SQL not updating properly
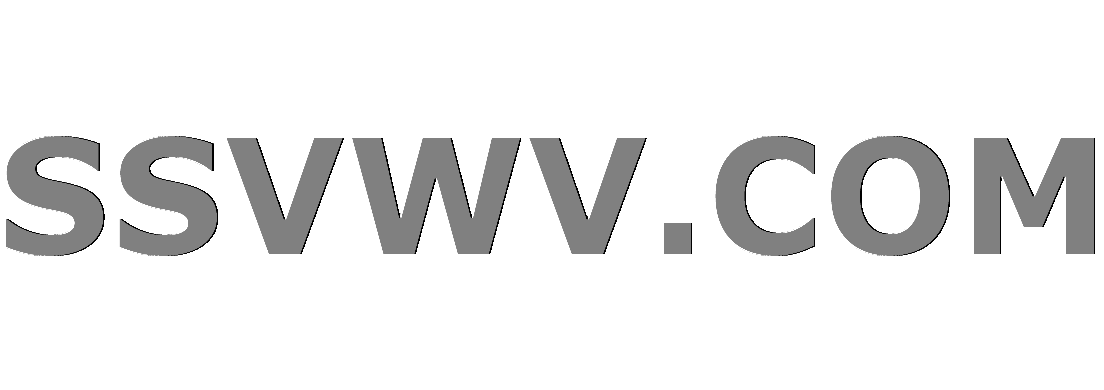
Multi tool use
For our project we have a table where we get all football results for one match day. Depending on their SQL database value, an input gets shown where the user can add the match results. When we hit the 'save' button, the insert into the database gets triggered. For some reason, from time to time not all lines get added to the database.
[HttpPost("UpdateMatchDayScore")]
public async void UpdateMatchDayScore([FromBody]UpdateMatchDayScoreViewModel model)
var matchDayId = model[0].matchDayId;
var poolId = model[0].poolId;
RankingSearch rankingSearch = await _rankingService.CreateNewRanking(matchDayId, poolId);
foreach (var t in model)
_matchDayService.UpdateMatchDayScore(rankingSearch.RankingId,t.poolId, t.homeTeamId, t.awayTeamId, Int32.Parse(t.scoreHome),Int32.Parse(t.scoreAway), t.matchDayId);
_calculateRanking.CalculatePosition(rankingSearch.RankingId);
This is our viewmodel:
public class UpdateMatchDayScoreViewModel
public int poolId get; set;
public int homeTeamId get; set;
public int awayTeamId get; set;
public string scoreHome get; set;
public string scoreAway get; set;
public int matchDayId get; set;
This is our matchday repository:
public async void UpdateMatchDayScore(int poolId, int homeTeamId, int awayTeamId,
int scoreHome, int scoreAway,
int matchDayId)
using (var conn = _connector.OpenConnection())
await conn.QueryAsync<MatchDay>(StoredProcs.UpdateMatchScore,
new
pouleId = poolId,
awayTeamId = awayTeamId,
homeTeamId= homeTeamId,
scoreAway = scoreAway,
scoreHome = scoreHome,
matchDayId = matchDayId
, CommandType.StoredProcedure);
This is our matchday service:
public void UpdateMatchDayScore(int rankingId, int poolId, int homeTeamId, int awayTeamId, int scoreHome, int scoreAway, int matchDayId)
_calculateRanking.CalculateRankings(rankingId, matchDayId, awayTeamId, homeTeamId, scoreHome, scoreAway);
_matchDayRepository.UpdateMatchDayScore(poolId, homeTeamId, awayTeamId, scoreHome, scoreAway,
matchDayId);
Sample model:
pouleId = 46273,
awayTeamId = 400069,
homeTeamId= 400002,
scoreAway = 2,
scoreHome = 4,
matchDayId = 12
We did some math and in total for one matchday(which contains 8 games) opens 41 connections. We think we might be doing to much at once? Could anyone help us out?
c# sql asp.net
add a comment |
For our project we have a table where we get all football results for one match day. Depending on their SQL database value, an input gets shown where the user can add the match results. When we hit the 'save' button, the insert into the database gets triggered. For some reason, from time to time not all lines get added to the database.
[HttpPost("UpdateMatchDayScore")]
public async void UpdateMatchDayScore([FromBody]UpdateMatchDayScoreViewModel model)
var matchDayId = model[0].matchDayId;
var poolId = model[0].poolId;
RankingSearch rankingSearch = await _rankingService.CreateNewRanking(matchDayId, poolId);
foreach (var t in model)
_matchDayService.UpdateMatchDayScore(rankingSearch.RankingId,t.poolId, t.homeTeamId, t.awayTeamId, Int32.Parse(t.scoreHome),Int32.Parse(t.scoreAway), t.matchDayId);
_calculateRanking.CalculatePosition(rankingSearch.RankingId);
This is our viewmodel:
public class UpdateMatchDayScoreViewModel
public int poolId get; set;
public int homeTeamId get; set;
public int awayTeamId get; set;
public string scoreHome get; set;
public string scoreAway get; set;
public int matchDayId get; set;
This is our matchday repository:
public async void UpdateMatchDayScore(int poolId, int homeTeamId, int awayTeamId,
int scoreHome, int scoreAway,
int matchDayId)
using (var conn = _connector.OpenConnection())
await conn.QueryAsync<MatchDay>(StoredProcs.UpdateMatchScore,
new
pouleId = poolId,
awayTeamId = awayTeamId,
homeTeamId= homeTeamId,
scoreAway = scoreAway,
scoreHome = scoreHome,
matchDayId = matchDayId
, CommandType.StoredProcedure);
This is our matchday service:
public void UpdateMatchDayScore(int rankingId, int poolId, int homeTeamId, int awayTeamId, int scoreHome, int scoreAway, int matchDayId)
_calculateRanking.CalculateRankings(rankingId, matchDayId, awayTeamId, homeTeamId, scoreHome, scoreAway);
_matchDayRepository.UpdateMatchDayScore(poolId, homeTeamId, awayTeamId, scoreHome, scoreAway,
matchDayId);
Sample model:
pouleId = 46273,
awayTeamId = 400069,
homeTeamId= 400002,
scoreAway = 2,
scoreHome = 4,
matchDayId = 12
We did some math and in total for one matchday(which contains 8 games) opens 41 connections. We think we might be doing to much at once? Could anyone help us out?
c# sql asp.net
Hi @VincentVH, welcome to SO. It would help if you post code for theUpdateMatchDayScoreViewModel
class as well a sample data for a test run.
– JuanR
Nov 15 '18 at 15:24
hi, sorry did not expect such a quick response. I updated it a bit, I might still be missing some stuff.
– VincentVH
Nov 15 '18 at 15:35
No worries. Can you post a samplemodel
? (The actual property values)
– JuanR
Nov 15 '18 at 15:49
I added a sample model, not sure if it will really help that much.
– VincentVH
Nov 15 '18 at 15:53
add a comment |
For our project we have a table where we get all football results for one match day. Depending on their SQL database value, an input gets shown where the user can add the match results. When we hit the 'save' button, the insert into the database gets triggered. For some reason, from time to time not all lines get added to the database.
[HttpPost("UpdateMatchDayScore")]
public async void UpdateMatchDayScore([FromBody]UpdateMatchDayScoreViewModel model)
var matchDayId = model[0].matchDayId;
var poolId = model[0].poolId;
RankingSearch rankingSearch = await _rankingService.CreateNewRanking(matchDayId, poolId);
foreach (var t in model)
_matchDayService.UpdateMatchDayScore(rankingSearch.RankingId,t.poolId, t.homeTeamId, t.awayTeamId, Int32.Parse(t.scoreHome),Int32.Parse(t.scoreAway), t.matchDayId);
_calculateRanking.CalculatePosition(rankingSearch.RankingId);
This is our viewmodel:
public class UpdateMatchDayScoreViewModel
public int poolId get; set;
public int homeTeamId get; set;
public int awayTeamId get; set;
public string scoreHome get; set;
public string scoreAway get; set;
public int matchDayId get; set;
This is our matchday repository:
public async void UpdateMatchDayScore(int poolId, int homeTeamId, int awayTeamId,
int scoreHome, int scoreAway,
int matchDayId)
using (var conn = _connector.OpenConnection())
await conn.QueryAsync<MatchDay>(StoredProcs.UpdateMatchScore,
new
pouleId = poolId,
awayTeamId = awayTeamId,
homeTeamId= homeTeamId,
scoreAway = scoreAway,
scoreHome = scoreHome,
matchDayId = matchDayId
, CommandType.StoredProcedure);
This is our matchday service:
public void UpdateMatchDayScore(int rankingId, int poolId, int homeTeamId, int awayTeamId, int scoreHome, int scoreAway, int matchDayId)
_calculateRanking.CalculateRankings(rankingId, matchDayId, awayTeamId, homeTeamId, scoreHome, scoreAway);
_matchDayRepository.UpdateMatchDayScore(poolId, homeTeamId, awayTeamId, scoreHome, scoreAway,
matchDayId);
Sample model:
pouleId = 46273,
awayTeamId = 400069,
homeTeamId= 400002,
scoreAway = 2,
scoreHome = 4,
matchDayId = 12
We did some math and in total for one matchday(which contains 8 games) opens 41 connections. We think we might be doing to much at once? Could anyone help us out?
c# sql asp.net
For our project we have a table where we get all football results for one match day. Depending on their SQL database value, an input gets shown where the user can add the match results. When we hit the 'save' button, the insert into the database gets triggered. For some reason, from time to time not all lines get added to the database.
[HttpPost("UpdateMatchDayScore")]
public async void UpdateMatchDayScore([FromBody]UpdateMatchDayScoreViewModel model)
var matchDayId = model[0].matchDayId;
var poolId = model[0].poolId;
RankingSearch rankingSearch = await _rankingService.CreateNewRanking(matchDayId, poolId);
foreach (var t in model)
_matchDayService.UpdateMatchDayScore(rankingSearch.RankingId,t.poolId, t.homeTeamId, t.awayTeamId, Int32.Parse(t.scoreHome),Int32.Parse(t.scoreAway), t.matchDayId);
_calculateRanking.CalculatePosition(rankingSearch.RankingId);
This is our viewmodel:
public class UpdateMatchDayScoreViewModel
public int poolId get; set;
public int homeTeamId get; set;
public int awayTeamId get; set;
public string scoreHome get; set;
public string scoreAway get; set;
public int matchDayId get; set;
This is our matchday repository:
public async void UpdateMatchDayScore(int poolId, int homeTeamId, int awayTeamId,
int scoreHome, int scoreAway,
int matchDayId)
using (var conn = _connector.OpenConnection())
await conn.QueryAsync<MatchDay>(StoredProcs.UpdateMatchScore,
new
pouleId = poolId,
awayTeamId = awayTeamId,
homeTeamId= homeTeamId,
scoreAway = scoreAway,
scoreHome = scoreHome,
matchDayId = matchDayId
, CommandType.StoredProcedure);
This is our matchday service:
public void UpdateMatchDayScore(int rankingId, int poolId, int homeTeamId, int awayTeamId, int scoreHome, int scoreAway, int matchDayId)
_calculateRanking.CalculateRankings(rankingId, matchDayId, awayTeamId, homeTeamId, scoreHome, scoreAway);
_matchDayRepository.UpdateMatchDayScore(poolId, homeTeamId, awayTeamId, scoreHome, scoreAway,
matchDayId);
Sample model:
pouleId = 46273,
awayTeamId = 400069,
homeTeamId= 400002,
scoreAway = 2,
scoreHome = 4,
matchDayId = 12
We did some math and in total for one matchday(which contains 8 games) opens 41 connections. We think we might be doing to much at once? Could anyone help us out?
c# sql asp.net
c# sql asp.net
edited Nov 15 '18 at 15:53
VincentVH
asked Nov 15 '18 at 15:20
VincentVHVincentVH
35
35
Hi @VincentVH, welcome to SO. It would help if you post code for theUpdateMatchDayScoreViewModel
class as well a sample data for a test run.
– JuanR
Nov 15 '18 at 15:24
hi, sorry did not expect such a quick response. I updated it a bit, I might still be missing some stuff.
– VincentVH
Nov 15 '18 at 15:35
No worries. Can you post a samplemodel
? (The actual property values)
– JuanR
Nov 15 '18 at 15:49
I added a sample model, not sure if it will really help that much.
– VincentVH
Nov 15 '18 at 15:53
add a comment |
Hi @VincentVH, welcome to SO. It would help if you post code for theUpdateMatchDayScoreViewModel
class as well a sample data for a test run.
– JuanR
Nov 15 '18 at 15:24
hi, sorry did not expect such a quick response. I updated it a bit, I might still be missing some stuff.
– VincentVH
Nov 15 '18 at 15:35
No worries. Can you post a samplemodel
? (The actual property values)
– JuanR
Nov 15 '18 at 15:49
I added a sample model, not sure if it will really help that much.
– VincentVH
Nov 15 '18 at 15:53
Hi @VincentVH, welcome to SO. It would help if you post code for the
UpdateMatchDayScoreViewModel
class as well a sample data for a test run.– JuanR
Nov 15 '18 at 15:24
Hi @VincentVH, welcome to SO. It would help if you post code for the
UpdateMatchDayScoreViewModel
class as well a sample data for a test run.– JuanR
Nov 15 '18 at 15:24
hi, sorry did not expect such a quick response. I updated it a bit, I might still be missing some stuff.
– VincentVH
Nov 15 '18 at 15:35
hi, sorry did not expect such a quick response. I updated it a bit, I might still be missing some stuff.
– VincentVH
Nov 15 '18 at 15:35
No worries. Can you post a sample
model
? (The actual property values)– JuanR
Nov 15 '18 at 15:49
No worries. Can you post a sample
model
? (The actual property values)– JuanR
Nov 15 '18 at 15:49
I added a sample model, not sure if it will really help that much.
– VincentVH
Nov 15 '18 at 15:53
I added a sample model, not sure if it will really help that much.
– VincentVH
Nov 15 '18 at 15:53
add a comment |
1 Answer
1
active
oldest
votes
This does not look like an issue with the number of connection, rather it’s the way you are calling your methods.
Your code is mix of Async and Sync methods. On top of it, the Async methods are not invoked properly using “await” at all the places. And this is an open invitation to trouble.
Go through this post to understand the issues you may face when you do that.
https://blog.stephencleary.com/2012/07/dont-block-on-async-code.html
Note: This post talks about dead-lock but it is applicable in your case as well.
I am assuming that the foreach
loop is missing updating some of the records due to not receiving correct Task
status in some of the cases.
I would suggest that if you are new to async-await
domain, try your code with only sync methods first and then try to apply the async
feature.
If some of the methods are async
-only, you can add .Wait()
method at the end for your code to wait for the Task
to get completed before moving to next statement of execution.
https://docs.microsoft.com/en-us/dotnet/api/system.threading.tasks.task.wait?view=netframework-4.7.2#System_Threading_Tasks_Task_Wait
Hope this helps!
1
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53322591%2fsql-not-updating-properly%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This does not look like an issue with the number of connection, rather it’s the way you are calling your methods.
Your code is mix of Async and Sync methods. On top of it, the Async methods are not invoked properly using “await” at all the places. And this is an open invitation to trouble.
Go through this post to understand the issues you may face when you do that.
https://blog.stephencleary.com/2012/07/dont-block-on-async-code.html
Note: This post talks about dead-lock but it is applicable in your case as well.
I am assuming that the foreach
loop is missing updating some of the records due to not receiving correct Task
status in some of the cases.
I would suggest that if you are new to async-await
domain, try your code with only sync methods first and then try to apply the async
feature.
If some of the methods are async
-only, you can add .Wait()
method at the end for your code to wait for the Task
to get completed before moving to next statement of execution.
https://docs.microsoft.com/en-us/dotnet/api/system.threading.tasks.task.wait?view=netframework-4.7.2#System_Threading_Tasks_Task_Wait
Hope this helps!
1
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
add a comment |
This does not look like an issue with the number of connection, rather it’s the way you are calling your methods.
Your code is mix of Async and Sync methods. On top of it, the Async methods are not invoked properly using “await” at all the places. And this is an open invitation to trouble.
Go through this post to understand the issues you may face when you do that.
https://blog.stephencleary.com/2012/07/dont-block-on-async-code.html
Note: This post talks about dead-lock but it is applicable in your case as well.
I am assuming that the foreach
loop is missing updating some of the records due to not receiving correct Task
status in some of the cases.
I would suggest that if you are new to async-await
domain, try your code with only sync methods first and then try to apply the async
feature.
If some of the methods are async
-only, you can add .Wait()
method at the end for your code to wait for the Task
to get completed before moving to next statement of execution.
https://docs.microsoft.com/en-us/dotnet/api/system.threading.tasks.task.wait?view=netframework-4.7.2#System_Threading_Tasks_Task_Wait
Hope this helps!
1
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
add a comment |
This does not look like an issue with the number of connection, rather it’s the way you are calling your methods.
Your code is mix of Async and Sync methods. On top of it, the Async methods are not invoked properly using “await” at all the places. And this is an open invitation to trouble.
Go through this post to understand the issues you may face when you do that.
https://blog.stephencleary.com/2012/07/dont-block-on-async-code.html
Note: This post talks about dead-lock but it is applicable in your case as well.
I am assuming that the foreach
loop is missing updating some of the records due to not receiving correct Task
status in some of the cases.
I would suggest that if you are new to async-await
domain, try your code with only sync methods first and then try to apply the async
feature.
If some of the methods are async
-only, you can add .Wait()
method at the end for your code to wait for the Task
to get completed before moving to next statement of execution.
https://docs.microsoft.com/en-us/dotnet/api/system.threading.tasks.task.wait?view=netframework-4.7.2#System_Threading_Tasks_Task_Wait
Hope this helps!
This does not look like an issue with the number of connection, rather it’s the way you are calling your methods.
Your code is mix of Async and Sync methods. On top of it, the Async methods are not invoked properly using “await” at all the places. And this is an open invitation to trouble.
Go through this post to understand the issues you may face when you do that.
https://blog.stephencleary.com/2012/07/dont-block-on-async-code.html
Note: This post talks about dead-lock but it is applicable in your case as well.
I am assuming that the foreach
loop is missing updating some of the records due to not receiving correct Task
status in some of the cases.
I would suggest that if you are new to async-await
domain, try your code with only sync methods first and then try to apply the async
feature.
If some of the methods are async
-only, you can add .Wait()
method at the end for your code to wait for the Task
to get completed before moving to next statement of execution.
https://docs.microsoft.com/en-us/dotnet/api/system.threading.tasks.task.wait?view=netframework-4.7.2#System_Threading_Tasks_Task_Wait
Hope this helps!
answered Nov 15 '18 at 16:07


dj79dj79
1,309214
1,309214
1
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
add a comment |
1
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
1
1
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
We did what you suggested, starting from everything in sync, and slowely adding in our async where required and it fixed our issue! Thanks!
– VincentVH
Nov 16 '18 at 10:46
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
You are welcome! :)
– dj79
Nov 16 '18 at 10:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53322591%2fsql-not-updating-properly%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
U CLGSXFsJUsB4gv,LQx4fbzcDBlpl4HJEI1hqKgAYsDSLGZtsuTw aVILryQ,0gmEINsbWKF4A,YcQw2M
Hi @VincentVH, welcome to SO. It would help if you post code for the
UpdateMatchDayScoreViewModel
class as well a sample data for a test run.– JuanR
Nov 15 '18 at 15:24
hi, sorry did not expect such a quick response. I updated it a bit, I might still be missing some stuff.
– VincentVH
Nov 15 '18 at 15:35
No worries. Can you post a sample
model
? (The actual property values)– JuanR
Nov 15 '18 at 15:49
I added a sample model, not sure if it will really help that much.
– VincentVH
Nov 15 '18 at 15:53