Setting up default input device in Ubuntu 18.04 by using PyAudio
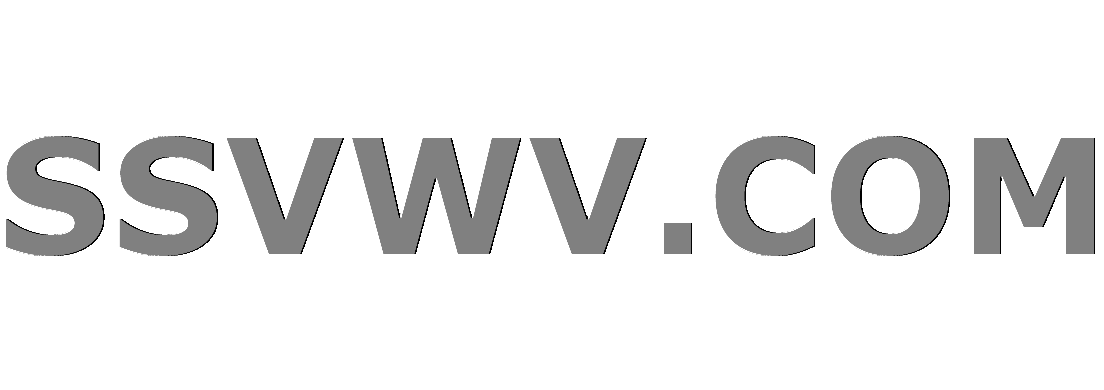
Multi tool use
up vote
0
down vote
favorite
I'm writing a program to take voice input from the built in microphone and then print the received voice on screen. My program is as follows :
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Speak Anything :")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print("You said : ".format(text))
except:
print("Sorry could not recognize what you said")
but I'm getting the error as follows :
Traceback (most recent call last):
File "text.py", line 4, in <module>
with sr.Microphone() as source:
File "/home/ashish/anaconda3/lib/python3.6/site-packages/speech_recognition/__init__.py", line 86, in __init__
device_info = audio.get_device_info_by_index(device_index) if device_index is not None else audio.get_default_input_device_info()
File "/home/ashish/anaconda3/lib/python3.6/site-packages/pyaudio.py", line 949, in get_default_input_device_info
device_index = pa.get_default_input_device()
OSError: No Default Input Device Available
python ubuntu module pyaudio
add a comment |
up vote
0
down vote
favorite
I'm writing a program to take voice input from the built in microphone and then print the received voice on screen. My program is as follows :
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Speak Anything :")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print("You said : ".format(text))
except:
print("Sorry could not recognize what you said")
but I'm getting the error as follows :
Traceback (most recent call last):
File "text.py", line 4, in <module>
with sr.Microphone() as source:
File "/home/ashish/anaconda3/lib/python3.6/site-packages/speech_recognition/__init__.py", line 86, in __init__
device_info = audio.get_device_info_by_index(device_index) if device_index is not None else audio.get_default_input_device_info()
File "/home/ashish/anaconda3/lib/python3.6/site-packages/pyaudio.py", line 949, in get_default_input_device_info
device_index = pa.get_default_input_device()
OSError: No Default Input Device Available
python ubuntu module pyaudio
Question has nothing to do withmachine-learning
- kindly do not spam the tag (removed).
– desertnaut
Nov 10 at 19:10
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm writing a program to take voice input from the built in microphone and then print the received voice on screen. My program is as follows :
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Speak Anything :")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print("You said : ".format(text))
except:
print("Sorry could not recognize what you said")
but I'm getting the error as follows :
Traceback (most recent call last):
File "text.py", line 4, in <module>
with sr.Microphone() as source:
File "/home/ashish/anaconda3/lib/python3.6/site-packages/speech_recognition/__init__.py", line 86, in __init__
device_info = audio.get_device_info_by_index(device_index) if device_index is not None else audio.get_default_input_device_info()
File "/home/ashish/anaconda3/lib/python3.6/site-packages/pyaudio.py", line 949, in get_default_input_device_info
device_index = pa.get_default_input_device()
OSError: No Default Input Device Available
python ubuntu module pyaudio
I'm writing a program to take voice input from the built in microphone and then print the received voice on screen. My program is as follows :
import speech_recognition as sr
r = sr.Recognizer()
with sr.Microphone() as source:
print("Speak Anything :")
audio = r.listen(source)
try:
text = r.recognize_google(audio)
print("You said : ".format(text))
except:
print("Sorry could not recognize what you said")
but I'm getting the error as follows :
Traceback (most recent call last):
File "text.py", line 4, in <module>
with sr.Microphone() as source:
File "/home/ashish/anaconda3/lib/python3.6/site-packages/speech_recognition/__init__.py", line 86, in __init__
device_info = audio.get_device_info_by_index(device_index) if device_index is not None else audio.get_default_input_device_info()
File "/home/ashish/anaconda3/lib/python3.6/site-packages/pyaudio.py", line 949, in get_default_input_device_info
device_index = pa.get_default_input_device()
OSError: No Default Input Device Available
python ubuntu module pyaudio
python ubuntu module pyaudio
edited Nov 10 at 19:09
desertnaut
15.2k53161
15.2k53161
asked Nov 10 at 18:47
Ashish Siwal
54
54
Question has nothing to do withmachine-learning
- kindly do not spam the tag (removed).
– desertnaut
Nov 10 at 19:10
add a comment |
Question has nothing to do withmachine-learning
- kindly do not spam the tag (removed).
– desertnaut
Nov 10 at 19:10
Question has nothing to do with
machine-learning
- kindly do not spam the tag (removed).– desertnaut
Nov 10 at 19:10
Question has nothing to do with
machine-learning
- kindly do not spam the tag (removed).– desertnaut
Nov 10 at 19:10
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
If you are using Raspberry Pi board, you’ll need a USB sound card (or USB microphone).
Once you do this, change all instances of Microphone() to Microphone(device_index=MICROPHONE_INDEX), where MICROPHONE_INDEX is the hardware-specific index of the microphone.
To hack on this library, first make sure you have all the requirements listed in the “Requirements” section.
-Most of the library code lives in speech_recognition/init.py.
- Examples live under the examples/ directory, and the demo script lives in speech_recognition/main.py.
- The FLAC encoder binaries are in the speech_recognition/ directory.
- Documentation can be found in the reference/ directory.
To run all the tests:
python -m unittest discover --verbose code here
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
If you are using Raspberry Pi board, you’ll need a USB sound card (or USB microphone).
Once you do this, change all instances of Microphone() to Microphone(device_index=MICROPHONE_INDEX), where MICROPHONE_INDEX is the hardware-specific index of the microphone.
To hack on this library, first make sure you have all the requirements listed in the “Requirements” section.
-Most of the library code lives in speech_recognition/init.py.
- Examples live under the examples/ directory, and the demo script lives in speech_recognition/main.py.
- The FLAC encoder binaries are in the speech_recognition/ directory.
- Documentation can be found in the reference/ directory.
To run all the tests:
python -m unittest discover --verbose code here
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
add a comment |
up vote
0
down vote
If you are using Raspberry Pi board, you’ll need a USB sound card (or USB microphone).
Once you do this, change all instances of Microphone() to Microphone(device_index=MICROPHONE_INDEX), where MICROPHONE_INDEX is the hardware-specific index of the microphone.
To hack on this library, first make sure you have all the requirements listed in the “Requirements” section.
-Most of the library code lives in speech_recognition/init.py.
- Examples live under the examples/ directory, and the demo script lives in speech_recognition/main.py.
- The FLAC encoder binaries are in the speech_recognition/ directory.
- Documentation can be found in the reference/ directory.
To run all the tests:
python -m unittest discover --verbose code here
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
add a comment |
up vote
0
down vote
up vote
0
down vote
If you are using Raspberry Pi board, you’ll need a USB sound card (or USB microphone).
Once you do this, change all instances of Microphone() to Microphone(device_index=MICROPHONE_INDEX), where MICROPHONE_INDEX is the hardware-specific index of the microphone.
To hack on this library, first make sure you have all the requirements listed in the “Requirements” section.
-Most of the library code lives in speech_recognition/init.py.
- Examples live under the examples/ directory, and the demo script lives in speech_recognition/main.py.
- The FLAC encoder binaries are in the speech_recognition/ directory.
- Documentation can be found in the reference/ directory.
To run all the tests:
python -m unittest discover --verbose code here
If you are using Raspberry Pi board, you’ll need a USB sound card (or USB microphone).
Once you do this, change all instances of Microphone() to Microphone(device_index=MICROPHONE_INDEX), where MICROPHONE_INDEX is the hardware-specific index of the microphone.
To hack on this library, first make sure you have all the requirements listed in the “Requirements” section.
-Most of the library code lives in speech_recognition/init.py.
- Examples live under the examples/ directory, and the demo script lives in speech_recognition/main.py.
- The FLAC encoder binaries are in the speech_recognition/ directory.
- Documentation can be found in the reference/ directory.
To run all the tests:
python -m unittest discover --verbose code here
answered Nov 10 at 19:05
Luis Miguel Baqueiro
1
1
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
add a comment |
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
Is there anything in the question specifying Raspberry Pi?
– desertnaut
Nov 10 at 19:09
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
I'm not using Raspberry Pi. I'm simply using laptop with ubuntu 18.04 OS on it.
– Ashish Siwal
Nov 10 at 19:20
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242285%2fsetting-up-default-input-device-in-ubuntu-18-04-by-using-pyaudio%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BMIo7Mz4V12kuc,mHGQsS sW,myaXg54YPBvfj,V6Xz4ra AeMFnbwHNEUr KfCQn12BQVj e GdeT1Np
Question has nothing to do with
machine-learning
- kindly do not spam the tag (removed).– desertnaut
Nov 10 at 19:10