PYQT: mainwindow closes while having second and third window open
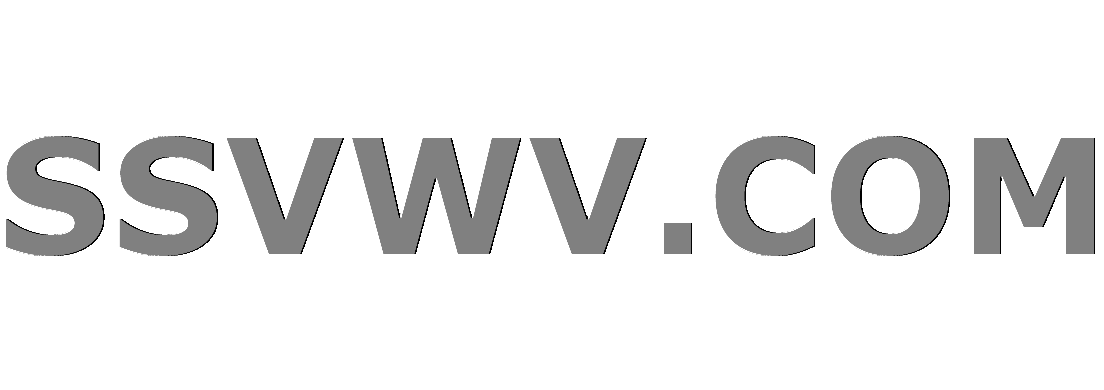
Multi tool use
I need some help in my current problem.
I have a window with three buttons. When pressing two of them ("GraphicButton" and "QuestionButton") a second and third window shall open. This works fine. The third button "ImportButton" is for populating a table widget in the mainwindow with a dataframe from excel.
When I press the "ImportButton" first everything works fine.
BUT when I press one of the other buttons first to open the other windows and THEN press the "ImportButton", the mainwindow and the other ones will close.
I could use some advice here.
Here is my code for main.py
:
import sys
import pandas as pd
import os
from qtpy import QtWidgets, QtCore, QtGui
from ui.mainwindow import Ui_MainWindow
from ui.graphwindow import Ui_GraphWindow
from ui.questionwindow import Ui_QuestionWindow
app = QtWidgets.QApplication(sys.argv)
class MainWindow(QtWidgets.QMainWindow):
def __init__(self, parent = None):
super().__init__(parent)
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
self.setWindowTitle("Test")
self.ui.ImportButton.clicked.connect(self.import_clicked)
self.ui.GraphicButton.clicked.connect(self.graphic_clicked)
self.ui.QuestionButton.clicked.connect(self.question_clicked)
def import_clicked(self):
self.ui.RefWidget.setSortingEnabled(False)
self.ui.LubWidget.setSortingEnabled(False)
self.ui.MixWidget.setSortingEnabled(False)
self.ui.RefWidget.setRowCount(0)
self.ui.LubWidget.setRowCount(0)
self.ui.MixWidget.setRowCount(0)
if self.ui.RefBoxC.currentIndex() == 0:
A = os.path.join(os.path.dirname(__file__),"./Datenbank/CO2.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
else:
A = os.path.join(os.path.dirname(__file__), "./Datenbank/PlatzhalterRef.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
self.ui.RefWidget.setSortingEnabled(True)
self.ui.LubWidget.setSortingEnabled(True)
self.ui.MixWidget.setSortingEnabled(True)
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.ui = Ui_GraphWindow()
self.ui.setupUi(self.Gwindow)
self.Gwindow.show()
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.ui = Ui_QuestionWindow()
self.ui.setupUi(self.Questwindow)
self.Questwindow.show()
window = MainWindow()
window.show()
sys.exit(app.exec_())
python-3.x pyqt pyqt5
add a comment |
I need some help in my current problem.
I have a window with three buttons. When pressing two of them ("GraphicButton" and "QuestionButton") a second and third window shall open. This works fine. The third button "ImportButton" is for populating a table widget in the mainwindow with a dataframe from excel.
When I press the "ImportButton" first everything works fine.
BUT when I press one of the other buttons first to open the other windows and THEN press the "ImportButton", the mainwindow and the other ones will close.
I could use some advice here.
Here is my code for main.py
:
import sys
import pandas as pd
import os
from qtpy import QtWidgets, QtCore, QtGui
from ui.mainwindow import Ui_MainWindow
from ui.graphwindow import Ui_GraphWindow
from ui.questionwindow import Ui_QuestionWindow
app = QtWidgets.QApplication(sys.argv)
class MainWindow(QtWidgets.QMainWindow):
def __init__(self, parent = None):
super().__init__(parent)
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
self.setWindowTitle("Test")
self.ui.ImportButton.clicked.connect(self.import_clicked)
self.ui.GraphicButton.clicked.connect(self.graphic_clicked)
self.ui.QuestionButton.clicked.connect(self.question_clicked)
def import_clicked(self):
self.ui.RefWidget.setSortingEnabled(False)
self.ui.LubWidget.setSortingEnabled(False)
self.ui.MixWidget.setSortingEnabled(False)
self.ui.RefWidget.setRowCount(0)
self.ui.LubWidget.setRowCount(0)
self.ui.MixWidget.setRowCount(0)
if self.ui.RefBoxC.currentIndex() == 0:
A = os.path.join(os.path.dirname(__file__),"./Datenbank/CO2.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
else:
A = os.path.join(os.path.dirname(__file__), "./Datenbank/PlatzhalterRef.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
self.ui.RefWidget.setSortingEnabled(True)
self.ui.LubWidget.setSortingEnabled(True)
self.ui.MixWidget.setSortingEnabled(True)
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.ui = Ui_GraphWindow()
self.ui.setupUi(self.Gwindow)
self.Gwindow.show()
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.ui = Ui_QuestionWindow()
self.ui.setupUi(self.Questwindow)
self.Questwindow.show()
window = MainWindow()
window.show()
sys.exit(app.exec_())
python-3.x pyqt pyqt5
add a comment |
I need some help in my current problem.
I have a window with three buttons. When pressing two of them ("GraphicButton" and "QuestionButton") a second and third window shall open. This works fine. The third button "ImportButton" is for populating a table widget in the mainwindow with a dataframe from excel.
When I press the "ImportButton" first everything works fine.
BUT when I press one of the other buttons first to open the other windows and THEN press the "ImportButton", the mainwindow and the other ones will close.
I could use some advice here.
Here is my code for main.py
:
import sys
import pandas as pd
import os
from qtpy import QtWidgets, QtCore, QtGui
from ui.mainwindow import Ui_MainWindow
from ui.graphwindow import Ui_GraphWindow
from ui.questionwindow import Ui_QuestionWindow
app = QtWidgets.QApplication(sys.argv)
class MainWindow(QtWidgets.QMainWindow):
def __init__(self, parent = None):
super().__init__(parent)
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
self.setWindowTitle("Test")
self.ui.ImportButton.clicked.connect(self.import_clicked)
self.ui.GraphicButton.clicked.connect(self.graphic_clicked)
self.ui.QuestionButton.clicked.connect(self.question_clicked)
def import_clicked(self):
self.ui.RefWidget.setSortingEnabled(False)
self.ui.LubWidget.setSortingEnabled(False)
self.ui.MixWidget.setSortingEnabled(False)
self.ui.RefWidget.setRowCount(0)
self.ui.LubWidget.setRowCount(0)
self.ui.MixWidget.setRowCount(0)
if self.ui.RefBoxC.currentIndex() == 0:
A = os.path.join(os.path.dirname(__file__),"./Datenbank/CO2.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
else:
A = os.path.join(os.path.dirname(__file__), "./Datenbank/PlatzhalterRef.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
self.ui.RefWidget.setSortingEnabled(True)
self.ui.LubWidget.setSortingEnabled(True)
self.ui.MixWidget.setSortingEnabled(True)
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.ui = Ui_GraphWindow()
self.ui.setupUi(self.Gwindow)
self.Gwindow.show()
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.ui = Ui_QuestionWindow()
self.ui.setupUi(self.Questwindow)
self.Questwindow.show()
window = MainWindow()
window.show()
sys.exit(app.exec_())
python-3.x pyqt pyqt5
I need some help in my current problem.
I have a window with three buttons. When pressing two of them ("GraphicButton" and "QuestionButton") a second and third window shall open. This works fine. The third button "ImportButton" is for populating a table widget in the mainwindow with a dataframe from excel.
When I press the "ImportButton" first everything works fine.
BUT when I press one of the other buttons first to open the other windows and THEN press the "ImportButton", the mainwindow and the other ones will close.
I could use some advice here.
Here is my code for main.py
:
import sys
import pandas as pd
import os
from qtpy import QtWidgets, QtCore, QtGui
from ui.mainwindow import Ui_MainWindow
from ui.graphwindow import Ui_GraphWindow
from ui.questionwindow import Ui_QuestionWindow
app = QtWidgets.QApplication(sys.argv)
class MainWindow(QtWidgets.QMainWindow):
def __init__(self, parent = None):
super().__init__(parent)
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
self.setWindowTitle("Test")
self.ui.ImportButton.clicked.connect(self.import_clicked)
self.ui.GraphicButton.clicked.connect(self.graphic_clicked)
self.ui.QuestionButton.clicked.connect(self.question_clicked)
def import_clicked(self):
self.ui.RefWidget.setSortingEnabled(False)
self.ui.LubWidget.setSortingEnabled(False)
self.ui.MixWidget.setSortingEnabled(False)
self.ui.RefWidget.setRowCount(0)
self.ui.LubWidget.setRowCount(0)
self.ui.MixWidget.setRowCount(0)
if self.ui.RefBoxC.currentIndex() == 0:
A = os.path.join(os.path.dirname(__file__),"./Datenbank/CO2.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
else:
A = os.path.join(os.path.dirname(__file__), "./Datenbank/PlatzhalterRef.xlsx")
df = pd.read_excel(A)
self.ui.RefWidget.setColumnCount(len(df.columns))
self.ui.RefWidget.setRowCount(len(df.index))
for i in range(len(df.index)):
for j in range(len(df.columns)):
self.ui.RefWidget.setItem(i, j, QtWidgets.QTableWidgetItem(str(df.iat[i, j])))
self.ui.RefWidget.resizeColumnsToContents()
self.ui.RefWidget.resizeRowsToContents()
self.ui.RefWidget.setSortingEnabled(True)
self.ui.LubWidget.setSortingEnabled(True)
self.ui.MixWidget.setSortingEnabled(True)
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.ui = Ui_GraphWindow()
self.ui.setupUi(self.Gwindow)
self.Gwindow.show()
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.ui = Ui_QuestionWindow()
self.ui.setupUi(self.Questwindow)
self.Questwindow.show()
window = MainWindow()
window.show()
sys.exit(app.exec_())
python-3.x pyqt pyqt5
python-3.x pyqt pyqt5
edited Nov 15 '18 at 13:58


TrebuchetMS
3,13511126
3,13511126
asked Nov 15 '18 at 12:04
Jack.O.Jack.O.
549
549
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Disclaimer: the solution provided by this answer has not been tested and may be incomplete.
When you click either the GraphicButton
or QuestionButton
,
self.ui = Ui_GraphWindow()
or
self.ui = Ui_QuestionWindow()
This will modify self.ui
. When the ImportButton
is clicked, self.ui
will refer to the other windows. This, I believe, is unintentional.
When the commands in import_clicked()
are run, there may be references to undefined classes, widgets, and whatnot.
self.ui.RefWidget.setSortingEnabled(False)
I'm guessing RefWidget is not defined in Ui_QuestionWindow or Ui_GraphWindow.
To solve this, try adding
def import_clicked(self):
self.ui = Ui_MainWindow() # set self.ui to refer to a MainWindow
# ...
and see if it works. (Warning: a new MainWindow may be created and the old MainWindow be left forsaken. Again, I haven't tested this to see if it does create a new MainWindow but my intuition tells me that it does.)
EDIT: Above suggestion in strikethrough did not work for OP.
If further trouble and chaos ensues, consider having three separate member variables instead of a single self.ui
.
def __init__(self, parent = None):
super().__init__(parent)
self.uiMain = Ui_MainWindow() # new member variable: self.uiMain
# ...
def import_clicked(self):
self.uiMain.RefWidget.setSortingEnabled(False)
self.uiMain.LubWidget.setSortingEnabled(False)
self.uiMain.MixWidget.setSortingEnabled(False)
# ...
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.uiGraphic = Ui_GraphWindow() # new member variable: self.uiGraphic
# ...
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.uiQuestion = Ui_QuestionWindow() # new member variable: self.uiQuestion
# ...
1
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53319130%2fpyqt-mainwindow-closes-while-having-second-and-third-window-open%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Disclaimer: the solution provided by this answer has not been tested and may be incomplete.
When you click either the GraphicButton
or QuestionButton
,
self.ui = Ui_GraphWindow()
or
self.ui = Ui_QuestionWindow()
This will modify self.ui
. When the ImportButton
is clicked, self.ui
will refer to the other windows. This, I believe, is unintentional.
When the commands in import_clicked()
are run, there may be references to undefined classes, widgets, and whatnot.
self.ui.RefWidget.setSortingEnabled(False)
I'm guessing RefWidget is not defined in Ui_QuestionWindow or Ui_GraphWindow.
To solve this, try adding
def import_clicked(self):
self.ui = Ui_MainWindow() # set self.ui to refer to a MainWindow
# ...
and see if it works. (Warning: a new MainWindow may be created and the old MainWindow be left forsaken. Again, I haven't tested this to see if it does create a new MainWindow but my intuition tells me that it does.)
EDIT: Above suggestion in strikethrough did not work for OP.
If further trouble and chaos ensues, consider having three separate member variables instead of a single self.ui
.
def __init__(self, parent = None):
super().__init__(parent)
self.uiMain = Ui_MainWindow() # new member variable: self.uiMain
# ...
def import_clicked(self):
self.uiMain.RefWidget.setSortingEnabled(False)
self.uiMain.LubWidget.setSortingEnabled(False)
self.uiMain.MixWidget.setSortingEnabled(False)
# ...
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.uiGraphic = Ui_GraphWindow() # new member variable: self.uiGraphic
# ...
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.uiQuestion = Ui_QuestionWindow() # new member variable: self.uiQuestion
# ...
1
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
add a comment |
Disclaimer: the solution provided by this answer has not been tested and may be incomplete.
When you click either the GraphicButton
or QuestionButton
,
self.ui = Ui_GraphWindow()
or
self.ui = Ui_QuestionWindow()
This will modify self.ui
. When the ImportButton
is clicked, self.ui
will refer to the other windows. This, I believe, is unintentional.
When the commands in import_clicked()
are run, there may be references to undefined classes, widgets, and whatnot.
self.ui.RefWidget.setSortingEnabled(False)
I'm guessing RefWidget is not defined in Ui_QuestionWindow or Ui_GraphWindow.
To solve this, try adding
def import_clicked(self):
self.ui = Ui_MainWindow() # set self.ui to refer to a MainWindow
# ...
and see if it works. (Warning: a new MainWindow may be created and the old MainWindow be left forsaken. Again, I haven't tested this to see if it does create a new MainWindow but my intuition tells me that it does.)
EDIT: Above suggestion in strikethrough did not work for OP.
If further trouble and chaos ensues, consider having three separate member variables instead of a single self.ui
.
def __init__(self, parent = None):
super().__init__(parent)
self.uiMain = Ui_MainWindow() # new member variable: self.uiMain
# ...
def import_clicked(self):
self.uiMain.RefWidget.setSortingEnabled(False)
self.uiMain.LubWidget.setSortingEnabled(False)
self.uiMain.MixWidget.setSortingEnabled(False)
# ...
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.uiGraphic = Ui_GraphWindow() # new member variable: self.uiGraphic
# ...
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.uiQuestion = Ui_QuestionWindow() # new member variable: self.uiQuestion
# ...
1
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
add a comment |
Disclaimer: the solution provided by this answer has not been tested and may be incomplete.
When you click either the GraphicButton
or QuestionButton
,
self.ui = Ui_GraphWindow()
or
self.ui = Ui_QuestionWindow()
This will modify self.ui
. When the ImportButton
is clicked, self.ui
will refer to the other windows. This, I believe, is unintentional.
When the commands in import_clicked()
are run, there may be references to undefined classes, widgets, and whatnot.
self.ui.RefWidget.setSortingEnabled(False)
I'm guessing RefWidget is not defined in Ui_QuestionWindow or Ui_GraphWindow.
To solve this, try adding
def import_clicked(self):
self.ui = Ui_MainWindow() # set self.ui to refer to a MainWindow
# ...
and see if it works. (Warning: a new MainWindow may be created and the old MainWindow be left forsaken. Again, I haven't tested this to see if it does create a new MainWindow but my intuition tells me that it does.)
EDIT: Above suggestion in strikethrough did not work for OP.
If further trouble and chaos ensues, consider having three separate member variables instead of a single self.ui
.
def __init__(self, parent = None):
super().__init__(parent)
self.uiMain = Ui_MainWindow() # new member variable: self.uiMain
# ...
def import_clicked(self):
self.uiMain.RefWidget.setSortingEnabled(False)
self.uiMain.LubWidget.setSortingEnabled(False)
self.uiMain.MixWidget.setSortingEnabled(False)
# ...
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.uiGraphic = Ui_GraphWindow() # new member variable: self.uiGraphic
# ...
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.uiQuestion = Ui_QuestionWindow() # new member variable: self.uiQuestion
# ...
Disclaimer: the solution provided by this answer has not been tested and may be incomplete.
When you click either the GraphicButton
or QuestionButton
,
self.ui = Ui_GraphWindow()
or
self.ui = Ui_QuestionWindow()
This will modify self.ui
. When the ImportButton
is clicked, self.ui
will refer to the other windows. This, I believe, is unintentional.
When the commands in import_clicked()
are run, there may be references to undefined classes, widgets, and whatnot.
self.ui.RefWidget.setSortingEnabled(False)
I'm guessing RefWidget is not defined in Ui_QuestionWindow or Ui_GraphWindow.
To solve this, try adding
def import_clicked(self):
self.ui = Ui_MainWindow() # set self.ui to refer to a MainWindow
# ...
and see if it works. (Warning: a new MainWindow may be created and the old MainWindow be left forsaken. Again, I haven't tested this to see if it does create a new MainWindow but my intuition tells me that it does.)
EDIT: Above suggestion in strikethrough did not work for OP.
If further trouble and chaos ensues, consider having three separate member variables instead of a single self.ui
.
def __init__(self, parent = None):
super().__init__(parent)
self.uiMain = Ui_MainWindow() # new member variable: self.uiMain
# ...
def import_clicked(self):
self.uiMain.RefWidget.setSortingEnabled(False)
self.uiMain.LubWidget.setSortingEnabled(False)
self.uiMain.MixWidget.setSortingEnabled(False)
# ...
def graphic_clicked(self):
self.Gwindow = QtWidgets.QMainWindow()
self.uiGraphic = Ui_GraphWindow() # new member variable: self.uiGraphic
# ...
def question_clicked(self):
self.Questwindow = QtWidgets.QMainWindow()
self.uiQuestion = Ui_QuestionWindow() # new member variable: self.uiQuestion
# ...
edited Nov 15 '18 at 14:24
answered Nov 15 '18 at 14:03


TrebuchetMSTrebuchetMS
3,13511126
3,13511126
1
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
add a comment |
1
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
1
1
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
Thanks! First advice did not work. The mainwindow closed no matter when i press the "ImportButton". Second advice works fine and makes sense. Thanks a lot!
– Jack.O.
Nov 15 '18 at 14:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53319130%2fpyqt-mainwindow-closes-while-having-second-and-third-window-open%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sw0hxa,z 5b6tC6vstTg 3DBs3McrUDc6cXJWL9jH7pFztdK M,L,B8P3LWD