How to shoot in the same direction my tank is facing?
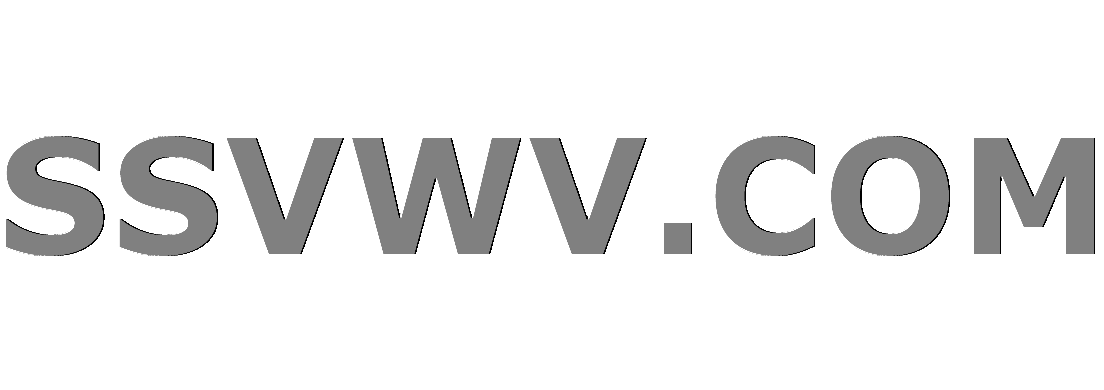
Multi tool use
up vote
0
down vote
favorite
I want to shoot a bullet in the direction the tank is facing. I use Accelerometer to control the angle of my tank. i want my tank to shoot on the direction it is facing as i tilt my device.
With my current script it only shoots vertically.
To duplicate the problem i couldn't shrink my code than this
from kivy.app import App
from kivy.uix.image import Image
from kivy.uix.widget import Widget
from kivy.properties import NumericProperty, ReferenceListProperty, ObjectProperty,ListProperty, BooleanProperty
from kivy.vector import Vector
from kivy.clock import Clock
import random
import math
from plyer import accelerometer
from kivy.lang import Builder
Builder.load_string("""
<Tank>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<Flame>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<AccelerometerGun>:
tank: tank
x_label: x_label
canvas:
Rectangle:
source: "wood.png"
#texture: root.bg_image.texture#source: "images/bg.png"
size: self.size#root.size[0]+200, root.size[1]
pos: self.pos#root.pos
Label:
id: x_label
text: "Tilt value "
center: self.parent.center
Tank:
id: tank
center: (root.width/2, 70) #self.parent.center
""")
class Flame(Image):
velocity_x = NumericProperty(0)
active = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(0)
def __init__(self, **kwargs):
super(Flame, self).__init__(**kwargs)
self.size_hint=(None, None)
self.source = "zn.zip"
self.anim_delay= 0.35
self.anim_loop = 1
rs = random.randint(70, 80)
self.size = (rs, rs)
self.bind(active=self.addV)
def addV(self, *args):
self.velocity_y += random.uniform(20.0, 25.0)
self.velocity_x += 0
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
self.angle = random.randint(0, 360)
self.size = (self.size[0]+1, self.size[1]+1)
if (self.y > pa.top/1.6):
pa.removeThis(self)
self.velocity_y = 0
self.velocity_x = 0
class Tank(Image):
velocity_x = NumericProperty(0)
activated = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(180)
def __init__(self, **kwargs):
super(Tank, self).__init__(**kwargs)
self.source = 'bomb.png'
self.anim_delay = 0.05
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
if (self.y < 0-self.size[0]):
self.velocity_y *= 0
if (self.x < 0):
self.velocity_x *= 0
if (self.right > pa.width):
self.velocity_x = 0
class AccelerometerGun(Widget):
tank = ObjectProperty(None)
angle = NumericProperty(180)
pr = NumericProperty(0.0)
flames =
def start(self):
self.tank.center = (self.width/2, 70)
self.x_label.center = self.center
self.tank.velocity = 0, 0
accelerometer.enable()
def update(self, dt):
#self.tank.move(self)
if self.tank.activated:
val = accelerometer.acceleration[:3]
if not val == (None, None, None):
x, y, z = val[0], val[1], val[2]
self.x_label.text = " ".join(["X", ": " + str(x)])
self.change(x, y)
if len(self.flames) != 0:
for tank in self.flames:
if tank.active:
tank.move(self)
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0
self.tank.velocity_x = 0
#self.tank.move(self)
def removeThis(self, bl):
self.flames.remove(bl)
self.remove_widget(bl)
bl = None
def startFlame(self, *args):
for tank in self.flames:
if not tank.active:
tank.active = True
def addFlame(self, *args):
self.fl = Flame()
self.add_widget(self.fl)
self.fl.center = self.tank.center
self.flames.append(self.fl)
self.fl.velocity = 0, 0
if len(self.flames) != 0:
self.startFlame()
def on_touch_down(self, touch):
self.tank.activated = True
class AccelerometerApp(App):
def build(self):
game = AccelerometerGun()
game.start()
Clock.schedule_interval(game.update, 1.0 / 60.0)
return game
if __name__ == '__main__':
AccelerometerApp().run()
Note: i want to move the bullet by using Vector not Animation
python python-2.7 vector kivy kivy-language
add a comment |
up vote
0
down vote
favorite
I want to shoot a bullet in the direction the tank is facing. I use Accelerometer to control the angle of my tank. i want my tank to shoot on the direction it is facing as i tilt my device.
With my current script it only shoots vertically.
To duplicate the problem i couldn't shrink my code than this
from kivy.app import App
from kivy.uix.image import Image
from kivy.uix.widget import Widget
from kivy.properties import NumericProperty, ReferenceListProperty, ObjectProperty,ListProperty, BooleanProperty
from kivy.vector import Vector
from kivy.clock import Clock
import random
import math
from plyer import accelerometer
from kivy.lang import Builder
Builder.load_string("""
<Tank>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<Flame>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<AccelerometerGun>:
tank: tank
x_label: x_label
canvas:
Rectangle:
source: "wood.png"
#texture: root.bg_image.texture#source: "images/bg.png"
size: self.size#root.size[0]+200, root.size[1]
pos: self.pos#root.pos
Label:
id: x_label
text: "Tilt value "
center: self.parent.center
Tank:
id: tank
center: (root.width/2, 70) #self.parent.center
""")
class Flame(Image):
velocity_x = NumericProperty(0)
active = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(0)
def __init__(self, **kwargs):
super(Flame, self).__init__(**kwargs)
self.size_hint=(None, None)
self.source = "zn.zip"
self.anim_delay= 0.35
self.anim_loop = 1
rs = random.randint(70, 80)
self.size = (rs, rs)
self.bind(active=self.addV)
def addV(self, *args):
self.velocity_y += random.uniform(20.0, 25.0)
self.velocity_x += 0
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
self.angle = random.randint(0, 360)
self.size = (self.size[0]+1, self.size[1]+1)
if (self.y > pa.top/1.6):
pa.removeThis(self)
self.velocity_y = 0
self.velocity_x = 0
class Tank(Image):
velocity_x = NumericProperty(0)
activated = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(180)
def __init__(self, **kwargs):
super(Tank, self).__init__(**kwargs)
self.source = 'bomb.png'
self.anim_delay = 0.05
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
if (self.y < 0-self.size[0]):
self.velocity_y *= 0
if (self.x < 0):
self.velocity_x *= 0
if (self.right > pa.width):
self.velocity_x = 0
class AccelerometerGun(Widget):
tank = ObjectProperty(None)
angle = NumericProperty(180)
pr = NumericProperty(0.0)
flames =
def start(self):
self.tank.center = (self.width/2, 70)
self.x_label.center = self.center
self.tank.velocity = 0, 0
accelerometer.enable()
def update(self, dt):
#self.tank.move(self)
if self.tank.activated:
val = accelerometer.acceleration[:3]
if not val == (None, None, None):
x, y, z = val[0], val[1], val[2]
self.x_label.text = " ".join(["X", ": " + str(x)])
self.change(x, y)
if len(self.flames) != 0:
for tank in self.flames:
if tank.active:
tank.move(self)
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0
self.tank.velocity_x = 0
#self.tank.move(self)
def removeThis(self, bl):
self.flames.remove(bl)
self.remove_widget(bl)
bl = None
def startFlame(self, *args):
for tank in self.flames:
if not tank.active:
tank.active = True
def addFlame(self, *args):
self.fl = Flame()
self.add_widget(self.fl)
self.fl.center = self.tank.center
self.flames.append(self.fl)
self.fl.velocity = 0, 0
if len(self.flames) != 0:
self.startFlame()
def on_touch_down(self, touch):
self.tank.activated = True
class AccelerometerApp(App):
def build(self):
game = AccelerometerGun()
game.start()
Clock.schedule_interval(game.update, 1.0 / 60.0)
return game
if __name__ == '__main__':
AccelerometerApp().run()
Note: i want to move the bullet by using Vector not Animation
python python-2.7 vector kivy kivy-language
I tried to run your code but got this error:[ERROR ] [Image ] Error reading file bomb.png
. Do I need some of your png files to run your example?
– Red Cricket
Nov 11 at 6:01
1
yeah you should get that error because there is no such image on your device. just rename bomb.png to another image that exists on your computer
– Denis
Nov 11 at 6:04
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I want to shoot a bullet in the direction the tank is facing. I use Accelerometer to control the angle of my tank. i want my tank to shoot on the direction it is facing as i tilt my device.
With my current script it only shoots vertically.
To duplicate the problem i couldn't shrink my code than this
from kivy.app import App
from kivy.uix.image import Image
from kivy.uix.widget import Widget
from kivy.properties import NumericProperty, ReferenceListProperty, ObjectProperty,ListProperty, BooleanProperty
from kivy.vector import Vector
from kivy.clock import Clock
import random
import math
from plyer import accelerometer
from kivy.lang import Builder
Builder.load_string("""
<Tank>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<Flame>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<AccelerometerGun>:
tank: tank
x_label: x_label
canvas:
Rectangle:
source: "wood.png"
#texture: root.bg_image.texture#source: "images/bg.png"
size: self.size#root.size[0]+200, root.size[1]
pos: self.pos#root.pos
Label:
id: x_label
text: "Tilt value "
center: self.parent.center
Tank:
id: tank
center: (root.width/2, 70) #self.parent.center
""")
class Flame(Image):
velocity_x = NumericProperty(0)
active = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(0)
def __init__(self, **kwargs):
super(Flame, self).__init__(**kwargs)
self.size_hint=(None, None)
self.source = "zn.zip"
self.anim_delay= 0.35
self.anim_loop = 1
rs = random.randint(70, 80)
self.size = (rs, rs)
self.bind(active=self.addV)
def addV(self, *args):
self.velocity_y += random.uniform(20.0, 25.0)
self.velocity_x += 0
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
self.angle = random.randint(0, 360)
self.size = (self.size[0]+1, self.size[1]+1)
if (self.y > pa.top/1.6):
pa.removeThis(self)
self.velocity_y = 0
self.velocity_x = 0
class Tank(Image):
velocity_x = NumericProperty(0)
activated = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(180)
def __init__(self, **kwargs):
super(Tank, self).__init__(**kwargs)
self.source = 'bomb.png'
self.anim_delay = 0.05
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
if (self.y < 0-self.size[0]):
self.velocity_y *= 0
if (self.x < 0):
self.velocity_x *= 0
if (self.right > pa.width):
self.velocity_x = 0
class AccelerometerGun(Widget):
tank = ObjectProperty(None)
angle = NumericProperty(180)
pr = NumericProperty(0.0)
flames =
def start(self):
self.tank.center = (self.width/2, 70)
self.x_label.center = self.center
self.tank.velocity = 0, 0
accelerometer.enable()
def update(self, dt):
#self.tank.move(self)
if self.tank.activated:
val = accelerometer.acceleration[:3]
if not val == (None, None, None):
x, y, z = val[0], val[1], val[2]
self.x_label.text = " ".join(["X", ": " + str(x)])
self.change(x, y)
if len(self.flames) != 0:
for tank in self.flames:
if tank.active:
tank.move(self)
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0
self.tank.velocity_x = 0
#self.tank.move(self)
def removeThis(self, bl):
self.flames.remove(bl)
self.remove_widget(bl)
bl = None
def startFlame(self, *args):
for tank in self.flames:
if not tank.active:
tank.active = True
def addFlame(self, *args):
self.fl = Flame()
self.add_widget(self.fl)
self.fl.center = self.tank.center
self.flames.append(self.fl)
self.fl.velocity = 0, 0
if len(self.flames) != 0:
self.startFlame()
def on_touch_down(self, touch):
self.tank.activated = True
class AccelerometerApp(App):
def build(self):
game = AccelerometerGun()
game.start()
Clock.schedule_interval(game.update, 1.0 / 60.0)
return game
if __name__ == '__main__':
AccelerometerApp().run()
Note: i want to move the bullet by using Vector not Animation
python python-2.7 vector kivy kivy-language
I want to shoot a bullet in the direction the tank is facing. I use Accelerometer to control the angle of my tank. i want my tank to shoot on the direction it is facing as i tilt my device.
With my current script it only shoots vertically.
To duplicate the problem i couldn't shrink my code than this
from kivy.app import App
from kivy.uix.image import Image
from kivy.uix.widget import Widget
from kivy.properties import NumericProperty, ReferenceListProperty, ObjectProperty,ListProperty, BooleanProperty
from kivy.vector import Vector
from kivy.clock import Clock
import random
import math
from plyer import accelerometer
from kivy.lang import Builder
Builder.load_string("""
<Tank>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<Flame>:
canvas.before:
PushMatrix
Rotate:
angle: self.angle
origin: self.center
canvas.after:
PopMatrix
<AccelerometerGun>:
tank: tank
x_label: x_label
canvas:
Rectangle:
source: "wood.png"
#texture: root.bg_image.texture#source: "images/bg.png"
size: self.size#root.size[0]+200, root.size[1]
pos: self.pos#root.pos
Label:
id: x_label
text: "Tilt value "
center: self.parent.center
Tank:
id: tank
center: (root.width/2, 70) #self.parent.center
""")
class Flame(Image):
velocity_x = NumericProperty(0)
active = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(0)
def __init__(self, **kwargs):
super(Flame, self).__init__(**kwargs)
self.size_hint=(None, None)
self.source = "zn.zip"
self.anim_delay= 0.35
self.anim_loop = 1
rs = random.randint(70, 80)
self.size = (rs, rs)
self.bind(active=self.addV)
def addV(self, *args):
self.velocity_y += random.uniform(20.0, 25.0)
self.velocity_x += 0
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
self.angle = random.randint(0, 360)
self.size = (self.size[0]+1, self.size[1]+1)
if (self.y > pa.top/1.6):
pa.removeThis(self)
self.velocity_y = 0
self.velocity_x = 0
class Tank(Image):
velocity_x = NumericProperty(0)
activated = BooleanProperty(False)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
angle = NumericProperty(180)
def __init__(self, **kwargs):
super(Tank, self).__init__(**kwargs)
self.source = 'bomb.png'
self.anim_delay = 0.05
def move(self, pa):
self.pos = Vector(*self.velocity) + self.pos
if (self.y < 0-self.size[0]):
self.velocity_y *= 0
if (self.x < 0):
self.velocity_x *= 0
if (self.right > pa.width):
self.velocity_x = 0
class AccelerometerGun(Widget):
tank = ObjectProperty(None)
angle = NumericProperty(180)
pr = NumericProperty(0.0)
flames =
def start(self):
self.tank.center = (self.width/2, 70)
self.x_label.center = self.center
self.tank.velocity = 0, 0
accelerometer.enable()
def update(self, dt):
#self.tank.move(self)
if self.tank.activated:
val = accelerometer.acceleration[:3]
if not val == (None, None, None):
x, y, z = val[0], val[1], val[2]
self.x_label.text = " ".join(["X", ": " + str(x)])
self.change(x, y)
if len(self.flames) != 0:
for tank in self.flames:
if tank.active:
tank.move(self)
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0
self.tank.velocity_x = 0
#self.tank.move(self)
def removeThis(self, bl):
self.flames.remove(bl)
self.remove_widget(bl)
bl = None
def startFlame(self, *args):
for tank in self.flames:
if not tank.active:
tank.active = True
def addFlame(self, *args):
self.fl = Flame()
self.add_widget(self.fl)
self.fl.center = self.tank.center
self.flames.append(self.fl)
self.fl.velocity = 0, 0
if len(self.flames) != 0:
self.startFlame()
def on_touch_down(self, touch):
self.tank.activated = True
class AccelerometerApp(App):
def build(self):
game = AccelerometerGun()
game.start()
Clock.schedule_interval(game.update, 1.0 / 60.0)
return game
if __name__ == '__main__':
AccelerometerApp().run()
Note: i want to move the bullet by using Vector not Animation
python python-2.7 vector kivy kivy-language
python python-2.7 vector kivy kivy-language
edited Nov 11 at 12:38
asked Nov 11 at 5:45


Denis
182111
182111
I tried to run your code but got this error:[ERROR ] [Image ] Error reading file bomb.png
. Do I need some of your png files to run your example?
– Red Cricket
Nov 11 at 6:01
1
yeah you should get that error because there is no such image on your device. just rename bomb.png to another image that exists on your computer
– Denis
Nov 11 at 6:04
add a comment |
I tried to run your code but got this error:[ERROR ] [Image ] Error reading file bomb.png
. Do I need some of your png files to run your example?
– Red Cricket
Nov 11 at 6:01
1
yeah you should get that error because there is no such image on your device. just rename bomb.png to another image that exists on your computer
– Denis
Nov 11 at 6:04
I tried to run your code but got this error:
[ERROR ] [Image ] Error reading file bomb.png
. Do I need some of your png files to run your example?– Red Cricket
Nov 11 at 6:01
I tried to run your code but got this error:
[ERROR ] [Image ] Error reading file bomb.png
. Do I need some of your png files to run your example?– Red Cricket
Nov 11 at 6:01
1
1
yeah you should get that error because there is no such image on your device. just rename bomb.png to another image that exists on your computer
– Denis
Nov 11 at 6:04
yeah you should get that error because there is no such image on your device. just rename bomb.png to another image that exists on your computer
– Denis
Nov 11 at 6:04
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
I tried solving the question myself.
def velX(self, vex):
for tank in self.flames:
if tank.active:
tank.velocity_x = -(vex*2.5) #+= -vel
and call it like this
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0 #(vy*-1)*3.5
self.tank.velocity_x = 0 #(vx*-1)*3.5
#self.tank.move(self)
self.velX(vx)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
I tried solving the question myself.
def velX(self, vex):
for tank in self.flames:
if tank.active:
tank.velocity_x = -(vex*2.5) #+= -vel
and call it like this
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0 #(vy*-1)*3.5
self.tank.velocity_x = 0 #(vx*-1)*3.5
#self.tank.move(self)
self.velX(vx)
add a comment |
up vote
0
down vote
accepted
I tried solving the question myself.
def velX(self, vex):
for tank in self.flames:
if tank.active:
tank.velocity_x = -(vex*2.5) #+= -vel
and call it like this
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0 #(vy*-1)*3.5
self.tank.velocity_x = 0 #(vx*-1)*3.5
#self.tank.move(self)
self.velX(vx)
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I tried solving the question myself.
def velX(self, vex):
for tank in self.flames:
if tank.active:
tank.velocity_x = -(vex*2.5) #+= -vel
and call it like this
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0 #(vy*-1)*3.5
self.tank.velocity_x = 0 #(vx*-1)*3.5
#self.tank.move(self)
self.velX(vx)
I tried solving the question myself.
def velX(self, vex):
for tank in self.flames:
if tank.active:
tank.velocity_x = -(vex*2.5) #+= -vel
and call it like this
def change(self, vx, vy):
max = 2.99
min = -2.9
range = max - min
correctedStartValue = vx - min
percentage = (correctedStartValue * 180) / range
if percentage > 180:
percentage = 180
if percentage < 0:
percentage = 0
if vx == 0.0:
percentage = 90
self.tank.angle = percentage
self.addFlame()
self.x_label.text = " ".join(["X: ", str(int(vx)), "%: ", str(percentage), "Fps: ", str(int(Clock.get_fps()))])
self.tank.velocity_y = 0 #(vy*-1)*3.5
self.tank.velocity_x = 0 #(vx*-1)*3.5
#self.tank.move(self)
self.velX(vx)
answered Nov 11 at 13:11


Denis
182111
182111
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246174%2fhow-to-shoot-in-the-same-direction-my-tank-is-facing%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bX6eE Q6SO528O,R6il1w k 1KWpxx4MzGMW TEKY
I tried to run your code but got this error:
[ERROR ] [Image ] Error reading file bomb.png
. Do I need some of your png files to run your example?– Red Cricket
Nov 11 at 6:01
1
yeah you should get that error because there is no such image on your device. just rename bomb.png to another image that exists on your computer
– Denis
Nov 11 at 6:04