getting an “org.hibernate.AnnotationException: No identifier specified for a child entity:” when using @Id on super class
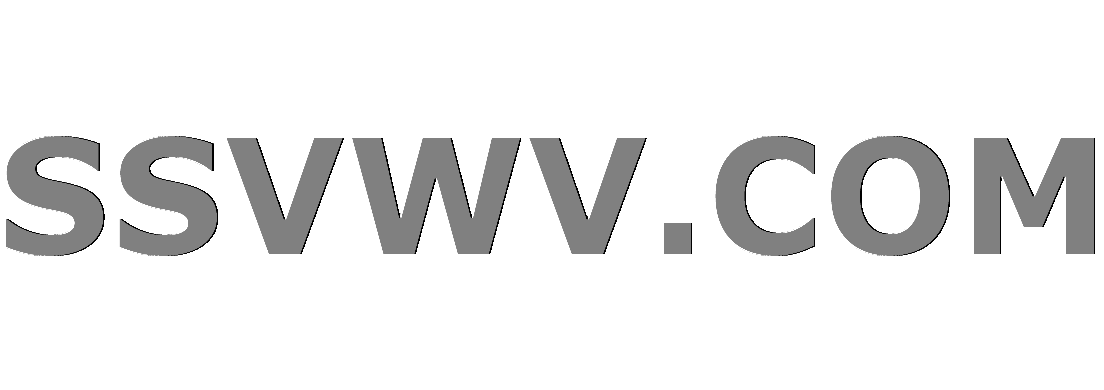
Multi tool use
I have two classes, a child class which is the entity, and a super class which contains the @Id for the entity; as shown below.
Basically it can't create the LocalContainerEntityManagerFactoryBean (code at the bottom) because it can't find the @Id on the super class. However when I remove the super class extension from the child class, it works. Any help appreciated, thanks.
Super class:
@MappedSuperclass
public class GeneralCampaign
@Id
protected String id;
protected String campaignName;
protected String accountId;
protected String createdBy;
protected STATUS status;
public GeneralCampaign()
this.id = ("cus-"+ UUID.randomUUID());
this.status = STATUS.DRAFT;
public GeneralCampaign(User userid,String accountId)
this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;
//this.isEditing = false;
public GeneralCampaign(String campaignName, String accountId, User user)
this.id = ("cus-"+ UUID.randomUUID());
this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();
// getter, setters
Child class:
@Entity(name = "local_search_campaign")
public class LocalSearchCampaign extends GeneralCampaign
@Column(name = "start_date")
private Date startDate;
@Column(name = "end_date")
private Date endDate;
@Column(name = "time")
private java.util.Date timeStamp;
@Column(name = "campaign_guard_level")
private Integer campaignGuardLevel = 4;
@Column(name = "campaign_guard_enabled")
private Boolean campaignGuardEnabled;
@ElementCollection
@CollectionTable(
name = "local_search_campaign_keyword",
joinColumns=@JoinColumn(name = "local_search_campaign_id", referencedColumnName = "id")
)
@Column(name="keyword")
private List<String> keywords = new ArrayList<>();
@OneToMany(
mappedBy="localSearchCampaign",
orphanRemoval = true,
cascade = CascadeType.ALL,
fetch=FetchType.LAZY
)
private List<Location> locations = new ArrayList<Location>();
@Enumerated(EnumType.STRING)
private STATUS status;
@OneToOne(mappedBy = "campaign", cascade = CascadeType.ALL)
private LocalSearchPayment campaignPayment;
@Column(name="landing_page_choice")
private String landingPageChoice;
@Column(name="landing_page_URI")
private String landingPageURI;
@Column(name="last_updated")
private java.util.Date lastUpdated;
@Column(name="is_editing",columnDefinition="default 0")
private boolean editing = false;
private String discountCode;
public LocalSearchCampaign()
super();
//this.id= ("LOC"+UUID.randomUUID().toString());
this.timeStamp = new java.util.Date() ;
//this.status=STATUS.DRAFT;
public LocalSearchCampaign(User userid,String accountId)
super(userid, accountId);
/*this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;*/
//this.isEditing = false;
public LocalSearchCampaign(String campaignName, String accountId, User user)
super(campaignName, accountId, user);
/*this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();*/
// getter, setters
I am creating a LocalContainerEntityManagerFactoryBean
as shown below
@Bean
@Primary
public LocalContainerEntityManagerFactoryBean userEntityManager()
LocalContainerEntityManagerFactoryBean em = new LocalContainerEntityManagerFactoryBean();
em.setDataSource(userDataSource());
em.setPackagesToScan(new String "com.packagename.etc");
HibernateJpaVendorAdapter vendorAdapter = new HibernateJpaVendorAdapter();
em.setJpaVendorAdapter(vendorAdapter);
HashMap<String, Object> properties = new HashMap<>();
properties.put("hibernate.dialect",env.getProperty("spring.datasource.hibernate.dialect"));
properties.put("hibernate.hbm2ddl.auto",env.getProperty("spring.datasource.hibernate.hbm2ddl.auto"));
//properties.put("hibernate.format_sql", true);
//properties.put("hibernate.show_sql", true);
em.setJpaPropertyMap(properties);
return em;
When I don't use the userEntityManager()
method above and just use the default, it seems to be able to find the @Id
of the super class.
spring hibernate
add a comment |
I have two classes, a child class which is the entity, and a super class which contains the @Id for the entity; as shown below.
Basically it can't create the LocalContainerEntityManagerFactoryBean (code at the bottom) because it can't find the @Id on the super class. However when I remove the super class extension from the child class, it works. Any help appreciated, thanks.
Super class:
@MappedSuperclass
public class GeneralCampaign
@Id
protected String id;
protected String campaignName;
protected String accountId;
protected String createdBy;
protected STATUS status;
public GeneralCampaign()
this.id = ("cus-"+ UUID.randomUUID());
this.status = STATUS.DRAFT;
public GeneralCampaign(User userid,String accountId)
this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;
//this.isEditing = false;
public GeneralCampaign(String campaignName, String accountId, User user)
this.id = ("cus-"+ UUID.randomUUID());
this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();
// getter, setters
Child class:
@Entity(name = "local_search_campaign")
public class LocalSearchCampaign extends GeneralCampaign
@Column(name = "start_date")
private Date startDate;
@Column(name = "end_date")
private Date endDate;
@Column(name = "time")
private java.util.Date timeStamp;
@Column(name = "campaign_guard_level")
private Integer campaignGuardLevel = 4;
@Column(name = "campaign_guard_enabled")
private Boolean campaignGuardEnabled;
@ElementCollection
@CollectionTable(
name = "local_search_campaign_keyword",
joinColumns=@JoinColumn(name = "local_search_campaign_id", referencedColumnName = "id")
)
@Column(name="keyword")
private List<String> keywords = new ArrayList<>();
@OneToMany(
mappedBy="localSearchCampaign",
orphanRemoval = true,
cascade = CascadeType.ALL,
fetch=FetchType.LAZY
)
private List<Location> locations = new ArrayList<Location>();
@Enumerated(EnumType.STRING)
private STATUS status;
@OneToOne(mappedBy = "campaign", cascade = CascadeType.ALL)
private LocalSearchPayment campaignPayment;
@Column(name="landing_page_choice")
private String landingPageChoice;
@Column(name="landing_page_URI")
private String landingPageURI;
@Column(name="last_updated")
private java.util.Date lastUpdated;
@Column(name="is_editing",columnDefinition="default 0")
private boolean editing = false;
private String discountCode;
public LocalSearchCampaign()
super();
//this.id= ("LOC"+UUID.randomUUID().toString());
this.timeStamp = new java.util.Date() ;
//this.status=STATUS.DRAFT;
public LocalSearchCampaign(User userid,String accountId)
super(userid, accountId);
/*this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;*/
//this.isEditing = false;
public LocalSearchCampaign(String campaignName, String accountId, User user)
super(campaignName, accountId, user);
/*this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();*/
// getter, setters
I am creating a LocalContainerEntityManagerFactoryBean
as shown below
@Bean
@Primary
public LocalContainerEntityManagerFactoryBean userEntityManager()
LocalContainerEntityManagerFactoryBean em = new LocalContainerEntityManagerFactoryBean();
em.setDataSource(userDataSource());
em.setPackagesToScan(new String "com.packagename.etc");
HibernateJpaVendorAdapter vendorAdapter = new HibernateJpaVendorAdapter();
em.setJpaVendorAdapter(vendorAdapter);
HashMap<String, Object> properties = new HashMap<>();
properties.put("hibernate.dialect",env.getProperty("spring.datasource.hibernate.dialect"));
properties.put("hibernate.hbm2ddl.auto",env.getProperty("spring.datasource.hibernate.hbm2ddl.auto"));
//properties.put("hibernate.format_sql", true);
//properties.put("hibernate.show_sql", true);
em.setJpaPropertyMap(properties);
return em;
When I don't use the userEntityManager()
method above and just use the default, it seems to be able to find the @Id
of the super class.
spring hibernate
Why do you need to have this custom beanuserEntityManager
? You can set those properties through spring, maybe yoursetPackagesToScan
value is the reason, how about putting both packages ofLocalSearchCampaign
andGeneralCampaign
explicitly in theString
, or just don't set this value, to have the default scanning? Because it does not say the root for the scan, but the explicit target for scan, maybe ignoring anything in subpackages?
– buræquete
Nov 13 '18 at 17:33
add a comment |
I have two classes, a child class which is the entity, and a super class which contains the @Id for the entity; as shown below.
Basically it can't create the LocalContainerEntityManagerFactoryBean (code at the bottom) because it can't find the @Id on the super class. However when I remove the super class extension from the child class, it works. Any help appreciated, thanks.
Super class:
@MappedSuperclass
public class GeneralCampaign
@Id
protected String id;
protected String campaignName;
protected String accountId;
protected String createdBy;
protected STATUS status;
public GeneralCampaign()
this.id = ("cus-"+ UUID.randomUUID());
this.status = STATUS.DRAFT;
public GeneralCampaign(User userid,String accountId)
this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;
//this.isEditing = false;
public GeneralCampaign(String campaignName, String accountId, User user)
this.id = ("cus-"+ UUID.randomUUID());
this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();
// getter, setters
Child class:
@Entity(name = "local_search_campaign")
public class LocalSearchCampaign extends GeneralCampaign
@Column(name = "start_date")
private Date startDate;
@Column(name = "end_date")
private Date endDate;
@Column(name = "time")
private java.util.Date timeStamp;
@Column(name = "campaign_guard_level")
private Integer campaignGuardLevel = 4;
@Column(name = "campaign_guard_enabled")
private Boolean campaignGuardEnabled;
@ElementCollection
@CollectionTable(
name = "local_search_campaign_keyword",
joinColumns=@JoinColumn(name = "local_search_campaign_id", referencedColumnName = "id")
)
@Column(name="keyword")
private List<String> keywords = new ArrayList<>();
@OneToMany(
mappedBy="localSearchCampaign",
orphanRemoval = true,
cascade = CascadeType.ALL,
fetch=FetchType.LAZY
)
private List<Location> locations = new ArrayList<Location>();
@Enumerated(EnumType.STRING)
private STATUS status;
@OneToOne(mappedBy = "campaign", cascade = CascadeType.ALL)
private LocalSearchPayment campaignPayment;
@Column(name="landing_page_choice")
private String landingPageChoice;
@Column(name="landing_page_URI")
private String landingPageURI;
@Column(name="last_updated")
private java.util.Date lastUpdated;
@Column(name="is_editing",columnDefinition="default 0")
private boolean editing = false;
private String discountCode;
public LocalSearchCampaign()
super();
//this.id= ("LOC"+UUID.randomUUID().toString());
this.timeStamp = new java.util.Date() ;
//this.status=STATUS.DRAFT;
public LocalSearchCampaign(User userid,String accountId)
super(userid, accountId);
/*this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;*/
//this.isEditing = false;
public LocalSearchCampaign(String campaignName, String accountId, User user)
super(campaignName, accountId, user);
/*this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();*/
// getter, setters
I am creating a LocalContainerEntityManagerFactoryBean
as shown below
@Bean
@Primary
public LocalContainerEntityManagerFactoryBean userEntityManager()
LocalContainerEntityManagerFactoryBean em = new LocalContainerEntityManagerFactoryBean();
em.setDataSource(userDataSource());
em.setPackagesToScan(new String "com.packagename.etc");
HibernateJpaVendorAdapter vendorAdapter = new HibernateJpaVendorAdapter();
em.setJpaVendorAdapter(vendorAdapter);
HashMap<String, Object> properties = new HashMap<>();
properties.put("hibernate.dialect",env.getProperty("spring.datasource.hibernate.dialect"));
properties.put("hibernate.hbm2ddl.auto",env.getProperty("spring.datasource.hibernate.hbm2ddl.auto"));
//properties.put("hibernate.format_sql", true);
//properties.put("hibernate.show_sql", true);
em.setJpaPropertyMap(properties);
return em;
When I don't use the userEntityManager()
method above and just use the default, it seems to be able to find the @Id
of the super class.
spring hibernate
I have two classes, a child class which is the entity, and a super class which contains the @Id for the entity; as shown below.
Basically it can't create the LocalContainerEntityManagerFactoryBean (code at the bottom) because it can't find the @Id on the super class. However when I remove the super class extension from the child class, it works. Any help appreciated, thanks.
Super class:
@MappedSuperclass
public class GeneralCampaign
@Id
protected String id;
protected String campaignName;
protected String accountId;
protected String createdBy;
protected STATUS status;
public GeneralCampaign()
this.id = ("cus-"+ UUID.randomUUID());
this.status = STATUS.DRAFT;
public GeneralCampaign(User userid,String accountId)
this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;
//this.isEditing = false;
public GeneralCampaign(String campaignName, String accountId, User user)
this.id = ("cus-"+ UUID.randomUUID());
this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();
// getter, setters
Child class:
@Entity(name = "local_search_campaign")
public class LocalSearchCampaign extends GeneralCampaign
@Column(name = "start_date")
private Date startDate;
@Column(name = "end_date")
private Date endDate;
@Column(name = "time")
private java.util.Date timeStamp;
@Column(name = "campaign_guard_level")
private Integer campaignGuardLevel = 4;
@Column(name = "campaign_guard_enabled")
private Boolean campaignGuardEnabled;
@ElementCollection
@CollectionTable(
name = "local_search_campaign_keyword",
joinColumns=@JoinColumn(name = "local_search_campaign_id", referencedColumnName = "id")
)
@Column(name="keyword")
private List<String> keywords = new ArrayList<>();
@OneToMany(
mappedBy="localSearchCampaign",
orphanRemoval = true,
cascade = CascadeType.ALL,
fetch=FetchType.LAZY
)
private List<Location> locations = new ArrayList<Location>();
@Enumerated(EnumType.STRING)
private STATUS status;
@OneToOne(mappedBy = "campaign", cascade = CascadeType.ALL)
private LocalSearchPayment campaignPayment;
@Column(name="landing_page_choice")
private String landingPageChoice;
@Column(name="landing_page_URI")
private String landingPageURI;
@Column(name="last_updated")
private java.util.Date lastUpdated;
@Column(name="is_editing",columnDefinition="default 0")
private boolean editing = false;
private String discountCode;
public LocalSearchCampaign()
super();
//this.id= ("LOC"+UUID.randomUUID().toString());
this.timeStamp = new java.util.Date() ;
//this.status=STATUS.DRAFT;
public LocalSearchCampaign(User userid,String accountId)
super(userid, accountId);
/*this.id = ("cus-"+ UUID.randomUUID());
this.createdBy=userid.getId();
this.status = STATUS.DRAFT;
this.accountId=accountId;*/
//this.isEditing = false;
public LocalSearchCampaign(String campaignName, String accountId, User user)
super(campaignName, accountId, user);
/*this.campaignName = campaignName;
this.accountId = accountId;
if (user != null)
this.createdBy = user.getId();*/
// getter, setters
I am creating a LocalContainerEntityManagerFactoryBean
as shown below
@Bean
@Primary
public LocalContainerEntityManagerFactoryBean userEntityManager()
LocalContainerEntityManagerFactoryBean em = new LocalContainerEntityManagerFactoryBean();
em.setDataSource(userDataSource());
em.setPackagesToScan(new String "com.packagename.etc");
HibernateJpaVendorAdapter vendorAdapter = new HibernateJpaVendorAdapter();
em.setJpaVendorAdapter(vendorAdapter);
HashMap<String, Object> properties = new HashMap<>();
properties.put("hibernate.dialect",env.getProperty("spring.datasource.hibernate.dialect"));
properties.put("hibernate.hbm2ddl.auto",env.getProperty("spring.datasource.hibernate.hbm2ddl.auto"));
//properties.put("hibernate.format_sql", true);
//properties.put("hibernate.show_sql", true);
em.setJpaPropertyMap(properties);
return em;
When I don't use the userEntityManager()
method above and just use the default, it seems to be able to find the @Id
of the super class.
spring hibernate
spring hibernate
edited Nov 13 '18 at 17:28


buræquete
5,27442049
5,27442049
asked Nov 13 '18 at 17:24
Adam JAdam J
657
657
Why do you need to have this custom beanuserEntityManager
? You can set those properties through spring, maybe yoursetPackagesToScan
value is the reason, how about putting both packages ofLocalSearchCampaign
andGeneralCampaign
explicitly in theString
, or just don't set this value, to have the default scanning? Because it does not say the root for the scan, but the explicit target for scan, maybe ignoring anything in subpackages?
– buræquete
Nov 13 '18 at 17:33
add a comment |
Why do you need to have this custom beanuserEntityManager
? You can set those properties through spring, maybe yoursetPackagesToScan
value is the reason, how about putting both packages ofLocalSearchCampaign
andGeneralCampaign
explicitly in theString
, or just don't set this value, to have the default scanning? Because it does not say the root for the scan, but the explicit target for scan, maybe ignoring anything in subpackages?
– buræquete
Nov 13 '18 at 17:33
Why do you need to have this custom bean
userEntityManager
? You can set those properties through spring, maybe your setPackagesToScan
value is the reason, how about putting both packages of LocalSearchCampaign
and GeneralCampaign
explicitly in the String
, or just don't set this value, to have the default scanning? Because it does not say the root for the scan, but the explicit target for scan, maybe ignoring anything in subpackages?– buræquete
Nov 13 '18 at 17:33
Why do you need to have this custom bean
userEntityManager
? You can set those properties through spring, maybe your setPackagesToScan
value is the reason, how about putting both packages of LocalSearchCampaign
and GeneralCampaign
explicitly in the String
, or just don't set this value, to have the default scanning? Because it does not say the root for the scan, but the explicit target for scan, maybe ignoring anything in subpackages?– buræquete
Nov 13 '18 at 17:33
add a comment |
1 Answer
1
active
oldest
votes
Check if your parent class is in package com.packagename.etc.
It seems that it could not found it ,
also you can set just :
em.setPackagesToScan(new String "*");//to scan all pacakges
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53286473%2fgetting-an-org-hibernate-annotationexception-no-identifier-specified-for-a-chi%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Check if your parent class is in package com.packagename.etc.
It seems that it could not found it ,
also you can set just :
em.setPackagesToScan(new String "*");//to scan all pacakges
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
add a comment |
Check if your parent class is in package com.packagename.etc.
It seems that it could not found it ,
also you can set just :
em.setPackagesToScan(new String "*");//to scan all pacakges
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
add a comment |
Check if your parent class is in package com.packagename.etc.
It seems that it could not found it ,
also you can set just :
em.setPackagesToScan(new String "*");//to scan all pacakges
Check if your parent class is in package com.packagename.etc.
It seems that it could not found it ,
also you can set just :
em.setPackagesToScan(new String "*");//to scan all pacakges
answered Nov 14 '18 at 0:52


Mykhailo MoskuraMykhailo Moskura
828113
828113
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
add a comment |
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Hi, the parent class is within that package but it still does not work.
– Adam J
Nov 14 '18 at 14:31
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
Child class is in the same or in subpackage or not?
– Mykhailo Moskura
Nov 14 '18 at 14:33
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
they're both in the same package, I also tried explicitly scanning the General Campaign package too but still the same issue.
– Adam J
Nov 14 '18 at 14:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53286473%2fgetting-an-org-hibernate-annotationexception-no-identifier-specified-for-a-chi%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mBZi Xkt5QN,hWAYtjYrlX 6Dt5bWVdAelvG0nJ,LKeCHg,u,ALzbCRocoDzEKw YTxvw4Qygu,iw 8
Why do you need to have this custom bean
userEntityManager
? You can set those properties through spring, maybe yoursetPackagesToScan
value is the reason, how about putting both packages ofLocalSearchCampaign
andGeneralCampaign
explicitly in theString
, or just don't set this value, to have the default scanning? Because it does not say the root for the scan, but the explicit target for scan, maybe ignoring anything in subpackages?– buræquete
Nov 13 '18 at 17:33