Inconsistency between server response size and client blob object size
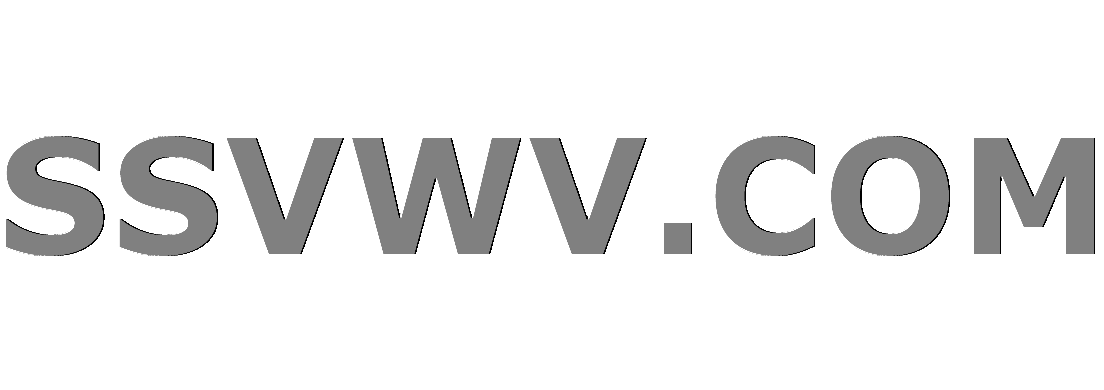
Multi tool use
I've an ASP.NET MVC application that in one page it has a button that when the user click the button in the web browser:
- the browser sends an AJAX POST to the server
- the server creates a ZIP archive using MemoryStream so there’s no physical file in the server
- the server returns the ZIP archive in the response’s body
- the browser gets the response body and creates a BLOB object which is downloaded by the user
Until step 3 everything is working as expected. I have used Fiddler to intercept the server’s response, I created manually a ZIP file with the bytes from the response, and it is OK.
When the user download the file from the web browser it is corrupt. I have realized that the size (length) of the file is bigger than the length of the response’s body.
Here’s the code that generates the response from the server
Response.AppendHeader("Content-Disposition", "attachment; filename=test.zip");
Response.AppendHeader("Content-Length", memoryStream.Length.ToString());
Response.AppendHeader("Content-Type", "application/zip; charset=utf-8");
Response.BinaryWrite(memoryStream.ToArray());
Response.End();
Here’s the code that create and download the file from the server’s response
saveZipFile = (zipFile: any /* zipFile is the server's response */) =>
if (!('Blob' in window))
alert('Your browser does not support the HTML5 Blob.');
return;
var fileName = "test.zip";
var textFileAsBlob = new Blob([zipFile], encoding: "UTF-8", type: "application/zip; charset=utf-8" as any);
if ('msSaveOrOpenBlob' in navigator)
navigator.msSaveOrOpenBlob(textFileAsBlob, fileName);
else
var downloadLink = document.createElement('a');
downloadLink.download = fileName;
downloadLink.innerHTML = 'Download File';
if ('webkitURL' in window)
// Chrome allows the link to be clicked without actually adding it to the DOM.
downloadLink.href = (window as any).webkitURL.createObjectURL(textFileAsBlob);
else
// Firefox requires the link to be added to the DOM before it can be clicked.
downloadLink.href = window.URL.createObjectURL(textFileAsBlob);
downloadLink.onclick = (event: any) =>
document.body.removeChild(event.target);
;
downloadLink.style.display = 'none';
document.body.appendChild(downloadLink);
downloadLink.click();
If you need more details, please let me know.
What can be wrong?
PD: I think it’s related to string encoding.
javascript c# .net asp.net-mvc html5
add a comment |
I've an ASP.NET MVC application that in one page it has a button that when the user click the button in the web browser:
- the browser sends an AJAX POST to the server
- the server creates a ZIP archive using MemoryStream so there’s no physical file in the server
- the server returns the ZIP archive in the response’s body
- the browser gets the response body and creates a BLOB object which is downloaded by the user
Until step 3 everything is working as expected. I have used Fiddler to intercept the server’s response, I created manually a ZIP file with the bytes from the response, and it is OK.
When the user download the file from the web browser it is corrupt. I have realized that the size (length) of the file is bigger than the length of the response’s body.
Here’s the code that generates the response from the server
Response.AppendHeader("Content-Disposition", "attachment; filename=test.zip");
Response.AppendHeader("Content-Length", memoryStream.Length.ToString());
Response.AppendHeader("Content-Type", "application/zip; charset=utf-8");
Response.BinaryWrite(memoryStream.ToArray());
Response.End();
Here’s the code that create and download the file from the server’s response
saveZipFile = (zipFile: any /* zipFile is the server's response */) =>
if (!('Blob' in window))
alert('Your browser does not support the HTML5 Blob.');
return;
var fileName = "test.zip";
var textFileAsBlob = new Blob([zipFile], encoding: "UTF-8", type: "application/zip; charset=utf-8" as any);
if ('msSaveOrOpenBlob' in navigator)
navigator.msSaveOrOpenBlob(textFileAsBlob, fileName);
else
var downloadLink = document.createElement('a');
downloadLink.download = fileName;
downloadLink.innerHTML = 'Download File';
if ('webkitURL' in window)
// Chrome allows the link to be clicked without actually adding it to the DOM.
downloadLink.href = (window as any).webkitURL.createObjectURL(textFileAsBlob);
else
// Firefox requires the link to be added to the DOM before it can be clicked.
downloadLink.href = window.URL.createObjectURL(textFileAsBlob);
downloadLink.onclick = (event: any) =>
document.body.removeChild(event.target);
;
downloadLink.style.display = 'none';
document.body.appendChild(downloadLink);
downloadLink.click();
If you need more details, please let me know.
What can be wrong?
PD: I think it’s related to string encoding.
javascript c# .net asp.net-mvc html5
I don't think you should do this Response.AppendHeader("Content-Length", memoryStream.Length.ToString()); The browser usually send that header automatically
– Enrique González
Nov 14 '18 at 20:08
add a comment |
I've an ASP.NET MVC application that in one page it has a button that when the user click the button in the web browser:
- the browser sends an AJAX POST to the server
- the server creates a ZIP archive using MemoryStream so there’s no physical file in the server
- the server returns the ZIP archive in the response’s body
- the browser gets the response body and creates a BLOB object which is downloaded by the user
Until step 3 everything is working as expected. I have used Fiddler to intercept the server’s response, I created manually a ZIP file with the bytes from the response, and it is OK.
When the user download the file from the web browser it is corrupt. I have realized that the size (length) of the file is bigger than the length of the response’s body.
Here’s the code that generates the response from the server
Response.AppendHeader("Content-Disposition", "attachment; filename=test.zip");
Response.AppendHeader("Content-Length", memoryStream.Length.ToString());
Response.AppendHeader("Content-Type", "application/zip; charset=utf-8");
Response.BinaryWrite(memoryStream.ToArray());
Response.End();
Here’s the code that create and download the file from the server’s response
saveZipFile = (zipFile: any /* zipFile is the server's response */) =>
if (!('Blob' in window))
alert('Your browser does not support the HTML5 Blob.');
return;
var fileName = "test.zip";
var textFileAsBlob = new Blob([zipFile], encoding: "UTF-8", type: "application/zip; charset=utf-8" as any);
if ('msSaveOrOpenBlob' in navigator)
navigator.msSaveOrOpenBlob(textFileAsBlob, fileName);
else
var downloadLink = document.createElement('a');
downloadLink.download = fileName;
downloadLink.innerHTML = 'Download File';
if ('webkitURL' in window)
// Chrome allows the link to be clicked without actually adding it to the DOM.
downloadLink.href = (window as any).webkitURL.createObjectURL(textFileAsBlob);
else
// Firefox requires the link to be added to the DOM before it can be clicked.
downloadLink.href = window.URL.createObjectURL(textFileAsBlob);
downloadLink.onclick = (event: any) =>
document.body.removeChild(event.target);
;
downloadLink.style.display = 'none';
document.body.appendChild(downloadLink);
downloadLink.click();
If you need more details, please let me know.
What can be wrong?
PD: I think it’s related to string encoding.
javascript c# .net asp.net-mvc html5
I've an ASP.NET MVC application that in one page it has a button that when the user click the button in the web browser:
- the browser sends an AJAX POST to the server
- the server creates a ZIP archive using MemoryStream so there’s no physical file in the server
- the server returns the ZIP archive in the response’s body
- the browser gets the response body and creates a BLOB object which is downloaded by the user
Until step 3 everything is working as expected. I have used Fiddler to intercept the server’s response, I created manually a ZIP file with the bytes from the response, and it is OK.
When the user download the file from the web browser it is corrupt. I have realized that the size (length) of the file is bigger than the length of the response’s body.
Here’s the code that generates the response from the server
Response.AppendHeader("Content-Disposition", "attachment; filename=test.zip");
Response.AppendHeader("Content-Length", memoryStream.Length.ToString());
Response.AppendHeader("Content-Type", "application/zip; charset=utf-8");
Response.BinaryWrite(memoryStream.ToArray());
Response.End();
Here’s the code that create and download the file from the server’s response
saveZipFile = (zipFile: any /* zipFile is the server's response */) =>
if (!('Blob' in window))
alert('Your browser does not support the HTML5 Blob.');
return;
var fileName = "test.zip";
var textFileAsBlob = new Blob([zipFile], encoding: "UTF-8", type: "application/zip; charset=utf-8" as any);
if ('msSaveOrOpenBlob' in navigator)
navigator.msSaveOrOpenBlob(textFileAsBlob, fileName);
else
var downloadLink = document.createElement('a');
downloadLink.download = fileName;
downloadLink.innerHTML = 'Download File';
if ('webkitURL' in window)
// Chrome allows the link to be clicked without actually adding it to the DOM.
downloadLink.href = (window as any).webkitURL.createObjectURL(textFileAsBlob);
else
// Firefox requires the link to be added to the DOM before it can be clicked.
downloadLink.href = window.URL.createObjectURL(textFileAsBlob);
downloadLink.onclick = (event: any) =>
document.body.removeChild(event.target);
;
downloadLink.style.display = 'none';
document.body.appendChild(downloadLink);
downloadLink.click();
If you need more details, please let me know.
What can be wrong?
PD: I think it’s related to string encoding.
javascript c# .net asp.net-mvc html5
javascript c# .net asp.net-mvc html5
asked Nov 14 '18 at 13:59
vcRobevcRobe
68131023
68131023
I don't think you should do this Response.AppendHeader("Content-Length", memoryStream.Length.ToString()); The browser usually send that header automatically
– Enrique González
Nov 14 '18 at 20:08
add a comment |
I don't think you should do this Response.AppendHeader("Content-Length", memoryStream.Length.ToString()); The browser usually send that header automatically
– Enrique González
Nov 14 '18 at 20:08
I don't think you should do this Response.AppendHeader("Content-Length", memoryStream.Length.ToString()); The browser usually send that header automatically
– Enrique González
Nov 14 '18 at 20:08
I don't think you should do this Response.AppendHeader("Content-Length", memoryStream.Length.ToString()); The browser usually send that header automatically
– Enrique González
Nov 14 '18 at 20:08
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301977%2finconsistency-between-server-response-size-and-client-blob-object-size%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301977%2finconsistency-between-server-response-size-and-client-blob-object-size%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0 HmqAjL4Nd5
I don't think you should do this Response.AppendHeader("Content-Length", memoryStream.Length.ToString()); The browser usually send that header automatically
– Enrique González
Nov 14 '18 at 20:08