Filter array of objects by comparing their properties
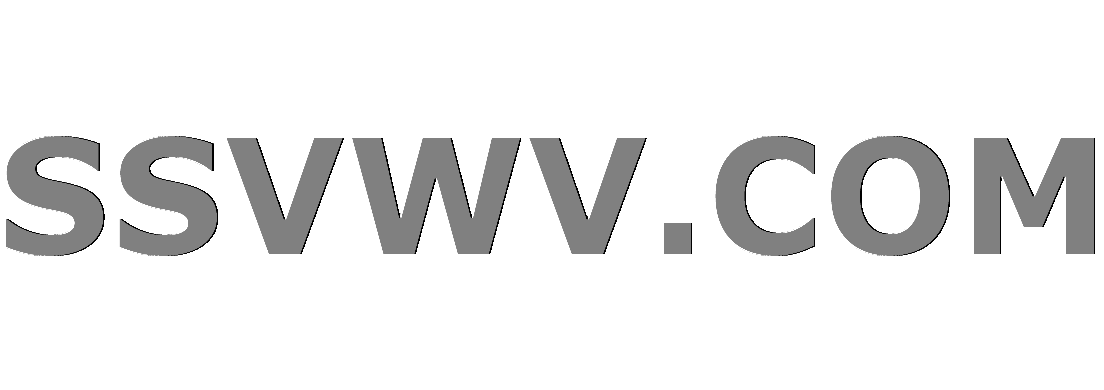
Multi tool use
up vote
1
down vote
favorite
I tried to find the highest score on an object in this array, but the result is incorrect. Should we reset the score to be 0 again? I was trying to put the score variable below the obj[i.class]
but nothing changed:
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(score < i.score)
obj[i.class] =
name: i.name,
score: i.score
;
;
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
Desired output:
A:
name: 'Sara',
score: 90
,
B:
name: 'Poyi',
score: 85
javascript arrays object
add a comment |
up vote
1
down vote
favorite
I tried to find the highest score on an object in this array, but the result is incorrect. Should we reset the score to be 0 again? I was trying to put the score variable below the obj[i.class]
but nothing changed:
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(score < i.score)
obj[i.class] =
name: i.name,
score: i.score
;
;
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
Desired output:
A:
name: 'Sara',
score: 90
,
B:
name: 'Poyi',
score: 85
javascript arrays object
Not clear if you want the highest overall score, or the highest score for each class. Looks like it is the latter, but please clarify.
– Matt Morgan
Nov 11 at 13:57
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I tried to find the highest score on an object in this array, but the result is incorrect. Should we reset the score to be 0 again? I was trying to put the score variable below the obj[i.class]
but nothing changed:
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(score < i.score)
obj[i.class] =
name: i.name,
score: i.score
;
;
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
Desired output:
A:
name: 'Sara',
score: 90
,
B:
name: 'Poyi',
score: 85
javascript arrays object
I tried to find the highest score on an object in this array, but the result is incorrect. Should we reset the score to be 0 again? I was trying to put the score variable below the obj[i.class]
but nothing changed:
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(score < i.score)
obj[i.class] =
name: i.name,
score: i.score
;
;
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
Desired output:
A:
name: 'Sara',
score: 90
,
B:
name: 'Poyi',
score: 85
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(score < i.score)
obj[i.class] =
name: i.name,
score: i.score
;
;
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(score < i.score)
obj[i.class] =
name: i.name,
score: i.score
;
;
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
javascript arrays object
javascript arrays object
edited Nov 11 at 21:13
Matt Morgan
2,1062720
2,1062720
asked Nov 11 at 13:37
Pi L
164
164
Not clear if you want the highest overall score, or the highest score for each class. Looks like it is the latter, but please clarify.
– Matt Morgan
Nov 11 at 13:57
add a comment |
Not clear if you want the highest overall score, or the highest score for each class. Looks like it is the latter, but please clarify.
– Matt Morgan
Nov 11 at 13:57
Not clear if you want the highest overall score, or the highest score for each class. Looks like it is the latter, but please clarify.
– Matt Morgan
Nov 11 at 13:57
Not clear if you want the highest overall score, or the highest score for each class. Looks like it is the latter, but please clarify.
– Matt Morgan
Nov 11 at 13:57
add a comment |
5 Answers
5
active
oldest
votes
up vote
0
down vote
accepted
The issue with your code was never actually comparing the the actual object score but to 0 always.
If you really want to use for in
loop for this you can do something like (with slight changes to your code):
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
Or you can use reduce
and solve it like this:
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
Since reduce returns an accumulator we simply need to assign the correct object prop based on which one of the 2 classes scores is larger and return.
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
add a comment |
up vote
1
down vote
Can't you massively simplify your function's obj assignment?
function theScore (students)
var obj = ;
var score = 0;
for(i of students)
if(i.score > score )
score = i.score; // Keep track of the highest-score-found-so-far
obj = i; // Keep track of the highest scoring object
return obj;
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
i want to print class a and b
– Pi L
Nov 11 at 14:04
look my edited question for output :D
– Pi L
Nov 11 at 14:08
add a comment |
up vote
0
down vote
The score is not being changed after you find the highest score. Change the score to the current score. Thanks. Instead of an object with map, use an object with index(Array). This should work.
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(obj.length === 0)
obj.push( i );
else
for( var x = 0; x < obj.length; x++ )
if( i.score > obj[x].score )
obj.splice( x, 0, i );
break;
if( x === obj.length )
obj.push(i);
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
thank you to help me
– Pi L
Nov 11 at 14:19
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
|
show 3 more comments
up vote
0
down vote
You need to track the already checked classes
.
A cleaner alternative is using the function Array.prototype.reduce
to build the desired output. As you can see, basically, this approach is keeping a track of the previously checked classes
.
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
add a comment |
up vote
0
down vote
The problem with your code is that you don't make any distinction between students' scores in one class versus another class. You also are not updating the score when you find a higher one, so every student's score (unless it's 0) will be higher than score
every time your loop runs, and you just end up with the last score in the list.
EDIT: edited to show how to do this with only one loop
You need to:
- Track score for each class
- Loop over the students
- Compare that student's score to the class high score for that student's class
- Update as needed
- Repeat until done.
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
The issue with your code was never actually comparing the the actual object score but to 0 always.
If you really want to use for in
loop for this you can do something like (with slight changes to your code):
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
Or you can use reduce
and solve it like this:
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
Since reduce returns an accumulator we simply need to assign the correct object prop based on which one of the 2 classes scores is larger and return.
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
add a comment |
up vote
0
down vote
accepted
The issue with your code was never actually comparing the the actual object score but to 0 always.
If you really want to use for in
loop for this you can do something like (with slight changes to your code):
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
Or you can use reduce
and solve it like this:
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
Since reduce returns an accumulator we simply need to assign the correct object prop based on which one of the 2 classes scores is larger and return.
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
The issue with your code was never actually comparing the the actual object score but to 0 always.
If you really want to use for in
loop for this you can do something like (with slight changes to your code):
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
Or you can use reduce
and solve it like this:
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
Since reduce returns an accumulator we simply need to assign the correct object prop based on which one of the 2 classes scores is larger and return.
The issue with your code was never actually comparing the the actual object score but to 0 always.
If you really want to use for in
loop for this you can do something like (with slight changes to your code):
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
Or you can use reduce
and solve it like this:
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
Since reduce returns an accumulator we simply need to assign the correct object prop based on which one of the 2 classes scores is larger and return.
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
function theScore (students)
var obj =
for(i of students) obj[i.class].score < i.score)
obj[i.class] = name: i.name, score: i.score
return obj
console.log(theScore(data))
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
var data = [ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]
const maxByClass = (d) => d.reduce((r,name, score, ...c) =>
r[c.class] = r[c.class] ? r[c.class].score > score ? r[c.class] : name, score : name, score
return r
, )
console.log(maxByClass(data))
edited Nov 11 at 21:11
answered Nov 11 at 20:57


Akrion
8,80411223
8,80411223
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
add a comment |
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
the first what i want , thank you :D
– Pi L
Nov 11 at 22:40
add a comment |
up vote
1
down vote
Can't you massively simplify your function's obj assignment?
function theScore (students)
var obj = ;
var score = 0;
for(i of students)
if(i.score > score )
score = i.score; // Keep track of the highest-score-found-so-far
obj = i; // Keep track of the highest scoring object
return obj;
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
i want to print class a and b
– Pi L
Nov 11 at 14:04
look my edited question for output :D
– Pi L
Nov 11 at 14:08
add a comment |
up vote
1
down vote
Can't you massively simplify your function's obj assignment?
function theScore (students)
var obj = ;
var score = 0;
for(i of students)
if(i.score > score )
score = i.score; // Keep track of the highest-score-found-so-far
obj = i; // Keep track of the highest scoring object
return obj;
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
i want to print class a and b
– Pi L
Nov 11 at 14:04
look my edited question for output :D
– Pi L
Nov 11 at 14:08
add a comment |
up vote
1
down vote
up vote
1
down vote
Can't you massively simplify your function's obj assignment?
function theScore (students)
var obj = ;
var score = 0;
for(i of students)
if(i.score > score )
score = i.score; // Keep track of the highest-score-found-so-far
obj = i; // Keep track of the highest scoring object
return obj;
Can't you massively simplify your function's obj assignment?
function theScore (students)
var obj = ;
var score = 0;
for(i of students)
if(i.score > score )
score = i.score; // Keep track of the highest-score-found-so-far
obj = i; // Keep track of the highest scoring object
return obj;
answered Nov 11 at 13:54
Vexen Crabtree
166113
166113
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
i want to print class a and b
– Pi L
Nov 11 at 14:04
look my edited question for output :D
– Pi L
Nov 11 at 14:08
add a comment |
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
i want to print class a and b
– Pi L
Nov 11 at 14:04
look my edited question for output :D
– Pi L
Nov 11 at 14:08
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
it just print the A class!!!!
– Pi L
Nov 11 at 14:01
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
If your original query was correct that "i tried fio find the higest score on this object in array", then, the A-class is the highest score, and it prints the A-class. So it looks like it works to me!
– Vexen Crabtree
Nov 11 at 14:03
i want to print class a and b
– Pi L
Nov 11 at 14:04
i want to print class a and b
– Pi L
Nov 11 at 14:04
look my edited question for output :D
– Pi L
Nov 11 at 14:08
look my edited question for output :D
– Pi L
Nov 11 at 14:08
add a comment |
up vote
0
down vote
The score is not being changed after you find the highest score. Change the score to the current score. Thanks. Instead of an object with map, use an object with index(Array). This should work.
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(obj.length === 0)
obj.push( i );
else
for( var x = 0; x < obj.length; x++ )
if( i.score > obj[x].score )
obj.splice( x, 0, i );
break;
if( x === obj.length )
obj.push(i);
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
thank you to help me
– Pi L
Nov 11 at 14:19
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
|
show 3 more comments
up vote
0
down vote
The score is not being changed after you find the highest score. Change the score to the current score. Thanks. Instead of an object with map, use an object with index(Array). This should work.
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(obj.length === 0)
obj.push( i );
else
for( var x = 0; x < obj.length; x++ )
if( i.score > obj[x].score )
obj.splice( x, 0, i );
break;
if( x === obj.length )
obj.push(i);
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
thank you to help me
– Pi L
Nov 11 at 14:19
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
|
show 3 more comments
up vote
0
down vote
up vote
0
down vote
The score is not being changed after you find the highest score. Change the score to the current score. Thanks. Instead of an object with map, use an object with index(Array). This should work.
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(obj.length === 0)
obj.push( i );
else
for( var x = 0; x < obj.length; x++ )
if( i.score > obj[x].score )
obj.splice( x, 0, i );
break;
if( x === obj.length )
obj.push(i);
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
The score is not being changed after you find the highest score. Change the score to the current score. Thanks. Instead of an object with map, use an object with index(Array). This should work.
function theScore (students)
var obj = ;
score = 0;
for(i of students)
if(obj.length === 0)
obj.push( i );
else
for( var x = 0; x < obj.length; x++ )
if( i.score > obj[x].score )
obj.splice( x, 0, i );
break;
if( x === obj.length )
obj.push(i);
;
return obj;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
edited Nov 11 at 14:25
answered Nov 11 at 13:54


Edwin Dijas Chiwona
34117
34117
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
thank you to help me
– Pi L
Nov 11 at 14:19
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
|
show 3 more comments
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
thank you to help me
– Pi L
Nov 11 at 14:19
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
it just print the highest score of class A, i want both class
– Pi L
Nov 11 at 14:03
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
look my edited question for output :D @edwin
– Pi L
Nov 11 at 14:08
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
Alright let me look at it
– Edwin Dijas Chiwona
Nov 11 at 14:11
thank you to help me
– Pi L
Nov 11 at 14:19
thank you to help me
– Pi L
Nov 11 at 14:19
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
It's done, don't forget to mark answer as useful. if it helps.
– Edwin Dijas Chiwona
Nov 11 at 14:26
|
show 3 more comments
up vote
0
down vote
You need to track the already checked classes
.
A cleaner alternative is using the function Array.prototype.reduce
to build the desired output. As you can see, basically, this approach is keeping a track of the previously checked classes
.
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
add a comment |
up vote
0
down vote
You need to track the already checked classes
.
A cleaner alternative is using the function Array.prototype.reduce
to build the desired output. As you can see, basically, this approach is keeping a track of the previously checked classes
.
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
add a comment |
up vote
0
down vote
up vote
0
down vote
You need to track the already checked classes
.
A cleaner alternative is using the function Array.prototype.reduce
to build the desired output. As you can see, basically, this approach is keeping a track of the previously checked classes
.
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
You need to track the already checked classes
.
A cleaner alternative is using the function Array.prototype.reduce
to build the desired output. As you can see, basically, this approach is keeping a track of the previously checked classes
.
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
function theScore (students)
return students.reduce((a, c) =>
a[c.class] = (a[c.class] , Object.create(null));
console.log(theScore([ name: 'Sara', score: 90, class: 'A' , name: 'Poyi', score: 85, class: 'B' , name: 'Adert', score: 74, class: 'A' , name: 'Shynta', score: 78, class: 'B' ]));
.as-console-wrapper max-height: 100% !important; top: 0;
answered Nov 11 at 15:03


Ele
22.6k42044
22.6k42044
add a comment |
add a comment |
up vote
0
down vote
The problem with your code is that you don't make any distinction between students' scores in one class versus another class. You also are not updating the score when you find a higher one, so every student's score (unless it's 0) will be higher than score
every time your loop runs, and you just end up with the last score in the list.
EDIT: edited to show how to do this with only one loop
You need to:
- Track score for each class
- Loop over the students
- Compare that student's score to the class high score for that student's class
- Update as needed
- Repeat until done.
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
add a comment |
up vote
0
down vote
The problem with your code is that you don't make any distinction between students' scores in one class versus another class. You also are not updating the score when you find a higher one, so every student's score (unless it's 0) will be higher than score
every time your loop runs, and you just end up with the last score in the list.
EDIT: edited to show how to do this with only one loop
You need to:
- Track score for each class
- Loop over the students
- Compare that student's score to the class high score for that student's class
- Update as needed
- Repeat until done.
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
add a comment |
up vote
0
down vote
up vote
0
down vote
The problem with your code is that you don't make any distinction between students' scores in one class versus another class. You also are not updating the score when you find a higher one, so every student's score (unless it's 0) will be higher than score
every time your loop runs, and you just end up with the last score in the list.
EDIT: edited to show how to do this with only one loop
You need to:
- Track score for each class
- Loop over the students
- Compare that student's score to the class high score for that student's class
- Update as needed
- Repeat until done.
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
The problem with your code is that you don't make any distinction between students' scores in one class versus another class. You also are not updating the score when you find a higher one, so every student's score (unless it's 0) will be higher than score
every time your loop runs, and you just end up with the last score in the list.
EDIT: edited to show how to do this with only one loop
You need to:
- Track score for each class
- Loop over the students
- Compare that student's score to the class high score for that student's class
- Update as needed
- Repeat until done.
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
function theScore (students)
const highScoresByClass = ;
const scores = A: 0, B: 0, C: 0;
for (const student of students)
const classHighScore = scores[student.class];
if(classHighScore < student.score)
scores[student.class] = student.score;
highScoresByClass[student.class] =
name: student.name,
score: student.score
;
;
;
return highScoresByClass;
;
console.log(theScore([
name: 'Sara',
score: 90,
class: 'A'
,
name: 'Poyi',
score: 85,
class: 'B'
,
name: 'Adert',
score: 74,
class: 'A'
,
name: 'Shynta',
score: 78,
class: 'B'
]));
edited Nov 11 at 19:14
answered Nov 11 at 13:54
Matt Morgan
2,1062720
2,1062720
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
add a comment |
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
cant we dont loop for twice times??
– Pi L
Nov 11 at 14:08
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
@PiL I have edited my answer to show how this can be done in a single loop.
– Matt Morgan
Nov 11 at 19:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249292%2ffilter-array-of-objects-by-comparing-their-properties%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OrXYU12bbUiNM m0r
Not clear if you want the highest overall score, or the highest score for each class. Looks like it is the latter, but please clarify.
– Matt Morgan
Nov 11 at 13:57