How do I get the api_version parameter from a request in node.js?
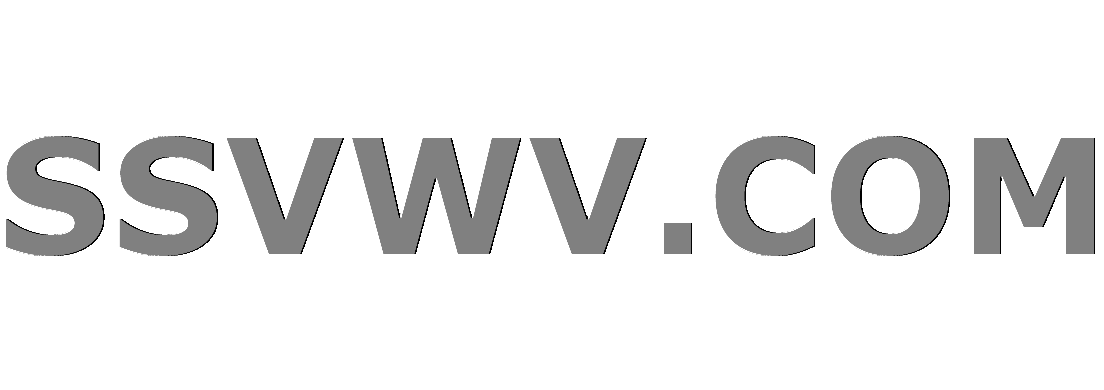
Multi tool use
Here is the request I am sending in Swift:
func createCustomerKey(withAPIVersion apiVersion: String, completion: @escaping STPJSONResponseCompletionBlock)
let url = self.baseURL.appendingPathComponent("ephemeral_keys")
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post, parameters: params)
.validate(statusCode: 200..<300)
.responseJSON responseJSON in
switch responseJSON.result
case .success(let json):
completion(json as? [String: AnyObject], nil)
case .failure(let error):
completion(nil, error)
Here is the cloud function I'm trying to execute. "api_version" is passed as a parameter, but api_version is undefined in the firebase logs. How do I get the api_version? What am I doing wrong?
const functions = require('firebase-functions');
const admin = require('firebase-admin');
const stripe = require('stripe')("sk_test_...");
stripe.setApiVersion('2018-11-08');
admin.initializeApp(functions.config().firebase);
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.api_version;
if (!stripe_version)
console.log('error with stripe version')
console.log(stripe_version)
res.status(400).end();
return;
const uid = req.params.uid;
const user = admin.firestore().collection('users').doc(uid);
console.log('Customer id ' + user.customerId);
stripe.ephemeralKeys.create(
customer: user.customerId,
stripe_version: stripe_version
).then((key) =>
console.log('Ephemeral key ' + key);
res.status(200).json(key);
).catch((err) =>
res.status(500).end();
);
);
node.js firebase google-cloud-functions
add a comment |
Here is the request I am sending in Swift:
func createCustomerKey(withAPIVersion apiVersion: String, completion: @escaping STPJSONResponseCompletionBlock)
let url = self.baseURL.appendingPathComponent("ephemeral_keys")
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post, parameters: params)
.validate(statusCode: 200..<300)
.responseJSON responseJSON in
switch responseJSON.result
case .success(let json):
completion(json as? [String: AnyObject], nil)
case .failure(let error):
completion(nil, error)
Here is the cloud function I'm trying to execute. "api_version" is passed as a parameter, but api_version is undefined in the firebase logs. How do I get the api_version? What am I doing wrong?
const functions = require('firebase-functions');
const admin = require('firebase-admin');
const stripe = require('stripe')("sk_test_...");
stripe.setApiVersion('2018-11-08');
admin.initializeApp(functions.config().firebase);
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.api_version;
if (!stripe_version)
console.log('error with stripe version')
console.log(stripe_version)
res.status(400).end();
return;
const uid = req.params.uid;
const user = admin.firestore().collection('users').doc(uid);
console.log('Customer id ' + user.customerId);
stripe.ephemeralKeys.create(
customer: user.customerId,
stripe_version: stripe_version
).then((key) =>
console.log('Ephemeral key ' + key);
res.status(200).json(key);
).catch((err) =>
res.status(500).end();
);
);
node.js firebase google-cloud-functions
add a comment |
Here is the request I am sending in Swift:
func createCustomerKey(withAPIVersion apiVersion: String, completion: @escaping STPJSONResponseCompletionBlock)
let url = self.baseURL.appendingPathComponent("ephemeral_keys")
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post, parameters: params)
.validate(statusCode: 200..<300)
.responseJSON responseJSON in
switch responseJSON.result
case .success(let json):
completion(json as? [String: AnyObject], nil)
case .failure(let error):
completion(nil, error)
Here is the cloud function I'm trying to execute. "api_version" is passed as a parameter, but api_version is undefined in the firebase logs. How do I get the api_version? What am I doing wrong?
const functions = require('firebase-functions');
const admin = require('firebase-admin');
const stripe = require('stripe')("sk_test_...");
stripe.setApiVersion('2018-11-08');
admin.initializeApp(functions.config().firebase);
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.api_version;
if (!stripe_version)
console.log('error with stripe version')
console.log(stripe_version)
res.status(400).end();
return;
const uid = req.params.uid;
const user = admin.firestore().collection('users').doc(uid);
console.log('Customer id ' + user.customerId);
stripe.ephemeralKeys.create(
customer: user.customerId,
stripe_version: stripe_version
).then((key) =>
console.log('Ephemeral key ' + key);
res.status(200).json(key);
).catch((err) =>
res.status(500).end();
);
);
node.js firebase google-cloud-functions
Here is the request I am sending in Swift:
func createCustomerKey(withAPIVersion apiVersion: String, completion: @escaping STPJSONResponseCompletionBlock)
let url = self.baseURL.appendingPathComponent("ephemeral_keys")
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post, parameters: params)
.validate(statusCode: 200..<300)
.responseJSON responseJSON in
switch responseJSON.result
case .success(let json):
completion(json as? [String: AnyObject], nil)
case .failure(let error):
completion(nil, error)
Here is the cloud function I'm trying to execute. "api_version" is passed as a parameter, but api_version is undefined in the firebase logs. How do I get the api_version? What am I doing wrong?
const functions = require('firebase-functions');
const admin = require('firebase-admin');
const stripe = require('stripe')("sk_test_...");
stripe.setApiVersion('2018-11-08');
admin.initializeApp(functions.config().firebase);
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.api_version;
if (!stripe_version)
console.log('error with stripe version')
console.log(stripe_version)
res.status(400).end();
return;
const uid = req.params.uid;
const user = admin.firestore().collection('users').doc(uid);
console.log('Customer id ' + user.customerId);
stripe.ephemeralKeys.create(
customer: user.customerId,
stripe_version: stripe_version
).then((key) =>
console.log('Ephemeral key ' + key);
res.status(200).json(key);
).catch((err) =>
res.status(500).end();
);
);
node.js firebase google-cloud-functions
node.js firebase google-cloud-functions
asked Nov 15 '18 at 0:33
p3scobarp3scobar
174
174
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I don't know much about Alamofire, but from reading a bit about it and searching google, what you can do is this:
Option 1
The best convention is shooting out your request using a URL similar to this:
http://server-host-name:<port>/someName/api_version/2.0/getDataApi
And in the node server side do this:
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.query.api_version;
// Do what ever you want with it...
// ...
Option 2
Using api_version as a query string parameter, so in your case i think you are doing it wrong, try this, in client side code:
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post,
parameters: params,
encoding: URLEncoding(destination: .queryString))
This is in order to tell Alamofire to encode the parameters as a query string parameters (more about this read this Stackoverflow post)
Option 3
Or put the api version in a custom HTTP header, like this:
let headers: HTTPHeaders = [
"api_version": MY_API_KEY,
"Accept": "application/json"
]
Alamofire.request(yourURL, headers: headers)
.responseJSON response in
debugPrint(response)
And try to exctract it in the node server side like this:
const stripe_version = req.headers.api_version;
BTW my best choice would be option 1 (best complies with REST API conventions)
(Not tested)
check and let us know if this worked for you!
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310815%2fhow-do-i-get-the-api-version-parameter-from-a-request-in-node-js%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I don't know much about Alamofire, but from reading a bit about it and searching google, what you can do is this:
Option 1
The best convention is shooting out your request using a URL similar to this:
http://server-host-name:<port>/someName/api_version/2.0/getDataApi
And in the node server side do this:
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.query.api_version;
// Do what ever you want with it...
// ...
Option 2
Using api_version as a query string parameter, so in your case i think you are doing it wrong, try this, in client side code:
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post,
parameters: params,
encoding: URLEncoding(destination: .queryString))
This is in order to tell Alamofire to encode the parameters as a query string parameters (more about this read this Stackoverflow post)
Option 3
Or put the api version in a custom HTTP header, like this:
let headers: HTTPHeaders = [
"api_version": MY_API_KEY,
"Accept": "application/json"
]
Alamofire.request(yourURL, headers: headers)
.responseJSON response in
debugPrint(response)
And try to exctract it in the node server side like this:
const stripe_version = req.headers.api_version;
BTW my best choice would be option 1 (best complies with REST API conventions)
(Not tested)
check and let us know if this worked for you!
add a comment |
I don't know much about Alamofire, but from reading a bit about it and searching google, what you can do is this:
Option 1
The best convention is shooting out your request using a URL similar to this:
http://server-host-name:<port>/someName/api_version/2.0/getDataApi
And in the node server side do this:
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.query.api_version;
// Do what ever you want with it...
// ...
Option 2
Using api_version as a query string parameter, so in your case i think you are doing it wrong, try this, in client side code:
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post,
parameters: params,
encoding: URLEncoding(destination: .queryString))
This is in order to tell Alamofire to encode the parameters as a query string parameters (more about this read this Stackoverflow post)
Option 3
Or put the api version in a custom HTTP header, like this:
let headers: HTTPHeaders = [
"api_version": MY_API_KEY,
"Accept": "application/json"
]
Alamofire.request(yourURL, headers: headers)
.responseJSON response in
debugPrint(response)
And try to exctract it in the node server side like this:
const stripe_version = req.headers.api_version;
BTW my best choice would be option 1 (best complies with REST API conventions)
(Not tested)
check and let us know if this worked for you!
add a comment |
I don't know much about Alamofire, but from reading a bit about it and searching google, what you can do is this:
Option 1
The best convention is shooting out your request using a URL similar to this:
http://server-host-name:<port>/someName/api_version/2.0/getDataApi
And in the node server side do this:
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.query.api_version;
// Do what ever you want with it...
// ...
Option 2
Using api_version as a query string parameter, so in your case i think you are doing it wrong, try this, in client side code:
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post,
parameters: params,
encoding: URLEncoding(destination: .queryString))
This is in order to tell Alamofire to encode the parameters as a query string parameters (more about this read this Stackoverflow post)
Option 3
Or put the api version in a custom HTTP header, like this:
let headers: HTTPHeaders = [
"api_version": MY_API_KEY,
"Accept": "application/json"
]
Alamofire.request(yourURL, headers: headers)
.responseJSON response in
debugPrint(response)
And try to exctract it in the node server side like this:
const stripe_version = req.headers.api_version;
BTW my best choice would be option 1 (best complies with REST API conventions)
(Not tested)
check and let us know if this worked for you!
I don't know much about Alamofire, but from reading a bit about it and searching google, what you can do is this:
Option 1
The best convention is shooting out your request using a URL similar to this:
http://server-host-name:<port>/someName/api_version/2.0/getDataApi
And in the node server side do this:
exports.ephemeral_keys = functions.https.onRequest((req, res) =>
const stripe_version = req.query.api_version;
// Do what ever you want with it...
// ...
Option 2
Using api_version as a query string parameter, so in your case i think you are doing it wrong, try this, in client side code:
let params: Parameters = [
"api_version":apiVersion,
"uid":Model.shared.uid
]
Alamofire.request(url, method: .post,
parameters: params,
encoding: URLEncoding(destination: .queryString))
This is in order to tell Alamofire to encode the parameters as a query string parameters (more about this read this Stackoverflow post)
Option 3
Or put the api version in a custom HTTP header, like this:
let headers: HTTPHeaders = [
"api_version": MY_API_KEY,
"Accept": "application/json"
]
Alamofire.request(yourURL, headers: headers)
.responseJSON response in
debugPrint(response)
And try to exctract it in the node server side like this:
const stripe_version = req.headers.api_version;
BTW my best choice would be option 1 (best complies with REST API conventions)
(Not tested)
check and let us know if this worked for you!
edited Nov 19 '18 at 7:33
answered Nov 15 '18 at 14:19


MercuryMercury
2,7732128
2,7732128
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53310815%2fhow-do-i-get-the-api-version-parameter-from-a-request-in-node-js%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MTfGPI5e,c,l,AD,hPVhPSiao6AmzE59