How can I get a gradient at two points with Keras/Tensorflow
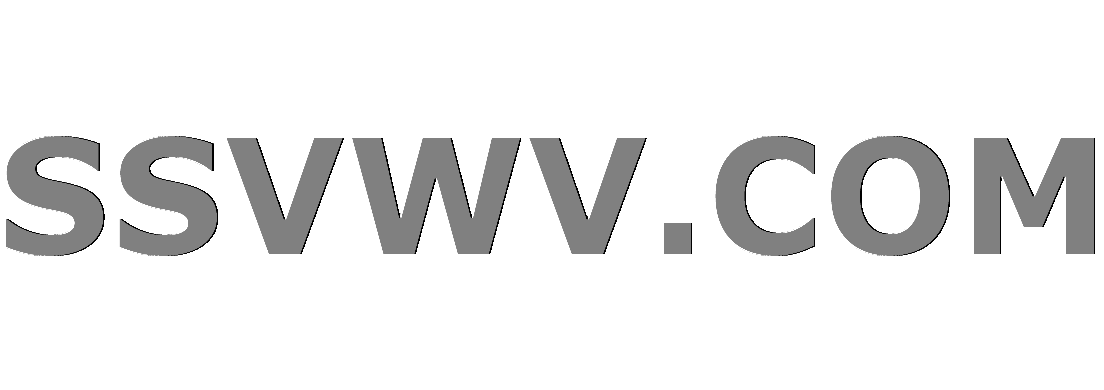
Multi tool use
I'm trying to get the gradient of a function at two different values of the independent variable. I am doing this in the context of modifying the get_updates
method of Keras' optimizer class.
The relevant section of the code I've written is
def get_updates(self, loss, params):
grads = self.get_gradients(loss, params)
self.updates = [K.update_add(self.iterations, 1)]
# Copy parameters
params2 =
for p in params:
params2.append(K.variable(K.get_value(p), name=p.name[:-2] + "_cpy1/"))
self.weights = [self.iterations]
for p, p2, g in zip(params, params2, grads):
v = - self.lr * g
new_p = p + 0.5*v # Intermediate/Partial step
new_p2 = p2 + v # Reference point for 2nd Gradient
grads2 = self.get_gradients(loss, params2)
....
The error I get when I execute this code is
File "/home/me/Projects/RungeKutta/rk_optimizers2.py", line 86, in get_updates
grads2 = self.get_gradients(loss, params2)
File "/home/me/anaconda2/lib/python2.7/site-packages/keras/optimizers.py", line 91, in get_gradients
raise ValueError('An operation has `None` for gradient. '
ValueError: An operation has `None` for gradient. Please make sure that all of your ops have a gradient defined (i.e. are differentiable). Common ops without gradient: K.argmax, K.round, K.eval.
The problematic section of code from Keras's optimizers.py is:
def get_gradients(self, loss, params):
grads = K.gradients(loss, params)
if None in grads:
raise ValueError('An operation has `None` for gradient. '
'Please make sure that all of your ops have a '
'gradient defined (i.e. are differentiable). '
'Common ops without gradient: '
'K.argmax, K.round, K.eval.')
In my case, K
corresponds to Tensorflow. So, the corresponding code for gradients
is:
def gradients(loss, variables):
"""Returns the gradients of `loss` w.r.t. `variables`.
# Arguments
loss: Scalar tensor to minimize.
variables: List of variables.
# Returns
A gradients tensor.
"""
return tf.gradients(loss, variables, colocate_gradients_with_ops=True)
Why do I get the error I see? How can I get a gradient at the first projected updated parameter so that I can get a final update using the average of the gradient at the starting point and the gradient at an (initially) projected end point?
python tensorflow keras
add a comment |
I'm trying to get the gradient of a function at two different values of the independent variable. I am doing this in the context of modifying the get_updates
method of Keras' optimizer class.
The relevant section of the code I've written is
def get_updates(self, loss, params):
grads = self.get_gradients(loss, params)
self.updates = [K.update_add(self.iterations, 1)]
# Copy parameters
params2 =
for p in params:
params2.append(K.variable(K.get_value(p), name=p.name[:-2] + "_cpy1/"))
self.weights = [self.iterations]
for p, p2, g in zip(params, params2, grads):
v = - self.lr * g
new_p = p + 0.5*v # Intermediate/Partial step
new_p2 = p2 + v # Reference point for 2nd Gradient
grads2 = self.get_gradients(loss, params2)
....
The error I get when I execute this code is
File "/home/me/Projects/RungeKutta/rk_optimizers2.py", line 86, in get_updates
grads2 = self.get_gradients(loss, params2)
File "/home/me/anaconda2/lib/python2.7/site-packages/keras/optimizers.py", line 91, in get_gradients
raise ValueError('An operation has `None` for gradient. '
ValueError: An operation has `None` for gradient. Please make sure that all of your ops have a gradient defined (i.e. are differentiable). Common ops without gradient: K.argmax, K.round, K.eval.
The problematic section of code from Keras's optimizers.py is:
def get_gradients(self, loss, params):
grads = K.gradients(loss, params)
if None in grads:
raise ValueError('An operation has `None` for gradient. '
'Please make sure that all of your ops have a '
'gradient defined (i.e. are differentiable). '
'Common ops without gradient: '
'K.argmax, K.round, K.eval.')
In my case, K
corresponds to Tensorflow. So, the corresponding code for gradients
is:
def gradients(loss, variables):
"""Returns the gradients of `loss` w.r.t. `variables`.
# Arguments
loss: Scalar tensor to minimize.
variables: List of variables.
# Returns
A gradients tensor.
"""
return tf.gradients(loss, variables, colocate_gradients_with_ops=True)
Why do I get the error I see? How can I get a gradient at the first projected updated parameter so that I can get a final update using the average of the gradient at the starting point and the gradient at an (initially) projected end point?
python tensorflow keras
add a comment |
I'm trying to get the gradient of a function at two different values of the independent variable. I am doing this in the context of modifying the get_updates
method of Keras' optimizer class.
The relevant section of the code I've written is
def get_updates(self, loss, params):
grads = self.get_gradients(loss, params)
self.updates = [K.update_add(self.iterations, 1)]
# Copy parameters
params2 =
for p in params:
params2.append(K.variable(K.get_value(p), name=p.name[:-2] + "_cpy1/"))
self.weights = [self.iterations]
for p, p2, g in zip(params, params2, grads):
v = - self.lr * g
new_p = p + 0.5*v # Intermediate/Partial step
new_p2 = p2 + v # Reference point for 2nd Gradient
grads2 = self.get_gradients(loss, params2)
....
The error I get when I execute this code is
File "/home/me/Projects/RungeKutta/rk_optimizers2.py", line 86, in get_updates
grads2 = self.get_gradients(loss, params2)
File "/home/me/anaconda2/lib/python2.7/site-packages/keras/optimizers.py", line 91, in get_gradients
raise ValueError('An operation has `None` for gradient. '
ValueError: An operation has `None` for gradient. Please make sure that all of your ops have a gradient defined (i.e. are differentiable). Common ops without gradient: K.argmax, K.round, K.eval.
The problematic section of code from Keras's optimizers.py is:
def get_gradients(self, loss, params):
grads = K.gradients(loss, params)
if None in grads:
raise ValueError('An operation has `None` for gradient. '
'Please make sure that all of your ops have a '
'gradient defined (i.e. are differentiable). '
'Common ops without gradient: '
'K.argmax, K.round, K.eval.')
In my case, K
corresponds to Tensorflow. So, the corresponding code for gradients
is:
def gradients(loss, variables):
"""Returns the gradients of `loss` w.r.t. `variables`.
# Arguments
loss: Scalar tensor to minimize.
variables: List of variables.
# Returns
A gradients tensor.
"""
return tf.gradients(loss, variables, colocate_gradients_with_ops=True)
Why do I get the error I see? How can I get a gradient at the first projected updated parameter so that I can get a final update using the average of the gradient at the starting point and the gradient at an (initially) projected end point?
python tensorflow keras
I'm trying to get the gradient of a function at two different values of the independent variable. I am doing this in the context of modifying the get_updates
method of Keras' optimizer class.
The relevant section of the code I've written is
def get_updates(self, loss, params):
grads = self.get_gradients(loss, params)
self.updates = [K.update_add(self.iterations, 1)]
# Copy parameters
params2 =
for p in params:
params2.append(K.variable(K.get_value(p), name=p.name[:-2] + "_cpy1/"))
self.weights = [self.iterations]
for p, p2, g in zip(params, params2, grads):
v = - self.lr * g
new_p = p + 0.5*v # Intermediate/Partial step
new_p2 = p2 + v # Reference point for 2nd Gradient
grads2 = self.get_gradients(loss, params2)
....
The error I get when I execute this code is
File "/home/me/Projects/RungeKutta/rk_optimizers2.py", line 86, in get_updates
grads2 = self.get_gradients(loss, params2)
File "/home/me/anaconda2/lib/python2.7/site-packages/keras/optimizers.py", line 91, in get_gradients
raise ValueError('An operation has `None` for gradient. '
ValueError: An operation has `None` for gradient. Please make sure that all of your ops have a gradient defined (i.e. are differentiable). Common ops without gradient: K.argmax, K.round, K.eval.
The problematic section of code from Keras's optimizers.py is:
def get_gradients(self, loss, params):
grads = K.gradients(loss, params)
if None in grads:
raise ValueError('An operation has `None` for gradient. '
'Please make sure that all of your ops have a '
'gradient defined (i.e. are differentiable). '
'Common ops without gradient: '
'K.argmax, K.round, K.eval.')
In my case, K
corresponds to Tensorflow. So, the corresponding code for gradients
is:
def gradients(loss, variables):
"""Returns the gradients of `loss` w.r.t. `variables`.
# Arguments
loss: Scalar tensor to minimize.
variables: List of variables.
# Returns
A gradients tensor.
"""
return tf.gradients(loss, variables, colocate_gradients_with_ops=True)
Why do I get the error I see? How can I get a gradient at the first projected updated parameter so that I can get a final update using the average of the gradient at the starting point and the gradient at an (initially) projected end point?
python tensorflow keras
python tensorflow keras
asked Nov 15 '18 at 6:35
user1245262user1245262
2,75432848
2,75432848
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53313713%2fhow-can-i-get-a-gradient-at-two-points-with-keras-tensorflow%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53313713%2fhow-can-i-get-a-gradient-at-two-points-with-keras-tensorflow%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kKUsgrVMqv wR059dRnhZOwOTT