accessing specific values in a table in sqlite3 of python 3 and then updating it
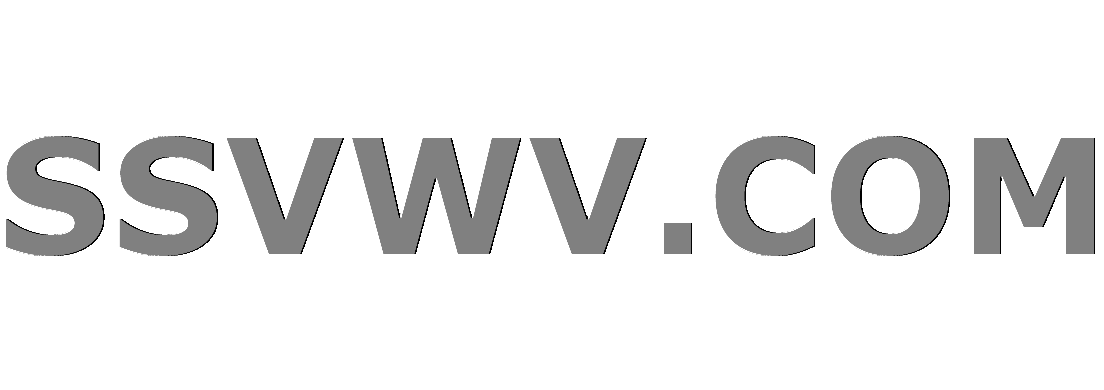
Multi tool use
up vote
0
down vote
favorite
I have created a database table which is basically a simple atm database which has records of some people with their pin
as the primary key. Now I need to create another file where a user can enter their pin
and then it checks if the pin
exists in the database and if it does it shows how much money he has and on entering the amount to withdraw, the database gets updated with a new value of amount
in that person's record. The table I created is as such:
import sqlite3
conn = sqlite3.connect('pin.db')
print("opened successfully")
conn.execute('''CREATE TABLE CUSTOMERS
(ID INT PRIMARY KEY NOT NULL,
NAME TEXT NOT NULL,
AGE INT NOT NULL,
ADDRESS CHAR(50),
AMOUNT INT);''')
print("table created")
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (1234, 'Paul', 32, 'California', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (2345, 'Allen', 25, 'Texas', 15000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (3456, 'Teddy', 23, 'Norway', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (4567, 'Mark', 25, 'Rich-Mond ', 65000.00 )");
conn.commit()
print ("Records created successfully")
conn.close()
The other file will be something like this I guess:
import sqlite3
conn = sqlite3.connect('pin.db')
print ("Opened database successfully")
pin=int(input("enter the 4 digit pin: "))
conn.row_factory = lambda cursor, row: row[0]
c = conn.cursor()
ids = c.execute('SELECT id FROM CUSTOMERS').fetchall()
if pin in ids:
query= f"SELECT AMOUNT FROM CUSTOMERS WHERE ID=?"
c.execute(query,(pin))
print(c.fetchone())
else:
print("no")
The problem is that I'm getting a Value Error as such: ValueError: parameters are of unsupported type
, I know the solution might be something very simple and basic but the thing is I'm completely new to this sqlite3 thing and so not good with it. Explanation will be of great help for me to learn.
sqlite3 python-3.7
add a comment |
up vote
0
down vote
favorite
I have created a database table which is basically a simple atm database which has records of some people with their pin
as the primary key. Now I need to create another file where a user can enter their pin
and then it checks if the pin
exists in the database and if it does it shows how much money he has and on entering the amount to withdraw, the database gets updated with a new value of amount
in that person's record. The table I created is as such:
import sqlite3
conn = sqlite3.connect('pin.db')
print("opened successfully")
conn.execute('''CREATE TABLE CUSTOMERS
(ID INT PRIMARY KEY NOT NULL,
NAME TEXT NOT NULL,
AGE INT NOT NULL,
ADDRESS CHAR(50),
AMOUNT INT);''')
print("table created")
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (1234, 'Paul', 32, 'California', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (2345, 'Allen', 25, 'Texas', 15000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (3456, 'Teddy', 23, 'Norway', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (4567, 'Mark', 25, 'Rich-Mond ', 65000.00 )");
conn.commit()
print ("Records created successfully")
conn.close()
The other file will be something like this I guess:
import sqlite3
conn = sqlite3.connect('pin.db')
print ("Opened database successfully")
pin=int(input("enter the 4 digit pin: "))
conn.row_factory = lambda cursor, row: row[0]
c = conn.cursor()
ids = c.execute('SELECT id FROM CUSTOMERS').fetchall()
if pin in ids:
query= f"SELECT AMOUNT FROM CUSTOMERS WHERE ID=?"
c.execute(query,(pin))
print(c.fetchone())
else:
print("no")
The problem is that I'm getting a Value Error as such: ValueError: parameters are of unsupported type
, I know the solution might be something very simple and basic but the thing is I'm completely new to this sqlite3 thing and so not good with it. Explanation will be of great help for me to learn.
sqlite3 python-3.7
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have created a database table which is basically a simple atm database which has records of some people with their pin
as the primary key. Now I need to create another file where a user can enter their pin
and then it checks if the pin
exists in the database and if it does it shows how much money he has and on entering the amount to withdraw, the database gets updated with a new value of amount
in that person's record. The table I created is as such:
import sqlite3
conn = sqlite3.connect('pin.db')
print("opened successfully")
conn.execute('''CREATE TABLE CUSTOMERS
(ID INT PRIMARY KEY NOT NULL,
NAME TEXT NOT NULL,
AGE INT NOT NULL,
ADDRESS CHAR(50),
AMOUNT INT);''')
print("table created")
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (1234, 'Paul', 32, 'California', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (2345, 'Allen', 25, 'Texas', 15000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (3456, 'Teddy', 23, 'Norway', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (4567, 'Mark', 25, 'Rich-Mond ', 65000.00 )");
conn.commit()
print ("Records created successfully")
conn.close()
The other file will be something like this I guess:
import sqlite3
conn = sqlite3.connect('pin.db')
print ("Opened database successfully")
pin=int(input("enter the 4 digit pin: "))
conn.row_factory = lambda cursor, row: row[0]
c = conn.cursor()
ids = c.execute('SELECT id FROM CUSTOMERS').fetchall()
if pin in ids:
query= f"SELECT AMOUNT FROM CUSTOMERS WHERE ID=?"
c.execute(query,(pin))
print(c.fetchone())
else:
print("no")
The problem is that I'm getting a Value Error as such: ValueError: parameters are of unsupported type
, I know the solution might be something very simple and basic but the thing is I'm completely new to this sqlite3 thing and so not good with it. Explanation will be of great help for me to learn.
sqlite3 python-3.7
I have created a database table which is basically a simple atm database which has records of some people with their pin
as the primary key. Now I need to create another file where a user can enter their pin
and then it checks if the pin
exists in the database and if it does it shows how much money he has and on entering the amount to withdraw, the database gets updated with a new value of amount
in that person's record. The table I created is as such:
import sqlite3
conn = sqlite3.connect('pin.db')
print("opened successfully")
conn.execute('''CREATE TABLE CUSTOMERS
(ID INT PRIMARY KEY NOT NULL,
NAME TEXT NOT NULL,
AGE INT NOT NULL,
ADDRESS CHAR(50),
AMOUNT INT);''')
print("table created")
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (1234, 'Paul', 32, 'California', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (2345, 'Allen', 25, 'Texas', 15000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (3456, 'Teddy', 23, 'Norway', 20000.00 )");
conn.execute("INSERT INTO CUSTOMERS (ID,NAME,AGE,ADDRESS,AMOUNT)
VALUES (4567, 'Mark', 25, 'Rich-Mond ', 65000.00 )");
conn.commit()
print ("Records created successfully")
conn.close()
The other file will be something like this I guess:
import sqlite3
conn = sqlite3.connect('pin.db')
print ("Opened database successfully")
pin=int(input("enter the 4 digit pin: "))
conn.row_factory = lambda cursor, row: row[0]
c = conn.cursor()
ids = c.execute('SELECT id FROM CUSTOMERS').fetchall()
if pin in ids:
query= f"SELECT AMOUNT FROM CUSTOMERS WHERE ID=?"
c.execute(query,(pin))
print(c.fetchone())
else:
print("no")
The problem is that I'm getting a Value Error as such: ValueError: parameters are of unsupported type
, I know the solution might be something very simple and basic but the thing is I'm completely new to this sqlite3 thing and so not good with it. Explanation will be of great help for me to learn.
sqlite3 python-3.7
sqlite3 python-3.7
edited Nov 10 at 15:03
asked Nov 8 at 17:39


H4rd_C0d3
54
54
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53213326%2faccessing-specific-values-in-a-table-in-sqlite3-of-python-3-and-then-updating-it%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pzP8d8iEuq9gh Ub,lYGwKfD