How to use numpy to interpolate between pairs of values in a list
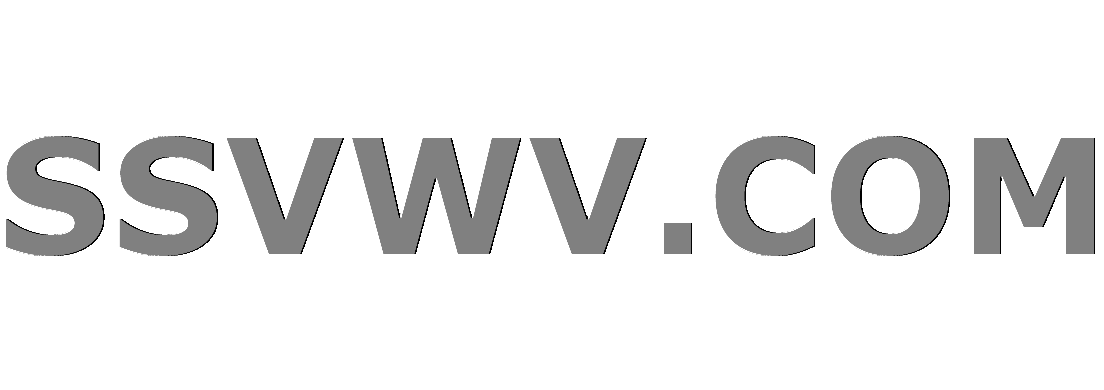
Multi tool use
I have lists like these:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
I'd like to interpolate between pairs of items in the list so that instead of have list of length 6, it's length n with equally space values between each pair of items within the list. My current approach is to use a list comprehension to go through pairs in the list and np.extend
an empty list with the results. Is there a better, ready-made function to do this?
My current approach:
import numpy as np
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
result_x =
result_y =
[result_x.extend(np.linspace(first, second, 5)) for first, second, in zip(x_data, x_data[1:])]
[result_y.extend(np.linspace(first, second, 5)) for first, second, in zip(y_data, y_data[1:])]
print(result_x, 'n'*2, result_y)
Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0, 6.5, 7.0, 7.0, 7.25, 7.5, 7.75, 8.0, 8.0, 7.25, 6.5, 5.75, 5.0, 5.0, 4.25, 3.5, 2.75, 2.0]
[15.0, 16.25, 17.5, 18.75, 20.0, 20.0, 20.5, 21.0, 21.5, 22.0, 22.0, 22.25, 22.5, 22.75, 23.0, 23.0, 22.5, 22.0, 21.5, 21.0, 21.0, 19.25, 17.5, 15.75, 14.0]
python numpy
|
show 3 more comments
I have lists like these:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
I'd like to interpolate between pairs of items in the list so that instead of have list of length 6, it's length n with equally space values between each pair of items within the list. My current approach is to use a list comprehension to go through pairs in the list and np.extend
an empty list with the results. Is there a better, ready-made function to do this?
My current approach:
import numpy as np
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
result_x =
result_y =
[result_x.extend(np.linspace(first, second, 5)) for first, second, in zip(x_data, x_data[1:])]
[result_y.extend(np.linspace(first, second, 5)) for first, second, in zip(y_data, y_data[1:])]
print(result_x, 'n'*2, result_y)
Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0, 6.5, 7.0, 7.0, 7.25, 7.5, 7.75, 8.0, 8.0, 7.25, 6.5, 5.75, 5.0, 5.0, 4.25, 3.5, 2.75, 2.0]
[15.0, 16.25, 17.5, 18.75, 20.0, 20.0, 20.5, 21.0, 21.5, 22.0, 22.0, 22.25, 22.5, 22.75, 23.0, 23.0, 22.5, 22.0, 21.5, 21.0, 21.0, 19.25, 17.5, 15.75, 14.0]
python numpy
There are the functions inscipy.interpolate
that would work. Or do you want something that is strictly numpy?
– busybear
Nov 14 '18 at 15:08
scipy is numpy under the hood
– d_kennetz
Nov 14 '18 at 15:09
1
The posted code is incorrect.y_data
is not used andresult
is used without being defined. Also the list comprehension is not assigned to any variable. Please post a working snippet so we can understand what exactly you need.
– jdehesa
Nov 14 '18 at 15:12
1
The problem with your code is that you are repeating elements when interpolating. (Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0,) To avoid that you can donp.linspace(first, second, 5)[:-1]
which will discard the last interpolated element and therefore avoid duplicates. Note that you will have to handle the very last element by adding it manually.
– Sembei Norimaki
Nov 14 '18 at 15:33
1
No, don't do this. Imagine your ranges are not monotonic, so at some point you can have a decreasing range. You might then have repeated values at different places, doingnp.unique
will then remove some needed values and giving you a hard time debugging your code.
– Sembei Norimaki
Nov 14 '18 at 15:46
|
show 3 more comments
I have lists like these:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
I'd like to interpolate between pairs of items in the list so that instead of have list of length 6, it's length n with equally space values between each pair of items within the list. My current approach is to use a list comprehension to go through pairs in the list and np.extend
an empty list with the results. Is there a better, ready-made function to do this?
My current approach:
import numpy as np
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
result_x =
result_y =
[result_x.extend(np.linspace(first, second, 5)) for first, second, in zip(x_data, x_data[1:])]
[result_y.extend(np.linspace(first, second, 5)) for first, second, in zip(y_data, y_data[1:])]
print(result_x, 'n'*2, result_y)
Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0, 6.5, 7.0, 7.0, 7.25, 7.5, 7.75, 8.0, 8.0, 7.25, 6.5, 5.75, 5.0, 5.0, 4.25, 3.5, 2.75, 2.0]
[15.0, 16.25, 17.5, 18.75, 20.0, 20.0, 20.5, 21.0, 21.5, 22.0, 22.0, 22.25, 22.5, 22.75, 23.0, 23.0, 22.5, 22.0, 21.5, 21.0, 21.0, 19.25, 17.5, 15.75, 14.0]
python numpy
I have lists like these:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
I'd like to interpolate between pairs of items in the list so that instead of have list of length 6, it's length n with equally space values between each pair of items within the list. My current approach is to use a list comprehension to go through pairs in the list and np.extend
an empty list with the results. Is there a better, ready-made function to do this?
My current approach:
import numpy as np
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
result_x =
result_y =
[result_x.extend(np.linspace(first, second, 5)) for first, second, in zip(x_data, x_data[1:])]
[result_y.extend(np.linspace(first, second, 5)) for first, second, in zip(y_data, y_data[1:])]
print(result_x, 'n'*2, result_y)
Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0, 6.5, 7.0, 7.0, 7.25, 7.5, 7.75, 8.0, 8.0, 7.25, 6.5, 5.75, 5.0, 5.0, 4.25, 3.5, 2.75, 2.0]
[15.0, 16.25, 17.5, 18.75, 20.0, 20.0, 20.5, 21.0, 21.5, 22.0, 22.0, 22.25, 22.5, 22.75, 23.0, 23.0, 22.5, 22.0, 21.5, 21.0, 21.0, 19.25, 17.5, 15.75, 14.0]
python numpy
python numpy
edited Nov 14 '18 at 15:27
Jason
asked Nov 14 '18 at 15:05
JasonJason
1,30653052
1,30653052
There are the functions inscipy.interpolate
that would work. Or do you want something that is strictly numpy?
– busybear
Nov 14 '18 at 15:08
scipy is numpy under the hood
– d_kennetz
Nov 14 '18 at 15:09
1
The posted code is incorrect.y_data
is not used andresult
is used without being defined. Also the list comprehension is not assigned to any variable. Please post a working snippet so we can understand what exactly you need.
– jdehesa
Nov 14 '18 at 15:12
1
The problem with your code is that you are repeating elements when interpolating. (Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0,) To avoid that you can donp.linspace(first, second, 5)[:-1]
which will discard the last interpolated element and therefore avoid duplicates. Note that you will have to handle the very last element by adding it manually.
– Sembei Norimaki
Nov 14 '18 at 15:33
1
No, don't do this. Imagine your ranges are not monotonic, so at some point you can have a decreasing range. You might then have repeated values at different places, doingnp.unique
will then remove some needed values and giving you a hard time debugging your code.
– Sembei Norimaki
Nov 14 '18 at 15:46
|
show 3 more comments
There are the functions inscipy.interpolate
that would work. Or do you want something that is strictly numpy?
– busybear
Nov 14 '18 at 15:08
scipy is numpy under the hood
– d_kennetz
Nov 14 '18 at 15:09
1
The posted code is incorrect.y_data
is not used andresult
is used without being defined. Also the list comprehension is not assigned to any variable. Please post a working snippet so we can understand what exactly you need.
– jdehesa
Nov 14 '18 at 15:12
1
The problem with your code is that you are repeating elements when interpolating. (Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0,) To avoid that you can donp.linspace(first, second, 5)[:-1]
which will discard the last interpolated element and therefore avoid duplicates. Note that you will have to handle the very last element by adding it manually.
– Sembei Norimaki
Nov 14 '18 at 15:33
1
No, don't do this. Imagine your ranges are not monotonic, so at some point you can have a decreasing range. You might then have repeated values at different places, doingnp.unique
will then remove some needed values and giving you a hard time debugging your code.
– Sembei Norimaki
Nov 14 '18 at 15:46
There are the functions in
scipy.interpolate
that would work. Or do you want something that is strictly numpy?– busybear
Nov 14 '18 at 15:08
There are the functions in
scipy.interpolate
that would work. Or do you want something that is strictly numpy?– busybear
Nov 14 '18 at 15:08
scipy is numpy under the hood
– d_kennetz
Nov 14 '18 at 15:09
scipy is numpy under the hood
– d_kennetz
Nov 14 '18 at 15:09
1
1
The posted code is incorrect.
y_data
is not used and result
is used without being defined. Also the list comprehension is not assigned to any variable. Please post a working snippet so we can understand what exactly you need.– jdehesa
Nov 14 '18 at 15:12
The posted code is incorrect.
y_data
is not used and result
is used without being defined. Also the list comprehension is not assigned to any variable. Please post a working snippet so we can understand what exactly you need.– jdehesa
Nov 14 '18 at 15:12
1
1
The problem with your code is that you are repeating elements when interpolating. (Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0,) To avoid that you can do
np.linspace(first, second, 5)[:-1]
which will discard the last interpolated element and therefore avoid duplicates. Note that you will have to handle the very last element by adding it manually.– Sembei Norimaki
Nov 14 '18 at 15:33
The problem with your code is that you are repeating elements when interpolating. (Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0,) To avoid that you can do
np.linspace(first, second, 5)[:-1]
which will discard the last interpolated element and therefore avoid duplicates. Note that you will have to handle the very last element by adding it manually.– Sembei Norimaki
Nov 14 '18 at 15:33
1
1
No, don't do this. Imagine your ranges are not monotonic, so at some point you can have a decreasing range. You might then have repeated values at different places, doing
np.unique
will then remove some needed values and giving you a hard time debugging your code.– Sembei Norimaki
Nov 14 '18 at 15:46
No, don't do this. Imagine your ranges are not monotonic, so at some point you can have a decreasing range. You might then have repeated values at different places, doing
np.unique
will then remove some needed values and giving you a hard time debugging your code.– Sembei Norimaki
Nov 14 '18 at 15:46
|
show 3 more comments
2 Answers
2
active
oldest
votes
I think this function does what you want using np.interp
:
import numpy as np
def interpolate_vector(data, factor):
n = len(data)
# X interpolation points. For factor=4, it is [0, 0.25, 0.5, 0.75, 1, 1.25, 1.5, ...]
x = np.linspace(0, n - 1, (n - 1) * factor + 1)
# Alternatively:
# x = np.arange((n - 1) * factor + 1) / factor
# X data points: [0, 1, 2, ...]
xp = np.arange(n)
# Interpolate
return np.interp(x, xp, np.asarray(data))
Example:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
print(interpolate_vector(x_data, 4))
# [3. 3.5 4. 4.5 5. 5.5 6. 6.5 7. 7.25 7.5 7.75 8. 7.25
# 6.5 5.75 5. 4.25 3.5 2.75 2. ]
print(interpolate_vector(y_data, 4))
# [15. 16.25 17.5 18.75 20. 20.5 21. 21.5 22. 22.25 22.5 22.75
# 23. 22.5 22. 21.5 21. 19.25 17.5 15.75 14. ]
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
add a comment |
Scipy has an interpolation functions that will easily handle this type of approach. You just provide your current data and the new "x" values that the interpolated data will be based on.
from scipy import interpolate
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
t1 = np.linspace(0, 1, len(x_data))
t2 = np.linspace(0, 1, len(y_data))
n = 50
t_new = np.linspace(0, 1, n)
f = interpolate.interp1d(t1, x_data)
x_new = f(t_new)
f = interpolate.interp1d(t2, y_data)
y_new = f(t_new)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303203%2fhow-to-use-numpy-to-interpolate-between-pairs-of-values-in-a-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think this function does what you want using np.interp
:
import numpy as np
def interpolate_vector(data, factor):
n = len(data)
# X interpolation points. For factor=4, it is [0, 0.25, 0.5, 0.75, 1, 1.25, 1.5, ...]
x = np.linspace(0, n - 1, (n - 1) * factor + 1)
# Alternatively:
# x = np.arange((n - 1) * factor + 1) / factor
# X data points: [0, 1, 2, ...]
xp = np.arange(n)
# Interpolate
return np.interp(x, xp, np.asarray(data))
Example:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
print(interpolate_vector(x_data, 4))
# [3. 3.5 4. 4.5 5. 5.5 6. 6.5 7. 7.25 7.5 7.75 8. 7.25
# 6.5 5.75 5. 4.25 3.5 2.75 2. ]
print(interpolate_vector(y_data, 4))
# [15. 16.25 17.5 18.75 20. 20.5 21. 21.5 22. 22.25 22.5 22.75
# 23. 22.5 22. 21.5 21. 19.25 17.5 15.75 14. ]
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
add a comment |
I think this function does what you want using np.interp
:
import numpy as np
def interpolate_vector(data, factor):
n = len(data)
# X interpolation points. For factor=4, it is [0, 0.25, 0.5, 0.75, 1, 1.25, 1.5, ...]
x = np.linspace(0, n - 1, (n - 1) * factor + 1)
# Alternatively:
# x = np.arange((n - 1) * factor + 1) / factor
# X data points: [0, 1, 2, ...]
xp = np.arange(n)
# Interpolate
return np.interp(x, xp, np.asarray(data))
Example:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
print(interpolate_vector(x_data, 4))
# [3. 3.5 4. 4.5 5. 5.5 6. 6.5 7. 7.25 7.5 7.75 8. 7.25
# 6.5 5.75 5. 4.25 3.5 2.75 2. ]
print(interpolate_vector(y_data, 4))
# [15. 16.25 17.5 18.75 20. 20.5 21. 21.5 22. 22.25 22.5 22.75
# 23. 22.5 22. 21.5 21. 19.25 17.5 15.75 14. ]
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
add a comment |
I think this function does what you want using np.interp
:
import numpy as np
def interpolate_vector(data, factor):
n = len(data)
# X interpolation points. For factor=4, it is [0, 0.25, 0.5, 0.75, 1, 1.25, 1.5, ...]
x = np.linspace(0, n - 1, (n - 1) * factor + 1)
# Alternatively:
# x = np.arange((n - 1) * factor + 1) / factor
# X data points: [0, 1, 2, ...]
xp = np.arange(n)
# Interpolate
return np.interp(x, xp, np.asarray(data))
Example:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
print(interpolate_vector(x_data, 4))
# [3. 3.5 4. 4.5 5. 5.5 6. 6.5 7. 7.25 7.5 7.75 8. 7.25
# 6.5 5.75 5. 4.25 3.5 2.75 2. ]
print(interpolate_vector(y_data, 4))
# [15. 16.25 17.5 18.75 20. 20.5 21. 21.5 22. 22.25 22.5 22.75
# 23. 22.5 22. 21.5 21. 19.25 17.5 15.75 14. ]
I think this function does what you want using np.interp
:
import numpy as np
def interpolate_vector(data, factor):
n = len(data)
# X interpolation points. For factor=4, it is [0, 0.25, 0.5, 0.75, 1, 1.25, 1.5, ...]
x = np.linspace(0, n - 1, (n - 1) * factor + 1)
# Alternatively:
# x = np.arange((n - 1) * factor + 1) / factor
# X data points: [0, 1, 2, ...]
xp = np.arange(n)
# Interpolate
return np.interp(x, xp, np.asarray(data))
Example:
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
print(interpolate_vector(x_data, 4))
# [3. 3.5 4. 4.5 5. 5.5 6. 6.5 7. 7.25 7.5 7.75 8. 7.25
# 6.5 5.75 5. 4.25 3.5 2.75 2. ]
print(interpolate_vector(y_data, 4))
# [15. 16.25 17.5 18.75 20. 20.5 21. 21.5 22. 22.25 22.5 22.75
# 23. 22.5 22. 21.5 21. 19.25 17.5 15.75 14. ]
edited Nov 14 '18 at 15:47
answered Nov 14 '18 at 15:39
jdehesajdehesa
24.5k43554
24.5k43554
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
add a comment |
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
I wasn't aware there is an interpolation function under numpy. Good to know!
– busybear
Nov 14 '18 at 15:44
add a comment |
Scipy has an interpolation functions that will easily handle this type of approach. You just provide your current data and the new "x" values that the interpolated data will be based on.
from scipy import interpolate
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
t1 = np.linspace(0, 1, len(x_data))
t2 = np.linspace(0, 1, len(y_data))
n = 50
t_new = np.linspace(0, 1, n)
f = interpolate.interp1d(t1, x_data)
x_new = f(t_new)
f = interpolate.interp1d(t2, y_data)
y_new = f(t_new)
add a comment |
Scipy has an interpolation functions that will easily handle this type of approach. You just provide your current data and the new "x" values that the interpolated data will be based on.
from scipy import interpolate
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
t1 = np.linspace(0, 1, len(x_data))
t2 = np.linspace(0, 1, len(y_data))
n = 50
t_new = np.linspace(0, 1, n)
f = interpolate.interp1d(t1, x_data)
x_new = f(t_new)
f = interpolate.interp1d(t2, y_data)
y_new = f(t_new)
add a comment |
Scipy has an interpolation functions that will easily handle this type of approach. You just provide your current data and the new "x" values that the interpolated data will be based on.
from scipy import interpolate
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
t1 = np.linspace(0, 1, len(x_data))
t2 = np.linspace(0, 1, len(y_data))
n = 50
t_new = np.linspace(0, 1, n)
f = interpolate.interp1d(t1, x_data)
x_new = f(t_new)
f = interpolate.interp1d(t2, y_data)
y_new = f(t_new)
Scipy has an interpolation functions that will easily handle this type of approach. You just provide your current data and the new "x" values that the interpolated data will be based on.
from scipy import interpolate
x_data = [3, 5, 7, 8, 5, 2]
y_data = [15, 20, 22, 23, 21, 14]
t1 = np.linspace(0, 1, len(x_data))
t2 = np.linspace(0, 1, len(y_data))
n = 50
t_new = np.linspace(0, 1, n)
f = interpolate.interp1d(t1, x_data)
x_new = f(t_new)
f = interpolate.interp1d(t2, y_data)
y_new = f(t_new)
answered Nov 14 '18 at 15:40
busybearbusybear
2,648824
2,648824
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303203%2fhow-to-use-numpy-to-interpolate-between-pairs-of-values-in-a-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
h4eGa ToXw73MbcfPOhNFZI,F1KNrpumr3IvTk30rG XphQBiMLWdwbXfLzrjDm2kA8n,HrM2z0kmYAZa5Xy 29YXnDkaZENVr KdQ
There are the functions in
scipy.interpolate
that would work. Or do you want something that is strictly numpy?– busybear
Nov 14 '18 at 15:08
scipy is numpy under the hood
– d_kennetz
Nov 14 '18 at 15:09
1
The posted code is incorrect.
y_data
is not used andresult
is used without being defined. Also the list comprehension is not assigned to any variable. Please post a working snippet so we can understand what exactly you need.– jdehesa
Nov 14 '18 at 15:12
1
The problem with your code is that you are repeating elements when interpolating. (Out: [3.0, 3.5, 4.0, 4.5, 5.0, 5.0, 5.5, 6.0,) To avoid that you can do
np.linspace(first, second, 5)[:-1]
which will discard the last interpolated element and therefore avoid duplicates. Note that you will have to handle the very last element by adding it manually.– Sembei Norimaki
Nov 14 '18 at 15:33
1
No, don't do this. Imagine your ranges are not monotonic, so at some point you can have a decreasing range. You might then have repeated values at different places, doing
np.unique
will then remove some needed values and giving you a hard time debugging your code.– Sembei Norimaki
Nov 14 '18 at 15:46