Should I use WTForms for buttons when adding items to a cart?
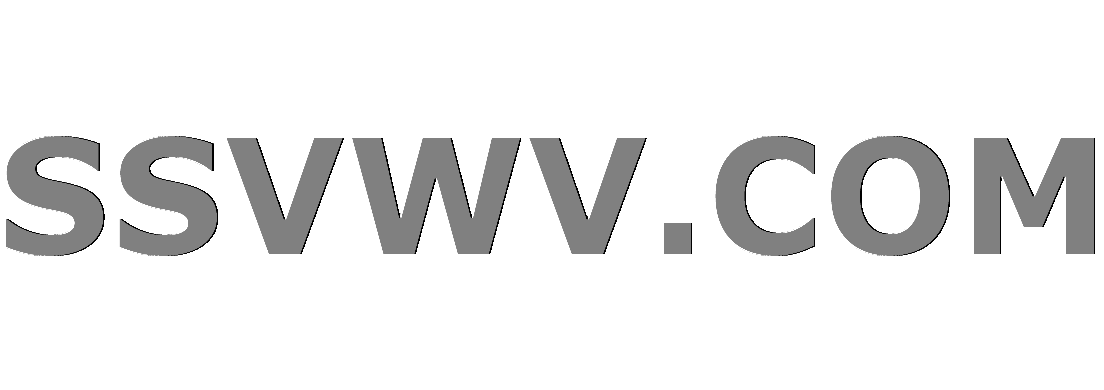
Multi tool use
So I am doing a 'rental application' where I have 3 classes in my Flask Application.
Below are the classes I implemented:
User
(as the top most level class)
Cart
(every user has a cart)
Rental
(A user / cart can have '0 to many' rentals)
cart_with_items = db.Table('cart_with_items',
db.Column('cart_id', db.Integer, db.ForeignKey('cart.id'), primary_key=True),
db.Column('rental_id', db.Integer, db.ForeignKey('rental.id'), primary_key=True)
)
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(15), unique=True)
email = db.Column(db.String(50), unique=True)
password = db.Column(db.String(80))
cart = db.relationship("Cart", backref='user', lazy=True, uselist=False)
class Cart(db.Model):
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False)
rentals = db.relationship('Rental', secondary=cart_with_items, lazy='subquery', backref=backref('carts', lazy=True))
@app.route("/dashboard/checkout", methods=['POST'])
def add_item(self,item):
try:
this.rentals.append(Rental(1,"2",3.5,"url"))
return '<h1> it worked</h1>'
except:
return '<h1>Didnt work</h1>'
class Rental(db.Model):
id = db.Column(db.Integer, primary_key=True)
item_name = db.Column(db.String(15), unique=True);
item_cost = db.Column(db.Float)
item_image_url = db.Column(db.String(100))
#foreign key is a primary key that refers to a key in another table
def __init__(self,item_id,item_name, item_cost, item_image_url):
self.id = item_id
self.item_name = item_name
self.item_cost = item_cost
self.item_image_url = item_image_url
def get_item_id():
return self.id
def getItemname():
return self.item_name
def getCost():
return self.item_cost
Then I render_template on a 'dashboard' page, and I'm passing in the user object which will by then have a cart created in composition with it.
I am also passing in a few rental objects into a template where they will be listed to show what you can rent, along with a button next to them.
The button is what I am having trouble with. I tried implementing a function in the Cart class to add an item to rentals, but it is proving to be difficult...
I don't know if I am able to do onClick()
in the jinja2 templates but I'm wondering should I just use WTForms and try to post the items they want back to the function that rendered given template?
Primarily, my question is asking if WTForms is the most accepted, and should WTForms be the solution to my problem ? Am I being too messy with my implementation?
python flask flask-sqlalchemy flask-wtforms
add a comment |
So I am doing a 'rental application' where I have 3 classes in my Flask Application.
Below are the classes I implemented:
User
(as the top most level class)
Cart
(every user has a cart)
Rental
(A user / cart can have '0 to many' rentals)
cart_with_items = db.Table('cart_with_items',
db.Column('cart_id', db.Integer, db.ForeignKey('cart.id'), primary_key=True),
db.Column('rental_id', db.Integer, db.ForeignKey('rental.id'), primary_key=True)
)
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(15), unique=True)
email = db.Column(db.String(50), unique=True)
password = db.Column(db.String(80))
cart = db.relationship("Cart", backref='user', lazy=True, uselist=False)
class Cart(db.Model):
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False)
rentals = db.relationship('Rental', secondary=cart_with_items, lazy='subquery', backref=backref('carts', lazy=True))
@app.route("/dashboard/checkout", methods=['POST'])
def add_item(self,item):
try:
this.rentals.append(Rental(1,"2",3.5,"url"))
return '<h1> it worked</h1>'
except:
return '<h1>Didnt work</h1>'
class Rental(db.Model):
id = db.Column(db.Integer, primary_key=True)
item_name = db.Column(db.String(15), unique=True);
item_cost = db.Column(db.Float)
item_image_url = db.Column(db.String(100))
#foreign key is a primary key that refers to a key in another table
def __init__(self,item_id,item_name, item_cost, item_image_url):
self.id = item_id
self.item_name = item_name
self.item_cost = item_cost
self.item_image_url = item_image_url
def get_item_id():
return self.id
def getItemname():
return self.item_name
def getCost():
return self.item_cost
Then I render_template on a 'dashboard' page, and I'm passing in the user object which will by then have a cart created in composition with it.
I am also passing in a few rental objects into a template where they will be listed to show what you can rent, along with a button next to them.
The button is what I am having trouble with. I tried implementing a function in the Cart class to add an item to rentals, but it is proving to be difficult...
I don't know if I am able to do onClick()
in the jinja2 templates but I'm wondering should I just use WTForms and try to post the items they want back to the function that rendered given template?
Primarily, my question is asking if WTForms is the most accepted, and should WTForms be the solution to my problem ? Am I being too messy with my implementation?
python flask flask-sqlalchemy flask-wtforms
add a comment |
So I am doing a 'rental application' where I have 3 classes in my Flask Application.
Below are the classes I implemented:
User
(as the top most level class)
Cart
(every user has a cart)
Rental
(A user / cart can have '0 to many' rentals)
cart_with_items = db.Table('cart_with_items',
db.Column('cart_id', db.Integer, db.ForeignKey('cart.id'), primary_key=True),
db.Column('rental_id', db.Integer, db.ForeignKey('rental.id'), primary_key=True)
)
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(15), unique=True)
email = db.Column(db.String(50), unique=True)
password = db.Column(db.String(80))
cart = db.relationship("Cart", backref='user', lazy=True, uselist=False)
class Cart(db.Model):
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False)
rentals = db.relationship('Rental', secondary=cart_with_items, lazy='subquery', backref=backref('carts', lazy=True))
@app.route("/dashboard/checkout", methods=['POST'])
def add_item(self,item):
try:
this.rentals.append(Rental(1,"2",3.5,"url"))
return '<h1> it worked</h1>'
except:
return '<h1>Didnt work</h1>'
class Rental(db.Model):
id = db.Column(db.Integer, primary_key=True)
item_name = db.Column(db.String(15), unique=True);
item_cost = db.Column(db.Float)
item_image_url = db.Column(db.String(100))
#foreign key is a primary key that refers to a key in another table
def __init__(self,item_id,item_name, item_cost, item_image_url):
self.id = item_id
self.item_name = item_name
self.item_cost = item_cost
self.item_image_url = item_image_url
def get_item_id():
return self.id
def getItemname():
return self.item_name
def getCost():
return self.item_cost
Then I render_template on a 'dashboard' page, and I'm passing in the user object which will by then have a cart created in composition with it.
I am also passing in a few rental objects into a template where they will be listed to show what you can rent, along with a button next to them.
The button is what I am having trouble with. I tried implementing a function in the Cart class to add an item to rentals, but it is proving to be difficult...
I don't know if I am able to do onClick()
in the jinja2 templates but I'm wondering should I just use WTForms and try to post the items they want back to the function that rendered given template?
Primarily, my question is asking if WTForms is the most accepted, and should WTForms be the solution to my problem ? Am I being too messy with my implementation?
python flask flask-sqlalchemy flask-wtforms
So I am doing a 'rental application' where I have 3 classes in my Flask Application.
Below are the classes I implemented:
User
(as the top most level class)
Cart
(every user has a cart)
Rental
(A user / cart can have '0 to many' rentals)
cart_with_items = db.Table('cart_with_items',
db.Column('cart_id', db.Integer, db.ForeignKey('cart.id'), primary_key=True),
db.Column('rental_id', db.Integer, db.ForeignKey('rental.id'), primary_key=True)
)
class User(UserMixin, db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(15), unique=True)
email = db.Column(db.String(50), unique=True)
password = db.Column(db.String(80))
cart = db.relationship("Cart", backref='user', lazy=True, uselist=False)
class Cart(db.Model):
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False)
rentals = db.relationship('Rental', secondary=cart_with_items, lazy='subquery', backref=backref('carts', lazy=True))
@app.route("/dashboard/checkout", methods=['POST'])
def add_item(self,item):
try:
this.rentals.append(Rental(1,"2",3.5,"url"))
return '<h1> it worked</h1>'
except:
return '<h1>Didnt work</h1>'
class Rental(db.Model):
id = db.Column(db.Integer, primary_key=True)
item_name = db.Column(db.String(15), unique=True);
item_cost = db.Column(db.Float)
item_image_url = db.Column(db.String(100))
#foreign key is a primary key that refers to a key in another table
def __init__(self,item_id,item_name, item_cost, item_image_url):
self.id = item_id
self.item_name = item_name
self.item_cost = item_cost
self.item_image_url = item_image_url
def get_item_id():
return self.id
def getItemname():
return self.item_name
def getCost():
return self.item_cost
Then I render_template on a 'dashboard' page, and I'm passing in the user object which will by then have a cart created in composition with it.
I am also passing in a few rental objects into a template where they will be listed to show what you can rent, along with a button next to them.
The button is what I am having trouble with. I tried implementing a function in the Cart class to add an item to rentals, but it is proving to be difficult...
I don't know if I am able to do onClick()
in the jinja2 templates but I'm wondering should I just use WTForms and try to post the items they want back to the function that rendered given template?
Primarily, my question is asking if WTForms is the most accepted, and should WTForms be the solution to my problem ? Am I being too messy with my implementation?
python flask flask-sqlalchemy flask-wtforms
python flask flask-sqlalchemy flask-wtforms
edited Nov 15 '18 at 2:26
Jessi
373719
373719
asked Nov 14 '18 at 2:44
RobRenRobRen
266
266
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
To answer your primary question, whether you should use WTForms depends on whether you need to gather any user input, besides simply clicking on the button. WTForms really shines in extracting form data from the request body, then coersing the data to the types you need and running validators on them. If you don't have any form fields, it probably isn't going to help much.
To your question on Javascript in Jinja2 templates - yes, you can put <script>
s in the template. You can put any text in the template, and it will be rendered exactly as-is (unless, of course, its a Jinja statement). So, you could embed your JS directly in the template, or put it in a .js file and link it with a <script src=>
tag.
And finally, unless you left something out, your /dashboard/checkout
route handler will crash at runtime with a message about how it expects 2 arguments, but none are provided. The reason for this is 1, free functions (those not in a class) do not have a self
parameter, and 2, there is no variable in your route. To get what I think you want, you need to do this:
@app.route("/dashboard/checkout/<item>", methods=['POST'])
def add_item(item):
The route handler will then pass whatever is in <item>
as the item
parameter to add_item
.
Assuming that you are iterating over Rentals as rental, a very handy way to generate this URL in the template is:
url_for(add_item, item=rental.id)
You can then use this URL to either make a JavaScript AJAX call (if you don't want to leave the page), or you can use it as the action
in a form with a submit button:
<form method="POST" action=" url_for(add_item, item=rental.id) ">
<input type="Submit" value="Rent This!">
</form>
This will allow you to make a POST to your endpoint (which will also navigate away from the page). But since this form has no fields and no data, you really don't need WTForms to process it.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292450%2fshould-i-use-wtforms-for-buttons-when-adding-items-to-a-cart%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To answer your primary question, whether you should use WTForms depends on whether you need to gather any user input, besides simply clicking on the button. WTForms really shines in extracting form data from the request body, then coersing the data to the types you need and running validators on them. If you don't have any form fields, it probably isn't going to help much.
To your question on Javascript in Jinja2 templates - yes, you can put <script>
s in the template. You can put any text in the template, and it will be rendered exactly as-is (unless, of course, its a Jinja statement). So, you could embed your JS directly in the template, or put it in a .js file and link it with a <script src=>
tag.
And finally, unless you left something out, your /dashboard/checkout
route handler will crash at runtime with a message about how it expects 2 arguments, but none are provided. The reason for this is 1, free functions (those not in a class) do not have a self
parameter, and 2, there is no variable in your route. To get what I think you want, you need to do this:
@app.route("/dashboard/checkout/<item>", methods=['POST'])
def add_item(item):
The route handler will then pass whatever is in <item>
as the item
parameter to add_item
.
Assuming that you are iterating over Rentals as rental, a very handy way to generate this URL in the template is:
url_for(add_item, item=rental.id)
You can then use this URL to either make a JavaScript AJAX call (if you don't want to leave the page), or you can use it as the action
in a form with a submit button:
<form method="POST" action=" url_for(add_item, item=rental.id) ">
<input type="Submit" value="Rent This!">
</form>
This will allow you to make a POST to your endpoint (which will also navigate away from the page). But since this form has no fields and no data, you really don't need WTForms to process it.
add a comment |
To answer your primary question, whether you should use WTForms depends on whether you need to gather any user input, besides simply clicking on the button. WTForms really shines in extracting form data from the request body, then coersing the data to the types you need and running validators on them. If you don't have any form fields, it probably isn't going to help much.
To your question on Javascript in Jinja2 templates - yes, you can put <script>
s in the template. You can put any text in the template, and it will be rendered exactly as-is (unless, of course, its a Jinja statement). So, you could embed your JS directly in the template, or put it in a .js file and link it with a <script src=>
tag.
And finally, unless you left something out, your /dashboard/checkout
route handler will crash at runtime with a message about how it expects 2 arguments, but none are provided. The reason for this is 1, free functions (those not in a class) do not have a self
parameter, and 2, there is no variable in your route. To get what I think you want, you need to do this:
@app.route("/dashboard/checkout/<item>", methods=['POST'])
def add_item(item):
The route handler will then pass whatever is in <item>
as the item
parameter to add_item
.
Assuming that you are iterating over Rentals as rental, a very handy way to generate this URL in the template is:
url_for(add_item, item=rental.id)
You can then use this URL to either make a JavaScript AJAX call (if you don't want to leave the page), or you can use it as the action
in a form with a submit button:
<form method="POST" action=" url_for(add_item, item=rental.id) ">
<input type="Submit" value="Rent This!">
</form>
This will allow you to make a POST to your endpoint (which will also navigate away from the page). But since this form has no fields and no data, you really don't need WTForms to process it.
add a comment |
To answer your primary question, whether you should use WTForms depends on whether you need to gather any user input, besides simply clicking on the button. WTForms really shines in extracting form data from the request body, then coersing the data to the types you need and running validators on them. If you don't have any form fields, it probably isn't going to help much.
To your question on Javascript in Jinja2 templates - yes, you can put <script>
s in the template. You can put any text in the template, and it will be rendered exactly as-is (unless, of course, its a Jinja statement). So, you could embed your JS directly in the template, or put it in a .js file and link it with a <script src=>
tag.
And finally, unless you left something out, your /dashboard/checkout
route handler will crash at runtime with a message about how it expects 2 arguments, but none are provided. The reason for this is 1, free functions (those not in a class) do not have a self
parameter, and 2, there is no variable in your route. To get what I think you want, you need to do this:
@app.route("/dashboard/checkout/<item>", methods=['POST'])
def add_item(item):
The route handler will then pass whatever is in <item>
as the item
parameter to add_item
.
Assuming that you are iterating over Rentals as rental, a very handy way to generate this URL in the template is:
url_for(add_item, item=rental.id)
You can then use this URL to either make a JavaScript AJAX call (if you don't want to leave the page), or you can use it as the action
in a form with a submit button:
<form method="POST" action=" url_for(add_item, item=rental.id) ">
<input type="Submit" value="Rent This!">
</form>
This will allow you to make a POST to your endpoint (which will also navigate away from the page). But since this form has no fields and no data, you really don't need WTForms to process it.
To answer your primary question, whether you should use WTForms depends on whether you need to gather any user input, besides simply clicking on the button. WTForms really shines in extracting form data from the request body, then coersing the data to the types you need and running validators on them. If you don't have any form fields, it probably isn't going to help much.
To your question on Javascript in Jinja2 templates - yes, you can put <script>
s in the template. You can put any text in the template, and it will be rendered exactly as-is (unless, of course, its a Jinja statement). So, you could embed your JS directly in the template, or put it in a .js file and link it with a <script src=>
tag.
And finally, unless you left something out, your /dashboard/checkout
route handler will crash at runtime with a message about how it expects 2 arguments, but none are provided. The reason for this is 1, free functions (those not in a class) do not have a self
parameter, and 2, there is no variable in your route. To get what I think you want, you need to do this:
@app.route("/dashboard/checkout/<item>", methods=['POST'])
def add_item(item):
The route handler will then pass whatever is in <item>
as the item
parameter to add_item
.
Assuming that you are iterating over Rentals as rental, a very handy way to generate this URL in the template is:
url_for(add_item, item=rental.id)
You can then use this URL to either make a JavaScript AJAX call (if you don't want to leave the page), or you can use it as the action
in a form with a submit button:
<form method="POST" action=" url_for(add_item, item=rental.id) ">
<input type="Submit" value="Rent This!">
</form>
This will allow you to make a POST to your endpoint (which will also navigate away from the page). But since this form has no fields and no data, you really don't need WTForms to process it.
answered Nov 15 '18 at 3:51
GniemGniem
914
914
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292450%2fshould-i-use-wtforms-for-buttons-when-adding-items-to-a-cart%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GdbL7AAs KA ZejtIFp59Uo7zNRaRa8AVD