Redux - Handling multiple simultaneous requests
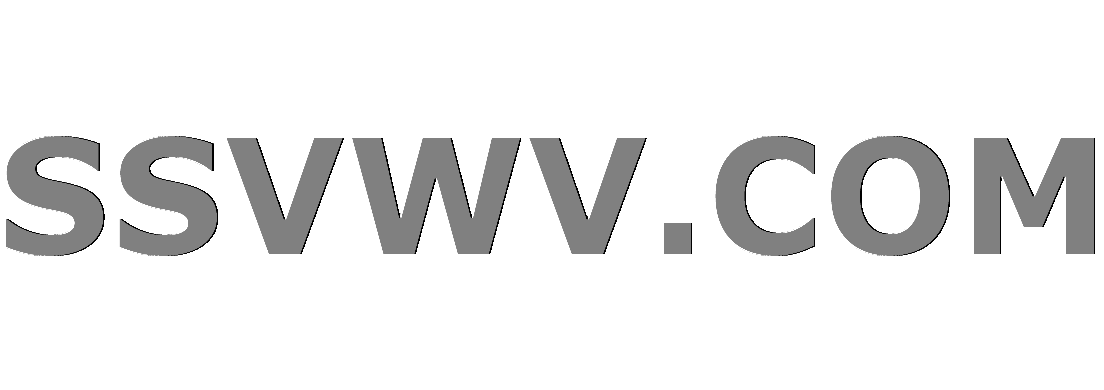
Multi tool use
In basically all Redux examples and documentations the example is shown when dealing with async requests that a boolean value, usually isFetching is toggled true when a request is made and false on success or fail.
When I load a page multiple API calls will be made from multiple components, I would like to show a loading indicator when any request to the API is being made. Therefore this might not be the best solution:
- Action1 toggles isFetching as true and starts fetching from API
- Action2 toggles isFetching as true and starts fetching from API
- Action1 is finished and turns isFetching false even though Action2 is still loading.
How would you solve these kind of situations?
Thanks!
redux request react-redux axios
add a comment |
In basically all Redux examples and documentations the example is shown when dealing with async requests that a boolean value, usually isFetching is toggled true when a request is made and false on success or fail.
When I load a page multiple API calls will be made from multiple components, I would like to show a loading indicator when any request to the API is being made. Therefore this might not be the best solution:
- Action1 toggles isFetching as true and starts fetching from API
- Action2 toggles isFetching as true and starts fetching from API
- Action1 is finished and turns isFetching false even though Action2 is still loading.
How would you solve these kind of situations?
Thanks!
redux request react-redux axios
add a comment |
In basically all Redux examples and documentations the example is shown when dealing with async requests that a boolean value, usually isFetching is toggled true when a request is made and false on success or fail.
When I load a page multiple API calls will be made from multiple components, I would like to show a loading indicator when any request to the API is being made. Therefore this might not be the best solution:
- Action1 toggles isFetching as true and starts fetching from API
- Action2 toggles isFetching as true and starts fetching from API
- Action1 is finished and turns isFetching false even though Action2 is still loading.
How would you solve these kind of situations?
Thanks!
redux request react-redux axios
In basically all Redux examples and documentations the example is shown when dealing with async requests that a boolean value, usually isFetching is toggled true when a request is made and false on success or fail.
When I load a page multiple API calls will be made from multiple components, I would like to show a loading indicator when any request to the API is being made. Therefore this might not be the best solution:
- Action1 toggles isFetching as true and starts fetching from API
- Action2 toggles isFetching as true and starts fetching from API
- Action1 is finished and turns isFetching false even though Action2 is still loading.
How would you solve these kind of situations?
Thanks!
redux request react-redux axios
redux request react-redux axios
asked Nov 14 '18 at 20:59
Eric VEric V
83
83
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Convert isFetching
to a number. Each pending call increments the number, while each success/failure decrements the number.
const reducer = (state = fetchingCounter: 0 , type ) =>
switch(type)
FETCHING_PENDING:
return fetchingCounter: state.fetchingCounter + 1 ;
FETCHING_DONE:
return fetchingCounter: state.fetchingCounter - 1 ;
return state;
;
Add a selector that converts the number to a boolean -
const isFetching = ( isFetching ) => !!isFetching
1
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
add a comment |
Hopefully within a couple months, you'll be able to handle this very easily using the new fetcher/createResource concepts in conjunction with Suspense. You can see a description of this in Andrew Clark's Concurrent Rendering in React presentation around minute 25. But that is a future feature (Suspense is already available, but only integrated with code-splitting, not yet integrated with API calls).
So, for now, you have two main options:
- use a counter that you increment/decrement as you start/end api calls (it looks like Ori Drori has just posted an answer with this approach)
- use multiple booleans -- one for each api call involved and show the loading indicator if any are true. The only reason to use this over a counter is if you want your loading indicator to be more specific (i.e. use the specific booleans to indicate in some way what is being loaded)
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53308623%2fredux-handling-multiple-simultaneous-requests%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Convert isFetching
to a number. Each pending call increments the number, while each success/failure decrements the number.
const reducer = (state = fetchingCounter: 0 , type ) =>
switch(type)
FETCHING_PENDING:
return fetchingCounter: state.fetchingCounter + 1 ;
FETCHING_DONE:
return fetchingCounter: state.fetchingCounter - 1 ;
return state;
;
Add a selector that converts the number to a boolean -
const isFetching = ( isFetching ) => !!isFetching
1
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
add a comment |
Convert isFetching
to a number. Each pending call increments the number, while each success/failure decrements the number.
const reducer = (state = fetchingCounter: 0 , type ) =>
switch(type)
FETCHING_PENDING:
return fetchingCounter: state.fetchingCounter + 1 ;
FETCHING_DONE:
return fetchingCounter: state.fetchingCounter - 1 ;
return state;
;
Add a selector that converts the number to a boolean -
const isFetching = ( isFetching ) => !!isFetching
1
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
add a comment |
Convert isFetching
to a number. Each pending call increments the number, while each success/failure decrements the number.
const reducer = (state = fetchingCounter: 0 , type ) =>
switch(type)
FETCHING_PENDING:
return fetchingCounter: state.fetchingCounter + 1 ;
FETCHING_DONE:
return fetchingCounter: state.fetchingCounter - 1 ;
return state;
;
Add a selector that converts the number to a boolean -
const isFetching = ( isFetching ) => !!isFetching
Convert isFetching
to a number. Each pending call increments the number, while each success/failure decrements the number.
const reducer = (state = fetchingCounter: 0 , type ) =>
switch(type)
FETCHING_PENDING:
return fetchingCounter: state.fetchingCounter + 1 ;
FETCHING_DONE:
return fetchingCounter: state.fetchingCounter - 1 ;
return state;
;
Add a selector that converts the number to a boolean -
const isFetching = ( isFetching ) => !!isFetching
answered Nov 14 '18 at 21:06


Ori DroriOri Drori
78.3k138494
78.3k138494
1
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
add a comment |
1
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
1
1
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
Thank you for your answer. I was thinking about storing an object of request IDs and then remove them when the request was done but I will probably use this approach.
– Eric V
Nov 14 '18 at 21:30
add a comment |
Hopefully within a couple months, you'll be able to handle this very easily using the new fetcher/createResource concepts in conjunction with Suspense. You can see a description of this in Andrew Clark's Concurrent Rendering in React presentation around minute 25. But that is a future feature (Suspense is already available, but only integrated with code-splitting, not yet integrated with API calls).
So, for now, you have two main options:
- use a counter that you increment/decrement as you start/end api calls (it looks like Ori Drori has just posted an answer with this approach)
- use multiple booleans -- one for each api call involved and show the loading indicator if any are true. The only reason to use this over a counter is if you want your loading indicator to be more specific (i.e. use the specific booleans to indicate in some way what is being loaded)
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
add a comment |
Hopefully within a couple months, you'll be able to handle this very easily using the new fetcher/createResource concepts in conjunction with Suspense. You can see a description of this in Andrew Clark's Concurrent Rendering in React presentation around minute 25. But that is a future feature (Suspense is already available, but only integrated with code-splitting, not yet integrated with API calls).
So, for now, you have two main options:
- use a counter that you increment/decrement as you start/end api calls (it looks like Ori Drori has just posted an answer with this approach)
- use multiple booleans -- one for each api call involved and show the loading indicator if any are true. The only reason to use this over a counter is if you want your loading indicator to be more specific (i.e. use the specific booleans to indicate in some way what is being loaded)
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
add a comment |
Hopefully within a couple months, you'll be able to handle this very easily using the new fetcher/createResource concepts in conjunction with Suspense. You can see a description of this in Andrew Clark's Concurrent Rendering in React presentation around minute 25. But that is a future feature (Suspense is already available, but only integrated with code-splitting, not yet integrated with API calls).
So, for now, you have two main options:
- use a counter that you increment/decrement as you start/end api calls (it looks like Ori Drori has just posted an answer with this approach)
- use multiple booleans -- one for each api call involved and show the loading indicator if any are true. The only reason to use this over a counter is if you want your loading indicator to be more specific (i.e. use the specific booleans to indicate in some way what is being loaded)
Hopefully within a couple months, you'll be able to handle this very easily using the new fetcher/createResource concepts in conjunction with Suspense. You can see a description of this in Andrew Clark's Concurrent Rendering in React presentation around minute 25. But that is a future feature (Suspense is already available, but only integrated with code-splitting, not yet integrated with API calls).
So, for now, you have two main options:
- use a counter that you increment/decrement as you start/end api calls (it looks like Ori Drori has just posted an answer with this approach)
- use multiple booleans -- one for each api call involved and show the loading indicator if any are true. The only reason to use this over a counter is if you want your loading indicator to be more specific (i.e. use the specific booleans to indicate in some way what is being loaded)
answered Nov 14 '18 at 21:18


Ryan CogswellRyan Cogswell
4,447421
4,447421
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
add a comment |
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
Thank you for the information, I hope the concepts you mentioned will be implemented soon since the counter approach feels kind of hacky.
– Eric V
Nov 14 '18 at 21:31
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
I know the react team is actively working on it, but I suspect it is at least a couple months away from being part of a production (non-alpha) release of react.
– Ryan Cogswell
Nov 14 '18 at 21:35
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
Will definitely follow the progress. A bonus question, I use Axios for API requests. Is it common praxis to wrap all requests in like an action or something similar? Just to perform common actions that always is performed when doing request, like increasing/decreasing the requestCount state variable?
– Eric V
Nov 15 '18 at 18:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53308623%2fredux-handling-multiple-simultaneous-requests%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7nQCJvVUpL5sn,6eKW5fJcSR jZOW13P,MBycbH