How deserialize [JsonExtensionData] Dictionary with casting
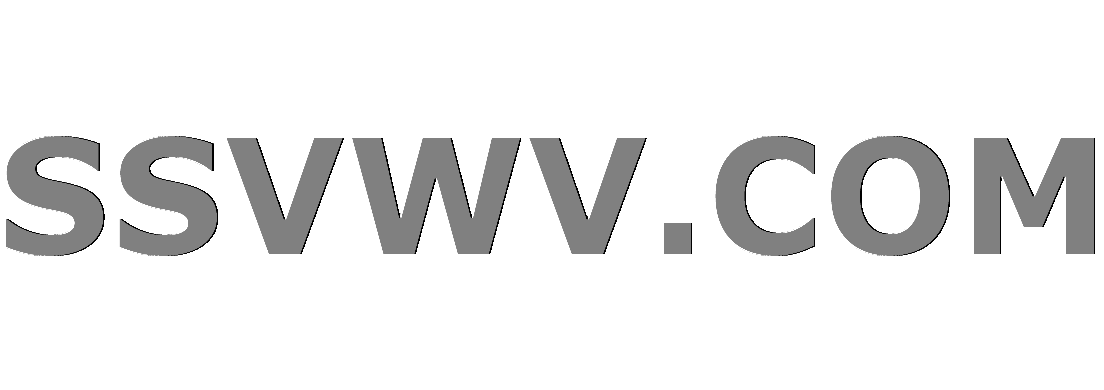
Multi tool use
[JsonExtensionData] allows you to do is to serialize elements of a JSON document which does not have matching properties on the destination object to the dictionary which is decorated with the [JsonExtensionData] attribute.
How to create a dictionary of objects cast into the appropriate type??
For example:
var json = "rn "sampleClass": "name":"Name" ,
"sampleOtherClass": "name":"OtherName" ,rn "X": "Jan"rn";
and
var result =JsonConvert.DeserializeObject<Test>(json);
and
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, object> Y get; set;
The dictionary should contain such elements:
Dictionary<string, object> students = new Dictionary<string, object>()
"sampleClass", new ClassName Name="MyName" ,
"sampleOtherClass", new SampleOtherClass Name="MyName"
;
Which means that for node sampleClass we want to create object SampleClass and next to add the dictionary Y.
Currently, the value in the DictionaryEntry is a string e.g. name: "Name"
The source:
https://dotnetfiddle.net/mhU6ME
Update:
Now I used the below approach to deserialize, but when I want to have one [JsonExtensionData] Dictionary to deserialize/serialize it brings problems to have two collections.
public class Class1
public string Code get; set;
public string Name get; set;
public bool IsActive get; set;
[JsonExtensionData]
public Dictionary<string, JToken> _JTokenProperty get; set;
public Dictionary<string, PropertiesClass> Properties1 get; set; = new Dictionary<string, PropertiesClass>();
c# json.net
|
show 1 more comment
[JsonExtensionData] allows you to do is to serialize elements of a JSON document which does not have matching properties on the destination object to the dictionary which is decorated with the [JsonExtensionData] attribute.
How to create a dictionary of objects cast into the appropriate type??
For example:
var json = "rn "sampleClass": "name":"Name" ,
"sampleOtherClass": "name":"OtherName" ,rn "X": "Jan"rn";
and
var result =JsonConvert.DeserializeObject<Test>(json);
and
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, object> Y get; set;
The dictionary should contain such elements:
Dictionary<string, object> students = new Dictionary<string, object>()
"sampleClass", new ClassName Name="MyName" ,
"sampleOtherClass", new SampleOtherClass Name="MyName"
;
Which means that for node sampleClass we want to create object SampleClass and next to add the dictionary Y.
Currently, the value in the DictionaryEntry is a string e.g. name: "Name"
The source:
https://dotnetfiddle.net/mhU6ME
Update:
Now I used the below approach to deserialize, but when I want to have one [JsonExtensionData] Dictionary to deserialize/serialize it brings problems to have two collections.
public class Class1
public string Code get; set;
public string Name get; set;
public bool IsActive get; set;
[JsonExtensionData]
public Dictionary<string, JToken> _JTokenProperty get; set;
public Dictionary<string, PropertiesClass> Properties1 get; set; = new Dictionary<string, PropertiesClass>();
c# json.net
Why do you have a data-structure storing instances that seem to have nothing in common in a single common map? But anyway: what is your problem exactly? What results do you get?
– HimBromBeere
Nov 14 '18 at 9:47
The reason is that the rest api returns user-defined objects.
– PiotrN
Nov 14 '18 at 9:50
Currently, the key and the string as value are created "key", "name: "Name"
– PiotrN
Nov 14 '18 at 9:55
I think you have to write your custom serializer and within that you hjave to tell Json.net of what type those properties are. I have made a quick search and it seems that here you find a simple example on how this may work. I have not testet this yet. stackoverflow.com/a/40439958/9809950
– Josef Biehler
Nov 14 '18 at 9:59
Possible duplicate of How to parse not fully corresponding json
– Adriani6
Nov 14 '18 at 10:02
|
show 1 more comment
[JsonExtensionData] allows you to do is to serialize elements of a JSON document which does not have matching properties on the destination object to the dictionary which is decorated with the [JsonExtensionData] attribute.
How to create a dictionary of objects cast into the appropriate type??
For example:
var json = "rn "sampleClass": "name":"Name" ,
"sampleOtherClass": "name":"OtherName" ,rn "X": "Jan"rn";
and
var result =JsonConvert.DeserializeObject<Test>(json);
and
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, object> Y get; set;
The dictionary should contain such elements:
Dictionary<string, object> students = new Dictionary<string, object>()
"sampleClass", new ClassName Name="MyName" ,
"sampleOtherClass", new SampleOtherClass Name="MyName"
;
Which means that for node sampleClass we want to create object SampleClass and next to add the dictionary Y.
Currently, the value in the DictionaryEntry is a string e.g. name: "Name"
The source:
https://dotnetfiddle.net/mhU6ME
Update:
Now I used the below approach to deserialize, but when I want to have one [JsonExtensionData] Dictionary to deserialize/serialize it brings problems to have two collections.
public class Class1
public string Code get; set;
public string Name get; set;
public bool IsActive get; set;
[JsonExtensionData]
public Dictionary<string, JToken> _JTokenProperty get; set;
public Dictionary<string, PropertiesClass> Properties1 get; set; = new Dictionary<string, PropertiesClass>();
c# json.net
[JsonExtensionData] allows you to do is to serialize elements of a JSON document which does not have matching properties on the destination object to the dictionary which is decorated with the [JsonExtensionData] attribute.
How to create a dictionary of objects cast into the appropriate type??
For example:
var json = "rn "sampleClass": "name":"Name" ,
"sampleOtherClass": "name":"OtherName" ,rn "X": "Jan"rn";
and
var result =JsonConvert.DeserializeObject<Test>(json);
and
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, object> Y get; set;
The dictionary should contain such elements:
Dictionary<string, object> students = new Dictionary<string, object>()
"sampleClass", new ClassName Name="MyName" ,
"sampleOtherClass", new SampleOtherClass Name="MyName"
;
Which means that for node sampleClass we want to create object SampleClass and next to add the dictionary Y.
Currently, the value in the DictionaryEntry is a string e.g. name: "Name"
The source:
https://dotnetfiddle.net/mhU6ME
Update:
Now I used the below approach to deserialize, but when I want to have one [JsonExtensionData] Dictionary to deserialize/serialize it brings problems to have two collections.
public class Class1
public string Code get; set;
public string Name get; set;
public bool IsActive get; set;
[JsonExtensionData]
public Dictionary<string, JToken> _JTokenProperty get; set;
public Dictionary<string, PropertiesClass> Properties1 get; set; = new Dictionary<string, PropertiesClass>();
c# json.net
c# json.net
edited Nov 15 '18 at 10:30
PiotrN
asked Nov 14 '18 at 9:45


PiotrNPiotrN
11
11
Why do you have a data-structure storing instances that seem to have nothing in common in a single common map? But anyway: what is your problem exactly? What results do you get?
– HimBromBeere
Nov 14 '18 at 9:47
The reason is that the rest api returns user-defined objects.
– PiotrN
Nov 14 '18 at 9:50
Currently, the key and the string as value are created "key", "name: "Name"
– PiotrN
Nov 14 '18 at 9:55
I think you have to write your custom serializer and within that you hjave to tell Json.net of what type those properties are. I have made a quick search and it seems that here you find a simple example on how this may work. I have not testet this yet. stackoverflow.com/a/40439958/9809950
– Josef Biehler
Nov 14 '18 at 9:59
Possible duplicate of How to parse not fully corresponding json
– Adriani6
Nov 14 '18 at 10:02
|
show 1 more comment
Why do you have a data-structure storing instances that seem to have nothing in common in a single common map? But anyway: what is your problem exactly? What results do you get?
– HimBromBeere
Nov 14 '18 at 9:47
The reason is that the rest api returns user-defined objects.
– PiotrN
Nov 14 '18 at 9:50
Currently, the key and the string as value are created "key", "name: "Name"
– PiotrN
Nov 14 '18 at 9:55
I think you have to write your custom serializer and within that you hjave to tell Json.net of what type those properties are. I have made a quick search and it seems that here you find a simple example on how this may work. I have not testet this yet. stackoverflow.com/a/40439958/9809950
– Josef Biehler
Nov 14 '18 at 9:59
Possible duplicate of How to parse not fully corresponding json
– Adriani6
Nov 14 '18 at 10:02
Why do you have a data-structure storing instances that seem to have nothing in common in a single common map? But anyway: what is your problem exactly? What results do you get?
– HimBromBeere
Nov 14 '18 at 9:47
Why do you have a data-structure storing instances that seem to have nothing in common in a single common map? But anyway: what is your problem exactly? What results do you get?
– HimBromBeere
Nov 14 '18 at 9:47
The reason is that the rest api returns user-defined objects.
– PiotrN
Nov 14 '18 at 9:50
The reason is that the rest api returns user-defined objects.
– PiotrN
Nov 14 '18 at 9:50
Currently, the key and the string as value are created "key", "name: "Name"
– PiotrN
Nov 14 '18 at 9:55
Currently, the key and the string as value are created "key", "name: "Name"
– PiotrN
Nov 14 '18 at 9:55
I think you have to write your custom serializer and within that you hjave to tell Json.net of what type those properties are. I have made a quick search and it seems that here you find a simple example on how this may work. I have not testet this yet. stackoverflow.com/a/40439958/9809950
– Josef Biehler
Nov 14 '18 at 9:59
I think you have to write your custom serializer and within that you hjave to tell Json.net of what type those properties are. I have made a quick search and it seems that here you find a simple example on how this may work. I have not testet this yet. stackoverflow.com/a/40439958/9809950
– Josef Biehler
Nov 14 '18 at 9:59
Possible duplicate of How to parse not fully corresponding json
– Adriani6
Nov 14 '18 at 10:02
Possible duplicate of How to parse not fully corresponding json
– Adriani6
Nov 14 '18 at 10:02
|
show 1 more comment
2 Answers
2
active
oldest
votes
how about if you try the dynamic instance instead of object.
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, dynamic> Y get; set;
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
add a comment |
Json.Net has the ability to (de)serialize objects specifying the actual type of the object.
In the serialization process a property "$type"
for storing the Type is added to the resulting JSON. Then in deserialization this property is checked to obtain the actual Type.
Of course it must be a Type which inherits/implements the declared Type in you class.
Since you're using object
, you shouldn't have problems.
You can activate this feature by setting the TypeNameHandling
property of the JsonSerializerSettings
to Auto
, which activates this behaviour when the object type is different from the declared type.
https://www.newtonsoft.com/json/help/html/SerializationSettings.htm#TypeNameHandling
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
1
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your ownJsonConverter
and place your custom logic into theReadJson
method.
– Matteo Melli
Nov 14 '18 at 15:05
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53297152%2fhow-deserialize-jsonextensiondata-dictionarystring-object-with-casting%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
how about if you try the dynamic instance instead of object.
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, dynamic> Y get; set;
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
add a comment |
how about if you try the dynamic instance instead of object.
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, dynamic> Y get; set;
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
add a comment |
how about if you try the dynamic instance instead of object.
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, dynamic> Y get; set;
how about if you try the dynamic instance instead of object.
public class Test
public string X get; set;
[JsonExtensionData]
public Dictionary<string, dynamic> Y get; set;
answered Nov 14 '18 at 10:08
zetawarszetawars
442212
442212
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
add a comment |
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
In my opinion that does not give us possibility to cast.
– PiotrN
Nov 14 '18 at 10:16
add a comment |
Json.Net has the ability to (de)serialize objects specifying the actual type of the object.
In the serialization process a property "$type"
for storing the Type is added to the resulting JSON. Then in deserialization this property is checked to obtain the actual Type.
Of course it must be a Type which inherits/implements the declared Type in you class.
Since you're using object
, you shouldn't have problems.
You can activate this feature by setting the TypeNameHandling
property of the JsonSerializerSettings
to Auto
, which activates this behaviour when the object type is different from the declared type.
https://www.newtonsoft.com/json/help/html/SerializationSettings.htm#TypeNameHandling
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
1
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your ownJsonConverter
and place your custom logic into theReadJson
method.
– Matteo Melli
Nov 14 '18 at 15:05
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
add a comment |
Json.Net has the ability to (de)serialize objects specifying the actual type of the object.
In the serialization process a property "$type"
for storing the Type is added to the resulting JSON. Then in deserialization this property is checked to obtain the actual Type.
Of course it must be a Type which inherits/implements the declared Type in you class.
Since you're using object
, you shouldn't have problems.
You can activate this feature by setting the TypeNameHandling
property of the JsonSerializerSettings
to Auto
, which activates this behaviour when the object type is different from the declared type.
https://www.newtonsoft.com/json/help/html/SerializationSettings.htm#TypeNameHandling
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
1
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your ownJsonConverter
and place your custom logic into theReadJson
method.
– Matteo Melli
Nov 14 '18 at 15:05
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
add a comment |
Json.Net has the ability to (de)serialize objects specifying the actual type of the object.
In the serialization process a property "$type"
for storing the Type is added to the resulting JSON. Then in deserialization this property is checked to obtain the actual Type.
Of course it must be a Type which inherits/implements the declared Type in you class.
Since you're using object
, you shouldn't have problems.
You can activate this feature by setting the TypeNameHandling
property of the JsonSerializerSettings
to Auto
, which activates this behaviour when the object type is different from the declared type.
https://www.newtonsoft.com/json/help/html/SerializationSettings.htm#TypeNameHandling
Json.Net has the ability to (de)serialize objects specifying the actual type of the object.
In the serialization process a property "$type"
for storing the Type is added to the resulting JSON. Then in deserialization this property is checked to obtain the actual Type.
Of course it must be a Type which inherits/implements the declared Type in you class.
Since you're using object
, you shouldn't have problems.
You can activate this feature by setting the TypeNameHandling
property of the JsonSerializerSettings
to Auto
, which activates this behaviour when the object type is different from the declared type.
https://www.newtonsoft.com/json/help/html/SerializationSettings.htm#TypeNameHandling
answered Nov 14 '18 at 10:24
Matteo MelliMatteo Melli
494
494
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
1
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your ownJsonConverter
and place your custom logic into theReadJson
method.
– Matteo Melli
Nov 14 '18 at 15:05
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
add a comment |
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
1
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your ownJsonConverter
and place your custom logic into theReadJson
method.
– Matteo Melli
Nov 14 '18 at 15:05
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
However this is not my restful service that's why it does not return $type and I do not have the option of adding $type
– PiotrN
Nov 14 '18 at 11:52
1
1
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your own
JsonConverter
and place your custom logic into the ReadJson
method.– Matteo Melli
Nov 14 '18 at 15:05
How, then, the program is supposed to decide which is the correct type? If you need to make assumptions based on things such as the name of the property (the key of the dictionary) or the content of the object, I think the only way is to write your own
JsonConverter
and place your custom logic into the ReadJson
method.– Matteo Melli
Nov 14 '18 at 15:05
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
Now I used [OnDeserialized] private void OnDeserialized(StreamingContext context)
– PiotrN
Nov 14 '18 at 15:38
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53297152%2fhow-deserialize-jsonextensiondata-dictionarystring-object-with-casting%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I5wRceTk1sNLsEirBs35zOTaVzXzYMF F9ymvkoHD,quCB6L7SycLLjcB Fcg5rCzb YgfFTs sS,QQWxlZ 3cU
Why do you have a data-structure storing instances that seem to have nothing in common in a single common map? But anyway: what is your problem exactly? What results do you get?
– HimBromBeere
Nov 14 '18 at 9:47
The reason is that the rest api returns user-defined objects.
– PiotrN
Nov 14 '18 at 9:50
Currently, the key and the string as value are created "key", "name: "Name"
– PiotrN
Nov 14 '18 at 9:55
I think you have to write your custom serializer and within that you hjave to tell Json.net of what type those properties are. I have made a quick search and it seems that here you find a simple example on how this may work. I have not testet this yet. stackoverflow.com/a/40439958/9809950
– Josef Biehler
Nov 14 '18 at 9:59
Possible duplicate of How to parse not fully corresponding json
– Adriani6
Nov 14 '18 at 10:02