How to save form data into 2 data tables?
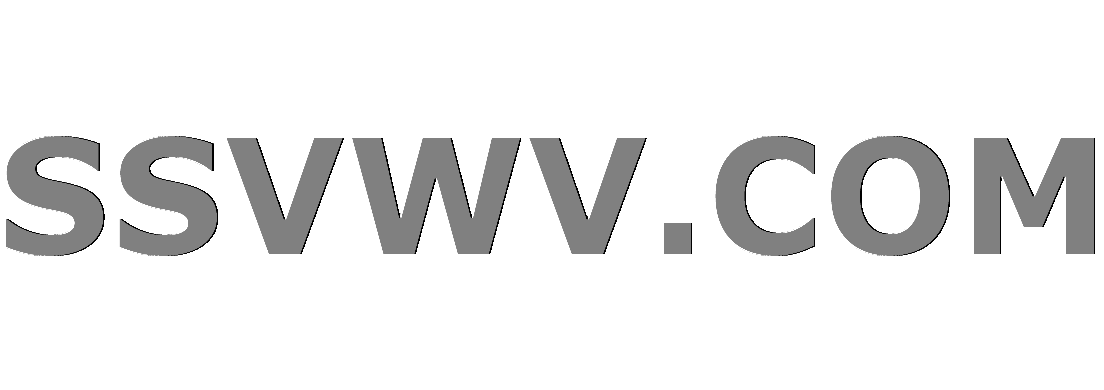
Multi tool use
up vote
0
down vote
favorite
I am looking to save data from a form into 2 different tables.
I have this in stripe account controller create:
def create
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
acct = Stripe::Account.create(
:country => "US",
:type => "custom",
legal_entity:
first_name: stripe_account_params[:first_name].capitalize,
last_name: stripe_account_params[:last_name].capitalize,
type: stripe_account_params[:account_type],
dob:
day: stripe_account_params[:dob_day],
month: stripe_account_params[:dob_month],
year: stripe_account_params[:dob_year]
,
address:
line1: stripe_account_params[:address_line1],
city: stripe_account_params[:address_city],
state: stripe_account_params[:address_state],
postal_code: stripe_account_params[:address_postal]
,
ssn_last_4: stripe_account_params[:ssn_last_4]
,
tos_acceptance:
date: Time.now.to_i,
ip: request.remote_ip
)
@stripe_account.acct_id = acct.id
#issue is here at @user
@user.stripe_token = acct.id
if @stripe_account.save!
format.html redirect_to new_bank_account_path, notice: 'Stripe account was successfully created.'
format.json render :show, status: :created, location: @stripe_account
else
format.html render :new
format.json render json: @stripe_account.errors, status: :unprocessable_entity
end
end
end
The form:
<%= form_for ([@user, @stripe_account]) do | f | %>
User Model:
has_one :stripe_account
Stripe_account Model:
belongs_to :users
the acct.id gets saved into the stripe_account table but not the user table. The user table has a user.stripe_account record.
How can i save the acct.id in both tables?
ruby-on-rails ruby
add a comment |
up vote
0
down vote
favorite
I am looking to save data from a form into 2 different tables.
I have this in stripe account controller create:
def create
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
acct = Stripe::Account.create(
:country => "US",
:type => "custom",
legal_entity:
first_name: stripe_account_params[:first_name].capitalize,
last_name: stripe_account_params[:last_name].capitalize,
type: stripe_account_params[:account_type],
dob:
day: stripe_account_params[:dob_day],
month: stripe_account_params[:dob_month],
year: stripe_account_params[:dob_year]
,
address:
line1: stripe_account_params[:address_line1],
city: stripe_account_params[:address_city],
state: stripe_account_params[:address_state],
postal_code: stripe_account_params[:address_postal]
,
ssn_last_4: stripe_account_params[:ssn_last_4]
,
tos_acceptance:
date: Time.now.to_i,
ip: request.remote_ip
)
@stripe_account.acct_id = acct.id
#issue is here at @user
@user.stripe_token = acct.id
if @stripe_account.save!
format.html redirect_to new_bank_account_path, notice: 'Stripe account was successfully created.'
format.json render :show, status: :created, location: @stripe_account
else
format.html render :new
format.json render json: @stripe_account.errors, status: :unprocessable_entity
end
end
end
The form:
<%= form_for ([@user, @stripe_account]) do | f | %>
User Model:
has_one :stripe_account
Stripe_account Model:
belongs_to :users
the acct.id gets saved into the stripe_account table but not the user table. The user table has a user.stripe_account record.
How can i save the acct.id in both tables?
ruby-on-rails ruby
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am looking to save data from a form into 2 different tables.
I have this in stripe account controller create:
def create
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
acct = Stripe::Account.create(
:country => "US",
:type => "custom",
legal_entity:
first_name: stripe_account_params[:first_name].capitalize,
last_name: stripe_account_params[:last_name].capitalize,
type: stripe_account_params[:account_type],
dob:
day: stripe_account_params[:dob_day],
month: stripe_account_params[:dob_month],
year: stripe_account_params[:dob_year]
,
address:
line1: stripe_account_params[:address_line1],
city: stripe_account_params[:address_city],
state: stripe_account_params[:address_state],
postal_code: stripe_account_params[:address_postal]
,
ssn_last_4: stripe_account_params[:ssn_last_4]
,
tos_acceptance:
date: Time.now.to_i,
ip: request.remote_ip
)
@stripe_account.acct_id = acct.id
#issue is here at @user
@user.stripe_token = acct.id
if @stripe_account.save!
format.html redirect_to new_bank_account_path, notice: 'Stripe account was successfully created.'
format.json render :show, status: :created, location: @stripe_account
else
format.html render :new
format.json render json: @stripe_account.errors, status: :unprocessable_entity
end
end
end
The form:
<%= form_for ([@user, @stripe_account]) do | f | %>
User Model:
has_one :stripe_account
Stripe_account Model:
belongs_to :users
the acct.id gets saved into the stripe_account table but not the user table. The user table has a user.stripe_account record.
How can i save the acct.id in both tables?
ruby-on-rails ruby
I am looking to save data from a form into 2 different tables.
I have this in stripe account controller create:
def create
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
acct = Stripe::Account.create(
:country => "US",
:type => "custom",
legal_entity:
first_name: stripe_account_params[:first_name].capitalize,
last_name: stripe_account_params[:last_name].capitalize,
type: stripe_account_params[:account_type],
dob:
day: stripe_account_params[:dob_day],
month: stripe_account_params[:dob_month],
year: stripe_account_params[:dob_year]
,
address:
line1: stripe_account_params[:address_line1],
city: stripe_account_params[:address_city],
state: stripe_account_params[:address_state],
postal_code: stripe_account_params[:address_postal]
,
ssn_last_4: stripe_account_params[:ssn_last_4]
,
tos_acceptance:
date: Time.now.to_i,
ip: request.remote_ip
)
@stripe_account.acct_id = acct.id
#issue is here at @user
@user.stripe_token = acct.id
if @stripe_account.save!
format.html redirect_to new_bank_account_path, notice: 'Stripe account was successfully created.'
format.json render :show, status: :created, location: @stripe_account
else
format.html render :new
format.json render json: @stripe_account.errors, status: :unprocessable_entity
end
end
end
The form:
<%= form_for ([@user, @stripe_account]) do | f | %>
User Model:
has_one :stripe_account
Stripe_account Model:
belongs_to :users
the acct.id gets saved into the stripe_account table but not the user table. The user table has a user.stripe_account record.
How can i save the acct.id in both tables?
ruby-on-rails ruby
ruby-on-rails ruby
edited Nov 11 at 1:48
asked Nov 10 at 23:31
uno
508
508
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
I'm guessing you are only doing @stripe_account.save
and not doing @user.save
(why did you cut your method?).
You can add a @user.save
or set the belongs_to assocaition with the autosave: true
option.
I'd recommend you to change this:
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
to this, though:
@user = User.find(params[:user_id])
@stripe_account = @user.build_stripe_account(stripe_account_params)
I don't think the autosave will works if you only set the id and not the actual object.
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
I don't understand your last question, if you set the relationship as autosave, when you save the@stripe_account
object it will also save the@user
associated object. If you set@user.stripe_token
before saving the stripe_account, it will also update the users table.
– arieljuod
Nov 11 at 1:44
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
|
show 3 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
I'm guessing you are only doing @stripe_account.save
and not doing @user.save
(why did you cut your method?).
You can add a @user.save
or set the belongs_to assocaition with the autosave: true
option.
I'd recommend you to change this:
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
to this, though:
@user = User.find(params[:user_id])
@stripe_account = @user.build_stripe_account(stripe_account_params)
I don't think the autosave will works if you only set the id and not the actual object.
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
I don't understand your last question, if you set the relationship as autosave, when you save the@stripe_account
object it will also save the@user
associated object. If you set@user.stripe_token
before saving the stripe_account, it will also update the users table.
– arieljuod
Nov 11 at 1:44
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
|
show 3 more comments
up vote
1
down vote
accepted
I'm guessing you are only doing @stripe_account.save
and not doing @user.save
(why did you cut your method?).
You can add a @user.save
or set the belongs_to assocaition with the autosave: true
option.
I'd recommend you to change this:
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
to this, though:
@user = User.find(params[:user_id])
@stripe_account = @user.build_stripe_account(stripe_account_params)
I don't think the autosave will works if you only set the id and not the actual object.
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
I don't understand your last question, if you set the relationship as autosave, when you save the@stripe_account
object it will also save the@user
associated object. If you set@user.stripe_token
before saving the stripe_account, it will also update the users table.
– arieljuod
Nov 11 at 1:44
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
|
show 3 more comments
up vote
1
down vote
accepted
up vote
1
down vote
accepted
I'm guessing you are only doing @stripe_account.save
and not doing @user.save
(why did you cut your method?).
You can add a @user.save
or set the belongs_to assocaition with the autosave: true
option.
I'd recommend you to change this:
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
to this, though:
@user = User.find(params[:user_id])
@stripe_account = @user.build_stripe_account(stripe_account_params)
I don't think the autosave will works if you only set the id and not the actual object.
I'm guessing you are only doing @stripe_account.save
and not doing @user.save
(why did you cut your method?).
You can add a @user.save
or set the belongs_to assocaition with the autosave: true
option.
I'd recommend you to change this:
@stripe_account = StripeAccount.new(stripe_account_params)
@user = User.find(params[:user_id])
@stripe_account.user_id = current_user.id
to this, though:
@user = User.find(params[:user_id])
@stripe_account = @user.build_stripe_account(stripe_account_params)
I don't think the autosave will works if you only set the id and not the actual object.
answered Nov 11 at 1:24


arieljuod
5,89211121
5,89211121
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
I don't understand your last question, if you set the relationship as autosave, when you save the@stripe_account
object it will also save the@user
associated object. If you set@user.stripe_token
before saving the stripe_account, it will also update the users table.
– arieljuod
Nov 11 at 1:44
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
|
show 3 more comments
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
I don't understand your last question, if you set the relationship as autosave, when you save the@stripe_account
object it will also save the@user
associated object. If you set@user.stripe_token
before saving the stripe_account, it will also update the users table.
– arieljuod
Nov 11 at 1:44
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
Well stripe_account and user are separate tables. The user table will be first. So once the user creates the stripe_account, i want the stripe_account (the account token which is acct.id) to be also in the User table under stripe_account..... im also not sure by cut my method, what do you mean?
– uno
Nov 11 at 1:40
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
and yes, i only do "if @stripe_account.save!" .. but if i do it that way, doesn't nothing get saved into the StripeAccount table? I only want the acct.id in the User table from the stripe_account form/create
– uno
Nov 11 at 1:41
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
With "why did you cut your method?" I meant that, why are you not showing the complete method? I had to guess you only save the stripe_account object.
– arieljuod
Nov 11 at 1:43
I don't understand your last question, if you set the relationship as autosave, when you save the
@stripe_account
object it will also save the @user
associated object. If you set @user.stripe_token
before saving the stripe_account, it will also update the users table.– arieljuod
Nov 11 at 1:44
I don't understand your last question, if you set the relationship as autosave, when you save the
@stripe_account
object it will also save the @user
associated object. If you set @user.stripe_token
before saving the stripe_account, it will also update the users table.– arieljuod
Nov 11 at 1:44
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
ok so to clarify, it will save in both places? Because im worried without "@stripe_account = StripeAccount.new(stripe_account_params)"... will it save in both?
– uno
Nov 11 at 1:46
|
show 3 more comments
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244457%2fhow-to-save-form-data-into-2-data-tables%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
4XVHJ dMZPSfE2wRUYDWaZ YlvOXJ3Mx2ETDI9yG19fw4zWr9UKK2YMdnsJuS,V07,JqC2jNyAnV,0i4eAH,ls dp4Y8XGxwrTHj7zt