I am creating a tkinter gui, and i need to make it a thread. But the thread doesn't work
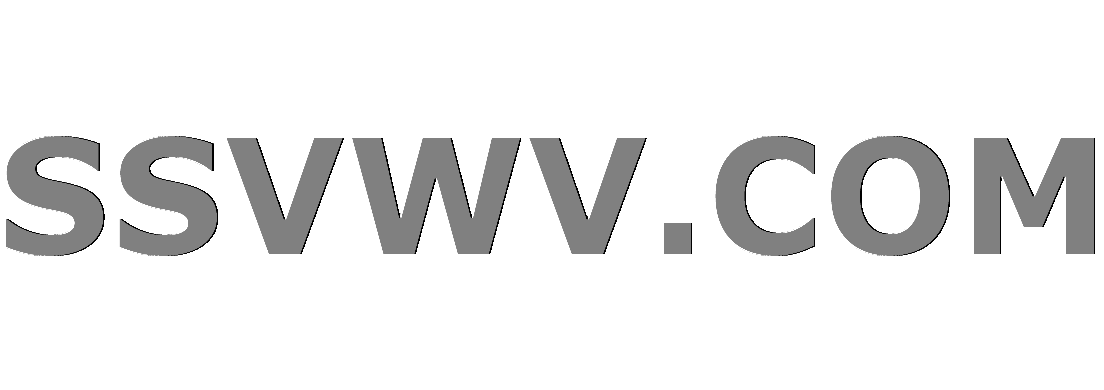
Multi tool use
up vote
-1
down vote
favorite
I am creating a chinese checkers AI. I want to make the board, and check the mouse position meanwhile. I decided to use multithreading:
from tkinter import *
from pyautogui import *
from threading import *
def create_board():
#a lot of tkinter stuff
def check_mouse:
if position()[0] > 390 & position()[0] < 455:
print("mouse detected")
board = Thread(target=create_board)
board.start()
But when I run it in terminal, I get:
2018-11-11 16:50:55.129 python[27074:8053445] WARNING: NSWindow drag regions should only be invalidated on the Main Thread! This will throw an exception in the future. Called from (
0 AppKit 0x00007fff44af22e3 -[NSWindow(NSWindow_Theme) _postWindowNeedsToResetDragMarginsUnlessPostingDisabled] + 386
1 AppKit 0x00007fff44aef68c -[NSWindow _initContent:styleMask:backing:defer:contentView:] + 1488
2 AppKit 0x00007fff44aef0b6 -[NSWindow initWithContentRect:styleMask:backing:defer:] + 45
3 libtk8.6.dylib 0x0000000101319426 TkMacOSXMakeRealWindowExist + 742
4 libtk8.6.dylib 0x0000000101318ffc TkWmMapWindow + 60
5 libtk8.6.dylib 0x0000000101261930 Tk_MapWindow + 192
6 libtk8.6.dylib 0x000000010126b541 MapFrame + 65
7 libtcl8.6.dylib 0x000000010119cc71 TclServiceIdle + 161
8 libtcl8.6.dylib 0x000000010117a39d Tcl_DoOneEvent + 397
9 _tkinter.cpython-37m-darwin.so 0x000000010108a88e _tkinter_tkapp_mainloop + 286
10 python 0x000000010098eba6 _PyMethodDef_RawFastCallKeywords + 230
11 python 0x000000010099bae4 _PyMethodDescr_FastCallKeywords + 84
12 python 0x0000000100acf32e call_function + 382
13 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
14 python 0x0000000100ac0a42 _PyEval_EvalCodeWithName + 418
15 python 0x000000010098ea73 _PyFunction_FastCallKeywords + 195
16 python 0x0000000100acf265 call_function + 181
17 python 0x0000000100accdaf _PyEval_EvalFrameDefault + 45215
18 python 0x000000010098e368 function_code_fastcall + 120
19 python 0x0000000100acd1c6 _PyEval_EvalFrameDefault + 46262
20 python 0x000000010098e368 function_code_fastcall + 120
21 python 0x0000000100acf265 call_function + 181
22 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
23 python 0x000000010098e368 function_code_fastcall + 120
24 python 0x0000000100acf265 call_function + 181
25 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
26 python 0x000000010098e368 function_code_fastcall + 120
27 python 0x0000000100991782 method_call + 130
28 python 0x000000010098f1e2 PyObject_Call + 130
29 python 0x0000000100baf5cb t_bootstrap + 123
30 libsystem_pthread.dylib 0x00007fff747a133d _pthread_body + 126
31 libsystem_pthread.dylib 0x00007fff747a42a7 _pthread_start + 70
32 libsystem_pthread.dylib 0x00007fff747a0425 thread_start + 13
)
What happened? Is there something wrong with the tkinter window?
python multithreading tkinter
add a comment |
up vote
-1
down vote
favorite
I am creating a chinese checkers AI. I want to make the board, and check the mouse position meanwhile. I decided to use multithreading:
from tkinter import *
from pyautogui import *
from threading import *
def create_board():
#a lot of tkinter stuff
def check_mouse:
if position()[0] > 390 & position()[0] < 455:
print("mouse detected")
board = Thread(target=create_board)
board.start()
But when I run it in terminal, I get:
2018-11-11 16:50:55.129 python[27074:8053445] WARNING: NSWindow drag regions should only be invalidated on the Main Thread! This will throw an exception in the future. Called from (
0 AppKit 0x00007fff44af22e3 -[NSWindow(NSWindow_Theme) _postWindowNeedsToResetDragMarginsUnlessPostingDisabled] + 386
1 AppKit 0x00007fff44aef68c -[NSWindow _initContent:styleMask:backing:defer:contentView:] + 1488
2 AppKit 0x00007fff44aef0b6 -[NSWindow initWithContentRect:styleMask:backing:defer:] + 45
3 libtk8.6.dylib 0x0000000101319426 TkMacOSXMakeRealWindowExist + 742
4 libtk8.6.dylib 0x0000000101318ffc TkWmMapWindow + 60
5 libtk8.6.dylib 0x0000000101261930 Tk_MapWindow + 192
6 libtk8.6.dylib 0x000000010126b541 MapFrame + 65
7 libtcl8.6.dylib 0x000000010119cc71 TclServiceIdle + 161
8 libtcl8.6.dylib 0x000000010117a39d Tcl_DoOneEvent + 397
9 _tkinter.cpython-37m-darwin.so 0x000000010108a88e _tkinter_tkapp_mainloop + 286
10 python 0x000000010098eba6 _PyMethodDef_RawFastCallKeywords + 230
11 python 0x000000010099bae4 _PyMethodDescr_FastCallKeywords + 84
12 python 0x0000000100acf32e call_function + 382
13 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
14 python 0x0000000100ac0a42 _PyEval_EvalCodeWithName + 418
15 python 0x000000010098ea73 _PyFunction_FastCallKeywords + 195
16 python 0x0000000100acf265 call_function + 181
17 python 0x0000000100accdaf _PyEval_EvalFrameDefault + 45215
18 python 0x000000010098e368 function_code_fastcall + 120
19 python 0x0000000100acd1c6 _PyEval_EvalFrameDefault + 46262
20 python 0x000000010098e368 function_code_fastcall + 120
21 python 0x0000000100acf265 call_function + 181
22 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
23 python 0x000000010098e368 function_code_fastcall + 120
24 python 0x0000000100acf265 call_function + 181
25 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
26 python 0x000000010098e368 function_code_fastcall + 120
27 python 0x0000000100991782 method_call + 130
28 python 0x000000010098f1e2 PyObject_Call + 130
29 python 0x0000000100baf5cb t_bootstrap + 123
30 libsystem_pthread.dylib 0x00007fff747a133d _pthread_body + 126
31 libsystem_pthread.dylib 0x00007fff747a42a7 _pthread_start + 70
32 libsystem_pthread.dylib 0x00007fff747a0425 thread_start + 13
)
What happened? Is there something wrong with the tkinter window?
python multithreading tkinter
1
Don't use threading with Tkinter if you don't have to. If you really have to, use Tkinter's TopLevel widget for your UI element, and implement your own event loop. It's a nuisance, to be avoided if possible.
– rd_nielsen
Nov 12 at 1:03
@rd_nielsen: the use of threads is completely unrelated to the use ofToplevel
. The important thing with threads is that you can only access tkinter objects from a single thread.
– Bryan Oakley
Nov 12 at 4:26
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I am creating a chinese checkers AI. I want to make the board, and check the mouse position meanwhile. I decided to use multithreading:
from tkinter import *
from pyautogui import *
from threading import *
def create_board():
#a lot of tkinter stuff
def check_mouse:
if position()[0] > 390 & position()[0] < 455:
print("mouse detected")
board = Thread(target=create_board)
board.start()
But when I run it in terminal, I get:
2018-11-11 16:50:55.129 python[27074:8053445] WARNING: NSWindow drag regions should only be invalidated on the Main Thread! This will throw an exception in the future. Called from (
0 AppKit 0x00007fff44af22e3 -[NSWindow(NSWindow_Theme) _postWindowNeedsToResetDragMarginsUnlessPostingDisabled] + 386
1 AppKit 0x00007fff44aef68c -[NSWindow _initContent:styleMask:backing:defer:contentView:] + 1488
2 AppKit 0x00007fff44aef0b6 -[NSWindow initWithContentRect:styleMask:backing:defer:] + 45
3 libtk8.6.dylib 0x0000000101319426 TkMacOSXMakeRealWindowExist + 742
4 libtk8.6.dylib 0x0000000101318ffc TkWmMapWindow + 60
5 libtk8.6.dylib 0x0000000101261930 Tk_MapWindow + 192
6 libtk8.6.dylib 0x000000010126b541 MapFrame + 65
7 libtcl8.6.dylib 0x000000010119cc71 TclServiceIdle + 161
8 libtcl8.6.dylib 0x000000010117a39d Tcl_DoOneEvent + 397
9 _tkinter.cpython-37m-darwin.so 0x000000010108a88e _tkinter_tkapp_mainloop + 286
10 python 0x000000010098eba6 _PyMethodDef_RawFastCallKeywords + 230
11 python 0x000000010099bae4 _PyMethodDescr_FastCallKeywords + 84
12 python 0x0000000100acf32e call_function + 382
13 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
14 python 0x0000000100ac0a42 _PyEval_EvalCodeWithName + 418
15 python 0x000000010098ea73 _PyFunction_FastCallKeywords + 195
16 python 0x0000000100acf265 call_function + 181
17 python 0x0000000100accdaf _PyEval_EvalFrameDefault + 45215
18 python 0x000000010098e368 function_code_fastcall + 120
19 python 0x0000000100acd1c6 _PyEval_EvalFrameDefault + 46262
20 python 0x000000010098e368 function_code_fastcall + 120
21 python 0x0000000100acf265 call_function + 181
22 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
23 python 0x000000010098e368 function_code_fastcall + 120
24 python 0x0000000100acf265 call_function + 181
25 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
26 python 0x000000010098e368 function_code_fastcall + 120
27 python 0x0000000100991782 method_call + 130
28 python 0x000000010098f1e2 PyObject_Call + 130
29 python 0x0000000100baf5cb t_bootstrap + 123
30 libsystem_pthread.dylib 0x00007fff747a133d _pthread_body + 126
31 libsystem_pthread.dylib 0x00007fff747a42a7 _pthread_start + 70
32 libsystem_pthread.dylib 0x00007fff747a0425 thread_start + 13
)
What happened? Is there something wrong with the tkinter window?
python multithreading tkinter
I am creating a chinese checkers AI. I want to make the board, and check the mouse position meanwhile. I decided to use multithreading:
from tkinter import *
from pyautogui import *
from threading import *
def create_board():
#a lot of tkinter stuff
def check_mouse:
if position()[0] > 390 & position()[0] < 455:
print("mouse detected")
board = Thread(target=create_board)
board.start()
But when I run it in terminal, I get:
2018-11-11 16:50:55.129 python[27074:8053445] WARNING: NSWindow drag regions should only be invalidated on the Main Thread! This will throw an exception in the future. Called from (
0 AppKit 0x00007fff44af22e3 -[NSWindow(NSWindow_Theme) _postWindowNeedsToResetDragMarginsUnlessPostingDisabled] + 386
1 AppKit 0x00007fff44aef68c -[NSWindow _initContent:styleMask:backing:defer:contentView:] + 1488
2 AppKit 0x00007fff44aef0b6 -[NSWindow initWithContentRect:styleMask:backing:defer:] + 45
3 libtk8.6.dylib 0x0000000101319426 TkMacOSXMakeRealWindowExist + 742
4 libtk8.6.dylib 0x0000000101318ffc TkWmMapWindow + 60
5 libtk8.6.dylib 0x0000000101261930 Tk_MapWindow + 192
6 libtk8.6.dylib 0x000000010126b541 MapFrame + 65
7 libtcl8.6.dylib 0x000000010119cc71 TclServiceIdle + 161
8 libtcl8.6.dylib 0x000000010117a39d Tcl_DoOneEvent + 397
9 _tkinter.cpython-37m-darwin.so 0x000000010108a88e _tkinter_tkapp_mainloop + 286
10 python 0x000000010098eba6 _PyMethodDef_RawFastCallKeywords + 230
11 python 0x000000010099bae4 _PyMethodDescr_FastCallKeywords + 84
12 python 0x0000000100acf32e call_function + 382
13 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
14 python 0x0000000100ac0a42 _PyEval_EvalCodeWithName + 418
15 python 0x000000010098ea73 _PyFunction_FastCallKeywords + 195
16 python 0x0000000100acf265 call_function + 181
17 python 0x0000000100accdaf _PyEval_EvalFrameDefault + 45215
18 python 0x000000010098e368 function_code_fastcall + 120
19 python 0x0000000100acd1c6 _PyEval_EvalFrameDefault + 46262
20 python 0x000000010098e368 function_code_fastcall + 120
21 python 0x0000000100acf265 call_function + 181
22 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
23 python 0x000000010098e368 function_code_fastcall + 120
24 python 0x0000000100acf265 call_function + 181
25 python 0x0000000100accd19 _PyEval_EvalFrameDefault + 45065
26 python 0x000000010098e368 function_code_fastcall + 120
27 python 0x0000000100991782 method_call + 130
28 python 0x000000010098f1e2 PyObject_Call + 130
29 python 0x0000000100baf5cb t_bootstrap + 123
30 libsystem_pthread.dylib 0x00007fff747a133d _pthread_body + 126
31 libsystem_pthread.dylib 0x00007fff747a42a7 _pthread_start + 70
32 libsystem_pthread.dylib 0x00007fff747a0425 thread_start + 13
)
What happened? Is there something wrong with the tkinter window?
python multithreading tkinter
python multithreading tkinter
edited Nov 12 at 2:37


martineau
65.4k988177
65.4k988177
asked Nov 12 at 0:53
F. Zeng
209
209
1
Don't use threading with Tkinter if you don't have to. If you really have to, use Tkinter's TopLevel widget for your UI element, and implement your own event loop. It's a nuisance, to be avoided if possible.
– rd_nielsen
Nov 12 at 1:03
@rd_nielsen: the use of threads is completely unrelated to the use ofToplevel
. The important thing with threads is that you can only access tkinter objects from a single thread.
– Bryan Oakley
Nov 12 at 4:26
add a comment |
1
Don't use threading with Tkinter if you don't have to. If you really have to, use Tkinter's TopLevel widget for your UI element, and implement your own event loop. It's a nuisance, to be avoided if possible.
– rd_nielsen
Nov 12 at 1:03
@rd_nielsen: the use of threads is completely unrelated to the use ofToplevel
. The important thing with threads is that you can only access tkinter objects from a single thread.
– Bryan Oakley
Nov 12 at 4:26
1
1
Don't use threading with Tkinter if you don't have to. If you really have to, use Tkinter's TopLevel widget for your UI element, and implement your own event loop. It's a nuisance, to be avoided if possible.
– rd_nielsen
Nov 12 at 1:03
Don't use threading with Tkinter if you don't have to. If you really have to, use Tkinter's TopLevel widget for your UI element, and implement your own event loop. It's a nuisance, to be avoided if possible.
– rd_nielsen
Nov 12 at 1:03
@rd_nielsen: the use of threads is completely unrelated to the use of
Toplevel
. The important thing with threads is that you can only access tkinter objects from a single thread.– Bryan Oakley
Nov 12 at 4:26
@rd_nielsen: the use of threads is completely unrelated to the use of
Toplevel
. The important thing with threads is that you can only access tkinter objects from a single thread.– Bryan Oakley
Nov 12 at 4:26
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
Tkinter (like almost all GUI frameworks I'm aware of), require that modifications to the GUI state happen on a particular thread. See this question for more discussion on why. This doesn't mean that there can't be any other threads in your application- just that you can't modify the state of the GUI from those other threads.
If you must use other threads to keep long-running tasks from hogging the thread being used for the GUI, you can have worker threads that interact with the main thread via a Queue
, as described here.
I can't caveat enough that multithreading will multiply the complexity of your application by 10x (especially if you are new to concepts like Queue
s). However, if you are unfamiliar with Queue
s and their typical use in concurrent programming and still want to get started, a good place to begin is with the producer/consumer pattern. For an even more foundational look at what the "queue" concept means in comp-sci, you can look at this page which describes a queue and has you implement your own simple version of one.
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
1
In general, queues are a data structure (like alist
ordict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue infrom queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try toget
from one thread while other threads are working to create things to put on the queue.
– augray
Nov 12 at 1:37
also added some useful references to the answer
– augray
Nov 12 at 1:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254763%2fi-am-creating-a-tkinter-gui-and-i-need-to-make-it-a-thread-but-the-thread-does%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
Tkinter (like almost all GUI frameworks I'm aware of), require that modifications to the GUI state happen on a particular thread. See this question for more discussion on why. This doesn't mean that there can't be any other threads in your application- just that you can't modify the state of the GUI from those other threads.
If you must use other threads to keep long-running tasks from hogging the thread being used for the GUI, you can have worker threads that interact with the main thread via a Queue
, as described here.
I can't caveat enough that multithreading will multiply the complexity of your application by 10x (especially if you are new to concepts like Queue
s). However, if you are unfamiliar with Queue
s and their typical use in concurrent programming and still want to get started, a good place to begin is with the producer/consumer pattern. For an even more foundational look at what the "queue" concept means in comp-sci, you can look at this page which describes a queue and has you implement your own simple version of one.
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
1
In general, queues are a data structure (like alist
ordict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue infrom queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try toget
from one thread while other threads are working to create things to put on the queue.
– augray
Nov 12 at 1:37
also added some useful references to the answer
– augray
Nov 12 at 1:45
add a comment |
up vote
2
down vote
Tkinter (like almost all GUI frameworks I'm aware of), require that modifications to the GUI state happen on a particular thread. See this question for more discussion on why. This doesn't mean that there can't be any other threads in your application- just that you can't modify the state of the GUI from those other threads.
If you must use other threads to keep long-running tasks from hogging the thread being used for the GUI, you can have worker threads that interact with the main thread via a Queue
, as described here.
I can't caveat enough that multithreading will multiply the complexity of your application by 10x (especially if you are new to concepts like Queue
s). However, if you are unfamiliar with Queue
s and their typical use in concurrent programming and still want to get started, a good place to begin is with the producer/consumer pattern. For an even more foundational look at what the "queue" concept means in comp-sci, you can look at this page which describes a queue and has you implement your own simple version of one.
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
1
In general, queues are a data structure (like alist
ordict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue infrom queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try toget
from one thread while other threads are working to create things to put on the queue.
– augray
Nov 12 at 1:37
also added some useful references to the answer
– augray
Nov 12 at 1:45
add a comment |
up vote
2
down vote
up vote
2
down vote
Tkinter (like almost all GUI frameworks I'm aware of), require that modifications to the GUI state happen on a particular thread. See this question for more discussion on why. This doesn't mean that there can't be any other threads in your application- just that you can't modify the state of the GUI from those other threads.
If you must use other threads to keep long-running tasks from hogging the thread being used for the GUI, you can have worker threads that interact with the main thread via a Queue
, as described here.
I can't caveat enough that multithreading will multiply the complexity of your application by 10x (especially if you are new to concepts like Queue
s). However, if you are unfamiliar with Queue
s and their typical use in concurrent programming and still want to get started, a good place to begin is with the producer/consumer pattern. For an even more foundational look at what the "queue" concept means in comp-sci, you can look at this page which describes a queue and has you implement your own simple version of one.
Tkinter (like almost all GUI frameworks I'm aware of), require that modifications to the GUI state happen on a particular thread. See this question for more discussion on why. This doesn't mean that there can't be any other threads in your application- just that you can't modify the state of the GUI from those other threads.
If you must use other threads to keep long-running tasks from hogging the thread being used for the GUI, you can have worker threads that interact with the main thread via a Queue
, as described here.
I can't caveat enough that multithreading will multiply the complexity of your application by 10x (especially if you are new to concepts like Queue
s). However, if you are unfamiliar with Queue
s and their typical use in concurrent programming and still want to get started, a good place to begin is with the producer/consumer pattern. For an even more foundational look at what the "queue" concept means in comp-sci, you can look at this page which describes a queue and has you implement your own simple version of one.
edited Nov 13 at 5:53
answered Nov 12 at 1:07


augray
2,1051123
2,1051123
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
1
In general, queues are a data structure (like alist
ordict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue infrom queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try toget
from one thread while other threads are working to create things to put on the queue.
– augray
Nov 12 at 1:37
also added some useful references to the answer
– augray
Nov 12 at 1:45
add a comment |
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
1
In general, queues are a data structure (like alist
ordict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue infrom queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try toget
from one thread while other threads are working to create things to put on the queue.
– augray
Nov 12 at 1:37
also added some useful references to the answer
– augray
Nov 12 at 1:45
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
I'm sorry, but i'm not good at python yet. I am, after all, in 5th grade. Can you explain to me what Queue is about?
– F. Zeng
Nov 12 at 1:30
1
1
In general, queues are a data structure (like a
list
or dict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue in from queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try to get
from one thread while other threads are working to create things to put on the queue.– augray
Nov 12 at 1:37
In general, queues are a data structure (like a
list
or dict
that hold objects), but they're made to work so that the order you place things in the queue is the order they get removed. Think of a checkout line at the store. The queue in from queue import Queue
in python is designed with other tools for concurrent programming. In particular, it can "block" when you try to get
from one thread while other threads are working to create things to put on the queue.– augray
Nov 12 at 1:37
also added some useful references to the answer
– augray
Nov 12 at 1:45
also added some useful references to the answer
– augray
Nov 12 at 1:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254763%2fi-am-creating-a-tkinter-gui-and-i-need-to-make-it-a-thread-but-the-thread-does%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ebTPRmUt2 m0CbvB4S5NybujkS8 SqO8,ESf U4FT5,HcJfJ,iOk2cPTLfea,eUlZklb9IXHWAzl3
1
Don't use threading with Tkinter if you don't have to. If you really have to, use Tkinter's TopLevel widget for your UI element, and implement your own event loop. It's a nuisance, to be avoided if possible.
– rd_nielsen
Nov 12 at 1:03
@rd_nielsen: the use of threads is completely unrelated to the use of
Toplevel
. The important thing with threads is that you can only access tkinter objects from a single thread.– Bryan Oakley
Nov 12 at 4:26