How do I add a jsonwebtoken (JWT) to the request header?
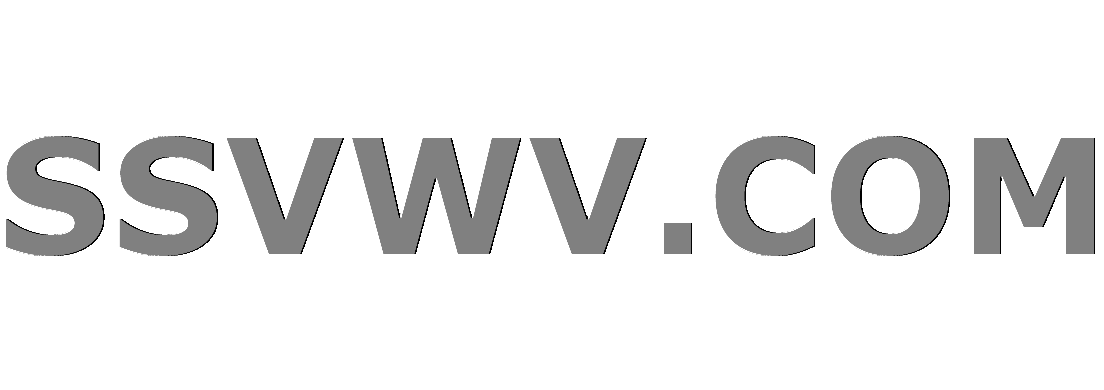
Multi tool use
I have a simple multi-page app written in vanilla JS, Pug, and Node, that uses login with JWT. When a user signs in, the client is returned a JWT. The JWT is stored in localStorage
. Here is my clientside ajax request for when a user logs in and is returned a token:
$.ajax(
url: "/login",
type: "POST",
data: arr ,
success: (res) =>
if (res.status == 200)
localStorage.setItem("token", res.token);
console.log("success");
,
error: (err) => console.log(err)
);
The above works fine.
Each page on the site has a number of different links or buttons: /home
, /profile
, /about
, etc. Some of the routes are protected (only for logged in users), which is why I'm using JWT in the first place. I've seen on YouTube tutorials that the JWT should be stored in localStorage
and sent in requests in the header. I believe I already have serverside middleware that will successfully check if a valid JWT is in the header. My question is this: How do I add the JWT to the request header when a user clicks a link on my page, so I can then do the verification serverside? Is this possible given that I'm using vanilla JS or do I need a different solution?
Here is a typical page for my site (written in Pug).
body
h1 History of changes
div View previous changes
br
each val, ind in histArr
div.history
a(href="/diff/" + val.numb)
button See changes
span= " " + val.date + " " + val.author + " " + val.message
br
a(href="/history")
button /history
a(href="/")
button home
a(href="/profile")
button profile
How do I add the JWT to the header for when a user clicks on these buttons?
EDIT
For example: A user is on my site's home page. They click on a link that is a protected route. I want to check if they are logged in (i.e., have a JWT) before rendering the page. How do I add the JWT to the request header to check that? More specifically, is using an ajax call the only way to add my JWT to the request header or is there another way?
jwt
add a comment |
I have a simple multi-page app written in vanilla JS, Pug, and Node, that uses login with JWT. When a user signs in, the client is returned a JWT. The JWT is stored in localStorage
. Here is my clientside ajax request for when a user logs in and is returned a token:
$.ajax(
url: "/login",
type: "POST",
data: arr ,
success: (res) =>
if (res.status == 200)
localStorage.setItem("token", res.token);
console.log("success");
,
error: (err) => console.log(err)
);
The above works fine.
Each page on the site has a number of different links or buttons: /home
, /profile
, /about
, etc. Some of the routes are protected (only for logged in users), which is why I'm using JWT in the first place. I've seen on YouTube tutorials that the JWT should be stored in localStorage
and sent in requests in the header. I believe I already have serverside middleware that will successfully check if a valid JWT is in the header. My question is this: How do I add the JWT to the request header when a user clicks a link on my page, so I can then do the verification serverside? Is this possible given that I'm using vanilla JS or do I need a different solution?
Here is a typical page for my site (written in Pug).
body
h1 History of changes
div View previous changes
br
each val, ind in histArr
div.history
a(href="/diff/" + val.numb)
button See changes
span= " " + val.date + " " + val.author + " " + val.message
br
a(href="/history")
button /history
a(href="/")
button home
a(href="/profile")
button profile
How do I add the JWT to the header for when a user clicks on these buttons?
EDIT
For example: A user is on my site's home page. They click on a link that is a protected route. I want to check if they are logged in (i.e., have a JWT) before rendering the page. How do I add the JWT to the request header to check that? More specifically, is using an ajax call the only way to add my JWT to the request header or is there another way?
jwt
Possible duplicate of Use basic authentication with jQuery and Ajax
– Suresh Prajapati
Nov 12 at 7:17
add a comment |
I have a simple multi-page app written in vanilla JS, Pug, and Node, that uses login with JWT. When a user signs in, the client is returned a JWT. The JWT is stored in localStorage
. Here is my clientside ajax request for when a user logs in and is returned a token:
$.ajax(
url: "/login",
type: "POST",
data: arr ,
success: (res) =>
if (res.status == 200)
localStorage.setItem("token", res.token);
console.log("success");
,
error: (err) => console.log(err)
);
The above works fine.
Each page on the site has a number of different links or buttons: /home
, /profile
, /about
, etc. Some of the routes are protected (only for logged in users), which is why I'm using JWT in the first place. I've seen on YouTube tutorials that the JWT should be stored in localStorage
and sent in requests in the header. I believe I already have serverside middleware that will successfully check if a valid JWT is in the header. My question is this: How do I add the JWT to the request header when a user clicks a link on my page, so I can then do the verification serverside? Is this possible given that I'm using vanilla JS or do I need a different solution?
Here is a typical page for my site (written in Pug).
body
h1 History of changes
div View previous changes
br
each val, ind in histArr
div.history
a(href="/diff/" + val.numb)
button See changes
span= " " + val.date + " " + val.author + " " + val.message
br
a(href="/history")
button /history
a(href="/")
button home
a(href="/profile")
button profile
How do I add the JWT to the header for when a user clicks on these buttons?
EDIT
For example: A user is on my site's home page. They click on a link that is a protected route. I want to check if they are logged in (i.e., have a JWT) before rendering the page. How do I add the JWT to the request header to check that? More specifically, is using an ajax call the only way to add my JWT to the request header or is there another way?
jwt
I have a simple multi-page app written in vanilla JS, Pug, and Node, that uses login with JWT. When a user signs in, the client is returned a JWT. The JWT is stored in localStorage
. Here is my clientside ajax request for when a user logs in and is returned a token:
$.ajax(
url: "/login",
type: "POST",
data: arr ,
success: (res) =>
if (res.status == 200)
localStorage.setItem("token", res.token);
console.log("success");
,
error: (err) => console.log(err)
);
The above works fine.
Each page on the site has a number of different links or buttons: /home
, /profile
, /about
, etc. Some of the routes are protected (only for logged in users), which is why I'm using JWT in the first place. I've seen on YouTube tutorials that the JWT should be stored in localStorage
and sent in requests in the header. I believe I already have serverside middleware that will successfully check if a valid JWT is in the header. My question is this: How do I add the JWT to the request header when a user clicks a link on my page, so I can then do the verification serverside? Is this possible given that I'm using vanilla JS or do I need a different solution?
Here is a typical page for my site (written in Pug).
body
h1 History of changes
div View previous changes
br
each val, ind in histArr
div.history
a(href="/diff/" + val.numb)
button See changes
span= " " + val.date + " " + val.author + " " + val.message
br
a(href="/history")
button /history
a(href="/")
button home
a(href="/profile")
button profile
How do I add the JWT to the header for when a user clicks on these buttons?
EDIT
For example: A user is on my site's home page. They click on a link that is a protected route. I want to check if they are logged in (i.e., have a JWT) before rendering the page. How do I add the JWT to the request header to check that? More specifically, is using an ajax call the only way to add my JWT to the request header or is there another way?
jwt
jwt
edited Nov 12 at 8:00
asked Nov 12 at 7:12
Scott
6117
6117
Possible duplicate of Use basic authentication with jQuery and Ajax
– Suresh Prajapati
Nov 12 at 7:17
add a comment |
Possible duplicate of Use basic authentication with jQuery and Ajax
– Suresh Prajapati
Nov 12 at 7:17
Possible duplicate of Use basic authentication with jQuery and Ajax
– Suresh Prajapati
Nov 12 at 7:17
Possible duplicate of Use basic authentication with jQuery and Ajax
– Suresh Prajapati
Nov 12 at 7:17
add a comment |
2 Answers
2
active
oldest
votes
Pass header from Ajax
in this way ...
$.ajax({
type: "POST",
beforeSend: function(request)
request.setRequestHeader("token", token); // Set dynamic token
,
url: "/login",
...
);
Another way :
$.ajax(
url: 'YourRestEndPoint',
headers:
'Authorization': token,
'Content-Type':'application/json'
,
method: 'POST',
dataType: 'json',
data: YourData,
success: function(data)
console.log('succes: '+data);
);
View this link
update
Please check this link to pass header in vanilla js.
var httpHandler = function (err, str, contentType)
if (err)
res.writeHead(500, 'Content-Type': 'text/plain');
res.end('An error has occured: ' + err.message);
else
res.writeHead(200, 'Content-Type': contentType);
res.end(str);
;
Now you can try to set near at writeHear
.
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
|
show 1 more comment
You can set headers like this:
$.ajax(
url: 'ajax_url',
...
headers:
'Authorization': 'Bearer ' + 'your_token'
)
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257372%2fhow-do-i-add-a-jsonwebtoken-jwt-to-the-request-header%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Pass header from Ajax
in this way ...
$.ajax({
type: "POST",
beforeSend: function(request)
request.setRequestHeader("token", token); // Set dynamic token
,
url: "/login",
...
);
Another way :
$.ajax(
url: 'YourRestEndPoint',
headers:
'Authorization': token,
'Content-Type':'application/json'
,
method: 'POST',
dataType: 'json',
data: YourData,
success: function(data)
console.log('succes: '+data);
);
View this link
update
Please check this link to pass header in vanilla js.
var httpHandler = function (err, str, contentType)
if (err)
res.writeHead(500, 'Content-Type': 'text/plain');
res.end('An error has occured: ' + err.message);
else
res.writeHead(200, 'Content-Type': contentType);
res.end(str);
;
Now you can try to set near at writeHear
.
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
|
show 1 more comment
Pass header from Ajax
in this way ...
$.ajax({
type: "POST",
beforeSend: function(request)
request.setRequestHeader("token", token); // Set dynamic token
,
url: "/login",
...
);
Another way :
$.ajax(
url: 'YourRestEndPoint',
headers:
'Authorization': token,
'Content-Type':'application/json'
,
method: 'POST',
dataType: 'json',
data: YourData,
success: function(data)
console.log('succes: '+data);
);
View this link
update
Please check this link to pass header in vanilla js.
var httpHandler = function (err, str, contentType)
if (err)
res.writeHead(500, 'Content-Type': 'text/plain');
res.end('An error has occured: ' + err.message);
else
res.writeHead(200, 'Content-Type': contentType);
res.end(str);
;
Now you can try to set near at writeHear
.
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
|
show 1 more comment
Pass header from Ajax
in this way ...
$.ajax({
type: "POST",
beforeSend: function(request)
request.setRequestHeader("token", token); // Set dynamic token
,
url: "/login",
...
);
Another way :
$.ajax(
url: 'YourRestEndPoint',
headers:
'Authorization': token,
'Content-Type':'application/json'
,
method: 'POST',
dataType: 'json',
data: YourData,
success: function(data)
console.log('succes: '+data);
);
View this link
update
Please check this link to pass header in vanilla js.
var httpHandler = function (err, str, contentType)
if (err)
res.writeHead(500, 'Content-Type': 'text/plain');
res.end('An error has occured: ' + err.message);
else
res.writeHead(200, 'Content-Type': contentType);
res.end(str);
;
Now you can try to set near at writeHear
.
Pass header from Ajax
in this way ...
$.ajax({
type: "POST",
beforeSend: function(request)
request.setRequestHeader("token", token); // Set dynamic token
,
url: "/login",
...
);
Another way :
$.ajax(
url: 'YourRestEndPoint',
headers:
'Authorization': token,
'Content-Type':'application/json'
,
method: 'POST',
dataType: 'json',
data: YourData,
success: function(data)
console.log('succes: '+data);
);
View this link
update
Please check this link to pass header in vanilla js.
var httpHandler = function (err, str, contentType)
if (err)
res.writeHead(500, 'Content-Type': 'text/plain');
res.end('An error has occured: ' + err.message);
else
res.writeHead(200, 'Content-Type': contentType);
res.end(str);
;
Now you can try to set near at writeHear
.
edited Nov 21 at 4:38
answered Nov 12 at 7:16


Sachin Shah
1,160414
1,160414
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
|
show 1 more comment
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
So I need every link to make an ajax request if I want to include the token? I can't just have a simple <a href="/profile">PROFILE</a> link any more?
– Scott
Nov 12 at 7:25
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
@Scott I didn't get you. :(
– Sachin Shah
Nov 12 at 7:31
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
If a user is on my site's home page and wants to view their profile, they can click on a link that says "PROFILE." But I want to check if they're logged in before I render their profile. Only if they are logged in, they can go to their profile page. Thus, I want to check if they have a valid JWT when they click the PROFILE link. I have to do this via an ajax request now to set the header? Can't just use a simple link any more?
– Scott
Nov 12 at 7:48
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
@Scott You can check user is logged in or not , then you can checked based on token and in a matter of link and other stuff , It's up to you that how you manage
– Sachin Shah
Nov 12 at 7:50
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
Basically what I think I've come to figure out is this solution only works with a framework like React (maybe Angular, maybe others, I've only worked with React), since you can pass the JWT token to the server with those frameworks and render a view, whereas with Vanilla JS (using ajax) you can't do that and still protect your routes. So since I'm using Vanilla JS I need another solution such as cookies.
– Scott
Nov 21 at 0:23
|
show 1 more comment
You can set headers like this:
$.ajax(
url: 'ajax_url',
...
headers:
'Authorization': 'Bearer ' + 'your_token'
)
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
add a comment |
You can set headers like this:
$.ajax(
url: 'ajax_url',
...
headers:
'Authorization': 'Bearer ' + 'your_token'
)
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
add a comment |
You can set headers like this:
$.ajax(
url: 'ajax_url',
...
headers:
'Authorization': 'Bearer ' + 'your_token'
)
You can set headers like this:
$.ajax(
url: 'ajax_url',
...
headers:
'Authorization': 'Bearer ' + 'your_token'
)
answered Nov 12 at 7:17


Cong Nguyen
31927
31927
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
add a comment |
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
So do I need to do an ajax call for every protected route on my website now? For example, if a user is on my site's home page and wants to view their profile, they can click a link that says "PROFILE", but I want to check to make sure they are signed in (i.e., have a valid JWT) before I render their profile page. So every protected route now needs an ajax request to set the header? I can't set the header separately and still have a simple <a href="/profile">PROFILE</a> link on my page?
– Scott
Nov 12 at 7:46
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
I guess you have to use middleware in this case to send token every request. I don't know to write it in Vanilla but with Vuejs or ReactJs we need do that.
– Cong Nguyen
Nov 12 at 7:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257372%2fhow-do-i-add-a-jsonwebtoken-jwt-to-the-request-header%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Q jS Fx7,xXZ2tS6ZG5
Possible duplicate of Use basic authentication with jQuery and Ajax
– Suresh Prajapati
Nov 12 at 7:17