How to group List of Lists using a certain criteria in Java 8
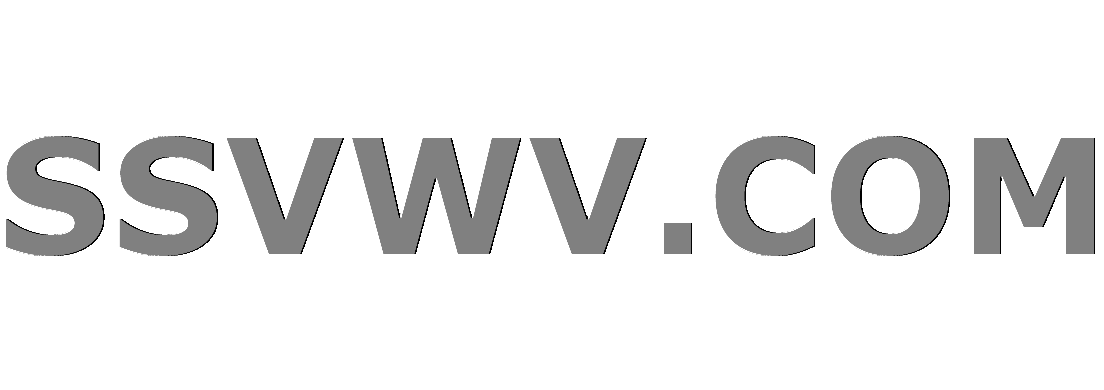
Multi tool use
up vote
3
down vote
favorite
I have a data structure like below. I'm trying to group the objects in such a way like Map<String, List<String>>
where key is the entryId and value is the List of groups it belongs to. entryId is always unique inside a group.
Example: entryId "1111" belongs to group1,group2,group3. I'm using the old java 7 way to iterate through the lists and checking. Is there any best possible way using Java8 Collectors/grouping to achieve this.
List<Group>
where each Group object will have a list of Entry objects.
[
"id":"group1",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"2222",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
,
"id":"group2",
"entries":[
"entryId":"4444",
"name":"test1"
,
"entryId":"1111",
"name":"test2"
,
"entryId":"2222",
"name":"test3"
]
,
"id":"group3",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"5555",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
]
So the expected out put is this :
[
"1111":[
"group1",
"group2",
"group3"
]
,
"2222":[
"group1",
"group2"
]
,
"3333":[
"group1",
"group3"
]
,
"4444":[
"group2"
]
,
"5555":[
"group3"
]
]
I'm using below way currently. which is working as expected, but is there a much simpler way in Java 8 I can achieve this.
public Map<String, List<String>> mapEntries(List<Group> groups)
Map<String, List<String>> entryMaps = new HashMap<>();
for (Group group : groups)
for (Entry entry : group.getEntries())
List<String> groupsEntryBelongs = new ArrayList<>();
if (groups.iterator().hasNext() && !entryMaps.keySet().contains(entry.getEntryId()))
updateGroups(groups, entry.getEntryId(), groupsEntryBelongs, entryMaps);
return entryMaps;
void updateGroups(List<Group> groups, String id, List<String> groupsEntryBelongs, Map<String, List<String>> entryMaps)
for (Group group : groups)
for (Entry entry : group.getEntries())
if (entry.getEntryId().equalsIgnoreCase(id))
groupsEntryBelongs.add(group.getId());
entryMaps.put(id, groupsEntryBelongs);
java java-8 java-stream grouping
|
show 1 more comment
up vote
3
down vote
favorite
I have a data structure like below. I'm trying to group the objects in such a way like Map<String, List<String>>
where key is the entryId and value is the List of groups it belongs to. entryId is always unique inside a group.
Example: entryId "1111" belongs to group1,group2,group3. I'm using the old java 7 way to iterate through the lists and checking. Is there any best possible way using Java8 Collectors/grouping to achieve this.
List<Group>
where each Group object will have a list of Entry objects.
[
"id":"group1",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"2222",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
,
"id":"group2",
"entries":[
"entryId":"4444",
"name":"test1"
,
"entryId":"1111",
"name":"test2"
,
"entryId":"2222",
"name":"test3"
]
,
"id":"group3",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"5555",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
]
So the expected out put is this :
[
"1111":[
"group1",
"group2",
"group3"
]
,
"2222":[
"group1",
"group2"
]
,
"3333":[
"group1",
"group3"
]
,
"4444":[
"group2"
]
,
"5555":[
"group3"
]
]
I'm using below way currently. which is working as expected, but is there a much simpler way in Java 8 I can achieve this.
public Map<String, List<String>> mapEntries(List<Group> groups)
Map<String, List<String>> entryMaps = new HashMap<>();
for (Group group : groups)
for (Entry entry : group.getEntries())
List<String> groupsEntryBelongs = new ArrayList<>();
if (groups.iterator().hasNext() && !entryMaps.keySet().contains(entry.getEntryId()))
updateGroups(groups, entry.getEntryId(), groupsEntryBelongs, entryMaps);
return entryMaps;
void updateGroups(List<Group> groups, String id, List<String> groupsEntryBelongs, Map<String, List<String>> entryMaps)
for (Group group : groups)
for (Entry entry : group.getEntries())
if (entry.getEntryId().equalsIgnoreCase(id))
groupsEntryBelongs.add(group.getId());
entryMaps.put(id, groupsEntryBelongs);
java java-8 java-stream grouping
and what have you tried so far?
– nullpointer
Nov 12 at 2:12
I used the way to iterate through the groups and getting the first entry id and then checking through the remaining groups to update the object Map<String, List<String>> I created. I 'm getting the results I need, but asking for suggestions to implement it in java8.
– Sreenivas Gundlapalli
Nov 12 at 2:24
Its always worth sharing what you've tried in the question to bring the clarity about your expected output.
– nullpointer
Nov 12 at 2:29
1
Possible duplicate of Java 8 lambdas group list into map
– uli
Nov 12 at 2:59
@uli no, its not what I'm looking for. I updated my question with the expected output and the way I'm doing it currently. The one you pointed is different. I'm trying to do the grouping by the values inside the inner lists.
– Sreenivas Gundlapalli
Nov 12 at 3:57
|
show 1 more comment
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I have a data structure like below. I'm trying to group the objects in such a way like Map<String, List<String>>
where key is the entryId and value is the List of groups it belongs to. entryId is always unique inside a group.
Example: entryId "1111" belongs to group1,group2,group3. I'm using the old java 7 way to iterate through the lists and checking. Is there any best possible way using Java8 Collectors/grouping to achieve this.
List<Group>
where each Group object will have a list of Entry objects.
[
"id":"group1",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"2222",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
,
"id":"group2",
"entries":[
"entryId":"4444",
"name":"test1"
,
"entryId":"1111",
"name":"test2"
,
"entryId":"2222",
"name":"test3"
]
,
"id":"group3",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"5555",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
]
So the expected out put is this :
[
"1111":[
"group1",
"group2",
"group3"
]
,
"2222":[
"group1",
"group2"
]
,
"3333":[
"group1",
"group3"
]
,
"4444":[
"group2"
]
,
"5555":[
"group3"
]
]
I'm using below way currently. which is working as expected, but is there a much simpler way in Java 8 I can achieve this.
public Map<String, List<String>> mapEntries(List<Group> groups)
Map<String, List<String>> entryMaps = new HashMap<>();
for (Group group : groups)
for (Entry entry : group.getEntries())
List<String> groupsEntryBelongs = new ArrayList<>();
if (groups.iterator().hasNext() && !entryMaps.keySet().contains(entry.getEntryId()))
updateGroups(groups, entry.getEntryId(), groupsEntryBelongs, entryMaps);
return entryMaps;
void updateGroups(List<Group> groups, String id, List<String> groupsEntryBelongs, Map<String, List<String>> entryMaps)
for (Group group : groups)
for (Entry entry : group.getEntries())
if (entry.getEntryId().equalsIgnoreCase(id))
groupsEntryBelongs.add(group.getId());
entryMaps.put(id, groupsEntryBelongs);
java java-8 java-stream grouping
I have a data structure like below. I'm trying to group the objects in such a way like Map<String, List<String>>
where key is the entryId and value is the List of groups it belongs to. entryId is always unique inside a group.
Example: entryId "1111" belongs to group1,group2,group3. I'm using the old java 7 way to iterate through the lists and checking. Is there any best possible way using Java8 Collectors/grouping to achieve this.
List<Group>
where each Group object will have a list of Entry objects.
[
"id":"group1",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"2222",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
,
"id":"group2",
"entries":[
"entryId":"4444",
"name":"test1"
,
"entryId":"1111",
"name":"test2"
,
"entryId":"2222",
"name":"test3"
]
,
"id":"group3",
"entries":[
"entryId":"1111",
"name":"test1"
,
"entryId":"5555",
"name":"test2"
,
"entryId":"3333",
"name":"test3"
]
]
So the expected out put is this :
[
"1111":[
"group1",
"group2",
"group3"
]
,
"2222":[
"group1",
"group2"
]
,
"3333":[
"group1",
"group3"
]
,
"4444":[
"group2"
]
,
"5555":[
"group3"
]
]
I'm using below way currently. which is working as expected, but is there a much simpler way in Java 8 I can achieve this.
public Map<String, List<String>> mapEntries(List<Group> groups)
Map<String, List<String>> entryMaps = new HashMap<>();
for (Group group : groups)
for (Entry entry : group.getEntries())
List<String> groupsEntryBelongs = new ArrayList<>();
if (groups.iterator().hasNext() && !entryMaps.keySet().contains(entry.getEntryId()))
updateGroups(groups, entry.getEntryId(), groupsEntryBelongs, entryMaps);
return entryMaps;
void updateGroups(List<Group> groups, String id, List<String> groupsEntryBelongs, Map<String, List<String>> entryMaps)
for (Group group : groups)
for (Entry entry : group.getEntries())
if (entry.getEntryId().equalsIgnoreCase(id))
groupsEntryBelongs.add(group.getId());
entryMaps.put(id, groupsEntryBelongs);
java java-8 java-stream grouping
java java-8 java-stream grouping
edited Nov 12 at 3:53
asked Nov 12 at 1:55
Sreenivas Gundlapalli
558
558
and what have you tried so far?
– nullpointer
Nov 12 at 2:12
I used the way to iterate through the groups and getting the first entry id and then checking through the remaining groups to update the object Map<String, List<String>> I created. I 'm getting the results I need, but asking for suggestions to implement it in java8.
– Sreenivas Gundlapalli
Nov 12 at 2:24
Its always worth sharing what you've tried in the question to bring the clarity about your expected output.
– nullpointer
Nov 12 at 2:29
1
Possible duplicate of Java 8 lambdas group list into map
– uli
Nov 12 at 2:59
@uli no, its not what I'm looking for. I updated my question with the expected output and the way I'm doing it currently. The one you pointed is different. I'm trying to do the grouping by the values inside the inner lists.
– Sreenivas Gundlapalli
Nov 12 at 3:57
|
show 1 more comment
and what have you tried so far?
– nullpointer
Nov 12 at 2:12
I used the way to iterate through the groups and getting the first entry id and then checking through the remaining groups to update the object Map<String, List<String>> I created. I 'm getting the results I need, but asking for suggestions to implement it in java8.
– Sreenivas Gundlapalli
Nov 12 at 2:24
Its always worth sharing what you've tried in the question to bring the clarity about your expected output.
– nullpointer
Nov 12 at 2:29
1
Possible duplicate of Java 8 lambdas group list into map
– uli
Nov 12 at 2:59
@uli no, its not what I'm looking for. I updated my question with the expected output and the way I'm doing it currently. The one you pointed is different. I'm trying to do the grouping by the values inside the inner lists.
– Sreenivas Gundlapalli
Nov 12 at 3:57
and what have you tried so far?
– nullpointer
Nov 12 at 2:12
and what have you tried so far?
– nullpointer
Nov 12 at 2:12
I used the way to iterate through the groups and getting the first entry id and then checking through the remaining groups to update the object Map<String, List<String>> I created. I 'm getting the results I need, but asking for suggestions to implement it in java8.
– Sreenivas Gundlapalli
Nov 12 at 2:24
I used the way to iterate through the groups and getting the first entry id and then checking through the remaining groups to update the object Map<String, List<String>> I created. I 'm getting the results I need, but asking for suggestions to implement it in java8.
– Sreenivas Gundlapalli
Nov 12 at 2:24
Its always worth sharing what you've tried in the question to bring the clarity about your expected output.
– nullpointer
Nov 12 at 2:29
Its always worth sharing what you've tried in the question to bring the clarity about your expected output.
– nullpointer
Nov 12 at 2:29
1
1
Possible duplicate of Java 8 lambdas group list into map
– uli
Nov 12 at 2:59
Possible duplicate of Java 8 lambdas group list into map
– uli
Nov 12 at 2:59
@uli no, its not what I'm looking for. I updated my question with the expected output and the way I'm doing it currently. The one you pointed is different. I'm trying to do the grouping by the values inside the inner lists.
– Sreenivas Gundlapalli
Nov 12 at 3:57
@uli no, its not what I'm looking for. I updated my question with the expected output and the way I'm doing it currently. The one you pointed is different. I'm trying to do the grouping by the values inside the inner lists.
– Sreenivas Gundlapalli
Nov 12 at 3:57
|
show 1 more comment
3 Answers
3
active
oldest
votes
up vote
4
down vote
accepted
You can do it as follows:
Map<String, Set<String>> entryMaps = new LinkedHashMap<>();
groups.forEach(group ->
group.getEntries().forEach(entry ->
entryMaps.computeIfAbsent(
entry.getEntryId().toLowerCase(),
k -> new LinkedHashSet<>())
.add(group.getId())));
This iterates the groups, then each group's entries and uses Map.computeIfAbsent
to put an entry with a new, empty LinkedHashSet
if the key wasn't present, returning either this empty set or the one matching that key. Then, the group id is added to this returned set.
Note: I'm using a Set
instead of a List
for values, to avoid possible duplicates. And LinkedHashMap
and LinkedhashSet
guarantee insertion-order.
1
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
add a comment |
up vote
1
down vote
You may do it like so,
Map<String, List<String>> groupIdsByEntryId = groups.stream()
.flatMap(g -> g.getEntries().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getEntryId(), g.getId())))
.collect(Collectors.groupingBy(Map.Entry::getKey, TreeMap::new,
Collectors.mapping(Map.Entry::getValue, Collectors.toList())));
Create a simple map
entry for each combination of the entryId and groupId values. Then use the groupingBy
collector to get the List
of groupId values against each entryId. If you need to sort by the keys, then pass in a TreeMap::new
to the mapFactory
overload of the operator.
And here's the output,
1111=[group1, group2, group3], 2222=[group1, group2], 3333=[group1, group3], 4444=[group2], 5555=[group3]
add a comment |
up vote
0
down vote
Something like this ought to work, it requires making some sort of intermediate tuple object:
list.stream()
.flatMap(group ->
group.getEntries.stream()
.map(entry -> new GroupEntry(group.getId(), entry.getEntryId()))
)
.collect(
Collectors.groupingBy(GroupEntry::getEntryId, Collectors.mapping(GroupEntry::getGroupId, Collectors.toList())));
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255091%2fhow-to-group-list-of-lists-using-a-certain-criteria-in-java-8%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
You can do it as follows:
Map<String, Set<String>> entryMaps = new LinkedHashMap<>();
groups.forEach(group ->
group.getEntries().forEach(entry ->
entryMaps.computeIfAbsent(
entry.getEntryId().toLowerCase(),
k -> new LinkedHashSet<>())
.add(group.getId())));
This iterates the groups, then each group's entries and uses Map.computeIfAbsent
to put an entry with a new, empty LinkedHashSet
if the key wasn't present, returning either this empty set or the one matching that key. Then, the group id is added to this returned set.
Note: I'm using a Set
instead of a List
for values, to avoid possible duplicates. And LinkedHashMap
and LinkedhashSet
guarantee insertion-order.
1
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
add a comment |
up vote
4
down vote
accepted
You can do it as follows:
Map<String, Set<String>> entryMaps = new LinkedHashMap<>();
groups.forEach(group ->
group.getEntries().forEach(entry ->
entryMaps.computeIfAbsent(
entry.getEntryId().toLowerCase(),
k -> new LinkedHashSet<>())
.add(group.getId())));
This iterates the groups, then each group's entries and uses Map.computeIfAbsent
to put an entry with a new, empty LinkedHashSet
if the key wasn't present, returning either this empty set or the one matching that key. Then, the group id is added to this returned set.
Note: I'm using a Set
instead of a List
for values, to avoid possible duplicates. And LinkedHashMap
and LinkedhashSet
guarantee insertion-order.
1
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
You can do it as follows:
Map<String, Set<String>> entryMaps = new LinkedHashMap<>();
groups.forEach(group ->
group.getEntries().forEach(entry ->
entryMaps.computeIfAbsent(
entry.getEntryId().toLowerCase(),
k -> new LinkedHashSet<>())
.add(group.getId())));
This iterates the groups, then each group's entries and uses Map.computeIfAbsent
to put an entry with a new, empty LinkedHashSet
if the key wasn't present, returning either this empty set or the one matching that key. Then, the group id is added to this returned set.
Note: I'm using a Set
instead of a List
for values, to avoid possible duplicates. And LinkedHashMap
and LinkedhashSet
guarantee insertion-order.
You can do it as follows:
Map<String, Set<String>> entryMaps = new LinkedHashMap<>();
groups.forEach(group ->
group.getEntries().forEach(entry ->
entryMaps.computeIfAbsent(
entry.getEntryId().toLowerCase(),
k -> new LinkedHashSet<>())
.add(group.getId())));
This iterates the groups, then each group's entries and uses Map.computeIfAbsent
to put an entry with a new, empty LinkedHashSet
if the key wasn't present, returning either this empty set or the one matching that key. Then, the group id is added to this returned set.
Note: I'm using a Set
instead of a List
for values, to avoid possible duplicates. And LinkedHashMap
and LinkedhashSet
guarantee insertion-order.
edited Nov 12 at 17:09
answered Nov 12 at 4:11


Federico Peralta Schaffner
21.8k43369
21.8k43369
1
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
add a comment |
1
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
1
1
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
Thanks. It worked. based on your implementation I had to declare as this. Map<String, LinkedHashSet<String>> entryMaps = new LinkedHashMap<>()
– Sreenivas Gundlapalli
Nov 12 at 4:25
add a comment |
up vote
1
down vote
You may do it like so,
Map<String, List<String>> groupIdsByEntryId = groups.stream()
.flatMap(g -> g.getEntries().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getEntryId(), g.getId())))
.collect(Collectors.groupingBy(Map.Entry::getKey, TreeMap::new,
Collectors.mapping(Map.Entry::getValue, Collectors.toList())));
Create a simple map
entry for each combination of the entryId and groupId values. Then use the groupingBy
collector to get the List
of groupId values against each entryId. If you need to sort by the keys, then pass in a TreeMap::new
to the mapFactory
overload of the operator.
And here's the output,
1111=[group1, group2, group3], 2222=[group1, group2], 3333=[group1, group3], 4444=[group2], 5555=[group3]
add a comment |
up vote
1
down vote
You may do it like so,
Map<String, List<String>> groupIdsByEntryId = groups.stream()
.flatMap(g -> g.getEntries().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getEntryId(), g.getId())))
.collect(Collectors.groupingBy(Map.Entry::getKey, TreeMap::new,
Collectors.mapping(Map.Entry::getValue, Collectors.toList())));
Create a simple map
entry for each combination of the entryId and groupId values. Then use the groupingBy
collector to get the List
of groupId values against each entryId. If you need to sort by the keys, then pass in a TreeMap::new
to the mapFactory
overload of the operator.
And here's the output,
1111=[group1, group2, group3], 2222=[group1, group2], 3333=[group1, group3], 4444=[group2], 5555=[group3]
add a comment |
up vote
1
down vote
up vote
1
down vote
You may do it like so,
Map<String, List<String>> groupIdsByEntryId = groups.stream()
.flatMap(g -> g.getEntries().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getEntryId(), g.getId())))
.collect(Collectors.groupingBy(Map.Entry::getKey, TreeMap::new,
Collectors.mapping(Map.Entry::getValue, Collectors.toList())));
Create a simple map
entry for each combination of the entryId and groupId values. Then use the groupingBy
collector to get the List
of groupId values against each entryId. If you need to sort by the keys, then pass in a TreeMap::new
to the mapFactory
overload of the operator.
And here's the output,
1111=[group1, group2, group3], 2222=[group1, group2], 3333=[group1, group3], 4444=[group2], 5555=[group3]
You may do it like so,
Map<String, List<String>> groupIdsByEntryId = groups.stream()
.flatMap(g -> g.getEntries().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getEntryId(), g.getId())))
.collect(Collectors.groupingBy(Map.Entry::getKey, TreeMap::new,
Collectors.mapping(Map.Entry::getValue, Collectors.toList())));
Create a simple map
entry for each combination of the entryId and groupId values. Then use the groupingBy
collector to get the List
of groupId values against each entryId. If you need to sort by the keys, then pass in a TreeMap::new
to the mapFactory
overload of the operator.
And here's the output,
1111=[group1, group2, group3], 2222=[group1, group2], 3333=[group1, group3], 4444=[group2], 5555=[group3]
edited Nov 12 at 4:53
answered Nov 12 at 4:24


Ravindra Ranwala
8,12431533
8,12431533
add a comment |
add a comment |
up vote
0
down vote
Something like this ought to work, it requires making some sort of intermediate tuple object:
list.stream()
.flatMap(group ->
group.getEntries.stream()
.map(entry -> new GroupEntry(group.getId(), entry.getEntryId()))
)
.collect(
Collectors.groupingBy(GroupEntry::getEntryId, Collectors.mapping(GroupEntry::getGroupId, Collectors.toList())));
add a comment |
up vote
0
down vote
Something like this ought to work, it requires making some sort of intermediate tuple object:
list.stream()
.flatMap(group ->
group.getEntries.stream()
.map(entry -> new GroupEntry(group.getId(), entry.getEntryId()))
)
.collect(
Collectors.groupingBy(GroupEntry::getEntryId, Collectors.mapping(GroupEntry::getGroupId, Collectors.toList())));
add a comment |
up vote
0
down vote
up vote
0
down vote
Something like this ought to work, it requires making some sort of intermediate tuple object:
list.stream()
.flatMap(group ->
group.getEntries.stream()
.map(entry -> new GroupEntry(group.getId(), entry.getEntryId()))
)
.collect(
Collectors.groupingBy(GroupEntry::getEntryId, Collectors.mapping(GroupEntry::getGroupId, Collectors.toList())));
Something like this ought to work, it requires making some sort of intermediate tuple object:
list.stream()
.flatMap(group ->
group.getEntries.stream()
.map(entry -> new GroupEntry(group.getId(), entry.getEntryId()))
)
.collect(
Collectors.groupingBy(GroupEntry::getEntryId, Collectors.mapping(GroupEntry::getGroupId, Collectors.toList())));
answered Nov 12 at 4:08
Dylan Bijnagte
1,156715
1,156715
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255091%2fhow-to-group-list-of-lists-using-a-certain-criteria-in-java-8%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mQtmQ zMd42Mrm,hYsQazp,WST0L4DlsKbbHyq,NYyruP eTTZeBBWuN7pao0kVlbWpdO6chT ZZffoOb0OW8wZ7 v
and what have you tried so far?
– nullpointer
Nov 12 at 2:12
I used the way to iterate through the groups and getting the first entry id and then checking through the remaining groups to update the object Map<String, List<String>> I created. I 'm getting the results I need, but asking for suggestions to implement it in java8.
– Sreenivas Gundlapalli
Nov 12 at 2:24
Its always worth sharing what you've tried in the question to bring the clarity about your expected output.
– nullpointer
Nov 12 at 2:29
1
Possible duplicate of Java 8 lambdas group list into map
– uli
Nov 12 at 2:59
@uli no, its not what I'm looking for. I updated my question with the expected output and the way I'm doing it currently. The one you pointed is different. I'm trying to do the grouping by the values inside the inner lists.
– Sreenivas Gundlapalli
Nov 12 at 3:57