How can I detect when form submission is complete, for a form that does not load a page, but rather, serves up a file?
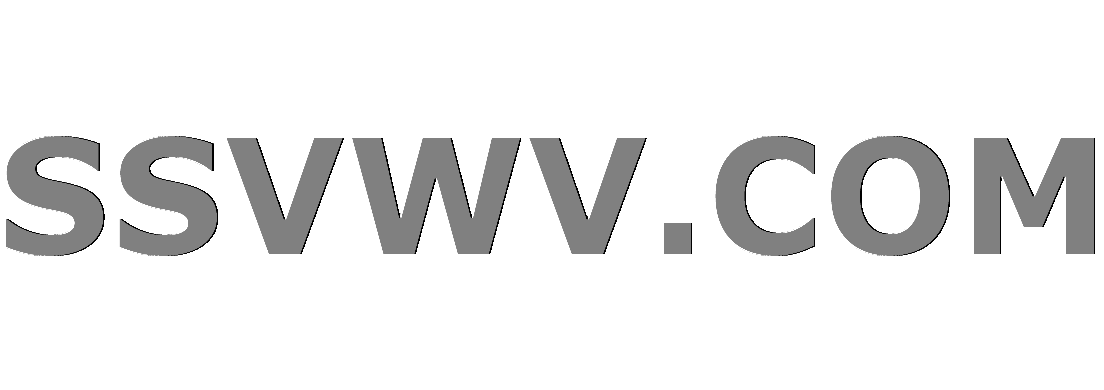
Multi tool use
When I have a form that I know will take a while to submit, I like to show a loading indicator on the submit event, so it shows up, and then gets cleared when the new page loads, something simple like this
$('#theForm').submit(function ()
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
);
The issue I'm having is I am trying to do this for a form that does not navigate away from the page when you submit it, it is just serving up a file. I am rendering a csv in php, and serving it up. It takes anywhere from a few seconds
to a minute to do it. When this happens, you never leave the page, so nothing can trigger the loader to go away. I thought I could submit to an iframe, and then kill the loader with the iframe's onLoad event:
<form action="/makeCSV" method="POST" target="iframe">
<iframe id="iframe" name="iframe"></iframe>
$("#iframe").load(function ()
$("#loadingGIF").remove();
);
But I guess since it is just serving up a file and not loading a page, the onload event does not trigger.
If I have a form that is serving up a file, rather than navigating to a new page, is there any way to detect that the file has been served up?
If it helps, csv rendering code is below:
<?php
//do some stuff to generate a csv...
$csv="heading1, heading2, heading3, heading4 rn";
$csv.="value1, value2, value3, value4 rn";
$csv.="a,b,c,d rn";
//...
header('Pragma: public');
header('Expires: 0');
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: private', false);
header('Content-Type: text/csv');
header("Content-Disposition: attachment;filename="file.csv"");
echo $csv;
exit;
?>
javascript php jquery iframe
add a comment |
When I have a form that I know will take a while to submit, I like to show a loading indicator on the submit event, so it shows up, and then gets cleared when the new page loads, something simple like this
$('#theForm').submit(function ()
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
);
The issue I'm having is I am trying to do this for a form that does not navigate away from the page when you submit it, it is just serving up a file. I am rendering a csv in php, and serving it up. It takes anywhere from a few seconds
to a minute to do it. When this happens, you never leave the page, so nothing can trigger the loader to go away. I thought I could submit to an iframe, and then kill the loader with the iframe's onLoad event:
<form action="/makeCSV" method="POST" target="iframe">
<iframe id="iframe" name="iframe"></iframe>
$("#iframe").load(function ()
$("#loadingGIF").remove();
);
But I guess since it is just serving up a file and not loading a page, the onload event does not trigger.
If I have a form that is serving up a file, rather than navigating to a new page, is there any way to detect that the file has been served up?
If it helps, csv rendering code is below:
<?php
//do some stuff to generate a csv...
$csv="heading1, heading2, heading3, heading4 rn";
$csv.="value1, value2, value3, value4 rn";
$csv.="a,b,c,d rn";
//...
header('Pragma: public');
header('Expires: 0');
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: private', false);
header('Content-Type: text/csv');
header("Content-Disposition: attachment;filename="file.csv"");
echo $csv;
exit;
?>
javascript php jquery iframe
1
See this old question I asked. The trick with the cookie works great.
– Pointy
Aug 7 '15 at 14:06
@Pointy The cookie trick looks great! Excellent idea. I had thought about polling for...something but I didn't know what. That's perfect.
– chiliNUT
Aug 7 '15 at 14:18
@chiliNUT I've been using it basically since Mr. Crowder provided that answer, and I've never had a problem with it. I usually have the JavaScript code make up a random number and post that with the form, and then the server-side code sends back the cookie with that random number as the value. That avoids potential problems with old cookies getting stuck. Because cookies come in the header of the response, it's usually the case that your "Loading ..." thing will go away before the browser's file handling dialog pops up (though that's not super important).
– Pointy
Aug 7 '15 at 14:29
@Pointy, working beautifully
– chiliNUT
Aug 7 '15 at 14:56
add a comment |
When I have a form that I know will take a while to submit, I like to show a loading indicator on the submit event, so it shows up, and then gets cleared when the new page loads, something simple like this
$('#theForm').submit(function ()
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
);
The issue I'm having is I am trying to do this for a form that does not navigate away from the page when you submit it, it is just serving up a file. I am rendering a csv in php, and serving it up. It takes anywhere from a few seconds
to a minute to do it. When this happens, you never leave the page, so nothing can trigger the loader to go away. I thought I could submit to an iframe, and then kill the loader with the iframe's onLoad event:
<form action="/makeCSV" method="POST" target="iframe">
<iframe id="iframe" name="iframe"></iframe>
$("#iframe").load(function ()
$("#loadingGIF").remove();
);
But I guess since it is just serving up a file and not loading a page, the onload event does not trigger.
If I have a form that is serving up a file, rather than navigating to a new page, is there any way to detect that the file has been served up?
If it helps, csv rendering code is below:
<?php
//do some stuff to generate a csv...
$csv="heading1, heading2, heading3, heading4 rn";
$csv.="value1, value2, value3, value4 rn";
$csv.="a,b,c,d rn";
//...
header('Pragma: public');
header('Expires: 0');
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: private', false);
header('Content-Type: text/csv');
header("Content-Disposition: attachment;filename="file.csv"");
echo $csv;
exit;
?>
javascript php jquery iframe
When I have a form that I know will take a while to submit, I like to show a loading indicator on the submit event, so it shows up, and then gets cleared when the new page loads, something simple like this
$('#theForm').submit(function ()
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
);
The issue I'm having is I am trying to do this for a form that does not navigate away from the page when you submit it, it is just serving up a file. I am rendering a csv in php, and serving it up. It takes anywhere from a few seconds
to a minute to do it. When this happens, you never leave the page, so nothing can trigger the loader to go away. I thought I could submit to an iframe, and then kill the loader with the iframe's onLoad event:
<form action="/makeCSV" method="POST" target="iframe">
<iframe id="iframe" name="iframe"></iframe>
$("#iframe").load(function ()
$("#loadingGIF").remove();
);
But I guess since it is just serving up a file and not loading a page, the onload event does not trigger.
If I have a form that is serving up a file, rather than navigating to a new page, is there any way to detect that the file has been served up?
If it helps, csv rendering code is below:
<?php
//do some stuff to generate a csv...
$csv="heading1, heading2, heading3, heading4 rn";
$csv.="value1, value2, value3, value4 rn";
$csv.="a,b,c,d rn";
//...
header('Pragma: public');
header('Expires: 0');
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: private', false);
header('Content-Type: text/csv');
header("Content-Disposition: attachment;filename="file.csv"");
echo $csv;
exit;
?>
javascript php jquery iframe
javascript php jquery iframe
edited Aug 7 '15 at 14:31
Jay Blanchard
35.8k125596
35.8k125596
asked Aug 7 '15 at 14:03


chiliNUTchiliNUT
12.7k94879
12.7k94879
1
See this old question I asked. The trick with the cookie works great.
– Pointy
Aug 7 '15 at 14:06
@Pointy The cookie trick looks great! Excellent idea. I had thought about polling for...something but I didn't know what. That's perfect.
– chiliNUT
Aug 7 '15 at 14:18
@chiliNUT I've been using it basically since Mr. Crowder provided that answer, and I've never had a problem with it. I usually have the JavaScript code make up a random number and post that with the form, and then the server-side code sends back the cookie with that random number as the value. That avoids potential problems with old cookies getting stuck. Because cookies come in the header of the response, it's usually the case that your "Loading ..." thing will go away before the browser's file handling dialog pops up (though that's not super important).
– Pointy
Aug 7 '15 at 14:29
@Pointy, working beautifully
– chiliNUT
Aug 7 '15 at 14:56
add a comment |
1
See this old question I asked. The trick with the cookie works great.
– Pointy
Aug 7 '15 at 14:06
@Pointy The cookie trick looks great! Excellent idea. I had thought about polling for...something but I didn't know what. That's perfect.
– chiliNUT
Aug 7 '15 at 14:18
@chiliNUT I've been using it basically since Mr. Crowder provided that answer, and I've never had a problem with it. I usually have the JavaScript code make up a random number and post that with the form, and then the server-side code sends back the cookie with that random number as the value. That avoids potential problems with old cookies getting stuck. Because cookies come in the header of the response, it's usually the case that your "Loading ..." thing will go away before the browser's file handling dialog pops up (though that's not super important).
– Pointy
Aug 7 '15 at 14:29
@Pointy, working beautifully
– chiliNUT
Aug 7 '15 at 14:56
1
1
See this old question I asked. The trick with the cookie works great.
– Pointy
Aug 7 '15 at 14:06
See this old question I asked. The trick with the cookie works great.
– Pointy
Aug 7 '15 at 14:06
@Pointy The cookie trick looks great! Excellent idea. I had thought about polling for...something but I didn't know what. That's perfect.
– chiliNUT
Aug 7 '15 at 14:18
@Pointy The cookie trick looks great! Excellent idea. I had thought about polling for...something but I didn't know what. That's perfect.
– chiliNUT
Aug 7 '15 at 14:18
@chiliNUT I've been using it basically since Mr. Crowder provided that answer, and I've never had a problem with it. I usually have the JavaScript code make up a random number and post that with the form, and then the server-side code sends back the cookie with that random number as the value. That avoids potential problems with old cookies getting stuck. Because cookies come in the header of the response, it's usually the case that your "Loading ..." thing will go away before the browser's file handling dialog pops up (though that's not super important).
– Pointy
Aug 7 '15 at 14:29
@chiliNUT I've been using it basically since Mr. Crowder provided that answer, and I've never had a problem with it. I usually have the JavaScript code make up a random number and post that with the form, and then the server-side code sends back the cookie with that random number as the value. That avoids potential problems with old cookies getting stuck. Because cookies come in the header of the response, it's usually the case that your "Loading ..." thing will go away before the browser's file handling dialog pops up (though that's not super important).
– Pointy
Aug 7 '15 at 14:29
@Pointy, working beautifully
– chiliNUT
Aug 7 '15 at 14:56
@Pointy, working beautifully
– chiliNUT
Aug 7 '15 at 14:56
add a comment |
3 Answers
3
active
oldest
votes
I would go with a 2 step process - submit the form via ajax, build the csv, save as a temp file and return an identifier. Then set the iframe to request the file, and in the handler foir that request, serve then delete the file:
$('#form').submit(function(ev)
ev.preventDefault();
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
$.post('createfile.php', $(this).serialize(), function(resp)
var identifier = resp.intentifier;
$('iframe').attr('src', 'servefile.php?i='+identifier);
$('#loaderContainer').html('<!-- -->');
//createfile.php
//create csv then
$identifier = uuid();
file_put_contents('somedir/'. $identifier .'.tmp', $csv);
echo json_encode(['indentifier'=>$identifier]);
//servefile.php
$identifier = $_GET['identifier'];
$file = 'somedir/' .$identifier .'.tmp'
//output correct headers here
readfile($file);
unlink($file);
add a comment |
Since you are already using JQuery, I suggest you use something that already has solved the issue in a compact/reliable way. I suggest giving jQuery File Download a try.
This answer led me to it. This may do exactly what you need and it is just a plugin that you can drop on your project and test :) Good luck.
add a comment |
You can check on window losing focus... pretty basic, but works for my need.
$([window, top.window]).blur(function()
$('BUTTON.btnLoading').removeClass('btnLoading');
);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f31879657%2fhow-can-i-detect-when-form-submission-is-complete-for-a-form-that-does-not-load%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I would go with a 2 step process - submit the form via ajax, build the csv, save as a temp file and return an identifier. Then set the iframe to request the file, and in the handler foir that request, serve then delete the file:
$('#form').submit(function(ev)
ev.preventDefault();
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
$.post('createfile.php', $(this).serialize(), function(resp)
var identifier = resp.intentifier;
$('iframe').attr('src', 'servefile.php?i='+identifier);
$('#loaderContainer').html('<!-- -->');
//createfile.php
//create csv then
$identifier = uuid();
file_put_contents('somedir/'. $identifier .'.tmp', $csv);
echo json_encode(['indentifier'=>$identifier]);
//servefile.php
$identifier = $_GET['identifier'];
$file = 'somedir/' .$identifier .'.tmp'
//output correct headers here
readfile($file);
unlink($file);
add a comment |
I would go with a 2 step process - submit the form via ajax, build the csv, save as a temp file and return an identifier. Then set the iframe to request the file, and in the handler foir that request, serve then delete the file:
$('#form').submit(function(ev)
ev.preventDefault();
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
$.post('createfile.php', $(this).serialize(), function(resp)
var identifier = resp.intentifier;
$('iframe').attr('src', 'servefile.php?i='+identifier);
$('#loaderContainer').html('<!-- -->');
//createfile.php
//create csv then
$identifier = uuid();
file_put_contents('somedir/'. $identifier .'.tmp', $csv);
echo json_encode(['indentifier'=>$identifier]);
//servefile.php
$identifier = $_GET['identifier'];
$file = 'somedir/' .$identifier .'.tmp'
//output correct headers here
readfile($file);
unlink($file);
add a comment |
I would go with a 2 step process - submit the form via ajax, build the csv, save as a temp file and return an identifier. Then set the iframe to request the file, and in the handler foir that request, serve then delete the file:
$('#form').submit(function(ev)
ev.preventDefault();
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
$.post('createfile.php', $(this).serialize(), function(resp)
var identifier = resp.intentifier;
$('iframe').attr('src', 'servefile.php?i='+identifier);
$('#loaderContainer').html('<!-- -->');
//createfile.php
//create csv then
$identifier = uuid();
file_put_contents('somedir/'. $identifier .'.tmp', $csv);
echo json_encode(['indentifier'=>$identifier]);
//servefile.php
$identifier = $_GET['identifier'];
$file = 'somedir/' .$identifier .'.tmp'
//output correct headers here
readfile($file);
unlink($file);
I would go with a 2 step process - submit the form via ajax, build the csv, save as a temp file and return an identifier. Then set the iframe to request the file, and in the handler foir that request, serve then delete the file:
$('#form').submit(function(ev)
ev.preventDefault();
$('#loaderContainer').html('<img src="loader.gif" id="loadingGIF" />');
$.post('createfile.php', $(this).serialize(), function(resp)
var identifier = resp.intentifier;
$('iframe').attr('src', 'servefile.php?i='+identifier);
$('#loaderContainer').html('<!-- -->');
//createfile.php
//create csv then
$identifier = uuid();
file_put_contents('somedir/'. $identifier .'.tmp', $csv);
echo json_encode(['indentifier'=>$identifier]);
//servefile.php
$identifier = $_GET['identifier'];
$file = 'somedir/' .$identifier .'.tmp'
//output correct headers here
readfile($file);
unlink($file);
answered Aug 7 '15 at 14:28
SteveSteve
17.4k42555
17.4k42555
add a comment |
add a comment |
Since you are already using JQuery, I suggest you use something that already has solved the issue in a compact/reliable way. I suggest giving jQuery File Download a try.
This answer led me to it. This may do exactly what you need and it is just a plugin that you can drop on your project and test :) Good luck.
add a comment |
Since you are already using JQuery, I suggest you use something that already has solved the issue in a compact/reliable way. I suggest giving jQuery File Download a try.
This answer led me to it. This may do exactly what you need and it is just a plugin that you can drop on your project and test :) Good luck.
add a comment |
Since you are already using JQuery, I suggest you use something that already has solved the issue in a compact/reliable way. I suggest giving jQuery File Download a try.
This answer led me to it. This may do exactly what you need and it is just a plugin that you can drop on your project and test :) Good luck.
Since you are already using JQuery, I suggest you use something that already has solved the issue in a compact/reliable way. I suggest giving jQuery File Download a try.
This answer led me to it. This may do exactly what you need and it is just a plugin that you can drop on your project and test :) Good luck.
edited May 23 '17 at 12:14
Community♦
11
11
answered Aug 7 '15 at 14:31
KatsukeKatsuke
540421
540421
add a comment |
add a comment |
You can check on window losing focus... pretty basic, but works for my need.
$([window, top.window]).blur(function()
$('BUTTON.btnLoading').removeClass('btnLoading');
);
add a comment |
You can check on window losing focus... pretty basic, but works for my need.
$([window, top.window]).blur(function()
$('BUTTON.btnLoading').removeClass('btnLoading');
);
add a comment |
You can check on window losing focus... pretty basic, but works for my need.
$([window, top.window]).blur(function()
$('BUTTON.btnLoading').removeClass('btnLoading');
);
You can check on window losing focus... pretty basic, but works for my need.
$([window, top.window]).blur(function()
$('BUTTON.btnLoading').removeClass('btnLoading');
);
answered Nov 15 '18 at 2:28


Martin ZvaríkMartin Zvarík
526513
526513
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f31879657%2fhow-can-i-detect-when-form-submission-is-complete-for-a-form-that-does-not-load%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Q7eXB,4O8ntww,k I8S9frGSBCA 4keGs,P,ONe7CL M6 gmJNNj,YTJ,n,UQOuFp,BRSps,smwf6zt8S2dgusWFPyX
1
See this old question I asked. The trick with the cookie works great.
– Pointy
Aug 7 '15 at 14:06
@Pointy The cookie trick looks great! Excellent idea. I had thought about polling for...something but I didn't know what. That's perfect.
– chiliNUT
Aug 7 '15 at 14:18
@chiliNUT I've been using it basically since Mr. Crowder provided that answer, and I've never had a problem with it. I usually have the JavaScript code make up a random number and post that with the form, and then the server-side code sends back the cookie with that random number as the value. That avoids potential problems with old cookies getting stuck. Because cookies come in the header of the response, it's usually the case that your "Loading ..." thing will go away before the browser's file handling dialog pops up (though that's not super important).
– Pointy
Aug 7 '15 at 14:29
@Pointy, working beautifully
– chiliNUT
Aug 7 '15 at 14:56