Store image somewhere except database or file manager where i can get to access it globally
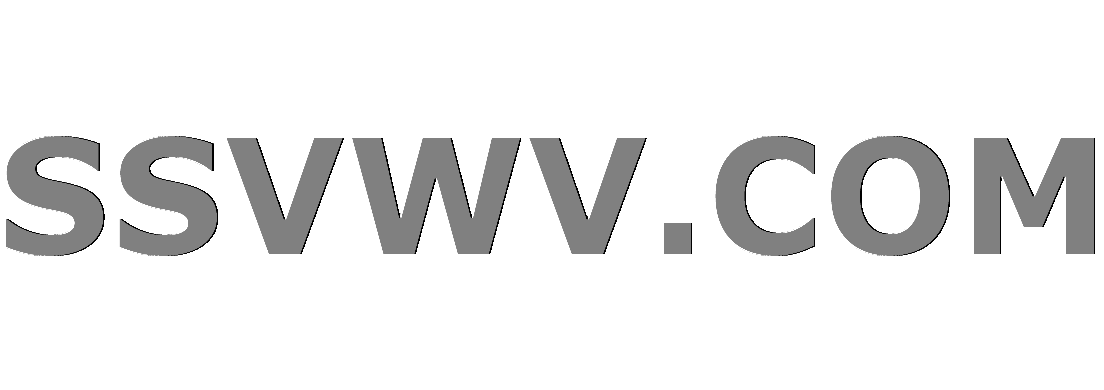
Multi tool use
up vote
0
down vote
favorite
I have a Multi Step form with 6 pages. Each page has 2-3 inputs. There are next and previous buttons on each page. Whenever user fills data in one of the page and submits, a php file is called through ajax call. Validation for all the steps are done in that same file. If all the inputs have correct data then next page is shown. All the steps are defined by their step no.
On the second last step there is a file input that takes an image. Sends it through ajax and stores in files. I have to store it in files somewhere to be able to show to the user.
Now on last step all the data has to be shown for last verification. Everything the user has entered is shown to him. Where he chooses to dismiss all the data or store it in the profile.
What if he does nothing and closes the tab. The Session variables will get destroyed but the image file he uploaded will be stuck in the files forever.
So, my question is how can i store that image in such a way so that whatever activity user does except (to dismiss or to accept), the image gets removed from the files.
A wild guess : can i store it in the sessions? Or cookies?
** Here Is The Code For Upload Verification **
/* Page 5 */
if($page == $page5)
if(isset($_FILES['uploadedVisitingCard']))
$image = new image;
$outImage;
try
if(!list($width, $height, $type) = getimagesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Image Must Be Below 5MB";
echo json_encode($result);
die();
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
$result['error'] = true;
$result['msg'] = "Width = ".$image->width." nHeight = ".$image->height." nImage Type = ".$image->type;
echo json_encode($result);
die();
else
$result['error'] = true;
$result['msg'] = "The Image Was NOt Uploaded";
echo json_encode($result);
die();
** Here Is The Function That Fetches Image Details, Resize Them And Renders Out Image In The File **
function resize_image($file, image $image, $dstWidth, $dstHeight)
$dst; $newwidth; $newheight;
$r = $image->width / $image->height;
if ($dstWidth/$dstHeight > $r) $newwidth = $dstHeight*$r; $newheight = $dstHeight;
else $newheight = $dstWidth/$r; $newwidth = $dstWidth;
switch($image->type)
case IMAGETYPE_JPEG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
return $dst;
function process_image($file)
global $allowedImageType;
$image = new Image;
try
if(!list($width, $height, $type) = getimagesize($file))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($file))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Images Must Be Below 5MB";
echo json_encode($result);
die();
return $image;
function render_image($image, $path)
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
php forms multi-step
|
show 2 more comments
up vote
0
down vote
favorite
I have a Multi Step form with 6 pages. Each page has 2-3 inputs. There are next and previous buttons on each page. Whenever user fills data in one of the page and submits, a php file is called through ajax call. Validation for all the steps are done in that same file. If all the inputs have correct data then next page is shown. All the steps are defined by their step no.
On the second last step there is a file input that takes an image. Sends it through ajax and stores in files. I have to store it in files somewhere to be able to show to the user.
Now on last step all the data has to be shown for last verification. Everything the user has entered is shown to him. Where he chooses to dismiss all the data or store it in the profile.
What if he does nothing and closes the tab. The Session variables will get destroyed but the image file he uploaded will be stuck in the files forever.
So, my question is how can i store that image in such a way so that whatever activity user does except (to dismiss or to accept), the image gets removed from the files.
A wild guess : can i store it in the sessions? Or cookies?
** Here Is The Code For Upload Verification **
/* Page 5 */
if($page == $page5)
if(isset($_FILES['uploadedVisitingCard']))
$image = new image;
$outImage;
try
if(!list($width, $height, $type) = getimagesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Image Must Be Below 5MB";
echo json_encode($result);
die();
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
$result['error'] = true;
$result['msg'] = "Width = ".$image->width." nHeight = ".$image->height." nImage Type = ".$image->type;
echo json_encode($result);
die();
else
$result['error'] = true;
$result['msg'] = "The Image Was NOt Uploaded";
echo json_encode($result);
die();
** Here Is The Function That Fetches Image Details, Resize Them And Renders Out Image In The File **
function resize_image($file, image $image, $dstWidth, $dstHeight)
$dst; $newwidth; $newheight;
$r = $image->width / $image->height;
if ($dstWidth/$dstHeight > $r) $newwidth = $dstHeight*$r; $newheight = $dstHeight;
else $newheight = $dstWidth/$r; $newwidth = $dstWidth;
switch($image->type)
case IMAGETYPE_JPEG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
return $dst;
function process_image($file)
global $allowedImageType;
$image = new Image;
try
if(!list($width, $height, $type) = getimagesize($file))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($file))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Images Must Be Below 5MB";
echo json_encode($result);
die();
return $image;
function render_image($image, $path)
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
php forms multi-step
2
You can upload the image to a temp folder, then when the user confirms - move it to wanted directory.
– HtmHell
2 days ago
@HtmHell. it has to be displayed to the user in the last step. So i have to move it somewhere else.
– Div's ladva
2 days ago
Show me your upload code & your "last step" code as well. I will post an answer so you'll understand better.
– HtmHell
2 days ago
Well, "temp folder" can mean a specific folder which is accessible publicly but is automatically cleaned out every so often.
– deceze♦
2 days ago
@HtmHell. I updated The Question.
– Div's ladva
2 days ago
|
show 2 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a Multi Step form with 6 pages. Each page has 2-3 inputs. There are next and previous buttons on each page. Whenever user fills data in one of the page and submits, a php file is called through ajax call. Validation for all the steps are done in that same file. If all the inputs have correct data then next page is shown. All the steps are defined by their step no.
On the second last step there is a file input that takes an image. Sends it through ajax and stores in files. I have to store it in files somewhere to be able to show to the user.
Now on last step all the data has to be shown for last verification. Everything the user has entered is shown to him. Where he chooses to dismiss all the data or store it in the profile.
What if he does nothing and closes the tab. The Session variables will get destroyed but the image file he uploaded will be stuck in the files forever.
So, my question is how can i store that image in such a way so that whatever activity user does except (to dismiss or to accept), the image gets removed from the files.
A wild guess : can i store it in the sessions? Or cookies?
** Here Is The Code For Upload Verification **
/* Page 5 */
if($page == $page5)
if(isset($_FILES['uploadedVisitingCard']))
$image = new image;
$outImage;
try
if(!list($width, $height, $type) = getimagesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Image Must Be Below 5MB";
echo json_encode($result);
die();
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
$result['error'] = true;
$result['msg'] = "Width = ".$image->width." nHeight = ".$image->height." nImage Type = ".$image->type;
echo json_encode($result);
die();
else
$result['error'] = true;
$result['msg'] = "The Image Was NOt Uploaded";
echo json_encode($result);
die();
** Here Is The Function That Fetches Image Details, Resize Them And Renders Out Image In The File **
function resize_image($file, image $image, $dstWidth, $dstHeight)
$dst; $newwidth; $newheight;
$r = $image->width / $image->height;
if ($dstWidth/$dstHeight > $r) $newwidth = $dstHeight*$r; $newheight = $dstHeight;
else $newheight = $dstWidth/$r; $newwidth = $dstWidth;
switch($image->type)
case IMAGETYPE_JPEG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
return $dst;
function process_image($file)
global $allowedImageType;
$image = new Image;
try
if(!list($width, $height, $type) = getimagesize($file))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($file))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Images Must Be Below 5MB";
echo json_encode($result);
die();
return $image;
function render_image($image, $path)
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
php forms multi-step
I have a Multi Step form with 6 pages. Each page has 2-3 inputs. There are next and previous buttons on each page. Whenever user fills data in one of the page and submits, a php file is called through ajax call. Validation for all the steps are done in that same file. If all the inputs have correct data then next page is shown. All the steps are defined by their step no.
On the second last step there is a file input that takes an image. Sends it through ajax and stores in files. I have to store it in files somewhere to be able to show to the user.
Now on last step all the data has to be shown for last verification. Everything the user has entered is shown to him. Where he chooses to dismiss all the data or store it in the profile.
What if he does nothing and closes the tab. The Session variables will get destroyed but the image file he uploaded will be stuck in the files forever.
So, my question is how can i store that image in such a way so that whatever activity user does except (to dismiss or to accept), the image gets removed from the files.
A wild guess : can i store it in the sessions? Or cookies?
** Here Is The Code For Upload Verification **
/* Page 5 */
if($page == $page5)
if(isset($_FILES['uploadedVisitingCard']))
$image = new image;
$outImage;
try
if(!list($width, $height, $type) = getimagesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Image Must Be Below 5MB";
echo json_encode($result);
die();
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
$result['error'] = true;
$result['msg'] = "Width = ".$image->width." nHeight = ".$image->height." nImage Type = ".$image->type;
echo json_encode($result);
die();
else
$result['error'] = true;
$result['msg'] = "The Image Was NOt Uploaded";
echo json_encode($result);
die();
** Here Is The Function That Fetches Image Details, Resize Them And Renders Out Image In The File **
function resize_image($file, image $image, $dstWidth, $dstHeight)
$dst; $newwidth; $newheight;
$r = $image->width / $image->height;
if ($dstWidth/$dstHeight > $r) $newwidth = $dstHeight*$r; $newheight = $dstHeight;
else $newheight = $dstWidth/$r; $newwidth = $dstWidth;
switch($image->type)
case IMAGETYPE_JPEG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPJPEGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
try
if(!$dst = imagecreatetruecolor($newwidth, $newheight))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC1");
if(!imagecopyresampled($dst, $file, 0, 0, 0, 0, $newwidth, $newheight, $image->width, $image->height))
throw new Exception("Problem In Duplication Of The File! Error Code: IMGDUPPNGFUNC2");
catch(Execption $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
break;
return $dst;
function process_image($file)
global $allowedImageType;
$image = new Image;
try
if(!list($width, $height, $type) = getimagesize($file))
throw new Exception("Either Uploaded File Is Not An Image or It An Image Type That Is Not Supported");
$image->type = $type;
$image->width = $width;
$image->height = $height;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if(!in_array($image->type, $allowedImageType))
$result['error'] = true;
$result['msg'] = "File Type Not Supported For Uploading! The File Must Be a PNG File or a JPG File";
echo json_encode($result);
die();
try
if(!$image->size = filesize($file))
throw new Exception("Unable To Get The Size OF The Uploaded Image.");
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
if($image->size > 5242880)
$result['error'] = true;
$result['msg'] = "The Images Must Be Below 5MB";
echo json_encode($result);
die();
return $image;
function render_image($image, $path)
try
switch ($image->type)
case IMAGETYPE_JPEG:
if(!$outImage = imagecreatefromjpeg($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEG");
if(!$outImage = resize_image($outImage, $image, 640, 480))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPJPEGFUNC");
if(!imagejpeg($outImage, "main.jpg", 70))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVJPEG");
$result['error'] = true;
$result['msg'] = "Image Is JPEG";
echo json_encode($result);
die();
break;
case IMAGETYPE_PNG:
if(!$outImage = imagecreatefrompng($_FILES['uploadedVisitingCard']['tmp_name']))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNG");
if(!$outImage = resize_image($outImage, $image, 800, 600))
throw new Exception("Cannot Reproduce The JPEG Image! Error Code: IMGDUPPNGFUNC");
if(!imagepng($outImage, "main.png", 9))
throw new Exception("Cannot Save The File Into The Servers! Error Code: IMGSAVPNG");
$result['error'] = true;
$result['msg'] = "Image Is PNG";
echo json_encode($result);
die();
break;
catch(Exception $e)
$result['error'] = true;
$result['msg'] = $e->getMessage();
echo json_encode($result);
die();
php forms multi-step
php forms multi-step
edited 2 days ago
asked 2 days ago
Div's ladva
87
87
2
You can upload the image to a temp folder, then when the user confirms - move it to wanted directory.
– HtmHell
2 days ago
@HtmHell. it has to be displayed to the user in the last step. So i have to move it somewhere else.
– Div's ladva
2 days ago
Show me your upload code & your "last step" code as well. I will post an answer so you'll understand better.
– HtmHell
2 days ago
Well, "temp folder" can mean a specific folder which is accessible publicly but is automatically cleaned out every so often.
– deceze♦
2 days ago
@HtmHell. I updated The Question.
– Div's ladva
2 days ago
|
show 2 more comments
2
You can upload the image to a temp folder, then when the user confirms - move it to wanted directory.
– HtmHell
2 days ago
@HtmHell. it has to be displayed to the user in the last step. So i have to move it somewhere else.
– Div's ladva
2 days ago
Show me your upload code & your "last step" code as well. I will post an answer so you'll understand better.
– HtmHell
2 days ago
Well, "temp folder" can mean a specific folder which is accessible publicly but is automatically cleaned out every so often.
– deceze♦
2 days ago
@HtmHell. I updated The Question.
– Div's ladva
2 days ago
2
2
You can upload the image to a temp folder, then when the user confirms - move it to wanted directory.
– HtmHell
2 days ago
You can upload the image to a temp folder, then when the user confirms - move it to wanted directory.
– HtmHell
2 days ago
@HtmHell. it has to be displayed to the user in the last step. So i have to move it somewhere else.
– Div's ladva
2 days ago
@HtmHell. it has to be displayed to the user in the last step. So i have to move it somewhere else.
– Div's ladva
2 days ago
Show me your upload code & your "last step" code as well. I will post an answer so you'll understand better.
– HtmHell
2 days ago
Show me your upload code & your "last step" code as well. I will post an answer so you'll understand better.
– HtmHell
2 days ago
Well, "temp folder" can mean a specific folder which is accessible publicly but is automatically cleaned out every so often.
– deceze♦
2 days ago
Well, "temp folder" can mean a specific folder which is accessible publicly but is automatically cleaned out every so often.
– deceze♦
2 days ago
@HtmHell. I updated The Question.
– Div's ladva
2 days ago
@HtmHell. I updated The Question.
– Div's ladva
2 days ago
|
show 2 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
The easiest way is to create an actual file management system. Meaning, you have a database table somewhere in which you store information about the files. The actual files should be stored in a folder structure somewhere on disk, with randomly generated names. UUIDs are very useful for this purpose. In the database then you store that UUID/file path, and any additional information about the file you want; e.g. its original user-supplied name, when it was uploaded, by whom it was uploaded etc.
Having this information allows you to act on it. E.g., you can query for all files which have been uploaded more than 24 hours ago, but have not been used anywhere else after that, so which can be deleted. Or perhaps you set a temporary = true
flag when you upload them, and at the end of your wizard you remove the temporary
flag, and you delete all temporary
files after 24 hours. This can all be done with a simple, regular cron job.
In other words: you don't treat the files any differently from any other uploaded files, you merely retain enough information about them somewhere that allows you to remove them later as necessary.
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The easiest way is to create an actual file management system. Meaning, you have a database table somewhere in which you store information about the files. The actual files should be stored in a folder structure somewhere on disk, with randomly generated names. UUIDs are very useful for this purpose. In the database then you store that UUID/file path, and any additional information about the file you want; e.g. its original user-supplied name, when it was uploaded, by whom it was uploaded etc.
Having this information allows you to act on it. E.g., you can query for all files which have been uploaded more than 24 hours ago, but have not been used anywhere else after that, so which can be deleted. Or perhaps you set a temporary = true
flag when you upload them, and at the end of your wizard you remove the temporary
flag, and you delete all temporary
files after 24 hours. This can all be done with a simple, regular cron job.
In other words: you don't treat the files any differently from any other uploaded files, you merely retain enough information about them somewhere that allows you to remove them later as necessary.
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
add a comment |
up vote
0
down vote
The easiest way is to create an actual file management system. Meaning, you have a database table somewhere in which you store information about the files. The actual files should be stored in a folder structure somewhere on disk, with randomly generated names. UUIDs are very useful for this purpose. In the database then you store that UUID/file path, and any additional information about the file you want; e.g. its original user-supplied name, when it was uploaded, by whom it was uploaded etc.
Having this information allows you to act on it. E.g., you can query for all files which have been uploaded more than 24 hours ago, but have not been used anywhere else after that, so which can be deleted. Or perhaps you set a temporary = true
flag when you upload them, and at the end of your wizard you remove the temporary
flag, and you delete all temporary
files after 24 hours. This can all be done with a simple, regular cron job.
In other words: you don't treat the files any differently from any other uploaded files, you merely retain enough information about them somewhere that allows you to remove them later as necessary.
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
add a comment |
up vote
0
down vote
up vote
0
down vote
The easiest way is to create an actual file management system. Meaning, you have a database table somewhere in which you store information about the files. The actual files should be stored in a folder structure somewhere on disk, with randomly generated names. UUIDs are very useful for this purpose. In the database then you store that UUID/file path, and any additional information about the file you want; e.g. its original user-supplied name, when it was uploaded, by whom it was uploaded etc.
Having this information allows you to act on it. E.g., you can query for all files which have been uploaded more than 24 hours ago, but have not been used anywhere else after that, so which can be deleted. Or perhaps you set a temporary = true
flag when you upload them, and at the end of your wizard you remove the temporary
flag, and you delete all temporary
files after 24 hours. This can all be done with a simple, regular cron job.
In other words: you don't treat the files any differently from any other uploaded files, you merely retain enough information about them somewhere that allows you to remove them later as necessary.
The easiest way is to create an actual file management system. Meaning, you have a database table somewhere in which you store information about the files. The actual files should be stored in a folder structure somewhere on disk, with randomly generated names. UUIDs are very useful for this purpose. In the database then you store that UUID/file path, and any additional information about the file you want; e.g. its original user-supplied name, when it was uploaded, by whom it was uploaded etc.
Having this information allows you to act on it. E.g., you can query for all files which have been uploaded more than 24 hours ago, but have not been used anywhere else after that, so which can be deleted. Or perhaps you set a temporary = true
flag when you upload them, and at the end of your wizard you remove the temporary
flag, and you delete all temporary
files after 24 hours. This can all be done with a simple, regular cron job.
In other words: you don't treat the files any differently from any other uploaded files, you merely retain enough information about them somewhere that allows you to remove them later as necessary.
answered 2 days ago
deceze♦
387k60523675
387k60523675
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
add a comment |
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
Can you give an example. I can't grasp what you're trying to say. Sorry ☺
– Div's ladva
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53237904%2fstore-image-somewhere-except-database-or-file-manager-where-i-can-get-to-access%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
hLXzfGrO9t0m544kvbbsqp,7OvhEf,b,RUPNN9sXrXsaTMzQmB
2
You can upload the image to a temp folder, then when the user confirms - move it to wanted directory.
– HtmHell
2 days ago
@HtmHell. it has to be displayed to the user in the last step. So i have to move it somewhere else.
– Div's ladva
2 days ago
Show me your upload code & your "last step" code as well. I will post an answer so you'll understand better.
– HtmHell
2 days ago
Well, "temp folder" can mean a specific folder which is accessible publicly but is automatically cleaned out every so often.
– deceze♦
2 days ago
@HtmHell. I updated The Question.
– Div's ladva
2 days ago