Firebase Realtime Database - reading nested data
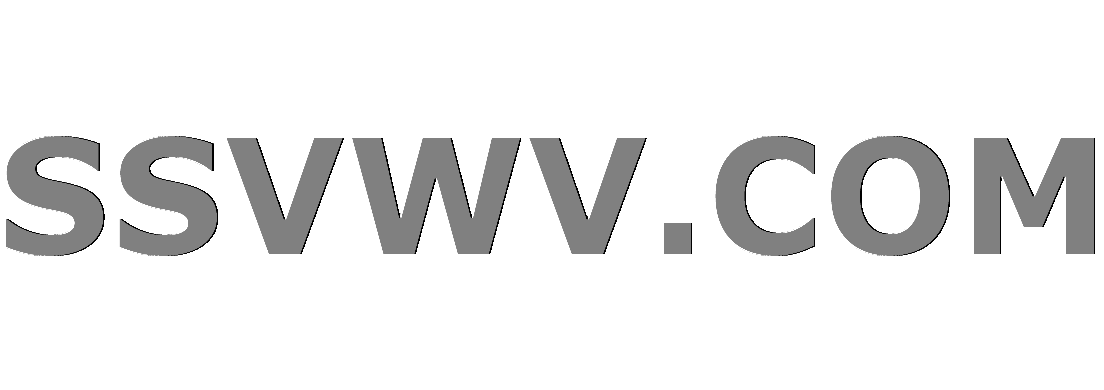
Multi tool use
up vote
1
down vote
favorite
I am doing a project with firebase, able to save some records on the database, but retrieving it has been an issue for me, I've meddled with other posts from SO but they haven't worked for me. This is how the database looks like (An example):
And my code for retrieving the data:
private void readDataFromDB()
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
for (DataSnapshot snapshot : dataSnapshot.getChildren())
user.setStrName(//Get the Name of the user);
user.setStrScore(//Get the Score of the user));
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
The User class:
public class User
String strName, strScore;
public String getStrName()
return strName;
public void setStrName(String strName)
this.strName = strName;
public String getStrScore()
return strScore;
public void setStrScore(String strScore)
this.strScore = strScore;
How can I get the name and score from each specific user


add a comment |
up vote
1
down vote
favorite
I am doing a project with firebase, able to save some records on the database, but retrieving it has been an issue for me, I've meddled with other posts from SO but they haven't worked for me. This is how the database looks like (An example):
And my code for retrieving the data:
private void readDataFromDB()
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
for (DataSnapshot snapshot : dataSnapshot.getChildren())
user.setStrName(//Get the Name of the user);
user.setStrScore(//Get the Score of the user));
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
The User class:
public class User
String strName, strScore;
public String getStrName()
return strName;
public void setStrName(String strName)
this.strName = strName;
public String getStrScore()
return strScore;
public void setStrScore(String strScore)
this.strScore = strScore;
How can I get the name and score from each specific user


You can also check this out.
– Alex Mamo
Nov 12 at 19:03
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am doing a project with firebase, able to save some records on the database, but retrieving it has been an issue for me, I've meddled with other posts from SO but they haven't worked for me. This is how the database looks like (An example):
And my code for retrieving the data:
private void readDataFromDB()
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
for (DataSnapshot snapshot : dataSnapshot.getChildren())
user.setStrName(//Get the Name of the user);
user.setStrScore(//Get the Score of the user));
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
The User class:
public class User
String strName, strScore;
public String getStrName()
return strName;
public void setStrName(String strName)
this.strName = strName;
public String getStrScore()
return strScore;
public void setStrScore(String strScore)
this.strScore = strScore;
How can I get the name and score from each specific user


I am doing a project with firebase, able to save some records on the database, but retrieving it has been an issue for me, I've meddled with other posts from SO but they haven't worked for me. This is how the database looks like (An example):
And my code for retrieving the data:
private void readDataFromDB()
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
for (DataSnapshot snapshot : dataSnapshot.getChildren())
user.setStrName(//Get the Name of the user);
user.setStrScore(//Get the Score of the user));
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
The User class:
public class User
String strName, strScore;
public String getStrName()
return strName;
public void setStrName(String strName)
this.strName = strName;
public String getStrScore()
return strScore;
public void setStrScore(String strScore)
this.strScore = strScore;
How can I get the name and score from each specific user




asked Nov 11 at 14:22
user8284066
365
365
You can also check this out.
– Alex Mamo
Nov 12 at 19:03
add a comment |
You can also check this out.
– Alex Mamo
Nov 12 at 19:03
You can also check this out.
– Alex Mamo
Nov 12 at 19:03
You can also check this out.
– Alex Mamo
Nov 12 at 19:03
add a comment |
2 Answers
2
active
oldest
votes
up vote
3
down vote
In your code, you are setting values, you need to be retrieving values using the getters.
Try the following:
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = dataSnapshot.getValue(User.class);
String name = user.getStrName();
String score = user.getStrScore();
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
But, first you need to add the values to the database example:
User user = new User();
user.setStrName("my_name");
user.setStrScore("20");
DatabaseReference ref = FirebaseDatabase.getInstance().getReference().child("users");
ref.push().setValue(user);
Note setValue()
:
In addition, you can set instances of your own class into this location, provided they satisfy the following constraints:
- The class must have a default constructor that takes no arguments
- The class must define public getters for the properties to be assigned. Properties without a public getter will be set to their default value when an instance is deserialized
You need to add a default constructor to the POJO class public User()
and also the field names in the class should match the ones in the database. So change this String strName, strScore;
into this String name, score;
and generate the getters and setters again.
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then doFirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
If you are just generating a random id do thisString userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
did you try it?
– Peter Haddad
Nov 11 at 17:53
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that isdatabaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
|
show 2 more comments
up vote
0
down vote
Instead of creating profile in every node you can use a global profile node, and in that store the profile data with their UID, which would make it easier for you to fetch detail of single user.
-profile
-UID1
-name
-score
-UID2
-name
-score
While retrieving you can use getCurrentUser.getUid() to retrieve data for each user:
String uid = FirebaseAuth.getInstance().getCurrentUser().getUid();
databaseReference.child("users").child("profile").child(uid).addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
user = dataSnapshot.getValue(User.class);
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
In your code, you are setting values, you need to be retrieving values using the getters.
Try the following:
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = dataSnapshot.getValue(User.class);
String name = user.getStrName();
String score = user.getStrScore();
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
But, first you need to add the values to the database example:
User user = new User();
user.setStrName("my_name");
user.setStrScore("20");
DatabaseReference ref = FirebaseDatabase.getInstance().getReference().child("users");
ref.push().setValue(user);
Note setValue()
:
In addition, you can set instances of your own class into this location, provided they satisfy the following constraints:
- The class must have a default constructor that takes no arguments
- The class must define public getters for the properties to be assigned. Properties without a public getter will be set to their default value when an instance is deserialized
You need to add a default constructor to the POJO class public User()
and also the field names in the class should match the ones in the database. So change this String strName, strScore;
into this String name, score;
and generate the getters and setters again.
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then doFirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
If you are just generating a random id do thisString userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
did you try it?
– Peter Haddad
Nov 11 at 17:53
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that isdatabaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
|
show 2 more comments
up vote
3
down vote
In your code, you are setting values, you need to be retrieving values using the getters.
Try the following:
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = dataSnapshot.getValue(User.class);
String name = user.getStrName();
String score = user.getStrScore();
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
But, first you need to add the values to the database example:
User user = new User();
user.setStrName("my_name");
user.setStrScore("20");
DatabaseReference ref = FirebaseDatabase.getInstance().getReference().child("users");
ref.push().setValue(user);
Note setValue()
:
In addition, you can set instances of your own class into this location, provided they satisfy the following constraints:
- The class must have a default constructor that takes no arguments
- The class must define public getters for the properties to be assigned. Properties without a public getter will be set to their default value when an instance is deserialized
You need to add a default constructor to the POJO class public User()
and also the field names in the class should match the ones in the database. So change this String strName, strScore;
into this String name, score;
and generate the getters and setters again.
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then doFirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
If you are just generating a random id do thisString userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
did you try it?
– Peter Haddad
Nov 11 at 17:53
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that isdatabaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
|
show 2 more comments
up vote
3
down vote
up vote
3
down vote
In your code, you are setting values, you need to be retrieving values using the getters.
Try the following:
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = dataSnapshot.getValue(User.class);
String name = user.getStrName();
String score = user.getStrScore();
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
But, first you need to add the values to the database example:
User user = new User();
user.setStrName("my_name");
user.setStrScore("20");
DatabaseReference ref = FirebaseDatabase.getInstance().getReference().child("users");
ref.push().setValue(user);
Note setValue()
:
In addition, you can set instances of your own class into this location, provided they satisfy the following constraints:
- The class must have a default constructor that takes no arguments
- The class must define public getters for the properties to be assigned. Properties without a public getter will be set to their default value when an instance is deserialized
You need to add a default constructor to the POJO class public User()
and also the field names in the class should match the ones in the database. So change this String strName, strScore;
into this String name, score;
and generate the getters and setters again.
In your code, you are setting values, you need to be retrieving values using the getters.
Try the following:
databaseReference.child("users").addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = dataSnapshot.getValue(User.class);
String name = user.getStrName();
String score = user.getStrScore();
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
But, first you need to add the values to the database example:
User user = new User();
user.setStrName("my_name");
user.setStrScore("20");
DatabaseReference ref = FirebaseDatabase.getInstance().getReference().child("users");
ref.push().setValue(user);
Note setValue()
:
In addition, you can set instances of your own class into this location, provided they satisfy the following constraints:
- The class must have a default constructor that takes no arguments
- The class must define public getters for the properties to be assigned. Properties without a public getter will be set to their default value when an instance is deserialized
You need to add a default constructor to the POJO class public User()
and also the field names in the class should match the ones in the database. So change this String strName, strScore;
into this String name, score;
and generate the getters and setters again.
answered Nov 11 at 14:48


Peter Haddad
19.8k83955
19.8k83955
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then doFirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
If you are just generating a random id do thisString userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
did you try it?
– Peter Haddad
Nov 11 at 17:53
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that isdatabaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
|
show 2 more comments
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then doFirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
If you are just generating a random id do thisString userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
did you try it?
– Peter Haddad
Nov 11 at 17:53
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that isdatabaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
I modified my POJO class to match the field names. Do I have to set the data to the POJO first before I push to database, secondly, how do I get the userID, since it is a specific random value for every user
– user8284066
Nov 11 at 16:39
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then do
FirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
yes first set the data to add to the database then retrieve, by userid do you mean the id from firebase authentication? If so then do
FirebaseUser currentFirebaseUser = FirebaseAuth.getInstance().getCurrentUser() ; String userId=currentFirebaseUser.getUid();
– Peter Haddad
Nov 11 at 16:41
If you are just generating a random id do this
String userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
If you are just generating a random id do this
String userId=ref.push().getKey(); ref.child(userId).setValue(user);
– Peter Haddad
Nov 11 at 16:42
did you try it?
– Peter Haddad
Nov 11 at 17:53
did you try it?
– Peter Haddad
Nov 11 at 17:53
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that is
databaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
Yes sir, but my issue was that I'm trying to display all the names and their respective columns, after getting the users, that is
databaseReference.child("users").child(//what to pass here to get Firebase authentication id ->getKey() won't allow me to add another child chain after it).child("profile").addValueEventListener…
– user8284066
Nov 12 at 3:31
|
show 2 more comments
up vote
0
down vote
Instead of creating profile in every node you can use a global profile node, and in that store the profile data with their UID, which would make it easier for you to fetch detail of single user.
-profile
-UID1
-name
-score
-UID2
-name
-score
While retrieving you can use getCurrentUser.getUid() to retrieve data for each user:
String uid = FirebaseAuth.getInstance().getCurrentUser().getUid();
databaseReference.child("users").child("profile").child(uid).addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
user = dataSnapshot.getValue(User.class);
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
add a comment |
up vote
0
down vote
Instead of creating profile in every node you can use a global profile node, and in that store the profile data with their UID, which would make it easier for you to fetch detail of single user.
-profile
-UID1
-name
-score
-UID2
-name
-score
While retrieving you can use getCurrentUser.getUid() to retrieve data for each user:
String uid = FirebaseAuth.getInstance().getCurrentUser().getUid();
databaseReference.child("users").child("profile").child(uid).addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
user = dataSnapshot.getValue(User.class);
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
add a comment |
up vote
0
down vote
up vote
0
down vote
Instead of creating profile in every node you can use a global profile node, and in that store the profile data with their UID, which would make it easier for you to fetch detail of single user.
-profile
-UID1
-name
-score
-UID2
-name
-score
While retrieving you can use getCurrentUser.getUid() to retrieve data for each user:
String uid = FirebaseAuth.getInstance().getCurrentUser().getUid();
databaseReference.child("users").child("profile").child(uid).addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
user = dataSnapshot.getValue(User.class);
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
Instead of creating profile in every node you can use a global profile node, and in that store the profile data with their UID, which would make it easier for you to fetch detail of single user.
-profile
-UID1
-name
-score
-UID2
-name
-score
While retrieving you can use getCurrentUser.getUid() to retrieve data for each user:
String uid = FirebaseAuth.getInstance().getCurrentUser().getUid();
databaseReference.child("users").child("profile").child(uid).addValueEventListener(new ValueEventListener()
@Override
public void onDataChange(@NonNull DataSnapshot dataSnapshot)
User user = new User();
user = dataSnapshot.getValue(User.class);
@Override
public void onCancelled(@NonNull DatabaseError databaseError)
);
answered Nov 12 at 7:43
ManishPrajapati
211313
211313
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249652%2ffirebase-realtime-database-reading-nested-data%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ijt8FV1uGn TT7GU8WBJuzG FzI9u4sD7H0JIb8eGMuEBO 0nWO1U,uP
You can also check this out.
– Alex Mamo
Nov 12 at 19:03