Using MVC HtmlHelpers, how to send a form with a value from database along with User-filled values?
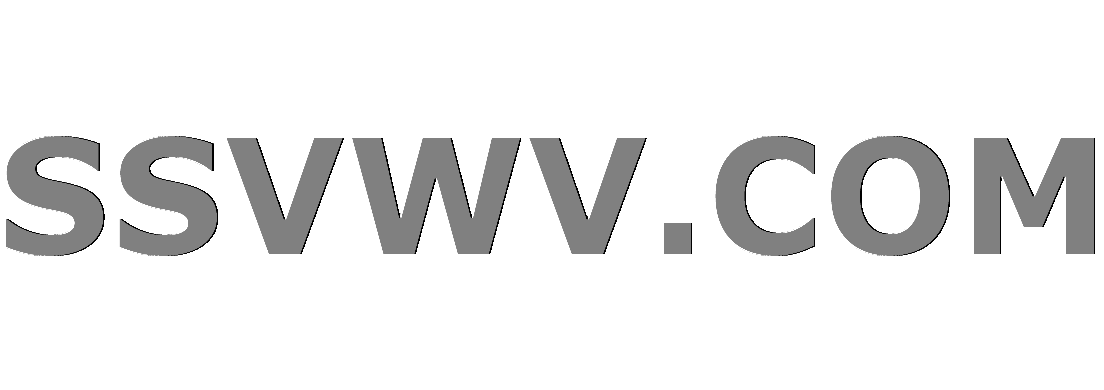
Multi tool use
up vote
0
down vote
favorite
I'm trying to write a Bank Application. In the Transaction Page, I want to take the value of CheckingAccountId from the User Accounts Database (User.Identity.GetUserId()). But, how should I assign the value of User.Identity.GetUserId() to CheckingAccountId. This is the relevant Razor View Page Code:
using (Html.BeginForm("Deposit", "Transaction", FormMethod.Post, new
@class = "form-horizontal", role = "form" ))
{
@Html.AntiForgeryToken();
<h4>Deposit Section</h4>
<hr />
@Html.ValidationSummary("", new @class = "text-danger" )
<div class="form-group">
@Html.LabelFor(m => m.Id, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.Id, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.TransactionAmount, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.TransactionAmount, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.CheckingAccountId, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@
var userName = User.Identity.GetUserName();
@*THIS IS THE BEST I COULD DO. I NEED HELP HERE*@
@*@m.CheckingAccountId = userName*@
@Html.DisplayName(userName)**
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" class="btn btn-default" value="Deposit" />
</div>
</div>
UPDATE:
I just found out that we can achieve this by adding the same in the ConnectionString when writing the SQL Query for pushing the transaction into the database. The solution would look something like this:
INSERT INTO table ( checkingaccountid, model_data ) VALUES ( $id, $data );
But I would still like to know if it's possible to send this id from the form when submitted along with the other data.
asp.net-mvc razor html-helper
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I'm trying to write a Bank Application. In the Transaction Page, I want to take the value of CheckingAccountId from the User Accounts Database (User.Identity.GetUserId()). But, how should I assign the value of User.Identity.GetUserId() to CheckingAccountId. This is the relevant Razor View Page Code:
using (Html.BeginForm("Deposit", "Transaction", FormMethod.Post, new
@class = "form-horizontal", role = "form" ))
{
@Html.AntiForgeryToken();
<h4>Deposit Section</h4>
<hr />
@Html.ValidationSummary("", new @class = "text-danger" )
<div class="form-group">
@Html.LabelFor(m => m.Id, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.Id, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.TransactionAmount, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.TransactionAmount, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.CheckingAccountId, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@
var userName = User.Identity.GetUserName();
@*THIS IS THE BEST I COULD DO. I NEED HELP HERE*@
@*@m.CheckingAccountId = userName*@
@Html.DisplayName(userName)**
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" class="btn btn-default" value="Deposit" />
</div>
</div>
UPDATE:
I just found out that we can achieve this by adding the same in the ConnectionString when writing the SQL Query for pushing the transaction into the database. The solution would look something like this:
INSERT INTO table ( checkingaccountid, model_data ) VALUES ( $id, $data );
But I would still like to know if it's possible to send this id from the form when submitted along with the other data.
asp.net-mvc razor html-helper
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Why would you want to include the value in your form (a malicious user could just change it to anything). You set the value in the POST method immediately before you save the record.
– Stephen Muecke
Nov 11 at 3:38
It is best to keep secret codes within the premises of your PostMethod. Like Stephen outlined, it is not recommended to let a malicious user to intercept your requests.
– Ashokan Sivapragasam
Nov 12 at 11:29
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to write a Bank Application. In the Transaction Page, I want to take the value of CheckingAccountId from the User Accounts Database (User.Identity.GetUserId()). But, how should I assign the value of User.Identity.GetUserId() to CheckingAccountId. This is the relevant Razor View Page Code:
using (Html.BeginForm("Deposit", "Transaction", FormMethod.Post, new
@class = "form-horizontal", role = "form" ))
{
@Html.AntiForgeryToken();
<h4>Deposit Section</h4>
<hr />
@Html.ValidationSummary("", new @class = "text-danger" )
<div class="form-group">
@Html.LabelFor(m => m.Id, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.Id, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.TransactionAmount, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.TransactionAmount, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.CheckingAccountId, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@
var userName = User.Identity.GetUserName();
@*THIS IS THE BEST I COULD DO. I NEED HELP HERE*@
@*@m.CheckingAccountId = userName*@
@Html.DisplayName(userName)**
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" class="btn btn-default" value="Deposit" />
</div>
</div>
UPDATE:
I just found out that we can achieve this by adding the same in the ConnectionString when writing the SQL Query for pushing the transaction into the database. The solution would look something like this:
INSERT INTO table ( checkingaccountid, model_data ) VALUES ( $id, $data );
But I would still like to know if it's possible to send this id from the form when submitted along with the other data.
asp.net-mvc razor html-helper
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I'm trying to write a Bank Application. In the Transaction Page, I want to take the value of CheckingAccountId from the User Accounts Database (User.Identity.GetUserId()). But, how should I assign the value of User.Identity.GetUserId() to CheckingAccountId. This is the relevant Razor View Page Code:
using (Html.BeginForm("Deposit", "Transaction", FormMethod.Post, new
@class = "form-horizontal", role = "form" ))
{
@Html.AntiForgeryToken();
<h4>Deposit Section</h4>
<hr />
@Html.ValidationSummary("", new @class = "text-danger" )
<div class="form-group">
@Html.LabelFor(m => m.Id, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.Id, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.TransactionAmount, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@Html.TextBoxFor(m => m.TransactionAmount, new @class = "form-control" )
</div>
</div>
<div class="form-group">
@Html.LabelFor(m => m.CheckingAccountId, new @class = "col-md-2 control-label" )
<div class="col-md-10">
@
var userName = User.Identity.GetUserName();
@*THIS IS THE BEST I COULD DO. I NEED HELP HERE*@
@*@m.CheckingAccountId = userName*@
@Html.DisplayName(userName)**
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" class="btn btn-default" value="Deposit" />
</div>
</div>
UPDATE:
I just found out that we can achieve this by adding the same in the ConnectionString when writing the SQL Query for pushing the transaction into the database. The solution would look something like this:
INSERT INTO table ( checkingaccountid, model_data ) VALUES ( $id, $data );
But I would still like to know if it's possible to send this id from the form when submitted along with the other data.
asp.net-mvc razor html-helper
asp.net-mvc razor html-helper
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Nov 11 at 7:41
Mike Brind
16.8k53768
16.8k53768
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 10 at 14:51
Venkatesh Gunda
11
11
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Venkatesh Gunda is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
Why would you want to include the value in your form (a malicious user could just change it to anything). You set the value in the POST method immediately before you save the record.
– Stephen Muecke
Nov 11 at 3:38
It is best to keep secret codes within the premises of your PostMethod. Like Stephen outlined, it is not recommended to let a malicious user to intercept your requests.
– Ashokan Sivapragasam
Nov 12 at 11:29
add a comment |
1
Why would you want to include the value in your form (a malicious user could just change it to anything). You set the value in the POST method immediately before you save the record.
– Stephen Muecke
Nov 11 at 3:38
It is best to keep secret codes within the premises of your PostMethod. Like Stephen outlined, it is not recommended to let a malicious user to intercept your requests.
– Ashokan Sivapragasam
Nov 12 at 11:29
1
1
Why would you want to include the value in your form (a malicious user could just change it to anything). You set the value in the POST method immediately before you save the record.
– Stephen Muecke
Nov 11 at 3:38
Why would you want to include the value in your form (a malicious user could just change it to anything). You set the value in the POST method immediately before you save the record.
– Stephen Muecke
Nov 11 at 3:38
It is best to keep secret codes within the premises of your PostMethod. Like Stephen outlined, it is not recommended to let a malicious user to intercept your requests.
– Ashokan Sivapragasam
Nov 12 at 11:29
It is best to keep secret codes within the premises of your PostMethod. Like Stephen outlined, it is not recommended to let a malicious user to intercept your requests.
– Ashokan Sivapragasam
Nov 12 at 11:29
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Venkatesh Gunda is a new contributor. Be nice, and check out our Code of Conduct.
Venkatesh Gunda is a new contributor. Be nice, and check out our Code of Conduct.
Venkatesh Gunda is a new contributor. Be nice, and check out our Code of Conduct.
Venkatesh Gunda is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240118%2fusing-mvc-htmlhelpers-how-to-send-a-form-with-a-value-from-database-along-with%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
B3IwM1A,tpfgFBi N74KS9TF,KB,mzQg0I4 x,dD ib7jF DMRq6YZSFDAdsvllBT,n tqhptorO45
1
Why would you want to include the value in your form (a malicious user could just change it to anything). You set the value in the POST method immediately before you save the record.
– Stephen Muecke
Nov 11 at 3:38
It is best to keep secret codes within the premises of your PostMethod. Like Stephen outlined, it is not recommended to let a malicious user to intercept your requests.
– Ashokan Sivapragasam
Nov 12 at 11:29