error while initializing int type flexible array in structure in c
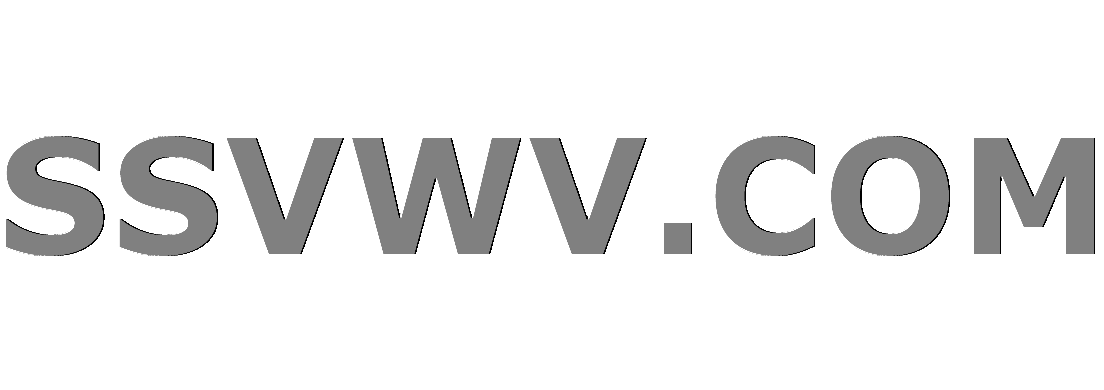
Multi tool use
i am trying to use flexible array for int variable.
Below is my code:
struct S2
int foo;
int bar;
int stud_roll;
s2g;
void test()
s2g.stud_roll = 0;
int main()
test();
return 0;
But its not working.
How can fix the problem? what is my error?
c
add a comment |
i am trying to use flexible array for int variable.
Below is my code:
struct S2
int foo;
int bar;
int stud_roll;
s2g;
void test()
s2g.stud_roll = 0;
int main()
test();
return 0;
But its not working.
How can fix the problem? what is my error?
c
I believe your struct needs to be allocated throughmalloc
and allocate extra space for the struct to hold the length of the flexible array.
– Christian Gibbons
Nov 13 '18 at 18:24
You use the compiler time initialization for dynamic sized array. When you want to initialize this array, the you have to allocate memory, and fill them. Sorry, it not that easy
– György Gulyás
Nov 13 '18 at 18:24
2
And you can't assign arrays, whether they are flexible or not.
– interjay
Nov 13 '18 at 18:28
add a comment |
i am trying to use flexible array for int variable.
Below is my code:
struct S2
int foo;
int bar;
int stud_roll;
s2g;
void test()
s2g.stud_roll = 0;
int main()
test();
return 0;
But its not working.
How can fix the problem? what is my error?
c
i am trying to use flexible array for int variable.
Below is my code:
struct S2
int foo;
int bar;
int stud_roll;
s2g;
void test()
s2g.stud_roll = 0;
int main()
test();
return 0;
But its not working.
How can fix the problem? what is my error?
c
c
edited Nov 13 '18 at 18:22
Christian Gibbons
1,869614
1,869614
asked Nov 13 '18 at 18:20
Eric IpsumEric Ipsum
1901315
1901315
I believe your struct needs to be allocated throughmalloc
and allocate extra space for the struct to hold the length of the flexible array.
– Christian Gibbons
Nov 13 '18 at 18:24
You use the compiler time initialization for dynamic sized array. When you want to initialize this array, the you have to allocate memory, and fill them. Sorry, it not that easy
– György Gulyás
Nov 13 '18 at 18:24
2
And you can't assign arrays, whether they are flexible or not.
– interjay
Nov 13 '18 at 18:28
add a comment |
I believe your struct needs to be allocated throughmalloc
and allocate extra space for the struct to hold the length of the flexible array.
– Christian Gibbons
Nov 13 '18 at 18:24
You use the compiler time initialization for dynamic sized array. When you want to initialize this array, the you have to allocate memory, and fill them. Sorry, it not that easy
– György Gulyás
Nov 13 '18 at 18:24
2
And you can't assign arrays, whether they are flexible or not.
– interjay
Nov 13 '18 at 18:28
I believe your struct needs to be allocated through
malloc
and allocate extra space for the struct to hold the length of the flexible array.– Christian Gibbons
Nov 13 '18 at 18:24
I believe your struct needs to be allocated through
malloc
and allocate extra space for the struct to hold the length of the flexible array.– Christian Gibbons
Nov 13 '18 at 18:24
You use the compiler time initialization for dynamic sized array. When you want to initialize this array, the you have to allocate memory, and fill them. Sorry, it not that easy
– György Gulyás
Nov 13 '18 at 18:24
You use the compiler time initialization for dynamic sized array. When you want to initialize this array, the you have to allocate memory, and fill them. Sorry, it not that easy
– György Gulyás
Nov 13 '18 at 18:24
2
2
And you can't assign arrays, whether they are flexible or not.
– interjay
Nov 13 '18 at 18:28
And you can't assign arrays, whether they are flexible or not.
– interjay
Nov 13 '18 at 18:28
add a comment |
2 Answers
2
active
oldest
votes
In order to use your struct with a Flexible Array Member (FAM) you need a pointer to struct, not type struct. The FAM provides the convenience of allowing allocation for the struct and the FAM in a single allocation, rather than needing to allocate for the struct and then for stud_roll
separately. For example:
struct S2
int foo;
int bar;
int stud_roll;
*s2g; /* note the declaration as a pointer */
void test (void)
{
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
...
There you allocate storage for both the struct sizeof *s2g
plus the element of stud_roll
, e.g. ELEMENTS * sizeof *s2g->stud_roll
. This provides the single allocation/single free.
A short example would be:
#include <stdio.h>
#include <stdlib.h>
#define ELEMENTS 10
struct S2
int foo;
int bar;
int stud_roll;
*s2g;
void test (void)
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
s2g->foo = 1;
s2g->bar = 2;
for (int i = 0; i < ELEMENTS; i++)
s2g->stud_roll[i] = i + 1;
int main (void)
test();
printf ("s2g->foo: %dns2g->bar: %dn", s2g->foo, s2g->bar);
for (int i = 0; i < ELEMENTS; i++)
printf (" %d", s2g->stud_roll[i]);
putchar ('n');
free (s2g);
return 0;
(note: since the flexible array member is static
, you cannot have an array of struct containing a flexible array member -- but you can have an array of pointers with separate allocation for each)
Example Use/Output
$ ./bin/famtst
s2g->foo: 1
s2g->bar: 2
1 2 3 4 5 6 7 8 9 10
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
add a comment |
You need to allocate memory for (struct S2).stud_roll
. Without any memory you will write out of bounds. Assimung there is no padding sizeof(struct S2) == sizeof(int) + sizeof(int)
- there is no memory allocated for stud_roll cause the member takes no memory "by itself".
s2g.stud_roll = 0;
You can't assign arrays in that way in C.
You can use compound literal to allocate some memory on stack:
#define STUD_ROLL_SIZE 4
struct S2 * const s2g = (void*)((char[sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int)]) 0 );
void test(void)
s2g->stud_roll[0] = 1;
// or
memcpy(s2g->stud_roll, (int) 1, 2, 3, 4 , 4 * sizeof(int));
or use malloc to dynamically allocate the memory, you need to allocate more memory than the sizeof(struct S2)
:
struct S2 *s2g = NULL;
void test(void)
s2g = malloc(sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int));
if (s2g == NULL)
fprintf(stderr, "Abort ship! Abort ship!n");
exit(-1);
s2g->stud_roll[0] = 1;
free(s2g);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287260%2ferror-while-initializing-int-type-flexible-array-in-structure-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
In order to use your struct with a Flexible Array Member (FAM) you need a pointer to struct, not type struct. The FAM provides the convenience of allowing allocation for the struct and the FAM in a single allocation, rather than needing to allocate for the struct and then for stud_roll
separately. For example:
struct S2
int foo;
int bar;
int stud_roll;
*s2g; /* note the declaration as a pointer */
void test (void)
{
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
...
There you allocate storage for both the struct sizeof *s2g
plus the element of stud_roll
, e.g. ELEMENTS * sizeof *s2g->stud_roll
. This provides the single allocation/single free.
A short example would be:
#include <stdio.h>
#include <stdlib.h>
#define ELEMENTS 10
struct S2
int foo;
int bar;
int stud_roll;
*s2g;
void test (void)
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
s2g->foo = 1;
s2g->bar = 2;
for (int i = 0; i < ELEMENTS; i++)
s2g->stud_roll[i] = i + 1;
int main (void)
test();
printf ("s2g->foo: %dns2g->bar: %dn", s2g->foo, s2g->bar);
for (int i = 0; i < ELEMENTS; i++)
printf (" %d", s2g->stud_roll[i]);
putchar ('n');
free (s2g);
return 0;
(note: since the flexible array member is static
, you cannot have an array of struct containing a flexible array member -- but you can have an array of pointers with separate allocation for each)
Example Use/Output
$ ./bin/famtst
s2g->foo: 1
s2g->bar: 2
1 2 3 4 5 6 7 8 9 10
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
add a comment |
In order to use your struct with a Flexible Array Member (FAM) you need a pointer to struct, not type struct. The FAM provides the convenience of allowing allocation for the struct and the FAM in a single allocation, rather than needing to allocate for the struct and then for stud_roll
separately. For example:
struct S2
int foo;
int bar;
int stud_roll;
*s2g; /* note the declaration as a pointer */
void test (void)
{
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
...
There you allocate storage for both the struct sizeof *s2g
plus the element of stud_roll
, e.g. ELEMENTS * sizeof *s2g->stud_roll
. This provides the single allocation/single free.
A short example would be:
#include <stdio.h>
#include <stdlib.h>
#define ELEMENTS 10
struct S2
int foo;
int bar;
int stud_roll;
*s2g;
void test (void)
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
s2g->foo = 1;
s2g->bar = 2;
for (int i = 0; i < ELEMENTS; i++)
s2g->stud_roll[i] = i + 1;
int main (void)
test();
printf ("s2g->foo: %dns2g->bar: %dn", s2g->foo, s2g->bar);
for (int i = 0; i < ELEMENTS; i++)
printf (" %d", s2g->stud_roll[i]);
putchar ('n');
free (s2g);
return 0;
(note: since the flexible array member is static
, you cannot have an array of struct containing a flexible array member -- but you can have an array of pointers with separate allocation for each)
Example Use/Output
$ ./bin/famtst
s2g->foo: 1
s2g->bar: 2
1 2 3 4 5 6 7 8 9 10
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
add a comment |
In order to use your struct with a Flexible Array Member (FAM) you need a pointer to struct, not type struct. The FAM provides the convenience of allowing allocation for the struct and the FAM in a single allocation, rather than needing to allocate for the struct and then for stud_roll
separately. For example:
struct S2
int foo;
int bar;
int stud_roll;
*s2g; /* note the declaration as a pointer */
void test (void)
{
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
...
There you allocate storage for both the struct sizeof *s2g
plus the element of stud_roll
, e.g. ELEMENTS * sizeof *s2g->stud_roll
. This provides the single allocation/single free.
A short example would be:
#include <stdio.h>
#include <stdlib.h>
#define ELEMENTS 10
struct S2
int foo;
int bar;
int stud_roll;
*s2g;
void test (void)
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
s2g->foo = 1;
s2g->bar = 2;
for (int i = 0; i < ELEMENTS; i++)
s2g->stud_roll[i] = i + 1;
int main (void)
test();
printf ("s2g->foo: %dns2g->bar: %dn", s2g->foo, s2g->bar);
for (int i = 0; i < ELEMENTS; i++)
printf (" %d", s2g->stud_roll[i]);
putchar ('n');
free (s2g);
return 0;
(note: since the flexible array member is static
, you cannot have an array of struct containing a flexible array member -- but you can have an array of pointers with separate allocation for each)
Example Use/Output
$ ./bin/famtst
s2g->foo: 1
s2g->bar: 2
1 2 3 4 5 6 7 8 9 10
In order to use your struct with a Flexible Array Member (FAM) you need a pointer to struct, not type struct. The FAM provides the convenience of allowing allocation for the struct and the FAM in a single allocation, rather than needing to allocate for the struct and then for stud_roll
separately. For example:
struct S2
int foo;
int bar;
int stud_roll;
*s2g; /* note the declaration as a pointer */
void test (void)
{
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
...
There you allocate storage for both the struct sizeof *s2g
plus the element of stud_roll
, e.g. ELEMENTS * sizeof *s2g->stud_roll
. This provides the single allocation/single free.
A short example would be:
#include <stdio.h>
#include <stdlib.h>
#define ELEMENTS 10
struct S2
int foo;
int bar;
int stud_roll;
*s2g;
void test (void)
s2g = malloc (sizeof *s2g + ELEMENTS * sizeof *s2g->stud_roll);
if (!s2g)
perror ("malloc-s2g");
exit (EXIT_FAILURE);
s2g->foo = 1;
s2g->bar = 2;
for (int i = 0; i < ELEMENTS; i++)
s2g->stud_roll[i] = i + 1;
int main (void)
test();
printf ("s2g->foo: %dns2g->bar: %dn", s2g->foo, s2g->bar);
for (int i = 0; i < ELEMENTS; i++)
printf (" %d", s2g->stud_roll[i]);
putchar ('n');
free (s2g);
return 0;
(note: since the flexible array member is static
, you cannot have an array of struct containing a flexible array member -- but you can have an array of pointers with separate allocation for each)
Example Use/Output
$ ./bin/famtst
s2g->foo: 1
s2g->bar: 2
1 2 3 4 5 6 7 8 9 10
edited Nov 13 '18 at 18:50
answered Nov 13 '18 at 18:41


David C. RankinDavid C. Rankin
41.2k32748
41.2k32748
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
add a comment |
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
Thanks for this answer.
– Eric Ipsum
Nov 13 '18 at 19:18
add a comment |
You need to allocate memory for (struct S2).stud_roll
. Without any memory you will write out of bounds. Assimung there is no padding sizeof(struct S2) == sizeof(int) + sizeof(int)
- there is no memory allocated for stud_roll cause the member takes no memory "by itself".
s2g.stud_roll = 0;
You can't assign arrays in that way in C.
You can use compound literal to allocate some memory on stack:
#define STUD_ROLL_SIZE 4
struct S2 * const s2g = (void*)((char[sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int)]) 0 );
void test(void)
s2g->stud_roll[0] = 1;
// or
memcpy(s2g->stud_roll, (int) 1, 2, 3, 4 , 4 * sizeof(int));
or use malloc to dynamically allocate the memory, you need to allocate more memory than the sizeof(struct S2)
:
struct S2 *s2g = NULL;
void test(void)
s2g = malloc(sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int));
if (s2g == NULL)
fprintf(stderr, "Abort ship! Abort ship!n");
exit(-1);
s2g->stud_roll[0] = 1;
free(s2g);
add a comment |
You need to allocate memory for (struct S2).stud_roll
. Without any memory you will write out of bounds. Assimung there is no padding sizeof(struct S2) == sizeof(int) + sizeof(int)
- there is no memory allocated for stud_roll cause the member takes no memory "by itself".
s2g.stud_roll = 0;
You can't assign arrays in that way in C.
You can use compound literal to allocate some memory on stack:
#define STUD_ROLL_SIZE 4
struct S2 * const s2g = (void*)((char[sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int)]) 0 );
void test(void)
s2g->stud_roll[0] = 1;
// or
memcpy(s2g->stud_roll, (int) 1, 2, 3, 4 , 4 * sizeof(int));
or use malloc to dynamically allocate the memory, you need to allocate more memory than the sizeof(struct S2)
:
struct S2 *s2g = NULL;
void test(void)
s2g = malloc(sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int));
if (s2g == NULL)
fprintf(stderr, "Abort ship! Abort ship!n");
exit(-1);
s2g->stud_roll[0] = 1;
free(s2g);
add a comment |
You need to allocate memory for (struct S2).stud_roll
. Without any memory you will write out of bounds. Assimung there is no padding sizeof(struct S2) == sizeof(int) + sizeof(int)
- there is no memory allocated for stud_roll cause the member takes no memory "by itself".
s2g.stud_roll = 0;
You can't assign arrays in that way in C.
You can use compound literal to allocate some memory on stack:
#define STUD_ROLL_SIZE 4
struct S2 * const s2g = (void*)((char[sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int)]) 0 );
void test(void)
s2g->stud_roll[0] = 1;
// or
memcpy(s2g->stud_roll, (int) 1, 2, 3, 4 , 4 * sizeof(int));
or use malloc to dynamically allocate the memory, you need to allocate more memory than the sizeof(struct S2)
:
struct S2 *s2g = NULL;
void test(void)
s2g = malloc(sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int));
if (s2g == NULL)
fprintf(stderr, "Abort ship! Abort ship!n");
exit(-1);
s2g->stud_roll[0] = 1;
free(s2g);
You need to allocate memory for (struct S2).stud_roll
. Without any memory you will write out of bounds. Assimung there is no padding sizeof(struct S2) == sizeof(int) + sizeof(int)
- there is no memory allocated for stud_roll cause the member takes no memory "by itself".
s2g.stud_roll = 0;
You can't assign arrays in that way in C.
You can use compound literal to allocate some memory on stack:
#define STUD_ROLL_SIZE 4
struct S2 * const s2g = (void*)((char[sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int)]) 0 );
void test(void)
s2g->stud_roll[0] = 1;
// or
memcpy(s2g->stud_roll, (int) 1, 2, 3, 4 , 4 * sizeof(int));
or use malloc to dynamically allocate the memory, you need to allocate more memory than the sizeof(struct S2)
:
struct S2 *s2g = NULL;
void test(void)
s2g = malloc(sizeof(struct S2) + STUD_ROLL_SIZE * sizeof(int));
if (s2g == NULL)
fprintf(stderr, "Abort ship! Abort ship!n");
exit(-1);
s2g->stud_roll[0] = 1;
free(s2g);
answered Nov 13 '18 at 18:40
Kamil CukKamil Cuk
10.1k1527
10.1k1527
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287260%2ferror-while-initializing-int-type-flexible-array-in-structure-in-c%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qT28PkDPSFdI99ByyopFi,F 1S13fN2Ay9RF,lANG
I believe your struct needs to be allocated through
malloc
and allocate extra space for the struct to hold the length of the flexible array.– Christian Gibbons
Nov 13 '18 at 18:24
You use the compiler time initialization for dynamic sized array. When you want to initialize this array, the you have to allocate memory, and fill them. Sorry, it not that easy
– György Gulyás
Nov 13 '18 at 18:24
2
And you can't assign arrays, whether they are flexible or not.
– interjay
Nov 13 '18 at 18:28