PHP/Laravel: Why this function is being recalled?
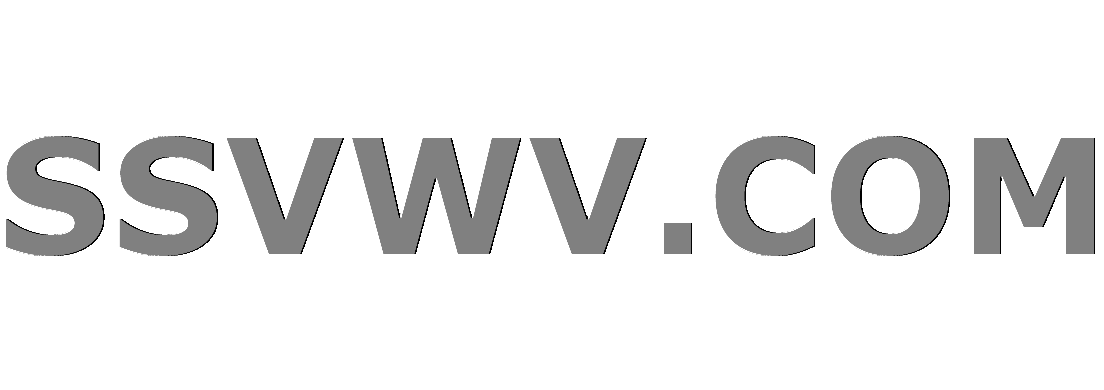
Multi tool use
I would like to understand why this function is being called twice in laravel, I have this Factory defined:
<?php
use FakerGenerator as Faker;
function my_callback (Faker $faker) // <= LINE 5
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
// <= LINE 29
$factory->define(AppBien::class, 'my_callback');
then, i have the following tests:
<?php
namespace TestsUnit;
use TestsTestCase;
use IlluminateFoundationTestingWithFaker;
use IlluminateFoundationTestingRefreshDatabase;
use FakerFactory as Faker;
class BienTest extends TestCase
{
use RefreshDatabase;
public function test__actualizar()
// setup
$registro_a_actualizar = factory('AppBien')->create(['id' => 1]);
// more code...
public function test__destruir()
// setup
$registro_a_destruir = factory('AppBien')->create(['id' => 1]);
// some code...
when I execute
phpunit
i get the following error
Fatal error: Cannot redeclare my_callback()
(previously declared in invendatabasefactoriesFactoryBien.php:5)
in invendatabasefactoriesFactoryBien.php on line 29
thanks if you can explain me: why do the function my_callback()
is being called more than once?
php laravel function callback
add a comment |
I would like to understand why this function is being called twice in laravel, I have this Factory defined:
<?php
use FakerGenerator as Faker;
function my_callback (Faker $faker) // <= LINE 5
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
// <= LINE 29
$factory->define(AppBien::class, 'my_callback');
then, i have the following tests:
<?php
namespace TestsUnit;
use TestsTestCase;
use IlluminateFoundationTestingWithFaker;
use IlluminateFoundationTestingRefreshDatabase;
use FakerFactory as Faker;
class BienTest extends TestCase
{
use RefreshDatabase;
public function test__actualizar()
// setup
$registro_a_actualizar = factory('AppBien')->create(['id' => 1]);
// more code...
public function test__destruir()
// setup
$registro_a_destruir = factory('AppBien')->create(['id' => 1]);
// some code...
when I execute
phpunit
i get the following error
Fatal error: Cannot redeclare my_callback()
(previously declared in invendatabasefactoriesFactoryBien.php:5)
in invendatabasefactoriesFactoryBien.php on line 29
thanks if you can explain me: why do the function my_callback()
is being called more than once?
php laravel function callback
add a comment |
I would like to understand why this function is being called twice in laravel, I have this Factory defined:
<?php
use FakerGenerator as Faker;
function my_callback (Faker $faker) // <= LINE 5
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
// <= LINE 29
$factory->define(AppBien::class, 'my_callback');
then, i have the following tests:
<?php
namespace TestsUnit;
use TestsTestCase;
use IlluminateFoundationTestingWithFaker;
use IlluminateFoundationTestingRefreshDatabase;
use FakerFactory as Faker;
class BienTest extends TestCase
{
use RefreshDatabase;
public function test__actualizar()
// setup
$registro_a_actualizar = factory('AppBien')->create(['id' => 1]);
// more code...
public function test__destruir()
// setup
$registro_a_destruir = factory('AppBien')->create(['id' => 1]);
// some code...
when I execute
phpunit
i get the following error
Fatal error: Cannot redeclare my_callback()
(previously declared in invendatabasefactoriesFactoryBien.php:5)
in invendatabasefactoriesFactoryBien.php on line 29
thanks if you can explain me: why do the function my_callback()
is being called more than once?
php laravel function callback
I would like to understand why this function is being called twice in laravel, I have this Factory defined:
<?php
use FakerGenerator as Faker;
function my_callback (Faker $faker) // <= LINE 5
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
// <= LINE 29
$factory->define(AppBien::class, 'my_callback');
then, i have the following tests:
<?php
namespace TestsUnit;
use TestsTestCase;
use IlluminateFoundationTestingWithFaker;
use IlluminateFoundationTestingRefreshDatabase;
use FakerFactory as Faker;
class BienTest extends TestCase
{
use RefreshDatabase;
public function test__actualizar()
// setup
$registro_a_actualizar = factory('AppBien')->create(['id' => 1]);
// more code...
public function test__destruir()
// setup
$registro_a_destruir = factory('AppBien')->create(['id' => 1]);
// some code...
when I execute
phpunit
i get the following error
Fatal error: Cannot redeclare my_callback()
(previously declared in invendatabasefactoriesFactoryBien.php:5)
in invendatabasefactoriesFactoryBien.php on line 29
thanks if you can explain me: why do the function my_callback()
is being called more than once?
php laravel function callback
php laravel function callback
asked Nov 14 '18 at 20:27


byron perezbyron perez
155
155
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
First, we have to understand how the test runner works.
Even though every test is run in what looks like a random order, that's not truly the case. Also, you have to understand that setUp
and tearDown
are always run before and after every test.
In the setUp
method in Laravel, it'll refresh the application if the app is not running :
if (! $this->app)
$this->refreshApplication();
So we might think hey, we're keeping one instance of the app running, so we shouldn't require ModelFactory
again, right ?
WRONG.
In the tearDown
method that's called after every test, it'll actually destroy the app right before the end of the method :
if ($this->app)
foreach ($this->beforeApplicationDestroyedCallbacks as $callback)
call_user_func($callback);
$this->app->flush();
$this->app = null;
That means your ModelFactory
file is included every single time a test is run. But this happens in the same PHP process. This is why you get an already defined function error.
To prove this is easy, simply write this in your ModelFactory
file :
global $a;
if (is_null($a))
$a = 0;
else
$a++;
var_dump($a);
You'll see $a
incrementing on each test running.
To fix your problem, you should simply use anonymous functions like so :
$factory->define(AppBien::class, function (Faker $faker)
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
);
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53308246%2fphp-laravel-why-this-function-is-being-recalled%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
First, we have to understand how the test runner works.
Even though every test is run in what looks like a random order, that's not truly the case. Also, you have to understand that setUp
and tearDown
are always run before and after every test.
In the setUp
method in Laravel, it'll refresh the application if the app is not running :
if (! $this->app)
$this->refreshApplication();
So we might think hey, we're keeping one instance of the app running, so we shouldn't require ModelFactory
again, right ?
WRONG.
In the tearDown
method that's called after every test, it'll actually destroy the app right before the end of the method :
if ($this->app)
foreach ($this->beforeApplicationDestroyedCallbacks as $callback)
call_user_func($callback);
$this->app->flush();
$this->app = null;
That means your ModelFactory
file is included every single time a test is run. But this happens in the same PHP process. This is why you get an already defined function error.
To prove this is easy, simply write this in your ModelFactory
file :
global $a;
if (is_null($a))
$a = 0;
else
$a++;
var_dump($a);
You'll see $a
incrementing on each test running.
To fix your problem, you should simply use anonymous functions like so :
$factory->define(AppBien::class, function (Faker $faker)
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
);
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
add a comment |
First, we have to understand how the test runner works.
Even though every test is run in what looks like a random order, that's not truly the case. Also, you have to understand that setUp
and tearDown
are always run before and after every test.
In the setUp
method in Laravel, it'll refresh the application if the app is not running :
if (! $this->app)
$this->refreshApplication();
So we might think hey, we're keeping one instance of the app running, so we shouldn't require ModelFactory
again, right ?
WRONG.
In the tearDown
method that's called after every test, it'll actually destroy the app right before the end of the method :
if ($this->app)
foreach ($this->beforeApplicationDestroyedCallbacks as $callback)
call_user_func($callback);
$this->app->flush();
$this->app = null;
That means your ModelFactory
file is included every single time a test is run. But this happens in the same PHP process. This is why you get an already defined function error.
To prove this is easy, simply write this in your ModelFactory
file :
global $a;
if (is_null($a))
$a = 0;
else
$a++;
var_dump($a);
You'll see $a
incrementing on each test running.
To fix your problem, you should simply use anonymous functions like so :
$factory->define(AppBien::class, function (Faker $faker)
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
);
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
add a comment |
First, we have to understand how the test runner works.
Even though every test is run in what looks like a random order, that's not truly the case. Also, you have to understand that setUp
and tearDown
are always run before and after every test.
In the setUp
method in Laravel, it'll refresh the application if the app is not running :
if (! $this->app)
$this->refreshApplication();
So we might think hey, we're keeping one instance of the app running, so we shouldn't require ModelFactory
again, right ?
WRONG.
In the tearDown
method that's called after every test, it'll actually destroy the app right before the end of the method :
if ($this->app)
foreach ($this->beforeApplicationDestroyedCallbacks as $callback)
call_user_func($callback);
$this->app->flush();
$this->app = null;
That means your ModelFactory
file is included every single time a test is run. But this happens in the same PHP process. This is why you get an already defined function error.
To prove this is easy, simply write this in your ModelFactory
file :
global $a;
if (is_null($a))
$a = 0;
else
$a++;
var_dump($a);
You'll see $a
incrementing on each test running.
To fix your problem, you should simply use anonymous functions like so :
$factory->define(AppBien::class, function (Faker $faker)
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
);
First, we have to understand how the test runner works.
Even though every test is run in what looks like a random order, that's not truly the case. Also, you have to understand that setUp
and tearDown
are always run before and after every test.
In the setUp
method in Laravel, it'll refresh the application if the app is not running :
if (! $this->app)
$this->refreshApplication();
So we might think hey, we're keeping one instance of the app running, so we shouldn't require ModelFactory
again, right ?
WRONG.
In the tearDown
method that's called after every test, it'll actually destroy the app right before the end of the method :
if ($this->app)
foreach ($this->beforeApplicationDestroyedCallbacks as $callback)
call_user_func($callback);
$this->app->flush();
$this->app = null;
That means your ModelFactory
file is included every single time a test is run. But this happens in the same PHP process. This is why you get an already defined function error.
To prove this is easy, simply write this in your ModelFactory
file :
global $a;
if (is_null($a))
$a = 0;
else
$a++;
var_dump($a);
You'll see $a
incrementing on each test running.
To fix your problem, you should simply use anonymous functions like so :
$factory->define(AppBien::class, function (Faker $faker)
return [
// some key-value pairs, don't focus on that
'id_ubicacion' => 1,
'nombre' => $faker->name,
'clase' => 'CONTROL ADMINISTRATIVO',
'codigo' => $faker->ean13,
// some other key-value pairs
];
);
answered Nov 14 '18 at 22:04
Steve ChamaillardSteve Chamaillard
1,3031023
1,3031023
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
add a comment |
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
I shouldn't declare any function in factory files, should I?
– byron perez
Nov 15 '18 at 20:57
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
No you shouldn't !
– Steve Chamaillard
Nov 15 '18 at 21:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53308246%2fphp-laravel-why-this-function-is-being-recalled%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
x8qbnUqGNyK7uQM8k