$uris = [
'https://httpbin.org/get',
'https://httpbin.org/delay/1',
'https://httpbin.org/delay/2',
'https://httpbin.org/status/500',
];
for ($i = 0; $i < count($uris); $i++)
yield new Request('GET', $uris[$i]);
;
$pool = new Pool($client, $requests(8), [
'concurrency' => 10,
'fulfilled' => function ($response, $index)
// this is delivered each successful response
print_r($index."fulfilledn");
,
'rejected' => function ($reason, $index)
// this is delivered each failed request
print_r($index."rejectedn");
,
]);
// Initiate the transfers and create a promise
$promise = $pool->promise();
// Force the pool of requests to complete.
$promise->wait();
response
0fulfilled
3fulfilled
1rejected
2rejected
if you want to use your code above you can also pass response status in your $promises, here is an example:
use PsrHttpMessageResponseInterface;
use GuzzleHttpExceptionRequestException;
....
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$promises = [
'success' => $client->getAsync('https://httpbin.org/get')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
)
,
'success' => $client->getAsync('https://httpbin.org/delay/1')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'fail500' => $client->getAsync('https://httpbin.org/status/500')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
];
$results = Promisesettle($promises)->wait();
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrappedPromiseunwrap
with atry
and then I catch exceptions forGuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?
– Tallboy
Nov 13 '18 at 1:32
1
if you don't want to usePool
and its clearly mentioned in docs that it throwsConnectException
so it is absolutely fine to usetry
block
– user969068
Nov 13 '18 at 2:01
1
why are you accessing its properties? you can just use$response->getStatusCode()
or$response->getReasonPhrase()
to determine the response status
– user969068
Nov 13 '18 at 2:19
1
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
1
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
|
show 7 more comments
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271764%2fhow-to-perform-concurrent-requests-with-guzzlehttp%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Guzzle provides fulfilled
and rejected
callabcks in the pool. here I performed a test by your values, read more at Guzzle docs:
$client = new Client([
'http_errors' => false,
'connect_timeout' => 0.50, //////////////// 0.50
'timeout' => 1.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$requests = function ($total)
$uris = [
'https://httpbin.org/get',
'https://httpbin.org/delay/1',
'https://httpbin.org/delay/2',
'https://httpbin.org/status/500',
];
for ($i = 0; $i < count($uris); $i++)
yield new Request('GET', $uris[$i]);
;
$pool = new Pool($client, $requests(8), [
'concurrency' => 10,
'fulfilled' => function ($response, $index)
// this is delivered each successful response
print_r($index."fulfilledn");
,
'rejected' => function ($reason, $index)
// this is delivered each failed request
print_r($index."rejectedn");
,
]);
// Initiate the transfers and create a promise
$promise = $pool->promise();
// Force the pool of requests to complete.
$promise->wait();
response
0fulfilled
3fulfilled
1rejected
2rejected
if you want to use your code above you can also pass response status in your $promises, here is an example:
use PsrHttpMessageResponseInterface;
use GuzzleHttpExceptionRequestException;
....
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$promises = [
'success' => $client->getAsync('https://httpbin.org/get')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
)
,
'success' => $client->getAsync('https://httpbin.org/delay/1')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'fail500' => $client->getAsync('https://httpbin.org/status/500')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
];
$results = Promisesettle($promises)->wait();
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrappedPromiseunwrap
with atry
and then I catch exceptions forGuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?
– Tallboy
Nov 13 '18 at 1:32
1
if you don't want to usePool
and its clearly mentioned in docs that it throwsConnectException
so it is absolutely fine to usetry
block
– user969068
Nov 13 '18 at 2:01
1
why are you accessing its properties? you can just use$response->getStatusCode()
or$response->getReasonPhrase()
to determine the response status
– user969068
Nov 13 '18 at 2:19
1
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
1
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
|
show 7 more comments
Guzzle provides fulfilled
and rejected
callabcks in the pool. here I performed a test by your values, read more at Guzzle docs:
$client = new Client([
'http_errors' => false,
'connect_timeout' => 0.50, //////////////// 0.50
'timeout' => 1.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$requests = function ($total)
$uris = [
'https://httpbin.org/get',
'https://httpbin.org/delay/1',
'https://httpbin.org/delay/2',
'https://httpbin.org/status/500',
];
for ($i = 0; $i < count($uris); $i++)
yield new Request('GET', $uris[$i]);
;
$pool = new Pool($client, $requests(8), [
'concurrency' => 10,
'fulfilled' => function ($response, $index)
// this is delivered each successful response
print_r($index."fulfilledn");
,
'rejected' => function ($reason, $index)
// this is delivered each failed request
print_r($index."rejectedn");
,
]);
// Initiate the transfers and create a promise
$promise = $pool->promise();
// Force the pool of requests to complete.
$promise->wait();
response
0fulfilled
3fulfilled
1rejected
2rejected
if you want to use your code above you can also pass response status in your $promises, here is an example:
use PsrHttpMessageResponseInterface;
use GuzzleHttpExceptionRequestException;
....
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$promises = [
'success' => $client->getAsync('https://httpbin.org/get')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
)
,
'success' => $client->getAsync('https://httpbin.org/delay/1')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'fail500' => $client->getAsync('https://httpbin.org/status/500')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
];
$results = Promisesettle($promises)->wait();
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrappedPromiseunwrap
with atry
and then I catch exceptions forGuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?
– Tallboy
Nov 13 '18 at 1:32
1
if you don't want to usePool
and its clearly mentioned in docs that it throwsConnectException
so it is absolutely fine to usetry
block
– user969068
Nov 13 '18 at 2:01
1
why are you accessing its properties? you can just use$response->getStatusCode()
or$response->getReasonPhrase()
to determine the response status
– user969068
Nov 13 '18 at 2:19
1
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
1
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
|
show 7 more comments
Guzzle provides fulfilled
and rejected
callabcks in the pool. here I performed a test by your values, read more at Guzzle docs:
$client = new Client([
'http_errors' => false,
'connect_timeout' => 0.50, //////////////// 0.50
'timeout' => 1.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$requests = function ($total)
$uris = [
'https://httpbin.org/get',
'https://httpbin.org/delay/1',
'https://httpbin.org/delay/2',
'https://httpbin.org/status/500',
];
for ($i = 0; $i < count($uris); $i++)
yield new Request('GET', $uris[$i]);
;
$pool = new Pool($client, $requests(8), [
'concurrency' => 10,
'fulfilled' => function ($response, $index)
// this is delivered each successful response
print_r($index."fulfilledn");
,
'rejected' => function ($reason, $index)
// this is delivered each failed request
print_r($index."rejectedn");
,
]);
// Initiate the transfers and create a promise
$promise = $pool->promise();
// Force the pool of requests to complete.
$promise->wait();
response
0fulfilled
3fulfilled
1rejected
2rejected
if you want to use your code above you can also pass response status in your $promises, here is an example:
use PsrHttpMessageResponseInterface;
use GuzzleHttpExceptionRequestException;
....
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$promises = [
'success' => $client->getAsync('https://httpbin.org/get')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
)
,
'success' => $client->getAsync('https://httpbin.org/delay/1')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'fail500' => $client->getAsync('https://httpbin.org/status/500')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
];
$results = Promisesettle($promises)->wait();
Guzzle provides fulfilled
and rejected
callabcks in the pool. here I performed a test by your values, read more at Guzzle docs:
$client = new Client([
'http_errors' => false,
'connect_timeout' => 0.50, //////////////// 0.50
'timeout' => 1.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$requests = function ($total)
$uris = [
'https://httpbin.org/get',
'https://httpbin.org/delay/1',
'https://httpbin.org/delay/2',
'https://httpbin.org/status/500',
];
for ($i = 0; $i < count($uris); $i++)
yield new Request('GET', $uris[$i]);
;
$pool = new Pool($client, $requests(8), [
'concurrency' => 10,
'fulfilled' => function ($response, $index)
// this is delivered each successful response
print_r($index."fulfilledn");
,
'rejected' => function ($reason, $index)
// this is delivered each failed request
print_r($index."rejectedn");
,
]);
// Initiate the transfers and create a promise
$promise = $pool->promise();
// Force the pool of requests to complete.
$promise->wait();
response
0fulfilled
3fulfilled
1rejected
2rejected
if you want to use your code above you can also pass response status in your $promises, here is an example:
use PsrHttpMessageResponseInterface;
use GuzzleHttpExceptionRequestException;
....
$client = new Client([
'http_errors' => false,
'connect_timeout' => 1.50, //////////////// 0.50
'timeout' => 2.00, //////////////// 1.00
'headers' => [
'User-Agent' => 'Test/1.0'
]
]);
$promises = [
'success' => $client->getAsync('https://httpbin.org/get')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
)
,
'success' => $client->getAsync('https://httpbin.org/delay/1')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'failconnecttimeout' => $client->getAsync('https://httpbin.org/delay/2')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
'fail500' => $client->getAsync('https://httpbin.org/status/500')->then(
function (ResponseInterface $res)
echo $res->getStatusCode() . "n";
,
function (RequestException $e)
echo $e->getMessage() . "n";
echo $e->getRequest()->getMethod();
),
];
$results = Promisesettle($promises)->wait();
edited Nov 13 '18 at 2:49
answered Nov 13 '18 at 1:29
user969068user969068
73512147
73512147
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrappedPromiseunwrap
with atry
and then I catch exceptions forGuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?
– Tallboy
Nov 13 '18 at 1:32
1
if you don't want to usePool
and its clearly mentioned in docs that it throwsConnectException
so it is absolutely fine to usetry
block
– user969068
Nov 13 '18 at 2:01
1
why are you accessing its properties? you can just use$response->getStatusCode()
or$response->getReasonPhrase()
to determine the response status
– user969068
Nov 13 '18 at 2:19
1
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
1
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
|
show 7 more comments
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrappedPromiseunwrap
with atry
and then I catch exceptions forGuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?
– Tallboy
Nov 13 '18 at 1:32
1
if you don't want to usePool
and its clearly mentioned in docs that it throwsConnectException
so it is absolutely fine to usetry
block
– user969068
Nov 13 '18 at 2:01
1
why are you accessing its properties? you can just use$response->getStatusCode()
or$response->getReasonPhrase()
to determine the response status
– user969068
Nov 13 '18 at 2:19
1
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
1
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrapped
Promiseunwrap
with a try
and then I catch exceptions for GuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?– Tallboy
Nov 13 '18 at 1:32
Wow this is great. I will make this answered, but before I do... while I was waiting for answers I simply did this... I wrapped
Promiseunwrap
with a try
and then I catch exceptions for GuzzleHttpExceptionConnectException
... is there any downside to doing it this way vs your way (which is a lot more complex)?– Tallboy
Nov 13 '18 at 1:32
1
1
if you don't want to use
Pool
and its clearly mentioned in docs that it throws ConnectException
so it is absolutely fine to use try
block– user969068
Nov 13 '18 at 2:01
if you don't want to use
Pool
and its clearly mentioned in docs that it throws ConnectException
so it is absolutely fine to use try
block– user969068
Nov 13 '18 at 2:01
1
1
why are you accessing its properties? you can just use
$response->getStatusCode()
or $response->getReasonPhrase()
to determine the response status– user969068
Nov 13 '18 at 2:19
why are you accessing its properties? you can just use
$response->getStatusCode()
or $response->getReasonPhrase()
to determine the response status– user969068
Nov 13 '18 at 2:19
1
1
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
see my updated answer for another way to get your response, I will try to avoid using properties that way.
– user969068
Nov 13 '18 at 2:51
1
1
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
ok....also a big issue on your code, you are using settle and unwrap together? you should only use one method, updated pastebin.com/2bczhK6R
– user969068
Nov 13 '18 at 3:09
|
show 7 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271764%2fhow-to-perform-concurrent-requests-with-guzzlehttp%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IKfVTJJutT2Lp RegkXd,LeUgYkiq9IA73nIt,LZLb47Ke66bITGouh,d9NBdGge15sT LRyaEIdbOyjcpOP
Have you looked at stackoverflow answer or Guzzle doc? If the requests succeed, an array of
GuzzleHttpMessageResponse
objects are returned. "A single request failure will not cause the entire pool of requests to fail. Any exceptions thrown while transferring a pool of requests will be aggregated into aGuzzleCommonExceptionMultiTransferException
exception. "These is the comment from their doc.– user119247
Nov 13 '18 at 0:24
Yes, I'm just trying to make it so no exception is thrown and the other requests can continue as normal. im going to update my code with the latest version i have
– Tallboy
Nov 13 '18 at 0:30
Thanks ill look at that answer, I dont use PHP often though so this is a bit of a headache.
– Tallboy
Nov 13 '18 at 0:32
So currently, if any of the 5 requests fails with 500 the entire response fails. how do i get each request to fail silently so I can just return 200 OK with the successful responses?
– Tallboy
Nov 13 '18 at 0:37
So i have everything working with
'http_errors' => false
... the onyl thing left is that timeouts still raise an exception– Tallboy
Nov 13 '18 at 0:51