Fetch with array and for loop
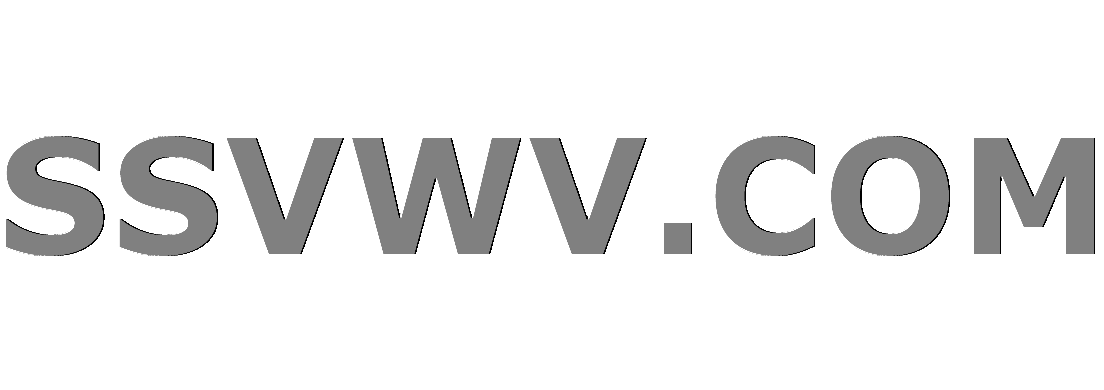
Multi tool use
I have around five URL(api) i need to fetch. I have made an array with the urls. I need to make a for loop so i can get each url-info into different boxes.
I started earlier with fetch url, then i wrote down +info.name+ etc on each thing i wanted to get out at the page, but it will take to long time, so i need to use a for loop.
How do i do that? So i dont have to fetch and write +info+ so many times?
var urls = ["url i need to fetch",
"url i need to fetch",
"url i need to fetch",];
var url = "url i wanted to fetch";
fetch (url)
.then (result => result.json())
.then ((res) =>
console.log(res);
createCards (res);
)
.catch(err => console.log(err))
function createCards(card) {
var div = document.getElementById('card');
div.innerHTML = `
<h2>`+card.name+`</h2>
<div>
<b>HTML text </b>`+info.from.card+`</div>
javascript arrays for-loop
add a comment |
I have around five URL(api) i need to fetch. I have made an array with the urls. I need to make a for loop so i can get each url-info into different boxes.
I started earlier with fetch url, then i wrote down +info.name+ etc on each thing i wanted to get out at the page, but it will take to long time, so i need to use a for loop.
How do i do that? So i dont have to fetch and write +info+ so many times?
var urls = ["url i need to fetch",
"url i need to fetch",
"url i need to fetch",];
var url = "url i wanted to fetch";
fetch (url)
.then (result => result.json())
.then ((res) =>
console.log(res);
createCards (res);
)
.catch(err => console.log(err))
function createCards(card) {
var div = document.getElementById('card');
div.innerHTML = `
<h2>`+card.name+`</h2>
<div>
<b>HTML text </b>`+info.from.card+`</div>
javascript arrays for-loop
Can you use async/await?
– ODYN-Kon
Nov 15 '18 at 15:23
Have not used that before, so the best is if someone can help me with how Im gonna fetch it all with a for loop
– KX0
Nov 15 '18 at 15:24
add a comment |
I have around five URL(api) i need to fetch. I have made an array with the urls. I need to make a for loop so i can get each url-info into different boxes.
I started earlier with fetch url, then i wrote down +info.name+ etc on each thing i wanted to get out at the page, but it will take to long time, so i need to use a for loop.
How do i do that? So i dont have to fetch and write +info+ so many times?
var urls = ["url i need to fetch",
"url i need to fetch",
"url i need to fetch",];
var url = "url i wanted to fetch";
fetch (url)
.then (result => result.json())
.then ((res) =>
console.log(res);
createCards (res);
)
.catch(err => console.log(err))
function createCards(card) {
var div = document.getElementById('card');
div.innerHTML = `
<h2>`+card.name+`</h2>
<div>
<b>HTML text </b>`+info.from.card+`</div>
javascript arrays for-loop
I have around five URL(api) i need to fetch. I have made an array with the urls. I need to make a for loop so i can get each url-info into different boxes.
I started earlier with fetch url, then i wrote down +info.name+ etc on each thing i wanted to get out at the page, but it will take to long time, so i need to use a for loop.
How do i do that? So i dont have to fetch and write +info+ so many times?
var urls = ["url i need to fetch",
"url i need to fetch",
"url i need to fetch",];
var url = "url i wanted to fetch";
fetch (url)
.then (result => result.json())
.then ((res) =>
console.log(res);
createCards (res);
)
.catch(err => console.log(err))
function createCards(card) {
var div = document.getElementById('card');
div.innerHTML = `
<h2>`+card.name+`</h2>
<div>
<b>HTML text </b>`+info.from.card+`</div>
javascript arrays for-loop
javascript arrays for-loop
asked Nov 15 '18 at 15:21
KX0KX0
346
346
Can you use async/await?
– ODYN-Kon
Nov 15 '18 at 15:23
Have not used that before, so the best is if someone can help me with how Im gonna fetch it all with a for loop
– KX0
Nov 15 '18 at 15:24
add a comment |
Can you use async/await?
– ODYN-Kon
Nov 15 '18 at 15:23
Have not used that before, so the best is if someone can help me with how Im gonna fetch it all with a for loop
– KX0
Nov 15 '18 at 15:24
Can you use async/await?
– ODYN-Kon
Nov 15 '18 at 15:23
Can you use async/await?
– ODYN-Kon
Nov 15 '18 at 15:23
Have not used that before, so the best is if someone can help me with how Im gonna fetch it all with a for loop
– KX0
Nov 15 '18 at 15:24
Have not used that before, so the best is if someone can help me with how Im gonna fetch it all with a for loop
– KX0
Nov 15 '18 at 15:24
add a comment |
2 Answers
2
active
oldest
votes
You can use Promise.all() to run all requests at the same time:
const urls = [
"url i need to fetch",
"url i need to fetch",
"url i need to fetch"
];
Promise
.all( urls.map( url => fetch( url)))
.then( responses => responses.map( response => response.json()))
.then( results =>
// do something with the results array
)
.catch( error =>
// handle the error
);
add a comment |
You can use a for loop and use let
which creates a block level scope
var urls = ["url1", "url2", "url3"];
for (let i = 0; i < urls.length; i++)
fetch(urls[i])
.then(result => result.json())
.then((res) =>
console.log(res);
createCards(res);
)
.catch(err => console.log(err))
function createCards(card)
var div = document.getElementById('card');
div.innerHTML += `<h2>$card.name</h2>
<div>
<b>HTML text </b>$someOtherText</div>`
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
div.innerHTML += ....
, else only the last result to arrive will be rendered.
– Shilly
Nov 15 '18 at 15:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53322611%2ffetch-with-array-and-for-loop%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use Promise.all() to run all requests at the same time:
const urls = [
"url i need to fetch",
"url i need to fetch",
"url i need to fetch"
];
Promise
.all( urls.map( url => fetch( url)))
.then( responses => responses.map( response => response.json()))
.then( results =>
// do something with the results array
)
.catch( error =>
// handle the error
);
add a comment |
You can use Promise.all() to run all requests at the same time:
const urls = [
"url i need to fetch",
"url i need to fetch",
"url i need to fetch"
];
Promise
.all( urls.map( url => fetch( url)))
.then( responses => responses.map( response => response.json()))
.then( results =>
// do something with the results array
)
.catch( error =>
// handle the error
);
add a comment |
You can use Promise.all() to run all requests at the same time:
const urls = [
"url i need to fetch",
"url i need to fetch",
"url i need to fetch"
];
Promise
.all( urls.map( url => fetch( url)))
.then( responses => responses.map( response => response.json()))
.then( results =>
// do something with the results array
)
.catch( error =>
// handle the error
);
You can use Promise.all() to run all requests at the same time:
const urls = [
"url i need to fetch",
"url i need to fetch",
"url i need to fetch"
];
Promise
.all( urls.map( url => fetch( url)))
.then( responses => responses.map( response => response.json()))
.then( results =>
// do something with the results array
)
.catch( error =>
// handle the error
);
answered Nov 15 '18 at 15:29
ShillyShilly
5,8331717
5,8331717
add a comment |
add a comment |
You can use a for loop and use let
which creates a block level scope
var urls = ["url1", "url2", "url3"];
for (let i = 0; i < urls.length; i++)
fetch(urls[i])
.then(result => result.json())
.then((res) =>
console.log(res);
createCards(res);
)
.catch(err => console.log(err))
function createCards(card)
var div = document.getElementById('card');
div.innerHTML += `<h2>$card.name</h2>
<div>
<b>HTML text </b>$someOtherText</div>`
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
div.innerHTML += ....
, else only the last result to arrive will be rendered.
– Shilly
Nov 15 '18 at 15:34
add a comment |
You can use a for loop and use let
which creates a block level scope
var urls = ["url1", "url2", "url3"];
for (let i = 0; i < urls.length; i++)
fetch(urls[i])
.then(result => result.json())
.then((res) =>
console.log(res);
createCards(res);
)
.catch(err => console.log(err))
function createCards(card)
var div = document.getElementById('card');
div.innerHTML += `<h2>$card.name</h2>
<div>
<b>HTML text </b>$someOtherText</div>`
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
div.innerHTML += ....
, else only the last result to arrive will be rendered.
– Shilly
Nov 15 '18 at 15:34
add a comment |
You can use a for loop and use let
which creates a block level scope
var urls = ["url1", "url2", "url3"];
for (let i = 0; i < urls.length; i++)
fetch(urls[i])
.then(result => result.json())
.then((res) =>
console.log(res);
createCards(res);
)
.catch(err => console.log(err))
function createCards(card)
var div = document.getElementById('card');
div.innerHTML += `<h2>$card.name</h2>
<div>
<b>HTML text </b>$someOtherText</div>`
You can use a for loop and use let
which creates a block level scope
var urls = ["url1", "url2", "url3"];
for (let i = 0; i < urls.length; i++)
fetch(urls[i])
.then(result => result.json())
.then((res) =>
console.log(res);
createCards(res);
)
.catch(err => console.log(err))
function createCards(card)
var div = document.getElementById('card');
div.innerHTML += `<h2>$card.name</h2>
<div>
<b>HTML text </b>$someOtherText</div>`
edited Nov 15 '18 at 15:34
answered Nov 15 '18 at 15:31
brkbrk
29.1k32243
29.1k32243
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
div.innerHTML += ....
, else only the last result to arrive will be rendered.
– Shilly
Nov 15 '18 at 15:34
add a comment |
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
div.innerHTML += ....
, else only the last result to arrive will be rendered.
– Shilly
Nov 15 '18 at 15:34
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
Should mention that the order of output may not be same as order of url array
– charlietfl
Nov 15 '18 at 15:33
div.innerHTML += ....
, else only the last result to arrive will be rendered.– Shilly
Nov 15 '18 at 15:34
div.innerHTML += ....
, else only the last result to arrive will be rendered.– Shilly
Nov 15 '18 at 15:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53322611%2ffetch-with-array-and-for-loop%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pUN Gp6,oYKG,7OTSFDkMRQ5rAYZbdII,UPxgf7 A8qCaDOXirGwa8eotADrV,W8OG4Cai
Can you use async/await?
– ODYN-Kon
Nov 15 '18 at 15:23
Have not used that before, so the best is if someone can help me with how Im gonna fetch it all with a for loop
– KX0
Nov 15 '18 at 15:24